var obstacle_map:Array=[];
for ( var i: int =0; i<5; i++) {
obstacle_map[i]=[];
for ( var j: int =0; j<5; j++) {
obstacle_map[i][j]= false ;
}
}
2) , true.
obstacle_map[0][0]= true ;
obstacle_map[1][1]= true ;
obstacle_map[2][1]= true ;
obstacle_map[2][3]= true ;
obstacle_map[3][3]= true ;
obstacle_map[4][3]= true ;
obstacle_map[0][4]= true ;
, 2 β .

2 β
, , AStar.
β
, , , :
var a_star:AStar= new AStar();
a_star a_star.ERROR:
a_star.addEventListener(a_star.ERROR,onError);
, . onError. , , :
private function onError(e:Event): void {
trace(a_star.lastError);
}
, a_star, lastError. β . 3 output . , , .

3 β
:
a_star.setObstacleMap(obstacle_map);
! AStar.ERROR AStar, . , . setObstacleMap - , lastError . , β , . , , .. , , , , AStar, .
Event.COMPLETE:
a_star.addEventListener(Event.COMPLETE,onComplete);
onComplete , clippingType ( ):
a_star.clippingType=<>
, : a_star.F ( full), a_star.P ( prevent) a_star.N ( none). a_star.N , . ? , . . 4 S (start) , f (finish) .
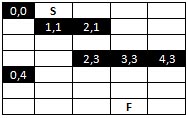
4 β
clippingType (. 5)

5. β clippingType=a_star.N .
5. β , . clippingType=a_star.P . : , ( , 3D ) , , . , «» , . 6, , β .
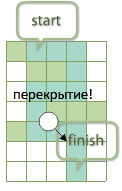
6 β
clippingType=a_star.P ( ) , . , , .
5. , β clippingType=a_star.F . .. .
, . , :
AStar.findPath(_startx:int,_starty:int,_targetx:int,_targety:int):void
{0,1} {6, 3}. findPath:
a_star.findPath(0,1,6,3);
, Event.COMPLETE (, ?), a_star . a_star my_path:Array:
private function onComplete(e:Event): void {
my_path=a_star.getPath();
}
, , . { _x: < >, _y: < >}. , 5.:
my_path=[
{_x:0,_y:1},
{_x:1,_y:0},
{_x:1,_y:2},
{_x:1,_y:3},
{_x:2,_y:4},
{_x:3,_y:5},
{_x:3,_y:6}
];
. , .
var obstacle_map:Array=[];
for ( var i: int =0; i<5; i++) {
obstacle_map[i]=[];
for ( var j: int =0; j<5; j++) {
obstacle_map[i][j]= false ;
}
}
2) , true.
obstacle_map[0][0]= true ;
obstacle_map[1][1]= true ;
obstacle_map[2][1]= true ;
obstacle_map[2][3]= true ;
obstacle_map[3][3]= true ;
obstacle_map[4][3]= true ;
obstacle_map[0][4]= true ;
, 2 β .

2 β
, , AStar.
β
, , , :
var a_star:AStar= new AStar();
a_star a_star.ERROR:
a_star.addEventListener(a_star.ERROR,onError);
, . onError. , , :
private function onError(e:Event): void {
trace(a_star.lastError);
}
, a_star, lastError. β . 3 output . , , .

3 β
:
a_star.setObstacleMap(obstacle_map);
! AStar.ERROR AStar, . , . setObstacleMap - , lastError . , β , . , , .. , , , , AStar, .
Event.COMPLETE:
a_star.addEventListener(Event.COMPLETE,onComplete);
onComplete , clippingType ( ):
a_star.clippingType=<>
, : a_star.F ( full), a_star.P ( prevent) a_star.N ( none). a_star.N , . ? , . . 4 S (start) , f (finish) .
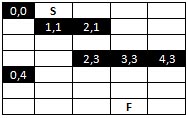
4 β
clippingType (. 5)

5. β clippingType=a_star.N .
5. β , . clippingType=a_star.P . : , ( , 3D ) , , . , «» , . 6, , β .
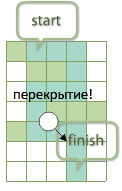
6 β
clippingType=a_star.P ( ) , . , , .
5. , β clippingType=a_star.F . .. .
, . , :
AStar.findPath(_startx:int,_starty:int,_targetx:int,_targety:int):void
{0,1} {6, 3}. findPath:
a_star.findPath(0,1,6,3);
, Event.COMPLETE (, ?), a_star . a_star my_path:Array:
private function onComplete(e:Event): void {
my_path=a_star.getPath();
}
, , . { _x: < >, _y: < >}. , 5.:
my_path=[
{_x:0,_y:1},
{_x:1,_y:0},
{_x:1,_y:2},
{_x:1,_y:3},
{_x:2,_y:4},
{_x:3,_y:5},
{_x:3,_y:6}
];
. , .
var obstacle_map:Array=[];
for ( var i: int =0; i<5; i++) {
obstacle_map[i]=[];
for ( var j: int =0; j<5; j++) {
obstacle_map[i][j]= false ;
}
}
2) , true.
obstacle_map[0][0]= true ;
obstacle_map[1][1]= true ;
obstacle_map[2][1]= true ;
obstacle_map[2][3]= true ;
obstacle_map[3][3]= true ;
obstacle_map[4][3]= true ;
obstacle_map[0][4]= true ;
, 2 β .

2 β
, , AStar.
β
, , , :
var a_star:AStar= new AStar();
a_star a_star.ERROR:
a_star.addEventListener(a_star.ERROR,onError);
, . onError. , , :
private function onError(e:Event): void {
trace(a_star.lastError);
}
, a_star, lastError. β . 3 output . , , .

