Content
My heart shines with a bright red light under my thin, transparent skin, and they have to inject me ten cubes of JavaScript to bring me back to life (I respond well to toxins in the blood). From this garbage you at once gills turn pale!
_why, Why's (Poignant) Guide to Ruby')
In this chapter we will begin to do what can already be called programming. We will expand the use of the JavaScript language beyond nouns and fragments of sentences to more or less meaningful prose.
Expressions and instructions
In the first chapter, we created values ​​and applied operators to them, obtaining new values. This is an important part of each program, but only a part.
The code fragment, the result of which is a certain value, is called an expression. Each value written literally (for example, 22 or “psychoanalysis”) is also an expression. An expression written in parentheses is also an expression, like a binary operator applied to two expressions or unary to one.
This is part of the beauty of the language interface. Expressions can include other expressions in the same way that a complex sentence consists of simple ones. This allows us to combine expressions to create calculations of any complexity.
If the expression is a sentence fragment, the instruction is the entire sentence. A program is simply a list of instructions.
The simplest instruction is an expression with a semicolon after it. This program:
1; !false;
True, this is a useless program. An expression can only be used to get a value that can be used in another expression that covers it. The instruction is on its own and its use changes something in the program world. It can display something on the screen (change in the world), or change the internal state of the machine in such a way that it will affect the instructions following it. These changes are called side effects. The instructions in the previous example just give out the values ​​1 and true, and immediately throw them away. They have no influence on the world of the program. When you run the program, nothing noticeable happens.
In some cases, JavaScript allows you to omit the semicolon at the end of the instruction. In other cases, it is mandatory, or the next line will be regarded as part of the same instruction. The rules according to which you can or can not lower the semicolon are quite complex and increase the likelihood of error. In this book we will not lower the semicolon, and I recommend doing the same in your programs until you gain experience.
Variables
How does the program store internal state? How does she remember him? We obtained new values ​​from the old ones, but the old values ​​did not change, and the new ones needed to be used immediately, or they disappeared. To capture and store them, JavaScript offers something called a “variable.”
var caught = 5 * 5;
And this gives us a second kind of instruction. The special keyword
var
shows that in this statement we declare a variable. It is followed by the name of the variable, and if we immediately want to assign a value to it, the operator = and expression.
The example creates a variable called caught and uses it to capture the number that is obtained by multiplying 5 and 5.
After defining a variable, its name can be used in expressions. The value of the variable will be what value it currently contains. Example:
var ten = 10; console.log(ten * ten);
Variables can be called any word that is not a key word (of type var). Do not use spaces. Numbers can also be used, but not the first character in the title. Punctuation is not allowed except for $ and _.
The variable is assigned a value not permanently. Operator = can be used on existing variables at any time to assign them a new value.
var mood = ""; console.log(mood);
Imagine variables not in the form of boxes, but in the form of tentacles. They do not contain value - they grab them. Two variables can refer to one value. The program has access only to the values ​​they contain. When you need to remember something, you grow a tentacle and hold on to it, or you use an existing tentacle to hold it.

Variables like tentacles
Example. To memorize the amount of money Vasily owes you, you create a variable. Then when he pays part of the debt, you give her a new value.
var vasyaDebt = 140; vasyaDebt = vasyaDebt - 35; console.log(vasyaDebt);
When you define a variable without assigning a value to it, the tentacle has nothing to hold on to, it hangs in the air. If you request the value of an empty variable, you will get undefined.
A var statement may contain several variables. Definitions must be separated by commas.
var one = 1, two = 2; console.log(one + two);
Keyword and reserved words
Words with a special meaning, such as var - key. They cannot be used as variable names. There are also a few words “reserved for use” in future versions of JavaScript. They cannot be used either, although this is possible in some execution environments. Their full list is quite large.
break case catch continue debugger default delete do else false finally for function if implements in instanceof interface let new null package private protected public return static switch throw true try typeof var void while with yield this
You do not need to memorize them, but keep in mind that the error may lie here if your variable definitions do not work as it should.
Environment
A collection of variables and their values ​​that exists at a certain moment is called an environment. When the program starts, the environment is not empty. There are always variables that are part of the software standard, and most of the time there are variables that help interact with the surrounding system. For example, the browser has variables and functions for examining the state of the loaded web page and the effect on it, for reading the input from the mouse and keyboard.
Functions
Many values ​​from the standard environment are of type
function
(function). Function - a separate piece of the program, which can be used together with other quantities. For example, in the browser, the alert variable contains a function that shows a small window with a message. Use it like this:
alert(" !");

