PHP-GTK is a binding toolkit for creating a
GTK + GUI to the
PHP programming
language . About it already
wrote on Habré . Many people are skeptical of this library, not so much of itself as of using PHP on the desktop. But, anyway, the library exists and, moreover, develops, as evidenced by the recent news on the
official website . Therefore, I suggest to get acquainted with PHP-GTK in more detail and write a small program.
The program creates a window and displays in it a text widget containing an integer (default is 0), an “Zoom In” button, when clicked, the number will be incremented by one, and a “Reset” button resetting the text widget value to zero.
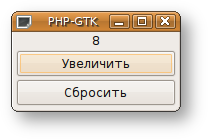
Creating a base window
The window is created using the
GtkWindow () class constructor. To determine the position occupied by the window after creation, the
set_position () method is used,
set_title () sets the title, and
set_size_request () - the window size (-1 means that the height will be selected automatically based on the size of the content). When the window is closed, the 'destroy' signal is emitted. At this point, for the program to shut down correctly, you must call Gtk :: main_quit (); the
connect_simple () method is used for this.
$ window = new GtkWindow ( ) ;
$ window -> set_position ( Gtk :: WIN_POS_CENTER ) ;
$ window -> set_size_request ( 160 , - 1 ) ;
$ window -> set_title ( 'PHP-GTK' ) ;
$ window -> connect_simple ( 'destroy' , 'Gtk :: main_quit' ) ;
Widgets
As mentioned earlier, the program uses one text
GtkLabel () widget and two
GtkButton () buttons. In order to place them in the window, you need to use a special vertical container
GtkVBox () , because GtkWindow () allows you to put only one widget.
$ vbox = new GtkVBox ( ) ;
$ label = new GtkLabel ( '0' ) ;
$ btn_inc = new GtkButton ( 'Zoom in' ) ;
$ btn_cancel = new GtkButton ( 'Reset' ) ;
$ vbox -> pack_start ( $ label ) ;
$ vbox -> pack_start ( $ btn_inc ) ;
$ vbox -> pack_start ( $ btn_cancel ) ;
')
User interaction
When you press the buttons, a 'clicked' signal is emitted, “hearing” which we call the increment () function to increase the number and cancel () to reset the value. In the connect_simple () method, after the function name there is a list of parameters passed to the function being called. In this case, we pass the GtkLabel () widget to both functions. In order to get the text currently in GtkLabel (), you need to use the
get_label () method, and to set the new value -
set_label () .
$ btn_inc -> connect_simple ( 'clicked' , 'increment' , $ label ) ;
$ btn_cancel -> connect_simple ( 'clicked' , 'cancel' , $ label ) ;
function increment ( $ label )
{
$ int = $ label -> get_text ( ) ;
$ int ++;
$ label -> set_text ( $ int ) ;
}
function cancel ( $ label )
{
$ label -> set_text ( '0' ) ;
}
Now it remains only to add the container to the window and display the window on the screen. Full program code:
<? php
$ window = new GtkWindow ( ) ;
$ window -> set_position ( Gtk :: WIN_POS_CENTER ) ;
$ window -> set_size_request ( 160 , - 1 ) ;
$ window -> set_title ( 'PHP-GTK' ) ;
$ window -> connect_simple ( 'destroy' , 'Gtk :: main_quit' ) ;
$ vbox = new GtkVBox ( ) ;
$ label = new GtkLabel ( '0' ) ;
$ btn_inc = new GtkButton ( 'Zoom in' ) ;
$ btn_cancel = new GtkButton ( 'Reset' ) ;
$ vbox -> pack_start ( $ label ) ;
$ vbox -> pack_start ( $ btn_inc ) ;
$ vbox -> pack_start ( $ btn_cancel ) ;
$ btn_inc -> connect_simple ( 'clicked' , 'increment' , $ label ) ;
$ btn_cancel -> connect_simple ( 'clicked' , 'cancel' , $ label ) ;
function increment ( $ label )
{
$ int = $ label -> get_text ( ) ;
$ int ++;
$ label -> set_text ( $ int ) ;
}
function cancel ( $ label )
{
$ label -> set_text ( '0' ) ;
}
$ window -> add ( $ vbox ) ;
$ window -> show_all ( ) ;
Gtk :: main ( ) ;
?>
PHP-GTK official websiteManual