3 β
:
a_star.setObstacleMap(obstacle_map);
! AStar.ERROR AStar, . , . setObstacleMap - , lastError . , β , . , , .. , , , , AStar, .
Event.COMPLETE:
a_star.addEventListener(Event.COMPLETE,onComplete);
onComplete , clippingType ( ):
a_star.clippingType=<>
, : a_star.F ( full), a_star.P ( prevent) a_star.N ( none). a_star.N , . ? , . . 4 S (start) , f (finish) .
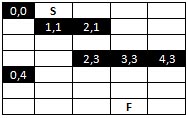
4 β
clippingType (. 5)

5. β clippingType=a_star.N .
5. β , . clippingType=a_star.P . : , ( , 3D ) , , . , «» , . 6, , β .
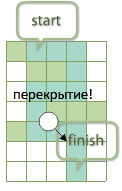
6 β
clippingType=a_star.P ( ) , . , , .
5. , β clippingType=a_star.F . .. .
, . , :
AStar.findPath(_startx:int,_starty:int,_targetx:int,_targety:int):void
{0,1} {6, 3}. findPath:
a_star.findPath(0,1,6,3);
, Event.COMPLETE (, ?), a_star . a_star my_path:Array:
private function onComplete(e:Event): void {
my_path=a_star.getPath();
}
, , . { _x: < >, _y: < >}. , 5.:
my_path=[
{_x:0,_y:1},
{_x:1,_y:0},
{_x:1,_y:2},
{_x:1,_y:3},
{_x:2,_y:4},
{_x:3,_y:5},
{_x:3,_y:6}
];
. , .
var obstacle_map:Array=[];
for ( var i: int =0; i<5; i++) {
obstacle_map[i]=[];
for ( var j: int =0; j<5; j++) {
obstacle_map[i][j]= false ;
}
}
2) , true.
obstacle_map[0][0]= true ;
obstacle_map[1][1]= true ;
obstacle_map[2][1]= true ;
obstacle_map[2][3]= true ;
obstacle_map[3][3]= true ;
obstacle_map[4][3]= true ;
obstacle_map[0][4]= true ;
, 2 β .

2 β
, , AStar.
β
, , , :
var a_star:AStar= new AStar();
a_star a_star.ERROR:
a_star.addEventListener(a_star.ERROR,onError);
, . onError. , , :
private function onError(e:Event): void {
trace(a_star.lastError);
}
, a_star, lastError. β . 3 output . , , .

3 β
:
a_star.setObstacleMap(obstacle_map);
! AStar.ERROR AStar, . , . setObstacleMap - , lastError . , β , . , , .. , , , , AStar, .
Event.COMPLETE:
a_star.addEventListener(Event.COMPLETE,onComplete);
onComplete , clippingType ( ):
a_star.clippingType=<>
, : a_star.F ( full), a_star.P ( prevent) a_star.N ( none). a_star.N , . ? , . . 4 S (start) , f (finish) .
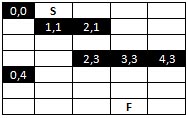
4 β
clippingType (. 5)

5. β clippingType=a_star.N .
5. β , . clippingType=a_star.P . : , ( , 3D ) , , . , «» , . 6, , β .
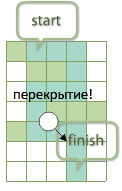
6 β
clippingType=a_star.P ( ) , . , , .
5. , β clippingType=a_star.F . .. .
, . , :
AStar.findPath(_startx:int,_starty:int,_targetx:int,_targety:int):void
{0,1} {6, 3}. findPath:
a_star.findPath(0,1,6,3);
, Event.COMPLETE (, ?), a_star . a_star my_path:Array:
private function onComplete(e:Event): void {
my_path=a_star.getPath();
}
, , . { _x: < >, _y: < >}. , 5.:
my_path=[
{_x:0,_y:1},
{_x:1,_y:0},
{_x:1,_y:2},
{_x:1,_y:3},
{_x:2,_y:4},
{_x:3,_y:5},
{_x:3,_y:6}
];
. , .
var obstacle_map:Array=[];
for ( var i: int =0; i<5; i++) {
obstacle_map[i]=[];
for ( var j: int =0; j<5; j++) {
obstacle_map[i][j]= false ;
}
}
2) , true.
obstacle_map[0][0]= true ;
obstacle_map[1][1]= true ;
obstacle_map[2][1]= true ;
obstacle_map[2][3]= true ;
obstacle_map[3][3]= true ;
obstacle_map[4][3]= true ;
obstacle_map[0][4]= true ;
, 2 β .

2 β
, , AStar.
β
, , , :
var a_star:AStar= new AStar();
a_star a_star.ERROR:
a_star.addEventListener(a_star.ERROR,onError);
, . onError. , , :
private function onError(e:Event): void {
trace(a_star.lastError);
}
, a_star, lastError. β . 3 output . , , .

3 β
:
a_star.setObstacleMap(obstacle_map);
! AStar.ERROR AStar, . , . setObstacleMap - , lastError . , β , . , , .. , , , , AStar, .
Event.COMPLETE:
a_star.addEventListener(Event.COMPLETE,onComplete);
onComplete , clippingType ( ):
a_star.clippingType=<>
, : a_star.F ( full), a_star.P ( prevent) a_star.N ( none). a_star.N , . ? , . . 4 S (start) , f (finish) .
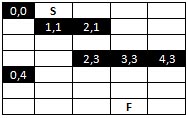
4 β
clippingType (. 5)

5. β clippingType=a_star.N .
5. β , . clippingType=a_star.P . : , ( , 3D ) , , . , «» , . 6, , β .
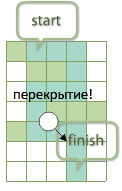
6 β
clippingType=a_star.P ( ) , . , , .
5. , β clippingType=a_star.F . .. .
, . , :
AStar.findPath(_startx:int,_starty:int,_targetx:int,_targety:int):void
{0,1} {6, 3}. findPath:
a_star.findPath(0,1,6,3);
, Event.COMPLETE (, ?), a_star . a_star my_path:Array:
private function onComplete(e:Event): void {
my_path=a_star.getPath();
}
, , . { _x: < >, _y: < >}. , 5.:
my_path=[
{_x:0,_y:1},
{_x:1,_y:0},
{_x:1,_y:2},
{_x:1,_y:3},
{_x:2,_y:4},
{_x:3,_y:5},
{_x:3,_y:6}
];
. , .
var obstacle_map:Array=[];
for ( var i: int =0; i<5; i++) {
obstacle_map[i]=[];
for ( var j: int =0; j<5; j++) {
obstacle_map[i][j]= false ;
}
}
2) , true.
obstacle_map[0][0]= true ;
obstacle_map[1][1]= true ;
obstacle_map[2][1]= true ;
obstacle_map[2][3]= true ;
obstacle_map[3][3]= true ;
obstacle_map[4][3]= true ;
obstacle_map[0][4]= true ;
, 2 β .

2 β
, , AStar.
β
, , , :
var a_star:AStar= new AStar();
a_star a_star.ERROR:
a_star.addEventListener(a_star.ERROR,onError);
, . onError. , , :
private function onError(e:Event): void {
trace(a_star.lastError);
}
, a_star, lastError. β . 3 output . , , .

