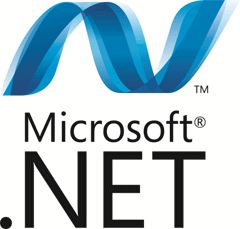
.NET 4.5 includes some changes to System.Reflection. The most significant of these is that Type is now divided into two separate classes: Type and TypeInfo. The TypeInfo object stores the full definition, and the Type itself now stores only shared data. If you use reflection from your desktop or web application under NET 4.5, then the old API is still available along with new methods of reflection. Today, I will focus on how to use some of the main features of the new API.
Type and TypeInfo overviewThe Type class provides an overview of the structure of an object. TypeInfo, on the other hand, represents the complete definition of an object, including its association with the parent and inherited classes. Moreover, the API of the Type class has been updated and now returns collections in the form of typed IEnumerable, and not as before - arrays. In the case of large and complex assemblies, this allows one to read the values of the reflection results one by one, and also simplifies the use of type metadata using LINQ. Windows Store apps have access only to these new IEnumerable collections.
')
Type API class changesLet's look at some metadata that can be obtained from Type without using TypeInfo, and then dive into the TypeInfo API. The API of the Type class allows you to get general information about types. This means that you can get metadata like name, namespace, full name, module, and so on. This is done through the same API as in .NET 4.0. For example, to get the full name and namespace of some type, you can do the following:
Type stringType = typeof(string); string fullName = stringType.FullName; string stringNameSpace = stringType.Namespace;
API TypeInfoAs you can see, Type provides an overview of the structure of a class. If you want to dive a little deeper, then you can use the new API class TypeInfo. You can get the TypeInfo value from a Type by calling its GetTypeInfo () method. Take, for example, the Person class, which contains first name properties (FirstName), last names (LastName), the Modified event, and the Save method:
public class Person { public string FirstName { get; set; } public string LastName { get; set; } public event EventHandler Modified; public void Save() {
Now let's say that you need to know which properties, methods and events are defined in the Person class. This can be easily learned from TypeInfo using its DeclaredProperties, DeclaredMethods, and DeclaredProperties properties:
TypeInfo personInfo = personType.GetTypeInfo(); IEnumerable<PropertyInfo> declaredProperties = personInfo.DeclaredProperties; IEnumerable<MethodInfo> declaredMethods = personInfo.DeclaredMethods; IEnumerable<EventInfo> declaredEvents = personInfo.DeclaredEvents;
Another common task of reflection is the need to find all types within an assembly. Using the updated System.Reflection API, the Assembly class now returns a TypeInfo collection instead of a Type array. For example, to get all the types defined in the assembly being executed, you can use the TypeInfo.Assembly property, and then read the DefinedTypes property on the resulting Assembly object:
Assembly myAssembly = this.GetType().GetTypeInfo().Assembly; IEnumerable<TypeInfo> myTypes = myAssembly.DefinedTypes;
To get a better idea of the new System.Reflection APIs, let's create an example of an application that receives a list of all types in the assembly being executed. And when we select a type, we will display its name, full name, properties, methods, and events.
Create an applicationTo begin with, create a new C # Windows Store application and add a class for Person there, as we described earlier. The next step is to open
MainPage.xaml and add
this XAML there as the root element of the Grid.
Now let's update the MainPage class so that it collects a list of the types defined in the assembly being executed. First add
using System.Reflection
to the top of the file. Then update the
OnNavigateTo
method to get the received types from the application assembly and link them to the
MyDefinedTypes
list:
protected override void OnNavigatedTo(NavigationEventArgs e) { Assembly myAssembly = this.GetType().GetTypeInfo().Assembly; IEnumerable<TypeInfo> myTypes = myAssembly.DefinedTypes; MyDefinedTypes.DataContext = myTypes; }
Now all we have left is to associate the
SelectionChanged
event of the
MyDefinedTypes
element with a handler that will show the selected TypeInfo in the
TypeInfoDetails
panel:
private void MyDefinedTypes_SelectionChanged(object sender, SelectionChangedEventArgs e) { if (e.AddedItems.Count > 0) { TypeInfo selectedTypeInfo = e.AddedItems.First() as TypeInfo; TypeInfoDetails.DataContext = selectedTypeInfo; TypeInfoDetails.Visibility = Windows.UI.Xaml.Visibility.Visible; } }
In the finished form, your MainPage class should look
like this .
Congratulations! Our application is ready and now displays the following result at runtime:
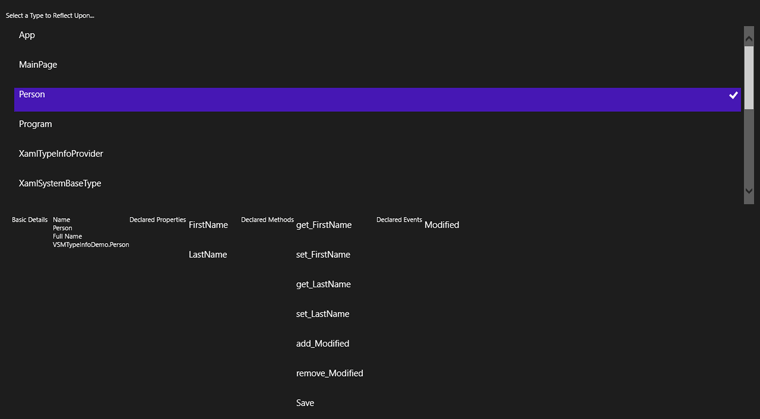
As you can see, there are quite significant changes in the Type API class. This is especially important if you are migrating from previous versions of .NET and want to create a Windows Store application in which only a new API is available. This API is also available in plain desktop and .NET 4.5 web applications, as well as in projects of the Portable Class Library. I believe that the new API has become cleaner due to the use of IEnumerable instead of arrays, as well as the separation of general and detailed reflection data into two classes: Type and TypeInfo.