<TabControl Height="400"
HorizontalAlignment="Left"
Name="Tabs"
VerticalAlignment="Top" Width="300">
/>
<Window x:Class="SimpleTwitterClient.MainWindow"
x:Name="MainWindowInstance"
xmlns:view="clr-namespace:SimpleTwitterClient.View"
xmlns:viewModel="clr-namespace:SimpleTwitterClient.ViewModel"
DataContext="{BindingViewModel,ElementName=MainWindowInstance}">
public partial class MainWindow : Window
{
private SimpleTwitterClientViewModel _viewModel;
public SimpleTwitterClientViewModel ViewModel
{
get { return _viewModel; }
set { _viewModel = value; }
}
public MainWindow()
{
_viewModel = new SimpleTwitterClientViewModel();
InitializeComponent();
}
}
public class SimpleTwitterClientViewModel
{
RecentViewModel _recentPage = new RecentViewModel();
public RecentViewModel RecentPage
{
get { return _recentPage; }
set { _recentPage = value; }
}
}
/>
<Window.Resources>
<DataTemplateDataType="{x:TypeviewModel:RecentViewModel}">
<view:RecentView />
FluentTwitter.SetClientInfo(
new TwitterClientInfo
{
ConsumerKey = Settings.Default.ConsumerKey,
ConsumerSecret = Settings.Default.ConsumerSecret
});
var twit = FluentTwitter.CreateRequest().Authentication.GetRequestToken();
var response = twit.Request();
var RequestToken = response.AsToken();
twit = twit.Authentication.AuthorizeDesktop(RequestToken.Token);
string verifier = getPinFromUser();
twit.Authentication.GetAccessToken(RequestToken.Token, verifier);
var response2 = twit.Request();
Model.OAuthHandler _oauthHandler = newModel.OAuthHandler();
public ObservableCollection Tweets
{
get; set;
}
ObservableCollection, - List View, .
. - _oauthHandler ViewModel. RecentViewModel, _oauthHandler .
, _oauthHandler , .
. LoadTweets RecentViewModel:
public void LoadTweets()
{
TwitterResult response = FluentTwitter
.CreateRequest()
.AuthenticateWith(
Settings.Default.ConsumerKey,
Settings.Default.ConsumerSecret,
Model.OAuthHandler.Token,
Model.OAuthHandler.TokenSecret)
.Statuses()
.OnHomeTimeline().AsJson().Request());
var statuses = response.AsStatuses();
foreach (TwitterStatus status in statuses)
{
Tweets.Add(status);
}
}
RecentViewModel:
public RecentViewModel(Model.OAuthHandleroauthHandler)
{
_oauthHandler = oauthHandler;
Tweets = newObservableCollection();
LoadTweets();
}
ListBox :
<ListBoxx:Name="RecentTweetList"
ItemsSource="{Binding Path=Tweets}"
IsSynchronizedWithCurrentItem="True"/>
, TweetStatus.ToString(). , ListBox , TweetStatus. , , DataTemplate UserControl.Resources. DataTemplate , TweetStatus. , TweetStatus Text, Binding Path=Text.
DataTemplate:
<DataTemplate x:Key="TweetItemTemplate">
<Grid x:Name="TTGrid">
<Grid.RowDefinitions>
/>
/>
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
/>
/>
/>
</Grid.ColumnDefinitions>
<Image Source="{Binding Path=User.ProfileImageUrl}"
Name="UserAvatarImage" />
<TextBlock
Name="ScreenNameTextBlock"
Text="{Binding Path=User.ScreenName}"
Grid.Row="1"Grid.ColumnSpan="2"/>
<TextBlock
Text="{Binding Path=Text}"
TextWrapping="Wrap"Grid.Column="1"Grid.ColumnSpan="2" />
ItemTemplate="{StaticResourceTweetItemTemplate}"
public interface IPageBase
{
void LoadTweets();
ObservableCollection Tweets { get; set; }
}
ViewModel:
ObservableCollection _pages;
public ObservableCollection Pages
{
get { return _pages; }
set { _pages = value; }
}
public SimpleTwitterClientViewModel()
{
_pages = new ObservableCollection();
_pages.Add(new RecentViewModel());
_pages.Add(new RetweetsViewModel());
foreach (var page in _pages)
{
page.LoadTweets();
}
}
View DataTemplate RetweetsPage. TabItem β tabItem ViewModel RecentPage:
/>
TabItems Pages. TabControl ItemsSource. Pages TabItems β Pages. β TabItems Header. header TabItems? Name IPageBase. -. , TabItem Header:
<TabControlName="Tabs"
ItemsSource="{Binding Pages}">
<TabControl.ItemContainerStyle>
</TabControl.ItemContainerStyle>
β RetweetsViewModel View. RetweetsView. RecentView! MVVM!
<DataTemplateDataType="{x:TypeviewModel:RetweetsViewModel}">
<view:RecentView />
. :
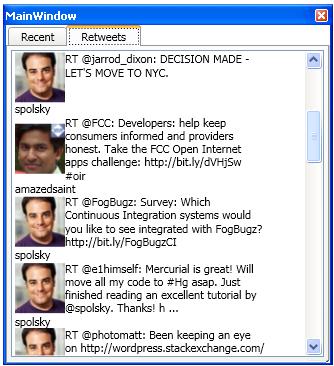
DataTemplate β ViewModel .
β follower & following.
4. ICommand
, . MVVM β OnMouseClick. . , . TextBox , :