3 β
:
a_star.setObstacleMap(obstacle_map);
! AStar.ERROR AStar, . , . setObstacleMap - , lastError . , β , . , , .. , , , , AStar, .
Event.COMPLETE:
a_star.addEventListener(Event.COMPLETE,onComplete);
onComplete , clippingType ( ):
a_star.clippingType=<>
, : a_star.F ( full), a_star.P ( prevent) a_star.N ( none). a_star.N , . ? , . . 4 S (start) , f (finish) .
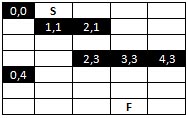
4 β
clippingType (. 5)

5. β clippingType=a_star.N .
5. β , . clippingType=a_star.P . : , ( , 3D ) , , . , «» , . 6, , β .
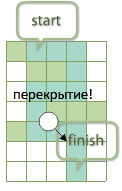
6 β
clippingType=a_star.P ( ) , . , , .
5. , β clippingType=a_star.F . .. .
, . , :
AStar.findPath(_startx:int,_starty:int,_targetx:int,_targety:int):void
{0,1} {6, 3}. findPath:
a_star.findPath(0,1,6,3);
, Event.COMPLETE (, ?), a_star . a_star my_path:Array:
private function onComplete(e:Event): void {
my_path=a_star.getPath();
}
, , . { _x: < >, _y: < >}. , 5.:
my_path=[
{_x:0,_y:1},
{_x:1,_y:0},
{_x:1,_y:2},
{_x:1,_y:3},
{_x:2,_y:4},
{_x:3,_y:5},
{_x:3,_y:6}
];
. , .
var obstacle_map:Array=[];
for ( var i: int =0; i<5; i++) {
obstacle_map[i]=[];
for ( var j: int =0; j<5; j++) {
obstacle_map[i][j]= false ;
}
}
2) , true.
obstacle_map[0][0]= true ;
obstacle_map[1][1]= true ;
obstacle_map[2][1]= true ;
obstacle_map[2][3]= true ;
obstacle_map[3][3]= true ;
obstacle_map[4][3]= true ;
obstacle_map[0][4]= true ;
, 2 β .

2 β
, , AStar.
β
, , , :
var a_star:AStar= new AStar();
a_star a_star.ERROR:
a_star.addEventListener(a_star.ERROR,onError);
, . onError. , , :
private function onError(e:Event): void {
trace(a_star.lastError);
}
, a_star, lastError. β . 3 output . , , .

3 β
:
a_star.setObstacleMap(obstacle_map);
! AStar.ERROR AStar, . , . setObstacleMap - , lastError . , β , . , , .. , , , , AStar, .
Event.COMPLETE:
a_star.addEventListener(Event.COMPLETE,onComplete);
onComplete , clippingType ( ):
a_star.clippingType=<>
, : a_star.F ( full), a_star.P ( prevent) a_star.N ( none). a_star.N , . ? , . . 4 S (start) , f (finish) .
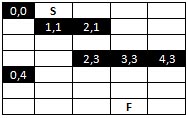
4 β
clippingType (. 5)

5. β clippingType=a_star.N .
5. β , . clippingType=a_star.P . : , ( , 3D ) , , . , «» , . 6, , β .
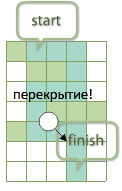
6 β
clippingType=a_star.P ( ) , . , , .
5. , β clippingType=a_star.F . .. .
, . , :
AStar.findPath(_startx:int,_starty:int,_targetx:int,_targety:int):void
{0,1} {6, 3}. findPath:
a_star.findPath(0,1,6,3);
, Event.COMPLETE (, ?), a_star . a_star my_path:Array:
private function onComplete(e:Event): void {
my_path=a_star.getPath();
}
, , . { _x: < >, _y: < >}. , 5.:
my_path=[
{_x:0,_y:1},
{_x:1,_y:0},
{_x:1,_y:2},
{_x:1,_y:3},
{_x:2,_y:4},
{_x:3,_y:5},
{_x:3,_y:6}
];
. , .
var obstacle_map:Array=[];
for ( var i: int =0; i<5; i++) {
obstacle_map[i]=[];
for ( var j: int =0; j<5; j++) {
obstacle_map[i][j]= false ;
}
}
2) , true.
obstacle_map[0][0]= true ;
obstacle_map[1][1]= true ;
obstacle_map[2][1]= true ;
obstacle_map[2][3]= true ;
obstacle_map[3][3]= true ;
obstacle_map[4][3]= true ;
obstacle_map[0][4]= true ;
, 2 β .

2 β
, , AStar.
β
, , , :
var a_star:AStar= new AStar();
a_star a_star.ERROR:
a_star.addEventListener(a_star.ERROR,onError);
, . onError. , , :
private function onError(e:Event): void {
trace(a_star.lastError);
}
, a_star, lastError. β . 3 output . , , .

3 β
:
a_star.setObstacleMap(obstacle_map);
! AStar.ERROR AStar, . , . setObstacleMap - , lastError . , β , . , , .. , , , , AStar, .
Event.COMPLETE:
a_star.addEventListener(Event.COMPLETE,onComplete);
onComplete , clippingType ( ):
a_star.clippingType=<>
, : a_star.F ( full), a_star.P ( prevent) a_star.N ( none). a_star.N , . ? , . . 4 S (start) , f (finish) .
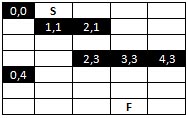
4 β
clippingType (. 5)

5. β clippingType=a_star.N .
5. β , . clippingType=a_star.P . : , ( , 3D ) , , . , «» , . 6, , β .
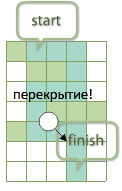
6 β
clippingType=a_star.P ( ) , . , , .
5. , β clippingType=a_star.F . .. .
, . , :
AStar.findPath(_startx:int,_starty:int,_targetx:int,_targety:int):void
{0,1} {6, 3}. findPath:
a_star.findPath(0,1,6,3);
, Event.COMPLETE (, ?), a_star . a_star my_path:Array:
private function onComplete(e:Event): void {
my_path=a_star.getPath();
}
, , . { _x: < >, _y: < >}. , 5.:
my_path=[
{_x:0,_y:1},
{_x:1,_y:0},
{_x:1,_y:2},
{_x:1,_y:3},
{_x:2,_y:4},
{_x:3,_y:5},
{_x:3,_y:6}
];
. , .
var obstacle_map:Array=[];
for ( var i: int =0; i<5; i++) {
obstacle_map[i]=[];
for ( var j: int =0; j<5; j++) {
obstacle_map[i][j]= false ;
}
}
2) , true.
obstacle_map[0][0]= true ;
obstacle_map[1][1]= true ;
obstacle_map[2][1]= true ;
obstacle_map[2][3]= true ;
obstacle_map[3][3]= true ;
obstacle_map[4][3]= true ;
obstacle_map[0][4]= true ;
, 2 β .

