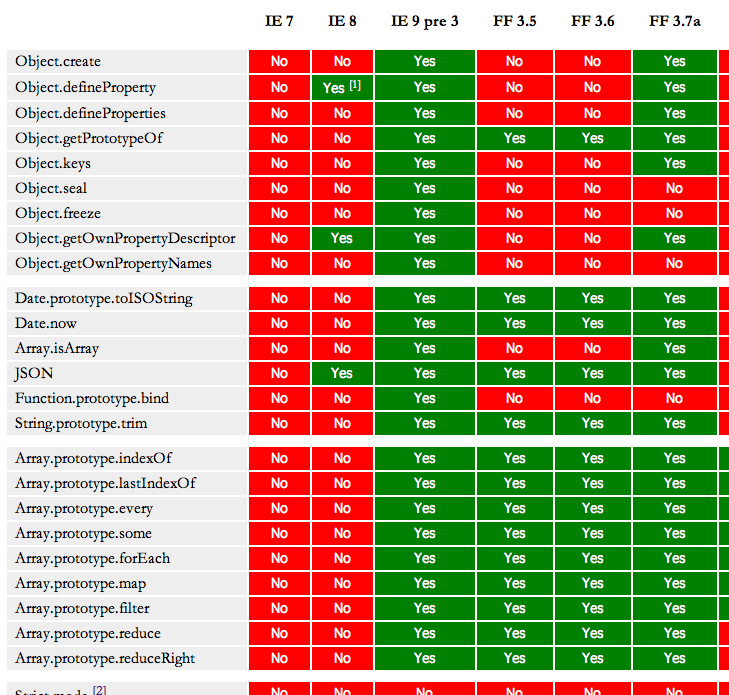
ECMAScript 5 and JScript
The biggest news is that IE9pre3 has (almost) full support for ES5. By "full support" is meant that most of the new APIs are now included, such as, for example, Object.create, Object.defineProperty, String.prototype.trim, Array.isArray, Date.now, and many other add-ons. Currently, IE9 supports the largest number of methods, even more than the latest versions of Chrome, Safari and Firefox. Incredible, isn't it? :)
Your attention is a
complete table [not] of compatibility (note that it lists the results of
obviously carried out tests, so far there are no correspondences).
It lacks only
strict strict mode, although, so far it has not actually been implemented in any of the browsers.
')
Some things you want to pay attention to:
ES5
Object.getPrototypeOf about host objects seems to be lying, constantly returning null instead of the correct [[Prototype]]:
Object. getPrototypeOf ( document. body ) ; // null
Object. getPrototypeOf ( document ) ; // null
Object. getPrototypeOf ( alert ) ; // null
Object. getPrototypeOf ( document. childNodes ) ; // null
In the latest Chrome, WebKit and Firefox browsers that implement Object.create, this will not work. For example, Chrome:
Object. getPrototypeOf ( document. body ) === HTMLBodyElement. prototype ;
Object. getPrototypeOf ( document ) === HTMLDocument. prototype ;
Object. getPrototypeOf ( alert ) === Function . prototype ;
Object. getPrototypeOf ( document. childNodes ) === NodeList. prototype
... and so on in the same vein.
Interestingly, the related functions in IE9pre3 are represented as
“function () {[native code]}” , which is similar to host objects:
var bound = ( function f ( x , y ) { return this ; } ) . bind ( { x : 1 } ) ;
bound + '' ; // "function () {[native code]}"
// versus
alert + " ; // "function alert () {[native code]}"
Note that the function view includes neither the identifier
(f) and the parameters
(x, y) , nor the function body itself
(return this;) . And this, of course, proves once again that
relying on the decompiling function is not a good idea .
The space character class (namely, / \ s /) still does not correspond to the
majority of "whitespace" characters (as provided by the specifications). These include “U + 00A0”, from “U + 2000” to “U + 200A”, “U + 3000”, and so on.
The test is available
here . It is curious that
ES5 String.prototype.trim seems to “understand” these characters as spaces very well, producing an empty string - as expected - something like
'\ u00A0'.trim () .
It's nice to see that
ES5 Array.isArray is about 20 times faster than manual processing, for example this:
function isArray ( o ) {
return object. prototype . toString . call ( o ) === "[object array]" ;
}
The difference in speed is more or less similar in other browsers that use this method.