code behind β EnterTweetTextBox.Text. MVVM . ViewModel , Send. , .
, EnterTweetTextBox . SimpleTwitterClientViewModel β Message. Text:
<TextBoxName="EnterTweetTextBox"
Text="{BindingMessage}"/>
SendTweet SimpleTwitterClientViewModel:
private void SendTweet()
{
var twitter = FluentTwitter.CreateRequest();
twitter.AuthenticateWith(
Settings.Default.ConsumerKey,
Settings.Default.ConsumerSecret,
OAuthHandler.Token,
OAuthHandler.TokenSecret);
twitter.Statuses().Update(Message);
var response = twitter.Request();
//you can verify the response here
}
β ? β Button Command, . ICommand. SendMessage, , . ? β -adapter ICommand. Josh Smith:
internal class RelayCommand : ICommand
{
#region Fields
readonly Action _execute;
readonly Func _canExecute;
#endregion
#region Constructors
public RelayCommand(Action execute)
: this(execute, null)
{
}
public RelayCommand(Action execute, Func canExecute)
{
if (execute == null)
throw new ArgumentNullException("execute");
_execute = execute;
_canExecute = canExecute;
}
#endregion // Constructors
#region ICommand Members
[DebuggerStepThrough]
public bool CanExecute(object parameter)
{
return _canExecute == null ? true : _canExecute();
}
public event EventHandler CanExecuteChanged
{
add
{
if (_canExecute != null)
CommandManager.RequerySuggested += value;
}
remove
{
if (_canExecute != null)
CommandManager.RequerySuggested -= value;
}
}
public void Execute(object parameter)
{
_execute();
}
#endregion // ICommand Members
}
RelayCoommand ICommand. , SendTweet ICommand:
RelayCommand _sendCommand;
public ICommand SendCommand
{
get
{
if (_sendCommand == null)
{
_sendCommand = new RelayCommand(() => this.SendTweet());
}
return _sendCommand;
}
}
, SendButton , SendCommand:
<Button Name="SendTweetButton"
Command="{Binding SendCommand}"/>
, ? :

, β .
, feature: userpicture, TabItem . TabItem , .
public interface IPageBase
{
void LoadTweets();
ObservableCollection Tweets { get; set; }
}
ViewModel:
ObservableCollection _pages;
public ObservableCollection Pages
{
get { return _pages; }
set { _pages = value; }
}
public SimpleTwitterClientViewModel()
{
_pages = new ObservableCollection();
_pages.Add(new RecentViewModel());
_pages.Add(new RetweetsViewModel());
foreach (var page in _pages)
{
page.LoadTweets();
}
}
View DataTemplate RetweetsPage. TabItem β tabItem ViewModel RecentPage:
/>
TabItems Pages. TabControl ItemsSource. Pages TabItems β Pages. β TabItems Header. header TabItems? Name IPageBase. -. , TabItem Header:
<TabControlName="Tabs"
ItemsSource="{Binding Pages}">
<TabControl.ItemContainerStyle>
</TabControl.ItemContainerStyle>
β RetweetsViewModel View. RetweetsView. RecentView! MVVM!
<DataTemplateDataType="{x:TypeviewModel:RetweetsViewModel}">
<view:RecentView />
. :
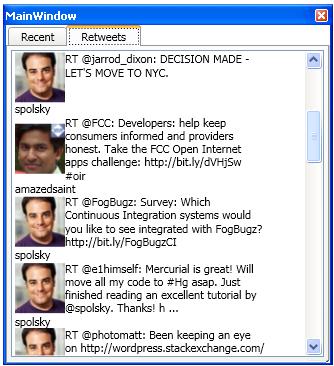
DataTemplate β ViewModel .
β follower & following.
4. ICommand
, . MVVM β OnMouseClick. . , . TextBox , :