2 β
, , AStar.
β
, , , :
var a_star:AStar= new AStar();
a_star a_star.ERROR:
a_star.addEventListener(a_star.ERROR,onError);
, . onError. , , :
private function onError(e:Event): void {
trace(a_star.lastError);
}
, a_star, lastError. β . 3 output . , , .

3 β
:
a_star.setObstacleMap(obstacle_map);
! AStar.ERROR AStar, . , . setObstacleMap - , lastError . , β , . , , .. , , , , AStar, .
Event.COMPLETE:
a_star.addEventListener(Event.COMPLETE,onComplete);
onComplete , clippingType ( ):
a_star.clippingType=<>
, : a_star.F ( full), a_star.P ( prevent) a_star.N ( none). a_star.N , . ? , . . 4 S (start) , f (finish) .
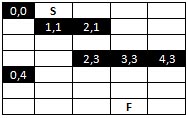
4 β
clippingType (. 5)

5. β clippingType=a_star.N .
5. β , . clippingType=a_star.P . : , ( , 3D ) , , . , «» , . 6, , β .
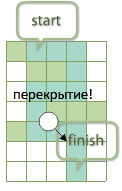
6 β
clippingType=a_star.P ( ) , . , , .
5. , β clippingType=a_star.F . .. .
, . , :
AStar.findPath(_startx:int,_starty:int,_targetx:int,_targety:int):void
{0,1} {6, 3}. findPath:
a_star.findPath(0,1,6,3);
, Event.COMPLETE (, ?), a_star . a_star my_path:Array:
private function onComplete(e:Event): void {
my_path=a_star.getPath();
}
, , . { _x: < >, _y: < >}. , 5.:
my_path=[
{_x:0,_y:1},
{_x:1,_y:0},
{_x:1,_y:2},
{_x:1,_y:3},
{_x:2,_y:4},
{_x:3,_y:5},
{_x:3,_y:6}
];
. , .
var obstacle_map:Array=[];
for ( var i: int =0; i<5; i++) {
obstacle_map[i]=[];
for ( var j: int =0; j<5; j++) {
obstacle_map[i][j]= false ;
}
}
2) , true.
obstacle_map[0][0]= true ;
obstacle_map[1][1]= true ;
obstacle_map[2][1]= true ;
obstacle_map[2][3]= true ;
obstacle_map[3][3]= true ;
obstacle_map[4][3]= true ;
obstacle_map[0][4]= true ;
, 2 β .

2 β
, , AStar.
β
, , , :
var a_star:AStar= new AStar();
a_star a_star.ERROR:
a_star.addEventListener(a_star.ERROR,onError);
, . onError. , , :
private function onError(e:Event): void {
trace(a_star.lastError);
}
, a_star, lastError. β . 3 output . , , .

3 β
:
a_star.setObstacleMap(obstacle_map);
! AStar.ERROR AStar, . , . setObstacleMap - , lastError . , β , . , , .. , , , , AStar, .
Event.COMPLETE:
a_star.addEventListener(Event.COMPLETE,onComplete);
onComplete , clippingType ( ):
a_star.clippingType=<>
, : a_star.F ( full), a_star.P ( prevent) a_star.N ( none). a_star.N , . ? , . . 4 S (start) , f (finish) .
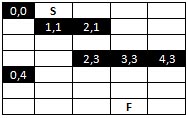
4 β
clippingType (. 5)

5. β clippingType=a_star.N .
5. β , . clippingType=a_star.P . : , ( , 3D ) , , . , «» , . 6, , β .
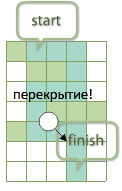
6 β
clippingType=a_star.P ( ) , . , , .
5. , β clippingType=a_star.F . .. .
, . , :
AStar.findPath(_startx:int,_starty:int,_targetx:int,_targety:int):void
{0,1} {6, 3}. findPath:
a_star.findPath(0,1,6,3);
, Event.COMPLETE (, ?), a_star . a_star my_path:Array:
private function onComplete(e:Event): void {
my_path=a_star.getPath();
}
, , . { _x: < >, _y: < >}. , 5.:
my_path=[
{_x:0,_y:1},
{_x:1,_y:0},
{_x:1,_y:2},
{_x:1,_y:3},
{_x:2,_y:4},
{_x:3,_y:5},
{_x:3,_y:6}
];
. , .
var obstacle_map:Array=[];
for ( var i: int =0; i<5; i++) {
obstacle_map[i]=[];
for ( var j: int =0; j<5; j++) {
obstacle_map[i][j]= false ;
}
}
2) , true.
obstacle_map[0][0]= true ;
obstacle_map[1][1]= true ;
obstacle_map[2][1]= true ;
obstacle_map[2][3]= true ;
obstacle_map[3][3]= true ;
obstacle_map[4][3]= true ;
obstacle_map[0][4]= true ;
, 2 β .

2 β
, , AStar.
β
, , , :
var a_star:AStar= new AStar();
a_star a_star.ERROR:
a_star.addEventListener(a_star.ERROR,onError);
, . onError. , , :
private function onError(e:Event): void {
trace(a_star.lastError);
}
, a_star, lastError. β . 3 output . , , .

3 β
:
a_star.setObstacleMap(obstacle_map);
! AStar.ERROR AStar, . , . setObstacleMap - , lastError . , β , . , , .. , , , , AStar, .
Event.COMPLETE:
a_star.addEventListener(Event.COMPLETE,onComplete);
onComplete , clippingType ( ):
a_star.clippingType=<>
, : a_star.F ( full), a_star.P ( prevent) a_star.N ( none). a_star.N , . ? , . . 4 S (start) , f (finish) .
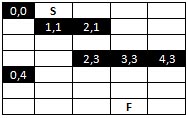
4 β
clippingType (. 5)

5. β clippingType=a_star.N .
5. β , . clippingType=a_star.P . : , ( , 3D ) , , . , «» , . 6, , β .
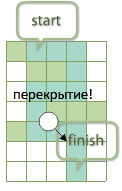
6 β
clippingType=a_star.P ( ) , . , , .
5. , β clippingType=a_star.F . .. .
, . , :
AStar.findPath(_startx:int,_starty:int,_targetx:int,_targety:int):void
{0,1} {6, 3}. findPath:
a_star.findPath(0,1,6,3);
, Event.COMPLETE (, ?), a_star . a_star my_path:Array:
private function onComplete(e:Event): void {
my_path=a_star.getPath();
}
, , . { _x: < >, _y: < >}. , 5.:
my_path=[
{_x:0,_y:1},
{_x:1,_y:0},
{_x:1,_y:2},
{_x:1,_y:3},
{_x:2,_y:4},
{_x:3,_y:5},
{_x:3,_y:6}
];
. , .
var obstacle_map:Array=[];
for ( var i: int =0; i<5; i++) {
obstacle_map[i]=[];
for ( var j: int =0; j<5; j++) {
obstacle_map[i][j]= false ;
}
}
2) , true.
obstacle_map[0][0]= true ;
obstacle_map[1][1]= true ;
obstacle_map[2][1]= true ;
obstacle_map[2][3]= true ;
obstacle_map[3][3]= true ;
obstacle_map[4][3]= true ;
obstacle_map[0][4]= true ;
, 2 β .

