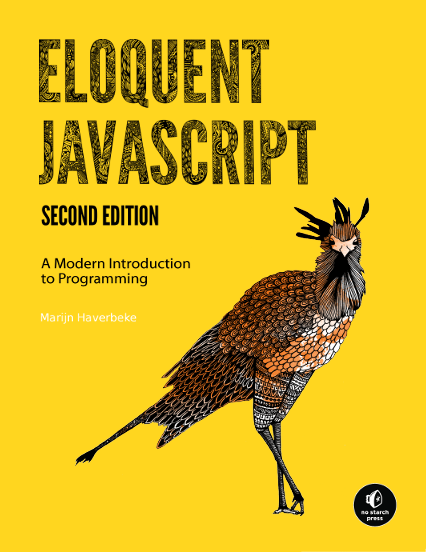
Content
This book tells how to make computers do what you want them to do. Computers today are just as common as screwdrivers - but they contain much more hidden difficulties, and therefore they are harder to understand and harder to work with. For many, they remain alien, slightly threatening things.
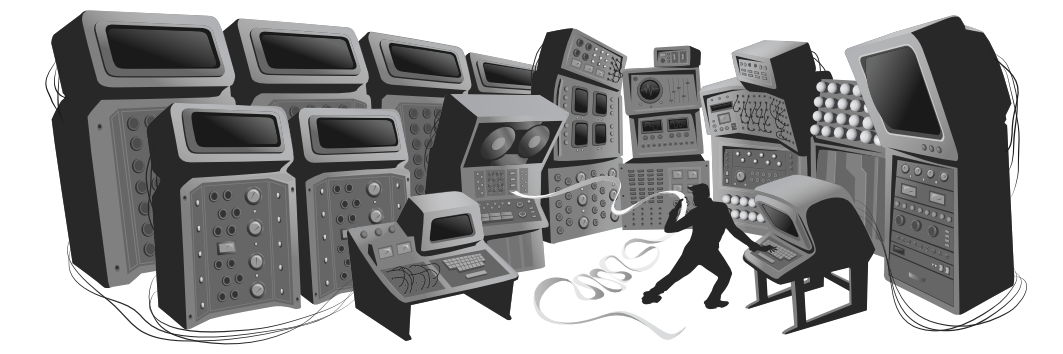
')
We found two effective ways to reduce the communication gap between us — watery biological organisms that have a talent for social connections and extensive reasoning, and computers — insensible manipulators that work with meaningless data. The first is to turn to our sense of the physical world and build interfaces that imitate it, so that we can manipulate the forms on the screen with our fingers. For a simple interaction with the computer is a good fit.
But we did not find a good way to transfer to the computer with the help of movements and mouse clicks those things that the interface designer did not provide. In order to interact with the computer at more complex levels, for example, to set arbitrary tasks for it to perform, our talent for communication is better suited: we teach the computer language.
Human languages ​​allow you to combine words in a great many ways, so that we can say so many different things. Computer languages ​​are arranged in much the same way, although less flexible grammatically.
Over the past 20 years, working with a computer has become a very common phenomenon, and interfaces that are built in a language (and this was once the only way to communicate with a computer) have almost been superseded by graphics. But they are still there - if you know where to look for them. One of these languages, JavaScript, is embedded in almost any web browser, and is therefore available on almost every computing device.
This book aims to introduce you to this language enough so that you can get the computer to do what you need.
About programming
I do not enlighten those who are not eager to learn, and do not encourage those who do not want to look for answers on their own. If I show one corner of the square, and they do not come to me with the other three, I don’t need to give repeated explanations.
ConfuciusIn addition to explaining JavaScript, I also want to explain the basic principles of programming. As it turns out, programming is hard. Usually the basic principles are simple and clear. But programs built on these principles become so complex that they introduce their own rules and levels of complexity. You build your own maze, and you can get lost in it.
Perhaps at times reading will disappoint you. If you are new to programming, you will need to digest a lot. A lot of material will be combined in such a way that you will need to establish new connections between its parts.
