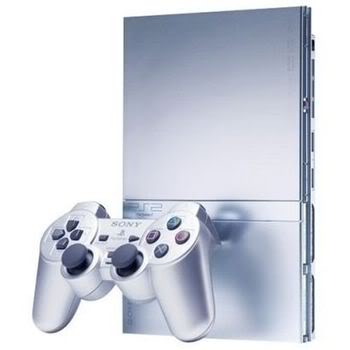
In the summer, a friend threw a couple of orders for development for Android. The first is a streaming video player for one French television, the second is a simple toy.
During development (my first development experience on Android and Java), I understood a few rules that need to be followed for the correct and stable operation of the programs I want to share ...
Perhaps for professionals the article will not be interesting, but for beginners who first encountered the development of mobile devices, the information will be useful.
Examples
For clarity, I created a small project in which a few examples of the rules are given below.
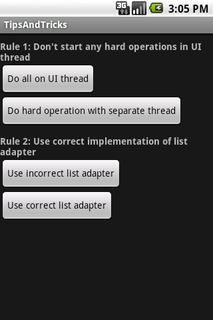
Sources:
fileshare.in.ua/3050399APK:
fileshare.in.ua/3050402Rule 1. Do not perform complex operations in the UI stream.

Performing any complicated operation in the UI thread will cause the UI to be inaccessible to the user (i.e., it will “hang”). At the same time, any actions of the user, during such “hang-ups”, may lead to the appearance of a message stating that the application has hung, with a proposal to close it.
Therefore, all complex calculations should be made in a separate thread.
')
For example:See how you do not need to do, and what it can lead to by clicking the "Do all on UI thread" button. At the moment of clicking, a child Activity is created, which has a pseudo-complex operation in the onCreate () method, which takes 20 seconds. After pressing, when you try to press the phone’s Back button, a message pops up saying that the application is “frozen”.
When you click the “Do hard operation with separate thread” button of the main window, an Activity will be created and a thread will be created to perform a complex operation.
Rule 2. Use adapters correctly
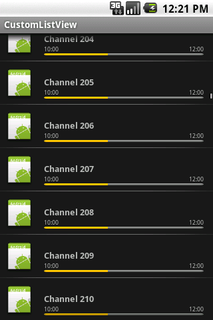
When working with non-standard lists, the creation of strings occurs by means of a hidden call to the getView () method of the adapter set for this list.
In the getView () adapter, the developer is given the opportunity to create a string of any kind. For example:
Example:How not to do:@Override
public View getView( int position, View convertView, ViewGroup parent) {
final View view = mInflater.inflate(R.layout.row, null );
TextView tv_programm = (TextView) view.findViewById(R.id.channel_proramm);
TextView tv_starttime = (TextView) view.findViewById(R.id.time_start);
TextView tv_endtime = (TextView) view.findViewById(R.id.time_end);
ImageView ib_logo = (ImageView) view.findViewById(R.id.channel_logo);
ProgressBar pb_progress = (ProgressBar) view.findViewById(R.id.programm_progress);
tv_programm.setFocusable( false );
tv_starttime.setFocusable( false );
tv_endtime.setFocusable( false );
ib_logo.setFocusable( false );
pb_progress.setFocusable( false );
ib_logo.setClickable( false );
final String name = mNames[position];
if ( name != null ) {
tv_programm.setText(name);
}
return view;
}
* This source code was highlighted with Source Code Highlighter .
Here, for each row in the list, a new View object is created. As a result, when scrolling through the list, we will get memory clogged with objects that are not displayed. And therefore the Garbage Collector will be called more often (see rule 3).
How to do it:private class ViewHolder {
TextView tv_programm;
TextView tv_starttime;
TextView tv_endtime;
ImageView ib_logo;
ProgressBar pb_progress;
}
@Override
public View getView( int position, View convertView, ViewGroup parent) {
ViewHolder holder = null ;
if ( convertView == null ) {
convertView = mInflater.inflate(R.layout.row, null );
holder = new ViewHolder();
holder.tv_programm = (TextView) convertView.findViewById(R.id.channel_proramm);
holder.tv_starttime = (TextView) convertView.findViewById(R.id.time_start);
holder.tv_endtime = (TextView) convertView.findViewById(R.id.time_end);
holder.ib_logo = (ImageView) convertView.findViewById(R.id.channel_logo);
holder.pb_progress = (ProgressBar) convertView.findViewById(R.id.programm_progress);
holder.tv_programm.setFocusable( false );
holder.tv_starttime.setFocusable( false );
holder.tv_endtime.setFocusable( false );
holder.ib_logo.setFocusable( false );
holder.pb_progress.setFocusable( false );
holder.ib_logo.setClickable( false );
convertView.setTag(holder);
} else {
holder = (ViewHolder) convertView.getTag();
}
final String name = mNames[position];
if ( name != null ) {
holder.tv_programm.setText(name);
}
return convertView;
}
* This source code was highlighted with Source Code Highlighter .
In this example, only the number of objects that will fit on the screen will be created. And for drawing new lines of the list, objects that are not currently displayed will be used. That is, the objects will be reused, without creating new ones.
What is the gain?In the example for rule 2 (the “Use incorrect list adapter” and “Use correct list adapter” buttons), a list of 1000 lines is created.
When launched, the application uses 3% of the total device memory.
When scrolling through the list with incorrect use of the adapter, you need an additional 14% of the device's memory to scroll through the list to the last item. At the same time drawing is jerky.
If the adapter is used correctly, it is additionally used <= 1% and flipping passes smoothly.
Rule 3. Less memory allocation (Java and Garbage Collector)
This rule is probably familiar to every Java developer. But since I previously programmed in C ++, the Garbage Collector (aka GC) was new to me.
The more often objects are created and deleted, the more often GC is called. And each GC call takes 100-200ms. In this case, all threads for the duration of the GC stop. In this case, frame drawing may be noticeable (sometimes <10 fps).
Conclusion:
This is only a small part of what I managed to learn while working with Android, and if someone is interested in the topic, I will write the next batch of tips and tricks.
Useful links:
android-developers.blogspot.com - Android developer blog
developer.android.com/videos/index.html - Android Development Video
I especially advise you to look at:
Google I / O 2009 - Make your Android UI Fast and Efficient. - Rule 2 is taken from here.
Google I / O 2009 - Writing Real-Time Games for Android. - Rule 3.
UPD: thanks for the karma, transferred to Android
UPD2: thanks to i-
home for another useful link
small-coding.blogspot.com in the
comments