
Development in the framework of one project has a number of advantages. First, it allows you to use a single implementation of the business logic of the program. Secondly, it is an opportunity to have a single set of unit tests. Thirdly, it is the use of the usual language (C ++) and the development environment.
This article describes some of the programming techniques and several libraries that help create cross-platform applications.
Based on the experience of creating a small application such as "calculator"
Cross Platform Development
It is convenient to write one code instead of three, it significantly reduces the time to create a program. But unfortunately, each platform has its dependent code (functionality). Often without the functionality provided by a specific platform simply can not do. A possible solution to this problem are design strategies (for more details, see Alexandrescu’s book “Modern Design in C ++”). All dependent code is placed into settings classes, the so-called
“policy” , which later will configure larger classes.
Using cross-platform libraries (
boost ,
stl and others) also simplifies development.
MVC
Using the
MVC model greatly simplifies the design of the application, since Most platform dependencies are localized in the "View (View)" component.
')
Htmlayout
To organize the graphical interface, the
Htmlayout library was used, which allows describing the graphical interface of the program using
html and
css . Implementing a GUI using web-based tools carries a lot of positive things, starting with a large number of web designers and ending with a huge number of ready-made design options. And having as one of the target platforms “web” it reduces the cost of implementing the design in the form of
html + css .
Htmlayout has an implementation for Windows Mobile that allows you to use it also for a mobile platform.
Program calculator
The program of the “calculator” type was chosen because it fully allows one to try to implement the MVC model and assumes the use of some components of the
“boost” library that were interesting to me.
Desktop
The implementation of the application for the desktop (native platform for me) involved, above all, the writing of the data models used in the program and the implementation of the overall architecture of the program. A new moment for me was the use of the
Htmlayout library. However, there were no problems with the implementation of the graphical interface.
The code of the main.cpp file in which the main classes are configured and instantiated:#include "stdafx.h"<br/>
<br/>
//Calculator includes<br/>
#include "RegexNumberCheckModel.h"<br/>
#include "CalculatorModel.hpp"<br/>
#include "DesktopView.h"<br/>
#include "CalculatorController.hpp"<br/>
<br/>
CAppModule _Module;<br/>
<br/>
int APIENTRY WinMain( HINSTANCE hInstance<br/>
,HINSTANCE hPrevInstance<br/>
,LPSTR lpCmdLine<br/>
, int nShowCmd)<br/>
{<br/>
<br/>
//MVC concept:<br/>
//Model<br/>
typedef CalculatorModel< double , Operations, RegexNumberCheckModel> MyCalculatorModel;<br/>
MyCalculatorModel calcModel;<br/>
//View<br/>
typedef DesktopView<MyCalculatorModel> MyCalculatorView;<br/>
MyCalculatorView calcView;<br/>
//Controller<br/>
typedef CalculatorController<MyCalculatorModel, MyCalculatorView::ViewPolicy> MyCalculatorController;<br/>
MyCalculatorController calcController(calcModel, calcView);<br/>
<br/>
calcView.Run(hInstance);<br/>
<br/>
return 0;<br/>
}
Mobile

The implementation for the mobile platform began with the writing of the “Hello, world” test program described in the article
“WTL: Programming for Mobile and Embedded WinCE / Windows Mobile Based Devices” . To implement the GUI calculator, it was necessary only to link the program window and the library window, plus a little change in the css template. To adapt the data model, I had to configure input validation with my own class, instead of using
“boost / xpressive” .
Mobile version main.cpp code:
#include "stdafx.h"<br/>
<br/>
//Calculator includes<br/>
//#include "RegexNumberCheckModel.h"<br/>
#include "NoNumberCheckModel.h"<br/>
#include "CalculatorModel.hpp"<br/>
#include "MobileView.h"<br/>
#include "CalculatorController.hpp"<br/>
<br/>
CAppModule _Module;<br/>
<br/>
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE, LPTSTR, int )<br/>
{<br/>
<br/>
//MVC concept<br/>
//Model<br/>
typedef CalculatorModel< double , Operations, NoNumberCheckModel> MyCalculatorModel;<br/>
MyCalculatorModel calcModel;<br/>
//View<br/>
typedef MobileView<MyCalculatorModel> MyCalculatorView;<br/>
MyCalculatorView calcView;<br/>
//Controller<br/>
typedef CalculatorController<MyCalculatorModel, MyCalculatorView::ViewPolicy> MyCalculatorController;<br/>
MyCalculatorController calcController(calcModel, calcView);<br/>
<br/>
calcView.Run(hInstance);<br/>
<br/>
<br/>
return 0;<br/>
}
The changes are minimal: the
CalculatorModel is configured by the
NoNumberCheckModel class, and the
DesktopView is replaced by a
MobileView .
Web
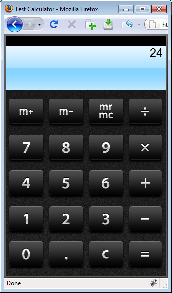
Immediately, I note that the described program “calculator” is not a web application, it is an application with a web interface.
With the implementation of the web was a little more difficult, too few articles and examples can be found on the Internet on this topic. The result of a small study was the article
"Apache, fastcgi and c ++:" Hello, world " , published a week ago on Habré. After choosing the main technology, there were no special problems with the implementation of the interface and the processing of AJAX requests.
The class that implements the “View (View)”
component of DesktopView has been replaced by
FastcgiView .
Conclusion
The correct approach to the design of the application allows without considerable effort to develop programs capable of working under most popular platforms.
And having libraries like
htmlayout ,
boost and
stl allows you to do this quickly.
I think this development technique is quite suitable for programs like torrent-clients or smart home control systems ...