This topic is a response to
this application in the
wizard about a habruiser that projects for working with a database on .Net take up a lot of disk space and in general, twenty years ago the grass was greener and the trees higher.
As one of the commentators of the aforementioned topic (
neuotq ) said:
to dig a hole in the sandboxes you should not use an excavator and the whole construction team
It is important to understand that modern frameworks provide the programmer with many tools for solving problems. And if you need, I quote, “get a couple of lines of data” from the database, you do not need to use “combines with a laser sight” for this purpose a la ADO.Net Entity Framework or NHibernate. It is important to understand that such tools are designed for
large projects. By a large project, I mean not a term paper or a thesis, but large-scale commercial systems that cost a lot of money and an extra “1 day (week, month)” (again,
quote ) spent writing my own bikes costs the customer hundreds (thousands, millions) is not Russian money. A good developer is different from a bad one in that he knows when to write a bike, and when it’s enough to take a ready one. A bad one, respectively, either shoves frameworks around, or else it writes bicycles everywhere. And the blame for this is not the developer’s tools, but himself.
Everything, I will not tire with my philosophy. Under habrakat you can read about how you can interact with the database in .Net without the involvement of excavators.
So, if your application has a simple database from several tables, and you want to achieve high performance of the pair of queries that you will need to perform, then it is not necessary to use the ADO.Net Entity Framework or something comparable. It will be enough to use the SqlConnection, SqlCommand and SqlDataReader classes. To demonstrate, we will write a simple window on WPF:
< Window x:Class ="sqlTest.Window1" <br> xmlns ="http://schemas.microsoft.com/winfx/2006/xaml/presentation" <br> xmlns:x ="http://schemas.microsoft.com/winfx/2006/xaml" <br> Title ="Window1" Height ="339" Width ="783" > <br> < Grid > <br> < Label Height ="28" HorizontalAlignment ="Left" Name ="label1" VerticalAlignment ="Top" Width ="120" > Connection String: </ Label > <br> < TextBox Height ="23" Margin ="126,2,0,0" Name ="connStr" VerticalAlignment ="Top" > Data Source=localhost;Initial Catalog=BlogNetTest;Integrated Security=True;MultipleActiveResultSets=True </ TextBox > <br> < TextBox Margin ="126,31,0,0" Name ="queryTB" AcceptsReturn ="True" TextWrapping ="Wrap" Height ="132" VerticalAlignment ="Top" > SELECT * FROM Users </ TextBox > <br> < Label Height ="28" HorizontalAlignment ="Left" Margin ="0,29,0,0" Name ="label2" VerticalAlignment ="Top" Width ="120" > Query: </ Label > <br> < TextBox Height ="125" Margin ="126,0,0,12" Name ="resultTB" VerticalAlignment ="Bottom" /> <br> < Button Height ="23" HorizontalAlignment ="Left" Margin ="12,0,0,114" Name ="button1" VerticalAlignment ="Bottom" Width ="108" Click ="button1_Click" > Execute </ Button > <br> </ Grid > <br> </ Window > <br><br> * This source code was highlighted with Source Code Highlighter .
It looks like this:
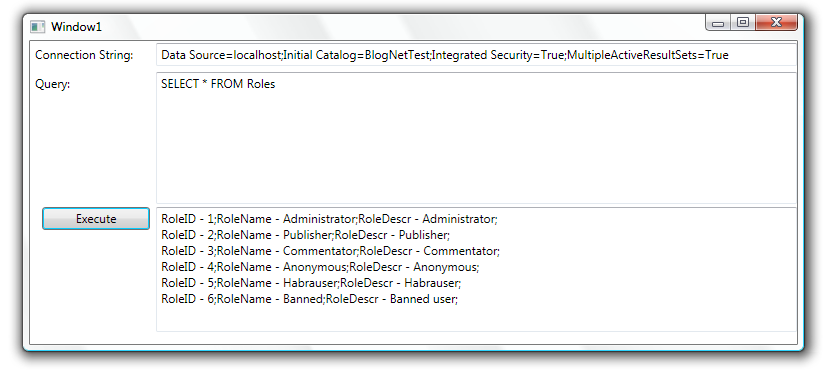
Ok, now let's write the code that will execute the query and insert the result in the field at the bottom of the window:
using System;<br> using System.Data.SqlClient;<br> using System.Text;<br> using System.Windows;<br><br> namespace sqlTest<br>{<br> public partial class Window1<br> {<br> public Window1()<br> {<br> InitializeComponent();<br> }<br><br> private void button1_Click( object sender, RoutedEventArgs e)<br> {<br> try <br> {<br> resultTB.Text = ReadData(connStr.Text, queryTB.Text);<br> }<br> catch (Exception ex)<br> {<br> resultTB.Text = string .Format( "Error: {0}" , ex.Message);<br> }<br> }<br><br> private static string ReadData( string connectionString, string queryString)<br> {<br> var strBuilder = new StringBuilder ();<br> using ( var connection =<br> new SqlConnection(connectionString))<br> {<br> var command =<br> new SqlCommand (queryString, connection);<br> connection.Open();<br><br> var reader = command.ExecuteReader();<br><br> while (reader.Read())<br> {<br> for ( int i = 0; i < reader.FieldCount; i++)<br> {<br> strBuilder.AppendFormat( "{0} - {1};" , reader.GetName(i), reader[i]);<br> }<br> strBuilder.AppendLine();<br> }<br> reader.Close();<br> }<br> return strBuilder.ToString();<br> }<br><br> }<br>}<br> <br> * This source code was highlighted with Source Code Highlighter .
A small explanation about the Connection String: this line is a description of how to connect to your database and how to form it for your database can be read
here . My line will not work for you, as it refers to a local SQL Server 2005 Express Edition with a test base of one of my projects.
But in general, that's all. Solyushen occupies hundreds of kilobytes on the disk and 20 megabytes of memory at work. This large amount of memory is due to the use of WPF, which has a minimum memory usage threshold of around 15 megabytes. To verify this statement, I rewrote the program to work in console mode and made sure that it eats about 4 megabytes. Of course, this is not small, but not too much today, when the memory amounts in gigabytes. Yes, in 1989 such a program would occupy 500 kilobytes of memory on a machine with 1 MB of RAM (50%). Now it takes 20 megabytes on a machine with 2 GB of RAM (1%). But in 1989, I would have spent a month trying to implement even such a primitive functionality, as in the example, but now it happened in just 30 minutes.
Archive with WPF and console projectPS I apologize for some confusion of the text, I spent only an hour and a half on it.
UPD: The fact that SQLClient is used with might and main as a basis for frameworks that work with the database I know firsthand - three of the three commercial projects in the development of which I took part use it. An article for students who do not know about them and stubbornly use “excavators for digging holes” in their coursework / diplomas and even scolding them for what you see “does not allow them to make a direct request to the database”. If you go superficially on-and-no in search of how you can work with a database from a datnet, you will see that 90% of the material is devoted to ADO.Net Datasets, LINQ2SQL and EF. Actually, the newcomers peck at it, and then swear because they have chosen the wrong instrument from the very beginning.