At one time, while making a report on the ASP.NET MVC Framework, I noticed that this framework allows the developer to use TDD practices when developing and immediately received the question of what TDD is, why and how ASP.NET MVC Framework gives these very possibilities.
ASP.NET MVC Framework + TDD
The very first hint of a TDD developer receives as soon as he tries to create a project using the “ASP.NET MVC Web application” template. It will also be proposed to create another project for tests.
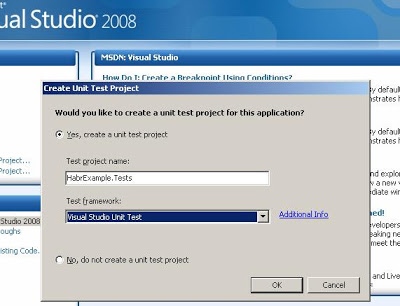
In this case, it is proposed to use standard MS testing technologies, but on the Internet there are solutions for how to add both xUnit, MbUnit, and
nUnit . If we use such a hint, we will get another project in which all the necessary libraries will be connected for testing, a cs-file with one class and method will be created, then you will have to do everything with pens.
Starting with Preview 2, all Action methods must return a result. The result can be any class inherited from the base ActionResult type (ViewResult, JsonResult, ActionRedirectResult, and so on). Thus, when testing, you can easily verify the data that your method passes to View (ViewData).
ViewResult data = blogController.Comments(5);
Assert.AreEqual(expect, data.ViewData.Model);
* This source code was highlighted with Source Code Highlighter .
In addition, if the method returns a ViewResult, you can just as easily check which View will be used.
ViewResult data = blogController.Comments(5);
Assert.AreEqual( "comments" , data.ViewName);
* This source code was highlighted with Source Code Highlighter .
')
Also in the framework itself, there is not a single sealed class, and all types are built on interfaces (IView, IController) or have their base abstract class (HttpResponseBase, HttpSessionStateBase). This approach will easily allow any class to get wet during testing. That, for example, will allow to test routes.
var httpContextMock = new Mock<HttpContextBase>();
var requestMock = new Mock<HttpRequestBase>();
httpContextMock.Expect(c => c.Request).Returns(requestMock.Object);
requestMock.Expect(r => r.AppRelativeCurrentExecutionFilePath)
.Returns(url);
RouteData routeData = routes.GetRouteData(httpContextMock.Object);
Assert.IsNotNull(routeData, "Should have found the route" );
* This source code was highlighted with Source Code Highlighter .
So MS having chosen the approach for this TDD technology, they are forced to use solutions that allow end users to easily follow their example.
So in RC1, it became possible to automatically create a View for the desired Action Method, why not go ahead and embed the ability to automatically create a test suite (Of course, it is not possible to cover the entire test case, but the most repetitive ... Why not.)
Well, at the end of one test for my method and how it looks in nUnit-e.
A small example of the test and method.
- [TestFixture]
- public class TestCase4BlogsController
- {
- [Test]
- public void TestGetCommentsList_IfPutIDExistBlog ()
- {
- MockModel mockModel = new MockModel ();
- BlogController blogController = new BlogController ();
- ArrayList expect = new ArrayList { new {Data = "This is not a fish for you, jamming with dynamite" , Date = "February 12, 2009" , Author = "Ivan Petrovich" },
- new {Data = "So dynamite, it is more effective" , Date = "02/13/2009" , Author = "Pacak" },
- new {Data = "And what will you do with this fish then ???" , Date = "February 13, 2009" , Author = "Ivan Petrovich" }};
- mockModel.comments = expect;
- blogController.Model = mockModel as IBlogModel;
- ViewResult data = blogController.Comments (5);
- Assert.AreEqual (expect, data.ViewData.Model);
- }
- }
* This source code was highlighted with Source Code Highlighter .
- public class MockModel: IBlogModel
- {
- public ArrayList comments = null ;
- #region IBlogModel Members
- public ArrayList GetComment4Blog ( int blogId)
- {
- return comments;
- }
- #endregion
- }
* This source code was highlighted with Source Code Highlighter .
Before the method was written.
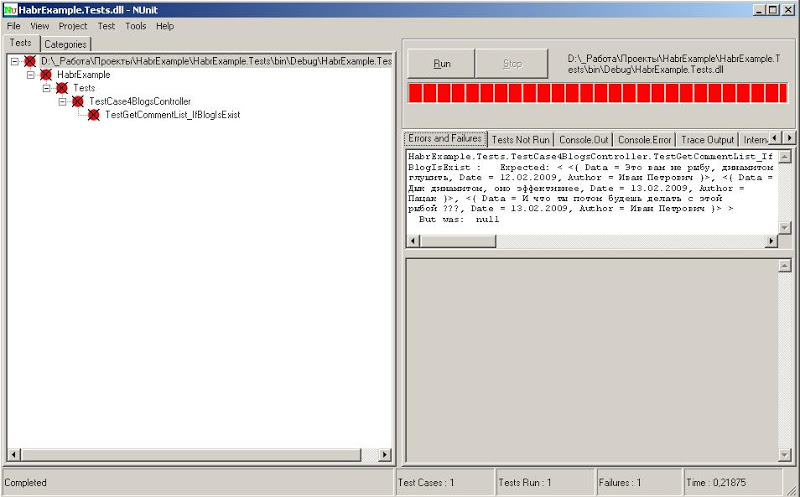
The implementation of the method.
- [AcceptVerbs (HttpVerbs.Get)]
- public ViewResult Comments ( int blogId)
- {
- return View ( "comments" , Model.GetComment4Blog (blogId));
- }
* This source code was highlighted with Source Code Highlighter .
After compilation.
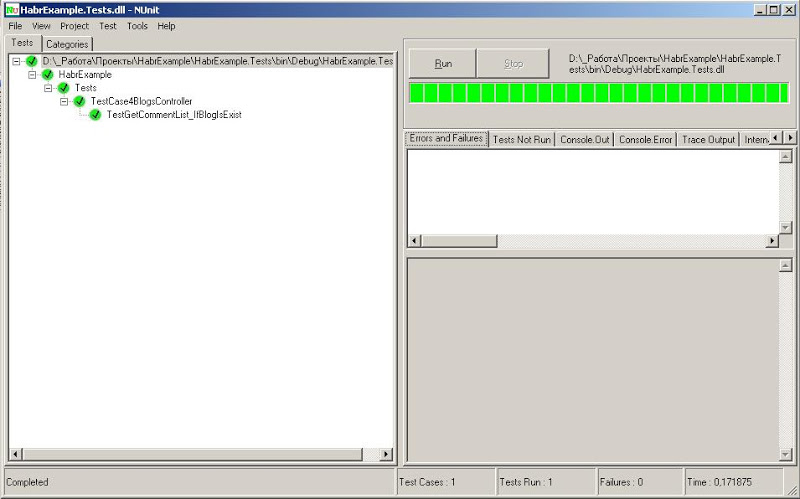