Alert dialog
The execution of a function is called a call. You can call a function by writing parentheses after the expression that returns the value of the function. Usually you directly use the function name as an expression. The values ​​that can be written inside parentheses are transferred to the program code inside the function. In the example, the alert function uses the string given to it for display in the dialog box. The values ​​passed to functions are called function arguments. The alert function requires one argument, but others may require a different number of arguments of different types.
Console.log function
The alert function can be used as a means of output during experiments, but you will soon get tired of closing this window every time. In the previous examples, we used the console.log function to display values. Most JavaScript systems (including all modern browsers and Node.js) provide a console.log function that outputs values ​​to any output device. In browsers, this is a JavaScript console. This part of the browser is usually hidden - most browsers show it by pressing F12, or Command-Option-I on a Mac. If that doesn't work, look in the “web console” or “developer tools” menu.
In the examples in this book, the output results are shown in the comments:
var x = 30; console.log("the value of x is", x);
Although the variable names can not be used point - it is obviously contained in the name console.log. This is because console.log is not a simple variable. This expression returns the log property of the console variable. We will talk about this in chapter 4.
Return values
Showing a dialog box or displaying text on the screen is a side effect. Many features are useful because they produce these effects. Functions can also produce values, in which case they do not need a side effect in order to be useful. For example, the Math.max function takes any number of variables and returns the largest value:
console.log(Math.max(2, 4));
When a function produces a value, it is said to return a value. Everything that produces a value is an expression, that is, function calls can be used inside complex expressions. For example, the value returned by the Math.min function (the opposite of Math.max) is used as one of the arguments of the addition operator:
console.log(Math.min(2, 4) + 100);
The next chapter describes how to write your own functions.
prompt and confirm
The browser environment contains functions other than alert that show pop-ups. You can bring up a window with a question and OK / Cancel buttons using the
confirm
function. It returns a boolean value - true if OK is pressed, and false if Cancel is clicked.
confirm(" , ?");

The
prompt
function can be used to ask an open-ended question. The first argument is the question, the second is the text from which the user starts. In the dialog box, you can enter a string of text, and the function returns it as a string.
prompt(" , .", "...");

These functions are rarely used, because you cannot change the appearance of these windows - but they can be useful for experimental programs.
Management of the program execution order
When the program has more than one instruction, they are executed from top to bottom. In this example, the program has two instructions. The first one asks the number, the second, performed by the trace, shows its square.
var theNumber = Number(prompt(" ", "")); alert(" – " + theNumber * theNumber);
The Number function converts a value to a number. We need this because the prompt returns a string. There are similar String and Boolean functions that convert values ​​to corresponding types.
A simple diagram of the direct order of execution of the program:

Conditional execution
Following the instructions in order is not the only option. Alternatively, there is a conditional implementation, where we choose from two possible paths based on a boolean value:
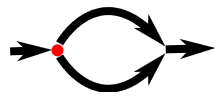
Conditional execution is recorded using the if keyword. In the simple case, we need a certain code to be executed only if some condition is met. For example, in the previous program, we can count a square only if it was just a number that was entered.
var theNumber = prompt(" ", ""); if (!isNaN(theNumber)) alert(" – " + theNumber * theNumber);
Now, having entered "cheese", you do not receive a conclusion.
The if keyword executes or skips the instruction, depending on the value of the boolean expression. This expression is written after the if in brackets, and the necessary instruction follows it.
The
isNaN
function is a standard JavaScript function that returns true only if its argument is NaN (not a number). The Number function returns NaN if you give it a string that is not a valid number. As a result, the condition goes like this: “to execute, unless theNumber is a non-number”.
Often you need to write code not only for the case when the expression is true, but also for the case when it is false. The options path is the second arrow of the diagram. The else keyword is used with if to create two separate execution paths.
var theNumber = Number(prompt(" ", "")); if (!isNaN(theNumber)) alert(" – " + theNumber * theNumber); else alert(" - ?");
If you need more different paths, you can use several if / else pairs along the chain.
var num = Number(prompt(" ", "0")); if (num < 10) alert(""); else if (num < 100) alert(""); else alert("");
The program checks if num is less than 10. If yes, selects this branch and shows “Not enough”. If not, select another - on which another if. If the following condition is met, then the number will be between 10 and 100, and “Normal” is displayed. If not, then the last branch is executed.
The sequence of execution is something like this:

While and do loops
Imagine a program that displays all even numbers from 0 to 12. You can write it like this:
console.log(0); console.log(2); console.log(4); console.log(6); console.log(8); console.log(10); console.log(12);
It works - but the point of programming is to work less than a computer, and not vice versa. If we needed all the numbers up to 1000, this solution would be unacceptable. We need the possibility of repetition. This kind of control over the order of execution is called a loop.