2 β
, , AStar.
β
, , , :
var a_star:AStar= new AStar();
a_star a_star.ERROR:
a_star.addEventListener(a_star.ERROR,onError);
, . onError. , , :
private function onError(e:Event): void {
trace(a_star.lastError);
}
, a_star, lastError. β . 3 output . , , .

3 β
:
a_star.setObstacleMap(obstacle_map);
! AStar.ERROR AStar, . , . setObstacleMap - , lastError . , β , . , , .. , , , , AStar, .
Event.COMPLETE:
a_star.addEventListener(Event.COMPLETE,onComplete);
onComplete , clippingType ( ):
a_star.clippingType=<>
, : a_star.F ( full), a_star.P ( prevent) a_star.N ( none). a_star.N , . ? , . . 4 S (start) , f (finish) .
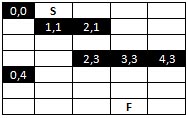
4 β
clippingType (. 5)

5. β clippingType=a_star.N .
5. β , . clippingType=a_star.P . : , ( , 3D ) , , . , «» , . 6, , β .
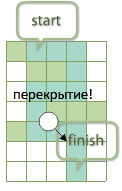
6 β
clippingType=a_star.P ( ) , . , , .
5. , β clippingType=a_star.F . .. .
, . , :
AStar.findPath(_startx:int,_starty:int,_targetx:int,_targety:int):void
{0,1} {6, 3}. findPath:
a_star.findPath(0,1,6,3);
, Event.COMPLETE (, ?), a_star . a_star my_path:Array:
private function onComplete(e:Event): void {
my_path=a_star.getPath();
}
, , . { _x: < >, _y: < >}. , 5.:
my_path=[
{_x:0,_y:1},
{_x:1,_y:0},
{_x:1,_y:2},
{_x:1,_y:3},
{_x:2,_y:4},
{_x:3,_y:5},
{_x:3,_y:6}
];
. , .
var obstacle_map:Array=[];
for ( var i: int =0; i<5; i++) {
obstacle_map[i]=[];
for ( var j: int =0; j<5; j++) {
obstacle_map[i][j]= false ;
}
}
2) , true.
obstacle_map[0][0]= true ;
obstacle_map[1][1]= true ;
obstacle_map[2][1]= true ;
obstacle_map[2][3]= true ;
obstacle_map[3][3]= true ;
obstacle_map[4][3]= true ;
obstacle_map[0][4]= true ;
, 2 β .

2 β
, , AStar.
β
, , , :
var a_star:AStar= new AStar();
a_star a_star.ERROR:
a_star.addEventListener(a_star.ERROR,onError);
, . onError. , , :
private function onError(e:Event): void {
trace(a_star.lastError);
}
, a_star, lastError. β . 3 output . , , .

3 β
:
a_star.setObstacleMap(obstacle_map);
! AStar.ERROR AStar, . , . setObstacleMap - , lastError . , β , . , , .. , , , , AStar, .
Event.COMPLETE:
a_star.addEventListener(Event.COMPLETE,onComplete);
onComplete , clippingType ( ):
a_star.clippingType=<>
, : a_star.F ( full), a_star.P ( prevent) a_star.N ( none). a_star.N , . ? , . . 4 S (start) , f (finish) .
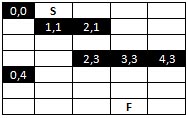
4 β
clippingType (. 5)

5. β clippingType=a_star.N .
5. β , . clippingType=a_star.P . : , ( , 3D ) , , . , «» , . 6, , β .
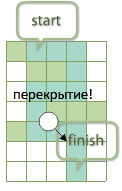
6 β
clippingType=a_star.P ( ) , . , , .
5. , β clippingType=a_star.F . .. .
, . , :
AStar.findPath(_startx:int,_starty:int,_targetx:int,_targety:int):void
{0,1} {6, 3}. findPath:
a_star.findPath(0,1,6,3);
, Event.COMPLETE (, ?), a_star . a_star my_path:Array:
private function onComplete(e:Event): void {
my_path=a_star.getPath();
}
, , . { _x: < >, _y: < >}. , 5.:
my_path=[
{_x:0,_y:1},
{_x:1,_y:0},
{_x:1,_y:2},
{_x:1,_y:3},
{_x:2,_y:4},
{_x:3,_y:5},
{_x:3,_y:6}
];
. , .
var obstacle_map:Array=[];
for ( var i: int =0; i<5; i++) {
obstacle_map[i]=[];
for ( var j: int =0; j<5; j++) {
obstacle_map[i][j]= false ;
}
}
2) , true.
obstacle_map[0][0]= true ;
obstacle_map[1][1]= true ;
obstacle_map[2][1]= true ;
obstacle_map[2][3]= true ;
obstacle_map[3][3]= true ;
obstacle_map[4][3]= true ;
obstacle_map[0][4]= true ;
, 2 β .

2 β
, , AStar.
β
, , , :
var a_star:AStar= new AStar();
a_star a_star.ERROR:
a_star.addEventListener(a_star.ERROR,onError);
, . onError. , , :
private function onError(e:Event): void {
trace(a_star.lastError);
}
, a_star, lastError. β . 3 output . , , .

3 β
:
a_star.setObstacleMap(obstacle_map);
! AStar.ERROR AStar, . , . setObstacleMap - , lastError . , β , . , , .. , , , , AStar, .
Event.COMPLETE:
a_star.addEventListener(Event.COMPLETE,onComplete);
onComplete , clippingType ( ):
a_star.clippingType=<>
, : a_star.F ( full), a_star.P ( prevent) a_star.N ( none). a_star.N , . ? , . . 4 S (start) , f (finish) .
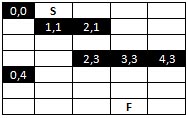
4 β
clippingType (. 5)

