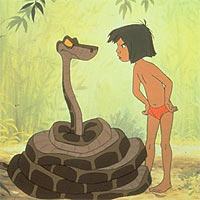
Continuing the theme
Configuring Ubuntu 8.10 for coding under NVIDIA CUDA , I decided to fix myself a workplace for using one of my favorite programming languages - Python. To do this, there is an excellent set of PyCUDA libraries that allows you to implement all the delights of the NVIDIA CUDA API using a convenient and beautiful code.
I will give an example of code from standard PyCUDA configuration:
import pycuda.autoinit
import pycuda.driver as drv
import numpy
mod = drv.SourceModule("""
__global__ void multiply_them(float *dest, float *a, float *b)
{
const int i = threadIdx.x;
dest[i] = a[i] * b[i];
}
""")
multiply_them = mod.get_function("multiply_them")
a = numpy.random.randn(400).astype(numpy.float32)
b = numpy.random.randn(400).astype(numpy.float32)
dest = numpy.zeros_like(a)
multiply_them(
drv.Out(dest), drv.In(a), drv.In(b),
block=(400,1,1))
print dest-a*b
Isn't it convenient and simple?
Let's start!
For normal PyCUDA operation, we will need:
- A machine with a UNIX-like system and access to the Internet.
- Nvidia CUDA toolkit. PyCuda was developed with an eye on version 2.0 beta, but for me it seems to work just fine with 2.1 beta.
- C ++ compiler, preferably gcc version not lower than 4.x.
- Installed Python interpreter, version 2.4 or newer.
')
Now install the
latest version of the Boost C ++ library . Before installing it is worth checking whether the developer package for Python is installed, it will be called
python-dev or
python-devel . The installation is actually standard, just don’t forget to set the prefix - the directory for the collected inclusions. In this case, let it be
$ HOME / pool .
$ tar xfj ~/downloads/boost_1_37_0.tar.bz2
$ cd boost_1_37_0
$ ./configure --prefix=$HOME/pool
$ make
$ make install
After the build (it should be successful) we check if the
$ HOME / pool folder has appeared. If yes - then all the way, go ahead :)
Important note: If something like this appears at the end of the installation
...failed updating 30 targets...
...skipped 2 targets...
then check again if you have Python developer headers installed. If it was not possible to update only a few files (less than 5), then most likely everything will work fine, but for fidelity you can install
libz-dev and
libbz2-dev and build
Boost again.
Now add the path to the newly compiled libraries to the environment variable:
$ export LD_LIBRARY_PATH=$HOME/pool/lib:${LD_LIBRARY_PATH}
If this is not done, then at the PyCUDA build stage, something like this will appear:
...blablabla...
ImportError: libboost_python-gcc42-mt-1_35.so.1.35.0:
cannot open shared object file: No such file or directory
...blablabla...
We download PyCUDA itself and go to the directory with it.
$ cd $HOME/downloads/pycuda-0.91.1
You need to install the
numpy package. You can do this either by downloading it yourself from
numpy.org and
building it, or by using PyCUDA itself. Since we have already moved to the directory with the distribution, just type:
$ sudo python ez_setup.py // setup-tools
$ sudo easy_install numpy // numpy setup-tools
Order.
We collect PyCUDA.
It remains the last and most important thing - to install PyCUDA itself. The main difficulty lies in the fact that you need to specify a bunch of options during configuration.
$ ./configure \
--boost-inc-dir=$HOME/pool/include/boost-1_37 \
--boost-lib-dir=$HOME/pool/lib \
--boost-python-libname=boost_python-gcc41-mt \
--cuda-root=/where/ever/you/installed/cuda
And now - attention! If everything is clear with the first two parameters (explained above), then the last two need to be explained.
boost_python-gcc41-mt is the name of the library in which
gcc41 is the version of the compiler with which you compiled the
boost . As I wrote in the previous article, when programming under CUDA, version 4.1 should usually be used, but if you did not set it to system default, then this is irrelevant in this case. You can check the version of the active compiler by typing
$ gcc -v
Well, in the last paragraph, as it is easy to guess, you need to replace
/ where / ever / you / installed / cuda with the directory with CUDA, that is, by default
/ usr / local / cuda .
By the way, before entering
make install, you can double-check the entered addresses. They are all stored in the
siteconf.py file, which is created during configuration. He looked like this before my build:
BOOST_INC_DIR = ['/ home / username / pool / include / boost-1_37']
BOOST_LIB_DIR = ['/ home / username / pool / lib']
BOOST_PYTHON_LIBNAME = ['boost_python-gcc41-mt']
CUDA_ROOT = '/ usr / local / cuda'
CUDADRV_LIB_DIR = []
CUDADRV_LIBNAME = ['cuda']
CXXFLAGS = []
LDFLAGS = []
If you want to reconfigure PyCUDA with new parameters, first delete this file. Or you can just make changes to it and type
$ sudo python setup.py build
Now you can safely compile:
$ sudo make install
If everything is done correctly, then there should be no errors during the assembly. Restart the computer, and for complete confidence you can go to the directory
/pycuda-0.91.1/test (or
whatever version you have there) and run
test_driver.py .
$ cd /pycuda-0.91.1/test
$ python test_driver.py
If the result is OK, then everything is in chocolate. If one or two errors (for some reason, I sometimes had glitches with memory) - just try a little later again.
Thank you for reading to the end, I will be glad to hear criticism :)
ADF : Everything was set up, including work in Eclipse. The problem was that the wrong rights to the cache folder were set up, the easiest way to do this:
$ sudo chmod 0777 -R /home/username/.pycuda-compiler-cache
Well, among other things, neither the interpreter nor the Eclipse itself should be launched from under the root. For other environment variables :) Thanks for the help in setting up a friend of
Riz .