Looping gives you the opportunity to go back to some instructions and repeat all over again with the new state of the program. If you combine this with a variable for counting, you can do the following:
var number = 0; while (number <= 12) { console.log(number); number = number + 2; }
The statement starting with the
while
keyword is a loop. The while is followed by an expression in brackets, and then the instruction (the body of the loop) is the same as the if statement. The loop executes the instruction until the expression returns a true result.
In the loop, we need to output the value and add to it. If we need to execute several instructions in a loop, we enclose it in curly brackets {}. The braces for instructions are like parentheses for expressions. They group them and turn them into one. The sequence of instructions enclosed in braces is called a block.
Many programmers enclose any loop body in brackets. They do this for consistency, so that they do not need to add and remove parentheses if they have to change the number of instructions in the loop. In the book, I will not write brackets around single instructions in a loop, because I like brevity. You can do as you please.
The number variable shows how the variable can track program progress. With each repetition of the cycle, the number is increased by 2. Before each repetition, it is compared with 12 to see if the program has done everything that was required.
For an example of more useful work, we can write a program for calculating 2 to 10 degrees. We use two variables: one to track the result, and the second to calculate the number of multiplications. The loop checks to see if the second variable has reached 10, and then updates both.
var result = 1; var counter = 0; while (counter < 10) { result = result * 2; counter = counter + 1; } console.log(result);
You can start a counter from 1 and check it for <= 10, but for reasons that will become clear further, it is always better to start counters from 0.
The do loop is similar to the while loop. It differs only in one thing: the do loop always executes the body at least once, and checks the condition after the first execution. Therefore, the test expression is written after the body of the cycle:
do { var name = prompt("Who are you?"); } while (!name); console.log(name);
This program forces to enter a name. She asks him again and again until she gets something other than an empty string. Adding "!" turns the value into a boolean and then applies a logical negation, and all but empty lines are converted to boolean true.
You probably noticed the spaces before some instructions. In JavaScript, this is not necessary - the program will work without them. Even line breaks don't have to be done. You can write a program in one line. The role of spaces in the blocks is to separate them from the rest of the program. In complex code, where there are other blocks in blocks, it can be difficult to see where one ends and another begins. By correctly separating them with spaces, you align the appearance of the code and its blocks. I like to separate each block with two spaces, but tastes differ - some use four, some use tabulation. The more spaces you use, the more noticeable the indentation is, but the faster the nested blocks escape to the right edge of the screen.
For loops
Many cycles are built on the same pattern as in the example. A counter variable is created, then there is a while loop, where the test expression usually checks to see if we have reached a certain limit. At the end of the loop body, the counter is updated.
Since this is such a frequent case, in JavaScript there is a shorter version, the
for
loop.
for (var number = 0; number <= 12; number = number + 2) console.log(number);
This program is equivalent to the previous one. Only now all the instructions related to tracking the state of the loop are grouped.
The brackets after for contain two semicolons, dividing the instruction into three parts. The first initializes the loop, usually specifying the initial value of the variable. The second is an expression for checking whether the cycle should continue. Third - updates the state after each pass. In most cases, such a record is shorter and more understandable than a while.
Calculate 2 ^ 10 with for:
var result = 1; for (var counter = 0; counter < 10; counter = counter + 1) result = result * 2; console.log(result);
Although I did not write curly braces, I separate the body of the loop with spaces.
Exit loop
Wait until the loop condition becomes false — not the only way to end the loop. The special
break
instruction causes an immediate exit from the loop.
In the following example, we leave the cycle when we find a number greater than 20 and divisible by 7 without a remainder.
for (var current = 20; ; current++) { if (current % 7 == 0) break; } console.log(current);
The for clause does not have a check portion — so the loop will not stop until the break statement is executed.
If you do not specify this instruction, or accidentally write a condition that is always executed, the program will hang in an infinite loop and never finish the work - usually this is bad.
If you make an infinite loop, usually after a few seconds, the execution environment will prompt you to interrupt it. If not, you will have to close a bookmark, or even the entire browser.
The
continue
keyword also affects the execution of the loop. When this word occurs in a loop, it immediately moves to the next iteration.
Short variable update