You yourself must justify the need for these efforts. If you find it hard to wade through a book, you don’t need to think badly of yourself. You're all right - you just need to keep moving. Take a break, go back - and always make sure that you read and understand the examples of programs. Training is a difficult job, but once you have learned something, it already belongs to you, and makes it easier to take further steps.
The programmer creates universes for which he alone is responsible. In computer programs, universes of virtually unlimited complexity can be created.
Joseph Weisenbaum, "The Power of Computers and the Mind of People"The program is a complex concept. This is a piece of text typed by a programmer, it is a directing force that causes the computer to do something, it is data in the computer’s memory, and at the same time it controls the work with the same memory. Analogies that try to compare programs with objects familiar to us usually do not cope with this. One more or less suitable - the analogy with the machine. Many of the individual parts are one, and in order to make it work, we need to imagine the ways in which these parts interact and what they bring to the work of the whole machine.
A computer is a machine that is designed to contain these intangible machines. Computers themselves can only perform simple actions. Their advantage is that they can do it very quickly. The program can combine these actions in a very tricky way so that the result is very complex actions.
For some of us, programming is an exciting game. The program is a mental construction. It costs nothing to build, it weighs nothing, and it easily grows under our fingers.
If you are not careful, size and complexity go out of control, confusing even the person who writes it. This is the main problem of programming: to maintain control over the programs. When the program works, that's great. The art of programming is the ability to control complexity. A large program is under control, and executed simply in its complexity.
Many programmers believe that this complexity is best managed by using a small set of well-known techniques in programs. They described strict rules ("best practices") of what form of the program they should have. And the most zealous among them consider those who deviate from these practices as bad programmers.
What animosity with regard to the richness of programming is to try to belittle it to something straightforward and predictable, to put a taboo on all strange and wonderful programs! The landscape of programming techniques is huge, fascinating for its diversity, and so far little has been learned. This is a dangerous journey, luring and confusing an inexperienced programmer, but this only means that you have to follow this path carefully and think with your head. As you learn, you will always meet new challenges and new uncharted territories. Programmers who do not learn new things are stagnating, forgetting their joy, they are bored with their work.
Why language matters
In the beginning, when computer disciplines were born, there were no programming languages. The programs looked like this:
00110001 00000000 00000000 00110001 00000001 00000001 00110011 00000001 00000010 01010001 00001011 00000010 00100010 00000010 00001000 01000011 00000001 00000000 01000001 00000001 00000001 00010000 00000010 00000000 01100010 00000000 00000000
This is a program that adds numbers from 1 to 10 and displays the result (1 + 2 + ... + 10 = 55). It can be performed on a very simple hypothetical machine. To program the first computers, it was necessary to install large switch arrays in the required positions, or punch holes in punch cards and feed them to the computer. You can imagine what a tedious, error-prone procedure it was. Writing even simple programs required a great deal of intelligence and discipline. Complex programs were almost unthinkable.
Of course, manual input of these mystical diagrams of bits (zeros and ones) gave the programmer the opportunity to feel like a magician. And it cost something in terms of job satisfaction.
Each line of the specified program contains one instruction. In ordinary language, they can be described as:
- write 0 to memory 0
- write 1 to memory 1
- write the value of cell 1 to cell 2
- subtract 11 from the value of cell 2
- if cell 2 has a value of 0, then continue with step 9.
- Add the value of cell 1 to cell 0
- add 1 to cell 1
- continue from point 3.
- output cell value 0
This option is easier to read than a bunch of bits, but it is still not very convenient. Using names instead of instruction numbers and memory cells can improve understanding.
'total' 0 'count' 1 [loop] 'compare' 'count' 11 'compare' 'compare' , [end] 'count' 'total' 1 'count' [loop] [end] 'total'
Now it is not so difficult to understand how the program works. Can you handle it? The first two lines assign initial values ​​to two memory areas. total will be used to calculate the result of the calculation, and count will follow the number we are working with at the moment. Lines using 'compare' are probably the strangest. The program needs to understand if count 11 is equal to stop counting. Since our imaginary machine is rather primitive, it can only perform a check for the equality of the variable to zero, and decide whether to jump to another line. Therefore, it uses a memory area called 'compare' to count the value of count - 11 and decide based on that value. The next two lines add the count value to the result counter and increment the count by 1 each time the program decides that it has not yet reached the value 11.