The shameful, 10+ year old JScript NFE bug,
which was described earlier , is finally fixed:
var f = function g ( ) { return f === g ; } ;
typeof g ; // "undefined"
f ( ) ; // true
The class
arguments [[Class]] is now calculated as “Arguments”, as ES5 and limits:
var args = ( function ( ) { return arguments ; } ) ( ) ;
Object. prototype . toString . call ( args ) ; // "[object arguments]"
Dom
Unfortunately, the entire host infrastructure is still very similar to the one that is now in IE8. Host objects do not inherit
Object.prototype , do not report the correct
typeof , and do not even have such basic properties as "length" or "prototype", which all objects of function type should have:
alert instanceof Object ; // false
typeof alert ; // "object"
alert . length ; // undefined
Due to the fact that they do not inherit the
Object.prototype, we do not locate any
Object.prototype methods
in a natural way:
alert . toString ; // undefined
alert . constructor ; // undefined
alert . hasOwnProperty ; // undefined
Host objects cannot inherit the
Object.prototype . But in most modern browsers, host objects also inherit from
Function.prototype and therefore have such inherited methods from
Function.prototype , like
call and
apply . In IE9pre3 this will not happen:
alert instanceof Function ; // false
document. createElement instanceof Function ; // false
alert . call ; // undefined
It is curious that
call and
apply is present on some basic objects, but they are still not inherited from
Function.prototype :
typeof document. createElement . call ; // "function"
document. createElement . call === Function . prototype . call ; // false
The host object
[[Class]] is generally far from ideal. IE9pre3 actually violates ES5, and this says that objects implementing
[[Call]] (in other words, they are callable) should have
[[Class]] as “Function” - even if they are host objects. In IE9pre3,
alert is the called host object, so far it defines its
[[Class]] as “Object” and not “Function”, which is, to put it mildly, not good.
Object. prototype . toString . call ( alert ) ; // "[object Object]"
Object. prototype . toString . call ( document. createElement ) ; // "[object Object]"
IE9pre3 still confuses the attributes and properties of DOM objects, although not as badly as its previous versions:
var el = document. createElement ( 'p' ) ;
el. setAttribute ( 'x' , 'y' ) ;
el. x ; // 'y'
el. foobarbaz = 'moo' ;
el. hasAttribute ( 'foobarbaz' ) ; // true
el. getAttribute ( 'foobarbaz' ) ; // 'moo'
Some old,
humorous bugs can still be seen in IE9pre3, such as methods that return
"string" when typeof is used, as an example:
typeof Option. create ; // "string"
typeof Image. create ; // "string"
typeof document. childNodes . item ; // "string"
Undeclared assignments still throw an error when elements with the same id are present in the DOM, which is no longer the case with elements with the same name attribute (as it was in previous versions):
< div id = "foo" > </ div >
<a name = "bar"> </ a >
...
< script >
foo = function ( ) { / * ... * / } ; // Error
bar = function ( ) { / * ... * / } ; // no Error
</ script >
It seems that, as in IE8, only
Element and specific typed
Element interfaces (HTMLDivElement, HTMLScriptElement, HTMLSpanElement, etc.) are defined by the same global properties.
Node and
HTMLElement are still unavailable, and the element prototype chain most likely looks like this:
document. createElement ( 'div' ) ;
|
| [ [ Prototype ] ]
|
v
HTMLDivElement. prototype
|
| [ [ Prototype ] ]
|
v
Element. prototype
|
| [ [ Prototype ] ]
|
v
null
... instead of what should be in all other modern browsers:
document. createElement ( 'div' ) ;
|
| [ [ Prototype ] ]
|
v
HTMLDivElement. prototype
|
| [ [ Prototype ] ]
|
v
HTMLElement. prototype
|
| [ [ Prototype ] ]
|
v
Element. prototype
|
| [ [ Prototype ] ]
|
v
Node. prototype
|
| [ [ Prototype ] ]
|
v
Object. prototype
|
| [ [ Prototype ] ]
|
v
null
The
getComputedStyle method from DOM2 is still not available, but its value is mysteriously
null , not
undefined . That is, the property actually exists in the object, but is
null . It is hoped that this is just a stub and the correct method will be added before the final release.
document. defaultView . getComputedStyle ; // null
'getComputedStyle' in document. defaultView ; // true
The
Array.prototype.slice method
can now convert certain host objects (for example, NodeList) into arrays - something that has been done for quite a long time in most modern browsers:
Array. prototype . slice . call ( document. childNodes ) instanceof Array ; // true
That's all for now.
Personally, I don’t leave the hope that, from a web developer’s point of view, ie9 will be a good browser. And this will rather simplify our work and reduce the precious time to catch bugs. And so be it!