5. β clippingType=a_star.N .
5. β , . clippingType=a_star.P . : , ( , 3D ) , , . , «» , . 6, , β .
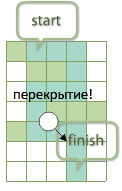
6 β
clippingType=a_star.P ( ) , . , , .
5. , β clippingType=a_star.F . .. .
, . , :
AStar.findPath(_startx:int,_starty:int,_targetx:int,_targety:int):void
{0,1} {6, 3}. findPath:
a_star.findPath(0,1,6,3);
, Event.COMPLETE (, ?), a_star . a_star my_path:Array:
private function onComplete(e:Event): void {
my_path=a_star.getPath();
}
, , . { _x: < >, _y: < >}. , 5.:
my_path=[
{_x:0,_y:1},
{_x:1,_y:0},
{_x:1,_y:2},
{_x:1,_y:3},
{_x:2,_y:4},
{_x:3,_y:5},
{_x:3,_y:6}
];
. , .
var obstacle_map:Array=[];
for ( var i: int =0; i<5; i++) {
obstacle_map[i]=[];
for ( var j: int =0; j<5; j++) {
obstacle_map[i][j]= false ;
}
}
2) , true.
obstacle_map[0][0]= true ;
obstacle_map[1][1]= true ;
obstacle_map[2][1]= true ;
obstacle_map[2][3]= true ;
obstacle_map[3][3]= true ;
obstacle_map[4][3]= true ;
obstacle_map[0][4]= true ;
, 2 β .

2 β
, , AStar.
β
, , , :
var a_star:AStar= new AStar();
a_star a_star.ERROR:
a_star.addEventListener(a_star.ERROR,onError);
, . onError. , , :
private function onError(e:Event): void {
trace(a_star.lastError);
}
, a_star, lastError. β . 3 output . , , .

3 β
:
a_star.setObstacleMap(obstacle_map);
! AStar.ERROR AStar, . , . setObstacleMap - , lastError . , β , . , , .. , , , , AStar, .
Event.COMPLETE:
a_star.addEventListener(Event.COMPLETE,onComplete);
onComplete , clippingType ( ):
a_star.clippingType=<>
, : a_star.F ( full), a_star.P ( prevent) a_star.N ( none). a_star.N , . ? , . . 4 S (start) , f (finish) .
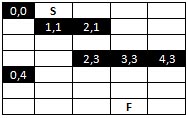
4 β
clippingType (. 5)

5. β clippingType=a_star.N .
5. β , . clippingType=a_star.P . : , ( , 3D ) , , . , «» , . 6, , β .
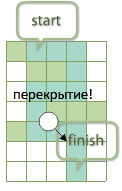
6 β
clippingType=a_star.P ( ) , . , , .
5. , β clippingType=a_star.F . .. .
, . , :
AStar.findPath(_startx:int,_starty:int,_targetx:int,_targety:int):void
{0,1} {6, 3}. findPath:
a_star.findPath(0,1,6,3);
, Event.COMPLETE (, ?), a_star . a_star my_path:Array:
private function onComplete(e:Event): void {
my_path=a_star.getPath();
}
, , . { _x: < >, _y: < >}. , 5.:
my_path=[
{_x:0,_y:1},
{_x:1,_y:0},
{_x:1,_y:2},
{_x:1,_y:3},
{_x:2,_y:4},
{_x:3,_y:5},
{_x:3,_y:6}
];
. , .
var obstacle_map:Array=[];
for ( var i: int =0; i<5; i++) {
obstacle_map[i]=[];
for ( var j: int =0; j<5; j++) {
obstacle_map[i][j]= false ;
}
}
2) , true.
obstacle_map[0][0]= true ;
obstacle_map[1][1]= true ;
obstacle_map[2][1]= true ;
obstacle_map[2][3]= true ;
obstacle_map[3][3]= true ;
obstacle_map[4][3]= true ;
obstacle_map[0][4]= true ;
, 2 β .

2 β
, , AStar.
β
, , , :
var a_star:AStar= new AStar();
a_star a_star.ERROR:
a_star.addEventListener(a_star.ERROR,onError);
, . onError. , , :
private function onError(e:Event): void {
trace(a_star.lastError);
}
, a_star, lastError. β . 3 output . , , .

3 β
:
a_star.setObstacleMap(obstacle_map);
! AStar.ERROR AStar, . , . setObstacleMap - , lastError . , β , . , , .. , , , , AStar, .
Event.COMPLETE:
a_star.addEventListener(Event.COMPLETE,onComplete);
onComplete , clippingType ( ):
a_star.clippingType=<>
, : a_star.F ( full), a_star.P ( prevent) a_star.N ( none). a_star.N , . ? , . . 4 S (start) , f (finish) .
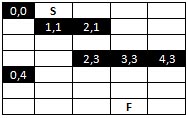
4 β
clippingType (. 5)

5. β clippingType=a_star.N .
5. β , . clippingType=a_star.P . : , ( , 3D ) , , . , «» , . 6, , β .
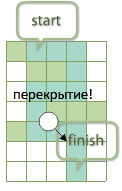
6 β
clippingType=a_star.P ( ) , . , , .
5. , β clippingType=a_star.F . .. .
, . , :
AStar.findPath(_startx:int,_starty:int,_targetx:int,_targety:int):void
{0,1} {6, 3}. findPath:
a_star.findPath(0,1,6,3);
, Event.COMPLETE (, ?), a_star . a_star my_path:Array:
private function onComplete(e:Event): void {
my_path=a_star.getPath();
}
, , . { _x: < >, _y: < >}. , 5.:
my_path=[
{_x:0,_y:1},
{_x:1,_y:0},
{_x:1,_y:2},
{_x:1,_y:3},
{_x:2,_y:4},
{_x:3,_y:5},
{_x:3,_y:6}
];
. , .
var obstacle_map:Array=[];
for ( var i: int =0; i<5; i++) {
obstacle_map[i]=[];
for ( var j: int =0; j<5; j++) {
obstacle_map[i][j]= false ;
}
}
2) , true.
obstacle_map[0][0]= true ;
obstacle_map[1][1]= true ;
obstacle_map[2][1]= true ;
obstacle_map[2][3]= true ;
obstacle_map[3][3]= true ;
obstacle_map[4][3]= true ;
obstacle_map[0][4]= true ;
, 2 β .

2 β
, , AStar.
β
, , , :
var a_star:AStar= new AStar();
a_star a_star.ERROR:
a_star.addEventListener(a_star.ERROR,onError);
, . onError. , , :
private function onError(e:Event): void {
trace(a_star.lastError);
}
, a_star, lastError. β . 3 output . , , .