code behind β EnterTweetTextBox.Text. MVVM . ViewModel , Send. , .
, EnterTweetTextBox . SimpleTwitterClientViewModel β Message. Text:
<TextBoxName="EnterTweetTextBox"
Text="{BindingMessage}"/>
SendTweet SimpleTwitterClientViewModel:
private void SendTweet()
{
var twitter = FluentTwitter.CreateRequest();
twitter.AuthenticateWith(
Settings.Default.ConsumerKey,
Settings.Default.ConsumerSecret,
OAuthHandler.Token,
OAuthHandler.TokenSecret);
twitter.Statuses().Update(Message);
var response = twitter.Request();
//you can verify the response here
}
β ? β Button Command, . ICommand. SendMessage, , . ? β -adapter ICommand. Josh Smith:
internal class RelayCommand : ICommand
{
#region Fields
readonly Action _execute;
readonly Func _canExecute;
#endregion
#region Constructors
public RelayCommand(Action execute)
: this(execute, null)
{
}
public RelayCommand(Action execute, Func canExecute)
{
if (execute == null)
throw new ArgumentNullException("execute");
_execute = execute;
_canExecute = canExecute;
}
#endregion // Constructors
#region ICommand Members
[DebuggerStepThrough]
public bool CanExecute(object parameter)
{
return _canExecute == null ? true : _canExecute();
}
public event EventHandler CanExecuteChanged
{
add
{
if (_canExecute != null)
CommandManager.RequerySuggested += value;
}
remove
{
if (_canExecute != null)
CommandManager.RequerySuggested -= value;
}
}
public void Execute(object parameter)
{
_execute();
}
#endregion // ICommand Members
}
RelayCoommand ICommand. , SendTweet ICommand:
RelayCommand _sendCommand;
public ICommand SendCommand
{
get
{
if (_sendCommand == null)
{
_sendCommand = new RelayCommand(() => this.SendTweet());
}
return _sendCommand;
}
}
, SendButton , SendCommand:
<Button Name="SendTweetButton"
Command="{Binding SendCommand}"/>
, ? :

, β .
, feature: userpicture, TabItem . TabItem , .
public interface IPageBase
{
void LoadTweets();
ObservableCollection Tweets { get; set; }
}
ViewModel:
ObservableCollection _pages;
public ObservableCollection Pages
{
get { return _pages; }
set { _pages = value; }
}
public SimpleTwitterClientViewModel()
{
_pages = new ObservableCollection();
_pages.Add(new RecentViewModel());
_pages.Add(new RetweetsViewModel());
foreach (var page in _pages)
{
page.LoadTweets();
}
}
View DataTemplate RetweetsPage. TabItem β tabItem ViewModel RecentPage:
/>
TabItems Pages. TabControl ItemsSource. Pages TabItems β Pages. β TabItems Header. header TabItems? Name IPageBase. -. , TabItem Header:
<TabControlName="Tabs"
ItemsSource="{Binding Pages}">
<TabControl.ItemContainerStyle>
</TabControl.ItemContainerStyle>
β RetweetsViewModel View. RetweetsView. RecentView! MVVM!
<DataTemplateDataType="{x:TypeviewModel:RetweetsViewModel}">
<view:RecentView />
. :
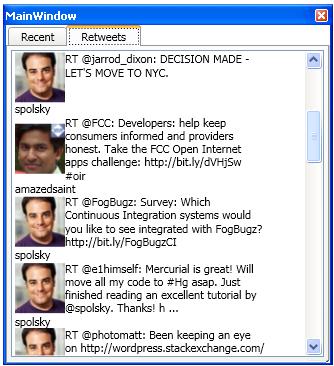
DataTemplate β ViewModel .
β follower & following.
4. ICommand
, . MVVM β OnMouseClick. . , . TextBox , :

code behind β EnterTweetTextBox.Text. MVVM . ViewModel , Send. , .
, EnterTweetTextBox . SimpleTwitterClientViewModel β Message. Text:
<TextBoxName="EnterTweetTextBox"
Text="{BindingMessage}"/>
SendTweet SimpleTwitterClientViewModel:
private void SendTweet()
{
var twitter = FluentTwitter.CreateRequest();
twitter.AuthenticateWith(
Settings.Default.ConsumerKey,
Settings.Default.ConsumerSecret,
OAuthHandler.Token,
OAuthHandler.TokenSecret);
twitter.Statuses().Update(Message);
var response = twitter.Request();
//you can verify the response here
}
β ? β Button Command, . ICommand. SendMessage, , . ? β -adapter ICommand. Josh Smith:
internal class RelayCommand : ICommand
{
#region Fields
readonly Action _execute;
readonly Func _canExecute;
#endregion
#region Constructors
public RelayCommand(Action execute)
: this(execute, null)
{
}
public RelayCommand(Action execute, Func canExecute)
{
if (execute == null)
throw new ArgumentNullException("execute");
_execute = execute;
_canExecute = canExecute;
}
#endregion // Constructors
#region ICommand Members
[DebuggerStepThrough]
public bool CanExecute(object parameter)
{
return _canExecute == null ? true : _canExecute();
}
public event EventHandler CanExecuteChanged
{
add
{
if (_canExecute != null)
CommandManager.RequerySuggested += value;
}
remove
{
if (_canExecute != null)
CommandManager.RequerySuggested -= value;
}
}
public void Execute(object parameter)
{
_execute();
}
#endregion // ICommand Members
}
RelayCoommand ICommand. , SendTweet ICommand:
RelayCommand _sendCommand;
public ICommand SendCommand
{
get
{
if (_sendCommand == null)
{
_sendCommand = new RelayCommand(() => this.SendTweet());
}
return _sendCommand;
}
}
, SendButton , SendCommand:
<Button Name="SendTweetButton"
Command="{Binding SendCommand}"/>
, ? :

, β .
, feature: userpicture, TabItem . TabItem , .
Source: https://habr.com/ru/post/111105/
All Articles