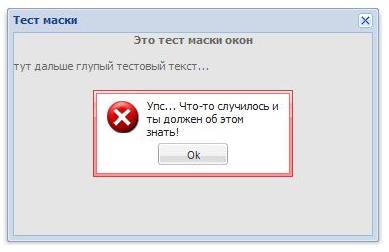
Hello, Habrachelovek! Now I’ll tell you about a small Ext.Window extension from ExtJS, a popular JavaScript framework in some circles. This extension is designed to block a separate window and display an error message.
I hope that you know what ExtJS is, but not much better than me, otherwise you will not be interested ...
')
There is a component in ExtJS -
Ext.Window , which is a window. And it has a body (property) -
body , which has a method of
mask () , because it is
Ext.Element . This method covers the window with a veil, hinting the user to wait for something. You can also display some text that explains what you actually have to wait. To do this, pass a message string to the method.
So, I needed a method that would report an error just for this window, without acting on other parts of the interface. But at the same time, it required a user response, i.e. so that the user has no suspicion that “something is not working”, but he knew “yeah, this is not working and that’s why!”. This method should be easily called and smartly work out, and at the same time be part of the window class. Because there may be many windows running at the same time, then the standard error
reporting tools (
Ext.MessageBox ), due to their modal operation, did not even fit me closely.
Having rummaged in the bowels of
mask () and the reverse
unmask () , it was decided to expand the window class using its own method based on these two. After seeing how
mask ( str ) works, I realized that it doesn’t really suit me, because does not provide finished containers. But thanks to the trepanning of this method, I figured out the CSS dies.

Without hesitation, I inserted a panel in which I comfortably placed a message line, an error icon and the “Ok” button:
new Ext.Panel({
renderTo: SomeDom,
width: 200,
height: 80,
border: false ,
cls: 'win-error-mask' ,
bodyCfg: {cls: 'win-error-mask-icon' },
html: ' . ...' ,
buttonAlign: 'center' ,
buttons: [ new Ext.Button({
text: 'Ok' ,
width: 70,
handler: function (){
}
})]
}
);
After experimenting with the panel I got the display I needed, and then I started writing CSS for it based on what I saw in mask. In fact, everything was ready, it was necessary only to pull it onto the panel and change colors. There were 4 such modified clones:
.win-error-mask {
z-index : 20001 ;
position : absolute ;
top : 0 ;
left : 0 ;
border : 1px solid ;
background : repeat-x 0 -16px ;
padding : 2px ;
border-color : #e03939 ;
background-color : #fe9295 ;
background-image : url(resources/tb-red.gif) ;
}
.win-error-mask div.x-panel-bwrap {
padding : 5px 10px 0px 10px ;
border : 1px solid ;
border-color : #f86c6c ;
background-color : #fff ;
}
.win-error-mask div {
background-color : #fff ;
color : #222 ;
font : normal 11px tahoma, arial, helvetica, sans-serif ;
}
.win-error-mask-icon {
background : transparent no-repeat center left ;
background-image : url(resources/icon-error.gif) ;
padding-left : 40px ;
padding-bottom : 36px ;
}
I note that I started screwing these CSS on a simple panel while it was not yet part of the
Ext.Window class
extension , since ExtJS is particularly relevant in the ascending development model when implementing logic blocks.
Then it was a matter of small things - to wrap all this in a function and stick it to the class window. The ExtJS website has a lot of documentation on working with classes and OOP in ExtJS, so if something is not clear,
RTFM . And here is my result with a couple of comments:
Ext.ux.Window = Ext.extend(Ext.Window, {
errorMaskPanel: null , //
errorMask: function (errorMsg){
this .body.mask(); //
this .errorMaskPanel = new Ext.Panel({ // ...
renderTo: this .body.dom, // mask(str)
width: 200,
height: 80,
border: false ,
cls: 'win-error-mask' ,
bodyCfg: {
cls: 'win-error-mask-icon'
},
html: errorMsg,
buttonAlign: 'center' ,
buttons: [ new Ext.Button({
text: 'Ok' ,
width: 70,
handler: function (){ // Ok
this .body.unmask();
this .errorMaskPanel.destroy();
},
scope: this //
})]
});
this .errorMaskPanel.el.center( this .body.dom); // ...
}
});
* yes, I packed everything into my Window class in ux space, rather than replacing the standard Ext.WindowThat's so easy and simple (maybe somewhere else is crooked) the base classes ExtJS are extended.