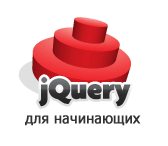
I present to you the third article in the jQuery series for beginners. This time I will try to talk about the implementation of AJAX requests ...
What is AJAX, I think you should not tell, because with the advent of the web two-zero, most users are already nosy from reloading pages completely, and with the advent of jQuery, the implementation has become simpler at times ...
Note :
In all examples, an abbreviated version of the jQuery method call is used using the $ (dollar sign) function')
Let's start with the simplest - loading HTML code into the DOM element we need on the page. For this purpose, the load method is suitable. This method can take the following parameters:
- url of the requested page
- transmitted data (optional)
- whose function will be fed the result (optional parameter)
I will give an example of javascript code:
// at the end of the page loading
$ ( document ) . ready ( function ( ) {
// hang on click on the element with id = example-1
$ ( '# example-1' ) . click ( function ( ) {
// load HTML code from example.html file
$ ( this ) . load ( 'ajax / example.html' ) ;
} )
} ) ;
Example of loadable data (the contents of the file
example.html ):
Example <br />
Data Loaded By AJAX <br />
Bye-bye
Work examplejQuery.ajax
This is the most basic method, and all subsequent methods are just wrappers for the jQuery.ajax method. This method has only one input parameter - an object that includes all the settings (the parameters that should be remembered are highlighted):
- async - asynchronous request, default true
- cache - on / off browser data caching, default true
- contentType - the default is "application / x-www-form-urlencoded"
- data - transmitted data - string or object
- dataFilter - filter for input data
- dataType - the data type returned in the callback function (xml, html, script, json, text, _default)
- global - the trigger - is responsible for using global AJAX events, true by default
- ifModified - trigger - checks if there were any changes in the server response, in order not to send another request, the default is false
- jsonp - reset the name of the callback function to work with JSONP (generated by default on the fly)
- processData - by default, the data sent is wrapped in an object, and sent as "application / x-www-form-urlencoded", if necessary otherwise - disable
- scriptCharset - encoding - relevant for JSONP and JavaScript loading
- timeout - timeout in milliseconds
- type - GET or POST
- url - url of the requested page
Local
AJAX Events :
- beforeSend - triggered before sending a request
- error - if an error occurred
- success - if no errors occurred
- complete - triggered at the end of the request
To organize HTTP authorization (O_o):
- username - login
- password - password
Example javaScript'a:
$. ajax ( {
url : '/ajax/example.html' , // specify the URL and
dataType : "json" , // type of loaded data
success : function ( data , textStatus ) { // hang our handler on the success function
$. each ( data , function ( i , val ) { // process the received data
/ * ... * /
} ) ;
}
} ) ;
jQuery.get
Loads the page using a GET request for data transfer. It can take the following parameters:
- url of the requested page
- transmitted data (optional)
- callback function to feed the result (optional)
- data type returned in the callback function (xml, html, script, json, text, _default)
This method is similar to the previous one, only the transmitted data will go to the server via POST. It can take the following parameters:
- url of the requested page
- transmitted data (optional)
- callback function to feed the result (optional)
- data type returned in the callback function (xml, html, script, json, text, _default)
Javascript:
$ ( document ) . ready ( function ( ) { // upon completion of page loading
$ ( '# example-3' ) . click ( function ( ) { // hang on the click on the element with id = example-3
$. post ( 'ajax / example.xml' , { } , function ( xml ) { // load XML from example.xml file
$ ( '# example-3' ) . html ( '' ) ;
$ ( xml ) find ( 'note' ) . each ( function ( ) { // fill the DOM element with XML data
$ ( '# example-3' ) . append ( 'To:' + $ ( this ) . find ( 'to' ) . text ( ) + '<br/>' )
. append ( 'From:' + $ ( this ) . find ( 'from' ) . text ( ) + '<br/>' )
. append ( '<b>' + $ ( this ) . find ( 'heading' ) . text ( ) + '</ b> <br/>' )
. append ( $ ( this ) . find ( 'body' ) . text ( ) + '<br/>' ) ;
} ) ;
} , 'xml' ) ; // we explicitly specify the data type
} )
} ) ;
File
example.xml :
<? xml version = "1.0" encoding = "UTF-8" ?>
<note >
<to > Tove </ to >
<from > Jani </ from >
<heading > Reminder </ heading >
<body > Don't forget me this weekend! </ body >
</ note >
Work exampleLoads data in JSON format (more convenient and faster than XML). It can take the following parameters:
- url of the requested page
- transmitted data (optional)
- callback function to feed the result (optional)
Javascript:
$ ( document ) . ready ( function ( ) { // upon completion of page loading