3 β
:
a_star.setObstacleMap(obstacle_map);
! AStar.ERROR AStar, . , . setObstacleMap - , lastError . , β , . , , .. , , , , AStar, .
Event.COMPLETE:
a_star.addEventListener(Event.COMPLETE,onComplete);
onComplete , clippingType ( ):
a_star.clippingType=<>
, : a_star.F ( full), a_star.P ( prevent) a_star.N ( none). a_star.N , . ? , . . 4 S (start) , f (finish) .
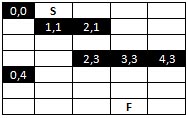
4 β
clippingType (. 5)

5. β clippingType=a_star.N .
5. β , . clippingType=a_star.P . : , ( , 3D ) , , . , «» , . 6, , β .
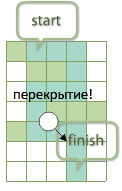
6 β
clippingType=a_star.P ( ) , . , , .
5. , β clippingType=a_star.F . .. .
, . , :
AStar.findPath(_startx:int,_starty:int,_targetx:int,_targety:int):void
{0,1} {6, 3}. findPath:
a_star.findPath(0,1,6,3);
, Event.COMPLETE (, ?), a_star . a_star my_path:Array:
private function onComplete(e:Event): void {
my_path=a_star.getPath();
}
, , . { _x: < >, _y: < >}. , 5.:
my_path=[
{_x:0,_y:1},
{_x:1,_y:0},
{_x:1,_y:2},
{_x:1,_y:3},
{_x:2,_y:4},
{_x:3,_y:5},
{_x:3,_y:6}
];
. , .
var obstacle_map:Array=[];
for ( var i: int =0; i<5; i++) {
obstacle_map[i]=[];
for ( var j: int =0; j<5; j++) {
obstacle_map[i][j]= false ;
}
}
2) , true.
obstacle_map[0][0]= true ;
obstacle_map[1][1]= true ;
obstacle_map[2][1]= true ;
obstacle_map[2][3]= true ;
obstacle_map[3][3]= true ;
obstacle_map[4][3]= true ;
obstacle_map[0][4]= true ;
, 2 β .

2 β
, , AStar.
β
, , , :
var a_star:AStar= new AStar();
a_star a_star.ERROR:
a_star.addEventListener(a_star.ERROR,onError);
, . onError. , , :
private function onError(e:Event): void {
trace(a_star.lastError);
}
, a_star, lastError. β . 3 output . , , .

3 β
:
a_star.setObstacleMap(obstacle_map);
! AStar.ERROR AStar, . , . setObstacleMap - , lastError . , β , . , , .. , , , , AStar, .
Event.COMPLETE:
a_star.addEventListener(Event.COMPLETE,onComplete);
onComplete , clippingType ( ):
a_star.clippingType=<>
, : a_star.F ( full), a_star.P ( prevent) a_star.N ( none). a_star.N , . ? , . . 4 S (start) , f (finish) .
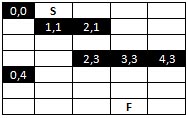
4 β
clippingType (. 5)

5. β clippingType=a_star.N .
5. β , . clippingType=a_star.P . : , ( , 3D ) , , . , «» , . 6, , β .
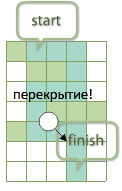
6 β
clippingType=a_star.P ( ) , . , , .
5. , β clippingType=a_star.F . .. .
, . , :
AStar.findPath(_startx:int,_starty:int,_targetx:int,_targety:int):void
{0,1} {6, 3}. findPath:
a_star.findPath(0,1,6,3);
, Event.COMPLETE (, ?), a_star . a_star my_path:Array:
private function onComplete(e:Event): void {
my_path=a_star.getPath();
}
, , . { _x: < >, _y: < >}. , 5.:
my_path=[
{_x:0,_y:1},
{_x:1,_y:0},
{_x:1,_y:2},
{_x:1,_y:3},
{_x:2,_y:4},
{_x:3,_y:5},
{_x:3,_y:6}
];
. , .
var obstacle_map:Array=[];
for ( var i: int =0; i<5; i++) {
obstacle_map[i]=[];
for ( var j: int =0; j<5; j++) {
obstacle_map[i][j]= false ;
}
}
2) , true.
obstacle_map[0][0]= true ;
obstacle_map[1][1]= true ;
obstacle_map[2][1]= true ;
obstacle_map[2][3]= true ;
obstacle_map[3][3]= true ;
obstacle_map[4][3]= true ;
obstacle_map[0][4]= true ;
, 2 β .

2 β
, , AStar.
β
, , , :
var a_star:AStar= new AStar();
a_star a_star.ERROR:
a_star.addEventListener(a_star.ERROR,onError);
, . onError. , , :
private function onError(e:Event): void {
trace(a_star.lastError);
}
, a_star, lastError. β . 3 output . , , .

3 β
:
a_star.setObstacleMap(obstacle_map);
! AStar.ERROR AStar, . , . setObstacleMap - , lastError . , β , . , , .. , , , , AStar, .
Event.COMPLETE:
a_star.addEventListener(Event.COMPLETE,onComplete);
onComplete , clippingType ( ):
a_star.clippingType=<>
, : a_star.F ( full), a_star.P ( prevent) a_star.N ( none). a_star.N , . ? , . . 4 S (start) , f (finish) .
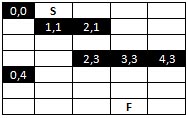
4 β
clippingType (. 5)

5. β clippingType=a_star.N .
5. β , . clippingType=a_star.P . : , ( , 3D ) , , . , «» , . 6, , β .
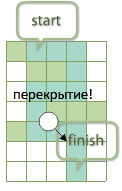
6 β
clippingType=a_star.P ( ) , . , , .
5. , β clippingType=a_star.F . .. .
, . , :
AStar.findPath(_startx:int,_starty:int,_targetx:int,_targety:int):void
{0,1} {6, 3}. findPath:
a_star.findPath(0,1,6,3);
, Event.COMPLETE (, ?), a_star . a_star my_path:Array:
private function onComplete(e:Event): void {
my_path=a_star.getPath();
}
, , . { _x: < >, _y: < >}. , 5.:
my_path=[
{_x:0,_y:1},
{_x:1,_y:0},
{_x:1,_y:2},
{_x:1,_y:3},
{_x:2,_y:4},
{_x:3,_y:5},
{_x:3,_y:6}
];
. , .
var obstacle_map:Array=[];
for ( var i: int =0; i<5; i++) {
obstacle_map[i]=[];
for ( var j: int =0; j<5; j++) {
obstacle_map[i][j]= false ;
}
}
2) , true.
obstacle_map[0][0]= true ;
obstacle_map[1][1]= true ;
obstacle_map[2][1]= true ;
obstacle_map[2][3]= true ;
obstacle_map[3][3]= true ;
obstacle_map[4][3]= true ;
obstacle_map[0][4]= true ;
, 2 β .