Especially often in cycles, the program needs to update a variable based on its previous state.
counter = counter + 1;
There is a short entry for this in JavaScript:
counter += 1;
Similar records work for many other operators, for example, result * = 2 for doubling, or counter - = 1 for countdown.
This allows us to reduce the program output even numbers:
for (var number = 0; number <= 12; number += 2) console.log(number);
For counter + = 1 and counter - = 1 there are even shorter entries: counter ++ and counter--.
We work with variables using switch
Often the code looks like this:
if (variable == "value1") action1(); else if (variable == "value2") action2(); else if (variable == "value3") action3(); else defaultAction();
There is a construction called
switch
, which simplifies such an entry. Unfortunately, the JavaScript syntax in this case is rather strange - often the if / else chain looks better. Example:
switch (prompt(" ?")) { case "": console.log(" ."); break; case "": console.log(", !"); break; case "": console.log(" ."); case "": console.log(" ."); break; default: console.log(" !"); break; }
Any number of
case
labels can be placed in the switch block. The program jumps to the label corresponding to the value of the variable in the switch, or to the label
default
, if no suitable label is found. After that, the instructions are executed before the first
break
instruction - even if we have already passed another mark. Sometimes it can be used to execute the same code in different cases (in both cases, "sunny" and "cloudy" the program will recommend to go for a walk). However, it is very easy to forget the break entry, which will lead to the execution of an unwanted piece of code.
Name register
Variable names cannot contain spaces, but it is often convenient to use several words to clearly describe a variable. You can choose from several options:
fuzzylittleturtle fuzzy_little_turtle FuzzyLittleTurtle fuzzyLittleTurtle
The first is quite difficult to read. I like underscores, although they are not very convenient to type. Standard JavaScript functions and most programmers use the last option - each word with a capital letter, except the first.
In some cases, for example in the case of the Number function, the first letter is also written large — when you need to select a function as a constructor. We'll talk about constructors in Chapter 6. For now, just ignore this.Comments
Often the code does not contain all the information that I would like to convey to human readers, or conveys it in an incomprehensible way. Sometimes you feel poetic inspiration, or just want to share thoughts in your program. For this are comments.A comment is a text that is recorded in the program, but is ignored by the computer. In JavaScript, comments can be written in two ways. For a single-line comment, you can use two slashes: var accountBalance = calculateBalance(account);
The comment continues only to the end of the line. Code between the characters / * and * / will be ignored along with possible line breaks. This is suitable for including entire information blocks in the program: var myCity = '';
Total
Now you know that the program consists of instructions, which themselves may contain instructions. The instructions contain expressions that can consist of expressions.Writing down instructions in a row, we get a program that runs from top to bottom. You can modify this thread of execution using conditional (if, else, and switch) statements and loop statements (while, do, and for).Variables can be used to store chunks of data under a specific name and to track the status of a program. Environment - a set of defined variables. Systems executing JavaScript always add several standard variables to your environment.Functions are special variables that include parts of the program. They can be called with the functionName (argument1, argument2) command. Such a call is an expression, and can produce a value.Exercises
Each exercise begins with a description of the problem. Read and try to fulfill. In difficult situations, refer to the tips. Ready-made solutions can be found on the eloquentjavascript.net/code book site . To make learning effective, do not look at the answers until you have solved the problem yourself, or at least do not try to solve it long enough for your head to get a little sore. You can also write code directly in the browser and execute it.Triangle in a loop
Write a loop that in 7 calls to console.log displays the following triangle: # ## ### #### ##### ###### #######
It will be useful to know that the length of a string can be found by assigning it to the variable .length. var abc = "abc"; console.log(abc.length);
Fizzbuzz
Write a program that displays through console.log all the numbers from 1 to 100, with two exceptions. For numbers divisible by 3, it should output 'Fizz', and for numbers divisible by 5 (but not 3) - 'Buzz'.When you can - correct it so that it displays "FizzBuzz" for all numbers that are divisible by 3 and 5.(Actually, this question is suitable for interviews, and they say it allows you to screen out quite a large number of candidates. Therefore, when you solve this problem, you can praise yourself)Chess board
Write a program that creates a string containing an 8x8 grid in which the lines are separated by newline characters. At each position, either a space or #. The result should be a chessboard. # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # #
When done, make the size of the board variable so that you can create boards of any size.