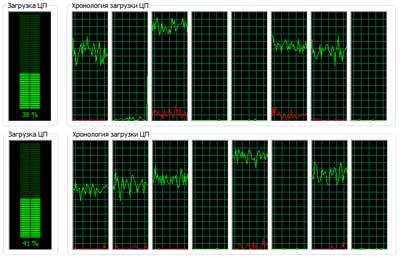
After the release of 10.1, the pre-release version decided to test it on drawTriangles ()
Written an application that in 800x600 draws 2700 spinning textured triangles (texture 32x32 random perlin noise)
Compiled for flash 10.0 and flash 10.1.
Compiled under flex builder 3, playerglobal.swc under 10.1 is taken from the official page
http://labs.adobe.com/downloads/flashplayer10.htmlDrivers for video delivered from the post
http://habrahabr.ru/blogs/adobe/75532/Core i7 920 + gtx295 system
www.rexxar.ru/stress10 (build under 10.0.xxx)
flash 10.0 - an average of 22 fps (debug) / 25 fps (release)
flash 10.1 - an average of 36 fps
www.rexxar.ru/stress101 (build under 10.1.xxx)
flash 10.0 - clearly not working, 10.1 needed
flash 10.1 - an average of 36 fps
By the way, in 10.1 version under opera / firefox on an empty clip, fps is cut to 120, although 200 are written in a flash. In 10.0 there is no such thing.
Sources of both applications -
www.rexxar.ru/data/stress.zipWell, here I will lay out, there are only two classes.
Main:
package {
import flash.display.Sprite;
[SWF(frameRate= "200" , width= "800" , height= "600" )]
public class stress101 extends Sprite
{
private static const NUM_COLS : int = 60;
private static const NUM_ROWS : int = 45;
private var canvas : Sprite = new Sprite();
public function stress101()
{
canvas.graphics.beginFill(0xDDDDFF);
canvas.graphics.drawRect(0, 0, stage.stageWidth, stage.stageHeight);
canvas.graphics.endFill();
addChild(canvas);
addChild( new FPSCounter());
drawTriangles();
}
private function drawTriangles() : void
{
const dx : Number = canvas.width / NUM_COLS;
const dy : Number = canvas.height / NUM_ROWS;
const size : Number = 0.5 * (dx > dy ? dx : dy);
var cy : Number = 0.5 * dy;
for ( var row : int = 0; row < NUM_ROWS; row++, cy += dy)
{
var cx : Number = 0.5 * dx;
for ( var col : int = 0; col < NUM_COLS; col++, cx += dx)
{
var triangle : Triangle = new Triangle(size);
triangle.x = cx;
triangle.y = cy;
canvas.addChild(triangle);
}
}
}
}
}
* This source code was highlighted with Source Code Highlighter .
')
Triangle:
package
{
import flash.display.BitmapData;
import flash.display.Shape;
import flash.events.Event;
public class Triangle extends Shape
{
private static const TEXTURE_WIDTH : int = 32;
private static const TEXTURE_HEIGHT : int = 32;
public function Triangle(size : Number)
{
super();
var bitmapData : BitmapData = new BitmapData(TEXTURE_WIDTH, TEXTURE_HEIGHT, false );
bitmapData.perlinNoise(TEXTURE_WIDTH, TEXTURE_HEIGHT, 6, Math.random() * int .MAX_VALUE, false , true );
graphics.beginBitmapFill(bitmapData, null , false , true );
graphics.drawTriangles(
Vector.<Number>([
0, -size,
size, size,
-size, size,
]),
Vector.< int >([
0, 1, 2,
]),
Vector.<Number>([
0.5, 0.0, 0.5,
1.0, 1.0, 0.5,
0.0, 1.0, 0.5,
])
);
graphics.endFill();
addEventListener(Event.ENTER_FRAME, onEnterFrame);
}
private function onEnterFrame( event : Event) : void
{
rotation += 1.0;
}
}
}
* This source code was highlighted with Source Code Highlighter .
So - either I am doing something wrong or one of the two. Persistent googling did not give any clues.
Of course, it is nice to increase the performance by ~ 1.6 times, but the tree-stick, 36fps by 2700 triangles is the 3dfx level of Voodoo.
Even for the test, you can go to
http://www.rexxar.ru/3d/ (flash player 10.0.xx required)
To start poking into the flash drive, otherwise I forget to rearrange the focus on it, without this, the keys will not work. And further:
key 1 - beginFill + moveTo / lineTo
key 2 - beginBitmapFill + moveTo / lineTo
key 3 - beginBitmapFill + drawTriangles
key 4 - beginShaderFill (3-point bilinear gradient) + drawTriangles
key 5 - beginBitmapFill (3-point bilinear gradient emulation with 2x2 texture) + drawTriangles
keys w + s + a + d - change the number of triangles along the axes
Now I tested it myself - everything was as it was (
The shy hopes that the pixel-bender shader will somehow compile will work under CUDA and allow me to draw EVERYTHING in general, they are covered either with my inability or with a copper basin.
It's sad all.
ps
Explanation of the picture in the title:
the first one is flash player 10.0, 3-point gradient shader, triangles are typed up to ~ 32fps, the full one is
http://www.rexxar.ru/img/flash100.jpgthe second is flash player 10.1, 3-point gradient shader, triangles are typed up to ~ 32fps, the full one is
http://www.rexxar.ru/img/flash101.jpgThe use of kernels is very strange.
First, 5, a little more triangles - 8, you go back - 4, again more - 6.