2 β
, , AStar.
β
, , , :
var a_star:AStar= new AStar();
a_star a_star.ERROR:
a_star.addEventListener(a_star.ERROR,onError);
, . onError. , , :
private function onError(e:Event): void {
trace(a_star.lastError);
}
, a_star, lastError. β . 3 output . , , .

3 β
:
a_star.setObstacleMap(obstacle_map);
! AStar.ERROR AStar, . , . setObstacleMap - , lastError . , β , . , , .. , , , , AStar, .
Event.COMPLETE:
a_star.addEventListener(Event.COMPLETE,onComplete);
onComplete , clippingType ( ):
a_star.clippingType=<>
, : a_star.F ( full), a_star.P ( prevent) a_star.N ( none). a_star.N , . ? , . . 4 S (start) , f (finish) .
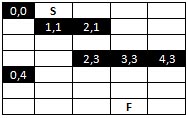
4 β
clippingType (. 5)

5. β clippingType=a_star.N .
5. β , . clippingType=a_star.P . : , ( , 3D ) , , . , «» , . 6, , β .
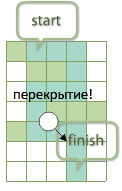
6 β
clippingType=a_star.P ( ) , . , , .
5. , β clippingType=a_star.F . .. .
, . , :
AStar.findPath(_startx:int,_starty:int,_targetx:int,_targety:int):void
{0,1} {6, 3}. findPath:
a_star.findPath(0,1,6,3);
, Event.COMPLETE (, ?), a_star . a_star my_path:Array:
private function onComplete(e:Event): void {
my_path=a_star.getPath();
}
, , . { _x: < >, _y: < >}. , 5.:
my_path=[
{_x:0,_y:1},
{_x:1,_y:0},
{_x:1,_y:2},
{_x:1,_y:3},
{_x:2,_y:4},
{_x:3,_y:5},
{_x:3,_y:6}
];
. , .
var obstacle_map:Array=[];
for ( var i: int =0; i<5; i++) {
obstacle_map[i]=[];
for ( var j: int =0; j<5; j++) {
obstacle_map[i][j]= false ;
}
}
2) , true.
obstacle_map[0][0]= true ;
obstacle_map[1][1]= true ;
obstacle_map[2][1]= true ;
obstacle_map[2][3]= true ;
obstacle_map[3][3]= true ;
obstacle_map[4][3]= true ;
obstacle_map[0][4]= true ;
, 2 β .

2 β
, , AStar.
β
, , , :
var a_star:AStar= new AStar();
a_star a_star.ERROR:
a_star.addEventListener(a_star.ERROR,onError);
, . onError. , , :
private function onError(e:Event): void {
trace(a_star.lastError);
}
, a_star, lastError. β . 3 output . , , .

3 β
:
a_star.setObstacleMap(obstacle_map);
! AStar.ERROR AStar, . , . setObstacleMap - , lastError . , β , . , , .. , , , , AStar, .
Event.COMPLETE:
a_star.addEventListener(Event.COMPLETE,onComplete);
onComplete , clippingType ( ):
a_star.clippingType=<>
, : a_star.F ( full), a_star.P ( prevent) a_star.N ( none). a_star.N , . ? , . . 4 S (start) , f (finish) .
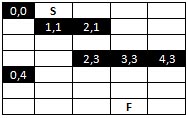
4 β
clippingType (. 5)

5. β clippingType=a_star.N .
5. β , . clippingType=a_star.P . : , ( , 3D ) , , . , «» , . 6, , β .
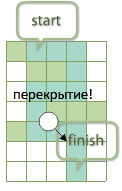
6 β
clippingType=a_star.P ( ) , . , , .
5. , β clippingType=a_star.F . .. .
, . , :
AStar.findPath(_startx:int,_starty:int,_targetx:int,_targety:int):void
{0,1} {6, 3}. findPath:
a_star.findPath(0,1,6,3);
, Event.COMPLETE (, ?), a_star . a_star my_path:Array:
private function onComplete(e:Event): void {
my_path=a_star.getPath();
}
, , . { _x: < >, _y: < >}. , 5.:
my_path=[
{_x:0,_y:1},
{_x:1,_y:0},
{_x:1,_y:2},
{_x:1,_y:3},
{_x:2,_y:4},
{_x:3,_y:5},
{_x:3,_y:6}
];
. , .
var obstacle_map:Array=[];
for ( var i: int =0; i<5; i++) {
obstacle_map[i]=[];
for ( var j: int =0; j<5; j++) {
obstacle_map[i][j]= false ;
}
}
2) , true.
obstacle_map[0][0]= true ;
obstacle_map[1][1]= true ;
obstacle_map[2][1]= true ;
obstacle_map[2][3]= true ;
obstacle_map[3][3]= true ;
obstacle_map[4][3]= true ;
obstacle_map[0][4]= true ;
, 2 β .

2 β
, , AStar.
β
, , , :
var a_star:AStar= new AStar();
a_star a_star.ERROR:
a_star.addEventListener(a_star.ERROR,onError);
, . onError. , , :
private function onError(e:Event): void {
trace(a_star.lastError);
}
, a_star, lastError. β . 3 output . , , .

3 β
:
a_star.setObstacleMap(obstacle_map);
! AStar.ERROR AStar, . , . setObstacleMap - , lastError . , β , . , , .. , , , , AStar, .
Event.COMPLETE:
a_star.addEventListener(Event.COMPLETE,onComplete);
onComplete , clippingType ( ):
a_star.clippingType=<>
, : a_star.F ( full), a_star.P ( prevent) a_star.N ( none). a_star.N , . ? , . . 4 S (start) , f (finish) .
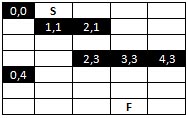
4 β
clippingType (. 5)

5. β clippingType=a_star.N .
5. β , . clippingType=a_star.P . : , ( , 3D ) , , . , «» , . 6, , β .
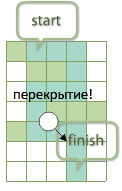
6 β
clippingType=a_star.P ( ) , . , , .
5. , β clippingType=a_star.F . .. .
, . , :
AStar.findPath(_startx:int,_starty:int,_targetx:int,_targety:int):void
{0,1} {6, 3}. findPath:
a_star.findPath(0,1,6,3);
, Event.COMPLETE (, ?), a_star . a_star my_path:Array:
private function onComplete(e:Event): void {
my_path=a_star.getPath();
}
, , . { _x: < >, _y: < >}. , 5.:
my_path=[
{_x:0,_y:1},
{_x:1,_y:0},
{_x:1,_y:2},
{_x:1,_y:3},
{_x:2,_y:4},
{_x:3,_y:5},
{_x:3,_y:6}
];
. , .
Source: https://habr.com/ru/post/71083/
All Articles