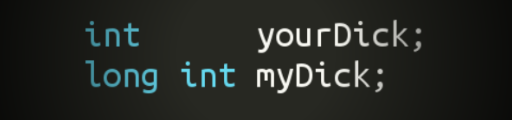
There were already several articles of the similar plan where various tricks and receptions for Unity were told. Something was quite obvious and only for beginners, something for more advanced comrades. I want to make a modest contribution.
1. Hints on variables.
')
When a small project is not relevant, but when a large project and a lot of people work on it, you can forget what this or that variable that you set in the editor is responsible for. Proper naming helps only partially, since it is too short. You can of course write the Custom Editor and do hints for each type there, but for each class it is too expensive. The following method allows to solve this problem. For this we make 2 classics:
TooltipAttribute.cs
using UnityEngine; public class TooltipAttribute : PropertyAttribute { public readonly string text; public TooltipAttribute(string text) { this.text = text; } }
TooltipDrawer.cs
#if UNITY_EDITOR using UnityEditor; using UnityEngine; [CustomPropertyDrawer(typeof(TooltipAttribute))] public class TooltipDrawer : PropertyDrawer { public override void OnGUI(Rect position, SerializedProperty prop, GUIContent label) { var atr = (TooltipAttribute) attribute; var content = new GUIContent(label.text, atr.text); EditorGUI.PropertyField(position, prop, content); } } #endif
Now, if we need the standard inspector to have a hint on the variable we need, we simply do this:
[Tooltip(" ")] public Color color; [Tooltip(" , - ")] public float speed;
Result:

For some reason, the mouse cursor is not visible in the screenshot, a hint appears when you hover.
Perfectionists, in order not to add garbage to the release code, can do this:
#if UNITY_EDITOR [Tooltip(" , - ")] #endif public float speed;
Although, in my opinion, this is unnecessary.
Now, even if in a year or two you need to correct something in the editor, you will quickly recall what kind of variable it is.
Here is the samurai collection PropertyDrawer. Comments in Japanese, but in principle everything is clear.
2. Nullable types
Sometimes it is necessary to check the value of a variable. Well, for example, like this:
public class Character : MonoBehaviour { Vector3 targetPosition; void MoveTowardsTargetPosition() { if(targetPosition != Vector3.zero) {
But what if our hero needs to come to a point (0, 0, 0)? Then this code does not fit.
You must use the nullable type. Just add the '?' at the end to the type, and to check the availability of use HasValue, and to get the value - Value.
public class Character : MonoBehaviour { //Notice the added "?" Vector3? targetPosition; void MoveTowardsTargetPosition() { if (targetPosition.HasValue) { //Move towards the target position! //use targetPosition.Value for the actual value } } public void SetTargetPosition(Vector3 newPosition) { targetPosition = newPosition; } }
3. Personal Log.
This hint can be useful for debugging AI, at least in my case it was like that. The meaning of the hint is quite simple - just in order not to dig a huge common log, we make each unit a personal one, in our case it is a string (string localLog). All the important events in the life of the monster are written there, and to view it you just need to select the monster in the editor. The code to display the personal log in the inspector:
using UnityEngine; using System.Collections; using UnityEditor; [CustomEditor(typeof(Monster))] public class MonsterEditor : Editor { Vector2 scrollPos = new Vector2(0, Mathf.Infinity); public override void OnInspectorGUI() { serializedObject.Update(); Monster monster = (Monster)target; if (Application.isPlaying) { scrollPos = GUILayout.BeginScrollView ( scrollPos, GUILayout.Height (250)); GUILayout.Label (monster.localLog); GUILayout.EndScrollView (); if (GUILayout.Button ("Clear")) monster.localLog = ""; } serializedObject.ApplyModifiedProperties(); DrawDefaultInspector(); } }
Here, in general, that's all. Now we see everything that the monster was thinking about us and other monsters. Such a simple hint makes debugging easier.
I would be glad if it helps someone.