Here is the same JavaScript program:
var total = 0, count = 1; while (count <= 10) { total += count; count += 1; } console.log(total);
A few more improvements. The main thing - there is no need to manually mark the transitions between the lines. The while language construct does this for itself. It continues to calculate the block enclosed in braces until the condition is satisfied (count <= 10), that is, the value of count is less than or equal to 10. It is no longer necessary to create a temporary value and compare it with zero. It was boring, and the power of programming languages ​​is that they help get rid of boring details.
At the end of the program, at the end of a while, the console.log operation is applied to the result for output.
And finally, the program could look like this if we had convenient range and sum operations, which, respectively, would create a set of numbers in a given interval and calculate the sum of the set:
console.log(sum(range(1, 10)));
The moral of this fable is that the same program can be written both long and short, readable and unreadable. The first version of the program was a vague one, and the last one — almost a real language — would write down the sum of the range of numbers from 1 to 10. In the next chapters we will look at how to do such things.
A good programming language helps the programmer inform the computer about the necessary operations at a high level. Allows you to omit boring details, gives convenient building blocks (while and console.log), allows you to create your own blocks (sum and range), and makes it easy to combine blocks.
What is javascript?
JavaScript was introduced in 1995 as a way to add programs to web pages in the Netscape Navigator browser. Since then, the language has taken root in all major graphical browsers. It made it possible for modern web applications to appear - browser email clients, maps, social networks. And it is also used on more traditional sites to provide interactivity and all sorts of bells and whistles.
It is important to note that JavaScript has little to do with another language called Java. A similar name was chosen for marketing reasons. When JavaScript appeared, the Java language was widely advertised and gained popularity. Someone decided that it would be nice to cling to this locomotive. And now we will not get anywhere on this behalf.
After the language went beyond Netscape, a document was written describing the work of the language, so that different programs that declare its support work in the same way. It is called an ECMAScript standard by the name of the ECMA organization. In practice, we can talk about ECMAScript and JavaScript as the same thing.
Many people criticize JavaScript and say a lot of bad things about it. And a lot of this is true. When I first had to write a program in JavaScript, I quickly felt disgusted - the language accepted almost everything I wrote, and at the same time I interpreted it in a completely different way than I intended. This was mainly due to the fact that I had no idea what I was doing, but there was also a problem: JavaScript is too liberal. It was conceived as a simplification of programming for beginners. In reality, this makes it difficult to find problems in the program, because the system does not report them.
Flexibility has its advantages. It leaves room for different techniques, impossible in more strict languages. Sometimes, as we will see in the “modules” chapter, it can be used to overcome some of the shortcomings of the language. After I really studied and worked with him, I learned to love JavaScript.
There are already several versions of the JavaScript language. ECMAScript 3 was the dominant, common version during the language upgrade, from about 2000 to 2010. At that time, an ambitious 4th version was being prepared, in which several radical language enhancements and extensions were planned. However, political reasons made changing the lively popular language very difficult, and work on version 4 was discontinued in 2008. Instead, the less ambitious version 5 came out in 2009. Now most browsers support version 5, which we will use in the book.
JavaScript support not only browsers. Databases like MongoDB and CouchDB use it as a scripting and query language. There are several platforms for desktops and servers, the most famous of which is Node.js, provide a powerful environment for programming outside of the browser.
Code and what to do with it
The code is the text of which the programs consist. Most chapters of the book have code. Reading and writing code is an integral part of learning to program. Try not just to run through the examples - read them carefully and understand. At first it will be slow and incomprehensible, but you will quickly master the skills. The same is about exercise. Do not mean that you understand them until you write a working version.
I recommend trying your solutions in a real interpreter of the language in order to immediately receive feedback, and, I hope, be tempted to experiment further.
You can install Node.js and run programs with it. You can also do this in the browser console. Chapter 12 will explain how to embed programs in HTML pages. There are also sites like
jsbin.com , allowing you to simply run the program in the browser. On the book's website there is a
sandbox for the code .