$ ( '# example-4' ) . click ( function ( ) { // hang on the click on the element with id = example-4
$. getJSON ( 'ajax / example.json' , { } , function ( json ) { // load JSON data from example.json
$ ( '# example-4' ) . html ( '' ) ;
// fill the DOM element with data from the JSON object
$ ( '# example-4' ) . append ( 'To:' + json. note . to + '<br/>' )
. append ( 'From:' + json. note . from + '<br/>' )
. append ( '<b>' + json. note . heading + '</ b> <br/>' )
. append ( json. note . body + '<br/>' ) ;
} ) ;
} )
} ) ;
File
example.json :
{
note : {
to : 'Tove' ,
from : 'Jani' ,
heading : 'Reminder' ,
body : 'Don \' t forget me this weekend! '
}
}
Work exampleThis function loads and executes local javascript. It can take the following parameters:
- url of the requested script
- callback function to feed the result (optional)
Javascript:
$ ( document ) . ready ( function ( ) { // upon completion of page loading
$ ( '# example-5' ) . click ( function ( ) { // hang on the click on the element with id = example-5
$. getScript ( 'ajax / example.js' , function ( ) { // loading JavaScript from example.js file
testAjax ( ) ; // execute loaded javascript
} ) ;
} )
} ) ;
File
example.js :
function testAjax ( ) {
$ ( '# example-5' ) . html ( 'Test completed' ) ; // change the element with id = example-5
}
Work exampleSubmitting a Form
To submit the form via jQuery, you can use any of the following methods, but for the convenience of “collecting” data from the form, it is better to use the
jQuery Form plugin
Sending Files
To send files via jQuery, you can use the plugin
Ajax File Upload or
One Click UploadPHP interaction
To organize work with PHP I use
jQuery-PHP library, it’s convenient if you like jQuery;), read more in the
PHP article
for jQueryJSONP usage examples
Separately, it is worth noting the use of
JSONP - for this is one of the ways to perform cross-domain data loading. If we exaggerate a little, this is the connection of remote JavaScript, which contains the necessary information in JSON format, as well as a call to our local function, the name of which we specify when accessing the remote server (usually this is the
callback parameter). Slightly more clearly this can be demonstrated by the following diagram (clickable):

When working with jQuery, the name of the callback function is automatically generated for each call to the remote server; to do this, just use the GET request in the view:
http://api.domain.com/?type=jsonp&query=test&callback=?
Instead of the last question mark (?), The name of the callback function will be substituted. If you do not want to use this method, you will need to explicitly specify the name of the callback function using the jsonp option when calling the jQuery.ajax () method.
An example of obtaining and processing search results using Google, for more information, see the article "
jQuery + AJAX + (Google Search API || Yahoo Search API) "
An example of obtaining and processing search results using Yahoo, more information can be found in the article "
jQuery + AJAX + (Google Search API || Yahoo Search API) "
JSONP API
I will also give a small list of open API with JSONP support:
Developments
For convenience of development, several event's hang on AJAX requests, they can be set for each AJAX request individually or globally. You can hang your function on all the events.
An example for displaying an element with id = "loading" during the execution of any AJAX request:
$ ( "#loading" ) . bind ( "ajaxSend" , function ( ) {
$ ( this ) . show ( ) ; // show item
} ) . bind ( "ajaxComplete" , function ( ) {
$ ( this ) . hide ( ) ; // hide item
} ) ;
For local events, we make changes to the options of the ajax () method:
$. ajax ( {
beforeSend : function ( ) {
// Handle the beforeSend event
} ,
complete : function ( ) {
// Handle the complete event
}
// ...
} ) ;
For greater clarity, give the following diagram (clickable):

Well, actually a list of all the events:
- ajaxStart - This method is called when an AJAX request ran, and there are no other requests.
- beforeSend - Fires before sending a request, allows you to edit XMLHttpRequest. Local event
- ajaxSend - Fires before sending a request, similar to beforeSend
- success - Fires when the response returns, when there are no server errors or returned data. Local event
- ajaxSuccess - Works on returning a response, similar to success
- error - Works in case of an error. Local event
- ajaxError - Fails in case of an error.
- complete - Fires when the current AJAX request is completed (with or without an error - it always works). Local event
- ajaxComplete - A global event similar to complete
- ajaxStop - This method is called when there are no more active requests
You can also download all the examples in
one archive .
Cycle of articles
- jQuery for beginners
- jQuery for beginners. Part 2. JavaScript Menu
- jQuery for beginners. Part 3. AJAX
PS For syntax highlighting I used the mini-service
http://highlight.hohli.com/