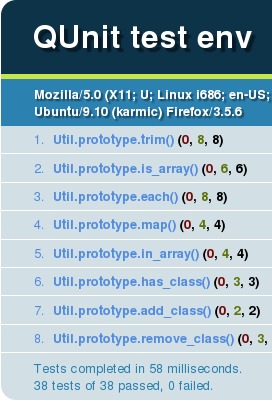
I stumbled upon this tool yesterday and could not pass by, spent the night writing tests, and now I want to share a find.
QUnit is a library from jQuery developers that allows you to write unit tests for javascript code. Easy to use, nothing superfluous, mastered in 20 minutes, the benefit from the use is enormous.
The most impatient at once links:
Official jquery documentation:
docs.jquery.com/QUnitReal Test Examples (for jquery modules):
view.jquery.com/trunk/jquery/test/unitBeginner's Guide (eng):
www.swift-lizard.com/2009/11/24/test-driven-development-with-jquery-qunitDistributed testing system (ingenious and simple):
testswarm.comUnder the cat information about the advantages of unit testing in relation to js and analysis of the possibilities of the library on examples.
Why write unit tests?
Imagine the usual development cycle: we got the task, solved it, tested it, fixed the bugs and released the version. Then we received bug reports and feature requests and embarked on a new development cycle. Upon completion of this cycle, we will again need to check that everything that has been implemented previously still works - to conduct regression testing. And it will be necessary to carry it out for each new development cycle. As the project grows, this will take more and more time. And how does regression testing occur in web projects? Click the mouse on the buttons and links. In each browser, for each feature, on each development cycle. Found a bug, corrected, refresh the page and click again, click, click.
')
Unit tests allow you to automate this routine. When implementing a feature, tests are written in parallel that verify that it functions correctly, and there will be fewer bugs in the development process right away. If an error is found, if the test did not cover it earlier, a new test is written, which will fix the error formally and never miss it in the future. Even if something goes wrong in the future, the test will immediately show errors, even before the program falls into the hands of testers.
Regarding web development there is another huge advantage - running tests under different platforms and browsers. There is no longer any need to check meticulously how this piece of code will work in msie, whether it will like the opera, and how it will be treated by safari. It is enough to write a test that checks the functionality. Moreover, this work can be distributed among ordinary users, a good example of such functionality is
testswarm.com .
How to use QUnit
It's very simple: you need two files:
QUnit.js and
QUnit.css , as well as a new html document of approximately the following content:
<! DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"
"http://www.w3.org/TR/html4/loose.dtd" >
< html >
< head >
< link rel ="stylesheet" href ="qunit.css" type ="text/css" media ="screen" >
< script type ="text/javascript" src ="qunit.js" ></ script >
<script type= "text/javascript" src= "your-code-for-testing.js" ></script>
<script type= "text/javascript" src= "your-tests.js" ></script>
</ script >
</ head >
< body >
< h1 id ="qunit-header" > QUnit test env </ h1 >
< h2 id ="qunit-banner" ></ h2 >
< h2 id ="qunit-userAgent" ></ h2 >
< ol id ="qunit-tests" >
</ ol >
</ body >
</ html >
* This source code was highlighted with Source Code Highlighter .
Now connect your code and you can write tests.
How to write tests
It is easier than it seems. Let's test the trim function, which removes spaces and tabs at the ends of a line. Here is its code:
function trim(text) {
return (text || "" ).replace(/^\s+|\s+$/g, "" );
}
* This source code was highlighted with Source Code Highlighter .
And so it can be tested:
test( 'trim()' , function () {
equals(trim( '' ), '' , ' ' );
ok(trim( ' ' ) === '' , ' ' );
same(trim(), '' , ' ' );
equals(trim( ' x' ), 'x' , ' ' );
equals(trim( 'x ' ), 'x' , ' ' );
equals(trim( ' x ' ), 'x' , ' ' );
equals(trim( ' x ' ), 'x' , '' );
equals(trim( ' x y ' ), 'x y' , ' ' );
});
* This source code was highlighted with Source Code Highlighter .
Let's sort the example line by line. In the first line of the function call test. The first parameter denotes the functionality that we are testing. The last is the testing function. Inside this function, various checks are performed. In this case, we check the consistency of the result of the function and the expected string. For checking for compliance, the following functions are used:
- equals - checks the equality of the first two parameters (weak test, only for scalar values)
- ok - the truth of the first parameter
- same - strict test for equality of the first two parameters (also checks the equality of two arrays and objects)
The last parameter of the function is the test case description.
As a result of this check we get the following picture:

All tests passed.
How to test ajax? Asynchronous checks.
With synchronous functions easy. And what about asynchronous? Obviously, for asynchrony, we have to stop the normal flow of control and resume it at the end of the test. This is done with the stop () and start () functions. Here is a simple example:
test( 'async' , function () {
//
stop();
setTimeout( function () {
ok( true );
//
//
start();
}, 500);
});
* This source code was highlighted with Source Code Highlighter .
Not to call stop () every time; The following function option is provided:
asyncTest( 'async' , function () {
//
setTimeout( function () {
ok( true );
//
start();
}, 500);
});
* This source code was highlighted with Source Code Highlighter .
And what if you need to call several asynchronous checks in one test? When in this case "start" the flow? The solution is:
asyncTest( 'asynctest' , function () {
// Pause the test
expect(3);
$.get( function () {
//
ok( true );
});
$.get( function () {
//
ok( true );
ok( true );
});
setTimeout( function () {
start();
}, 2000);
});
* This source code was highlighted with Source Code Highlighter .
The stream starts in 2 seconds. During this time, three checks must pass (the expect call reports this to the testing program). By the way, instead of calling expect, you can pass the second numeric parameter of the test (asyncTest) function. The behavior will be identical to the previous example.
Test grouping by modules
QUnit allows you to group tests by module. To do this, simply call the module function ('Name of the module or group of tests') immediately before calling the tests. It's comfortable.
Summary
That's basically all you need to start testing your code automatically. For more information contact here:
docs.jquery.com/QUnitVery good examples of tests can be found
here (these are
tests for core jquery).
Thanks for attention.