(Sorry for my Russian in advance)MEF is a new approach developed by Microsoft that allows you to download extensions for your application very easily. It allows you to detect and make up the parts that should be included in the application, all at runtime. You can extend the behavior of the application by simply adding new plugins. Managed Extensibility Framework will do it all for you.
"Hello MEF World!"Suppose we already have a really simple application, and we want it to greet us with "
Hello MEF world! ":
')
class program
{
static void Main (string [] args)
{
var program = new Program ();
program.Run ();
}
private void Run ()
{
ProgrammGreeter.SayHello ();
}
protected IGreeter ProgrammGreeter {get; set;}
}
public interface IGreeter
{
void SayHello ();
}
public class Greeter: IGreeter
{
public void SayHello ()
{
Console.WriteLine ("Hello MEF World!");
}
}
The main problem is that the instance is owned by
ProgrammGreeter . We wish that this would be a Greeter instance instance.
Let's do it with MEF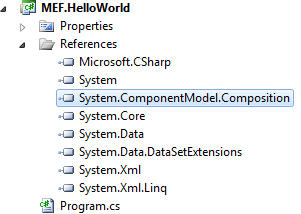
To do this, you must include a link to
System.ComponentModel.Composition .
Respectively with MSDN: “MEF is an integral part. NET Framework 4 Beta 1, and is used wherever the .NET Framework is used. ”
Most likely, MEF will be included in the NET Framework 4.0, while you can download it from the
CodePlex website
here .
After we added the link, you can add
import (Import) and
export (Export) attributes.
The export is intended to indicate some features that we want to use somewhere. And import is in favor of indicating dependencies on any features.
Our
Greeter class provides some capabilities, so we add an
Export :
[Export (typeof (IGreeter))]
public class Greeter: IGreeter
We want to use this feature in our
Programm class, i.e. we depend on this functionality. Add
Import :
[Import]
protected IGreeter ProgrammGreeter {get; set; }
Usually our capabilities live in other Assembles. But they can also live in the called assembly. In any case, we must tell the MEF how to configure all our dependencies.
This can be done using the
Compose () method, which we will call before we use our capabilities, as a rule, such a setup is done somewhere at the beginning of execution:
private void Run ()
{
Compose ();
ProgrammGreeter.SayHello ();
}
private void Compose ()
{
var assemblyCatalog = new AssemblyCatalog (Assembly.GetExecutingAssembly ());
var compositionContainer = new CompositionContainer (assemblyCatalog);
compositionContainer.ComposeParts (this);
}
I launched my program and got:
How does the Managed Extensibility Framework work?I think you are somewhat interested in the
Compose () method. Just read the comments in the code of this method, I made the method more detailed:
// We have a guide
private void Compose ()
{
// Where says exports live where
var assemblyCatalog = new AssemblyCatalog (Assembly.GetExecutingAssembly ());
// CompositionContainer holds defined dependencies and coordinates creation
var compositionContainer = new CompositionContainer (assemblyCatalog);
// CompositionBatch object holds references
var compositionBatch = new CompositionBatch ();
// one of such objects is Programm instance (this), it needs to be composed
compositionBatch.AddPart (this);
// And finally the containerall composing
compositionContainer.Compose (compositionBatch);
}
In the Managed Extensibility Framework there are primitives called
ComposablePart , which contain extensions for your application collection from the
catalog . But it can also contain dependencies that also need to be built.
Look at this chart from the Managed Extensibility Framework
page .
More complicated exampleIn this example, we will have an application that analyzes XML files (which contains information about some developers). After we have delivered our program to Clients, we want this XML to be able to check some rules, but they may differ from client to client. To do this, the rules are developed in individual Dll, which are supplied to different customers. We work with the rules as with plugins.
We have two assemblies:
- MEF.DEMO which defines ISecurityRule and IDataRule .
- MEF.Rules has the implementation of these interfaces.
The structure of the classes is as shown in the picture below. I just do not want to bore you with an explanation of the logic of all the rules. (If you need sorsy just let me know.)
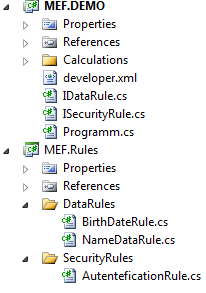
Our
Programm class wants to load rules from another assembly, so we mark them with the help of
Import in MEF.Rules:
[Import]
public ISecurityRule SecurityRule {get; set; }
[ImportMany]
public IDataRule [] DataRules {get; set; }
public void Run ()
{
Console.WriteLine ("Programm run.");
Compose ();
Console.WriteLine ("Composition completed.");
var document = XDocument.Load ("developer.xml");
Console.WriteLine (document.ToString ());
var passesValidation = SecurityRule.PassesValidation (document);
Console.WriteLine (string.Format ("Rule {0}: {1}", SecurityRule.GetType (), passesValidation));
foreach (var d in DataRules)
{
var valid = d.IsValid (document);
Console.WriteLine (string.Format ("Rule {0}: {1}", d.GetType (), valid));
}
}
As you noticed, we use
ImportMany , I hope that the name speaks for itself.
We have to change our
Compose layout method in order for it to search for Export-s in the directory where our application is located; for this we use
DirectoryCatalog .
private void Compose ()
{
var catalog = new DirectoryCatalog (Environment.CurrentDirectory);
var container = new CompositionContainer (catalog);
container.ComposeParts (this);
}
After I put these DLLs in one folder and started MEF.DEMO, I got:

The goal of MEF is to seamlessly glue together classes that import functionality from other parts of the application, as well as classes that export this functionality. Perhaps you decide that MEF is another DI container. Yes, it may still be the same as DI containers, but the Managed Extensibility Framework is primarily focused on composition. It is also important that the composition can be made during the execution of the program at any time when it is convenient for you. You can do
re-composition when you want to do. You can also load on-demand features (
Lazy load ) or add
metadata to the export. Finding dependencies in a folder can be done with
filtering . There are also many other features not found in DI containers.
Managed Extensibility Framework is a very interesting and necessary functionality that was added to the NET 4.0 Framework.
Sources:
Download MEF.DEMO.ZIP - 118.46 KB