Performance is one of the most important issues facing web developers or web applications. No one will be happy with an application, or a page that has been loading for ages, that crashes from excessive load. Website users are not ready to wait too long for their downloads or to bring their pages into working condition. According to
Kissmetrics , 47% of visitors expect a website to load in less than 2 seconds. 40% of visitors will leave the site if it takes more than 3 seconds to load it.
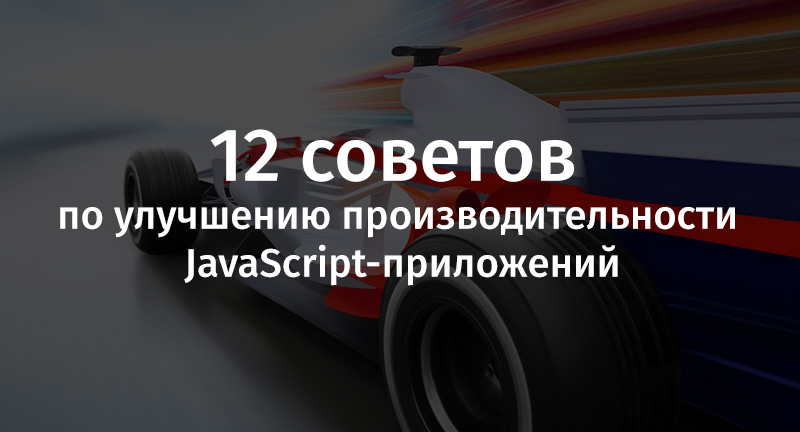
The author of the material, the translation of which we publish today, says that, given the above figures, it becomes clear that productivity is something that web developers should always remember. Here are 12 tips for improving the performance of JS projects.
1. Use browser caching mechanisms
There are two main ways to cache data using browsers. The first is the use of the Cache JavaScript JavaScript API, which is handled by service workers. The second is a regular HTTP cache.
')
Scripts are often used to organize access to certain objects. If you store a reference to an object that you often need to access in a variable, and also if you use this variable in repeated operations that require access to the object, you can improve code performance.
2. Optimize the code for those environments in which it will run
In order to adequately assess the improvements made to the program, it is recommended to form a set of environments in which measurements can be made.
In practice, you will not be able to perform code performance research, for example, in all existing versions of JS engines, as well as optimize the code for all the environments in which it can run. But it should be noted that testing code in any one environment is also not the best practice. Such an approach can give distorted results. Therefore, it is important to create a set of environments in which the code will most likely run, and test projects in these environments.
3. Get rid of unused JS code
Removing unused code from a project will improve not only the browser loading time for scripts, but also the time required for browsers to analyze and compile the code. In order to get rid of unused code, you should pay attention to the features of the project. So, if you find some functionality that users don’t work with, consider removing it from the project, and at the same time consider the JS code associated with it. As a result, the site will load faster, it will be faster to prepare for work in the browser. This will have a beneficial effect on the impressions that users will experience with the site. When analyzing a project, keep in mind that, for example, a certain library included in its composition may be included in it by mistake. It may very well not be used in it. It is worth getting rid of it. The same applies to the use of certain dependencies that implement what is already implemented in modern browsers. As a result, switching to standard browser features, duplicated by this dependency, will help get rid of unnecessary code.
4. Save memory
It is worth striving to ensure that web projects use only that memory, without which they are absolutely unable to do. The fact is that the developer cannot know in advance how much memory can be accessed by his application on a certain device. If the application unjustifiably uses large amounts of memory, this creates an increased load on the memory management mechanisms of the browser JS engine. In particular, this applies to the garbage collector. Frequent garbage collection calls slow down programs. This negatively affects the usability of the project.
5. Use deferred loading mechanisms for minor scripts
Users want webpages to load as quickly as possible. But it is unlikely that the entire JS code of the project is needed for the initial display of the page. If a user needs to perform some action (for example, click on an element or go to some tab in the application) to activate a certain code, then loading of this code can be postponed after completing it after the initial page load and the most important resources.
With this approach, you can avoid the browser loading and compiling a large amount of JS code at the very beginning, that is, avoid slowing down the page output caused by the need to perform these operations. After the download of all the most important is completed, you can begin to download additional code. As a result, when the user needs this code, it will already be available to him. According to the
RAIL model, Google recommends running lazy loading script sessions of about 50 ms duration. With this approach, code loading operations will not affect user interaction with the page.
6. Avoid memory leaks
If a memory leak occurs in your application, this will result in the loaded page requesting more and more memory from the browser. As a result, the memory consumption of this page can reach such a level that it will badly affect the performance of the entire system. You yourself probably faced a similar problem (and you probably didn’t like it). It is possible that the page on which there was a memory leak contained some means of viewing images - such as a slider or a "carousel."
Using Chrome Developer Tools, you can analyze your site for memory leaks. This is done by examining the indicators using the Performance tab. Typically, memory leaks come from DOM fragments removed from the page but tied to some variables. This prevents the garbage collector from clearing the memory occupied by these unnecessary DOM fragments.
7. If you need to do some heavy computing, use web workers
From the materials of the MDN resource, you can find out that web workers can run code in a background thread that is separate from the main thread of the web application. The advantage of this approach is that heavy calculations can be performed in a separate thread. This allows the main thread (usually responsible for maintaining the user interface) to execute without blocking or slowing down.
Web Workers allow you to perform calculations that use the processor intensively, while not blocking the user interface stream. This technology allows you to create new threads and give them tasks, which has a beneficial effect on application performance. With this approach, tasks that take a long time to complete do not block the execution of other tasks. When performing similar tasks in the main thread, other tasks are blocked.
8. If you access the DOM element several times, save the link to it in a variable
Getting a reference to a DOM element is a slow operation. If you are going to access an element several times, it is best to save a link to it in a local variable. But here it is important to remember that if the element, the link to which is stored in the variable, is later removed from the DOM, you need to remove the link to it from the variable. For example, you can do this by writing
null
to a variable. This will avoid memory leaks.
9. Strive to declare variables in the same scope in which they will be used.
JavaScript, when trying to access a variable, first looks for it in the local scope. If it does not appear there, the search continues in the areas of visibility into which the local area of visibility is embedded. This happens until the global variables are checked. Saving variables in local scopes speeds up access to them.
Try, without special need, not to use the
var
keyword when declaring variables. Instead, use the
let
and
const
keywords to declare variables and constants, respectively. They differ in block scope and some other useful features. Pay attention to the use of variables in functions, trying to ensure that the variables that you access inside the function are local to it. Be aware of the troubles that implicit declaration of global variables can cause.
10. Try not to use global variables
Global variables exist during the whole time the script runs. Local variables are destroyed when the local scope is destroyed. Therefore, global variables should be used only when it is really necessary.
11. Apply code optimizations in JavaScript that you would apply to programs written in other languages
- Always use algorithms with the smallest possible computational complexity, solve problems using optimal data structures.
- Optimize the algorithms used to get the same results with fewer calculations.
- Avoid recursive calls.
- Design repeating pieces of computing as functions.
- Simplify math calculations.
- Use search arrays instead of switch / case constructs.
- Try to ensure that conditions checked in conditional constructions more often take true values. This contributes to more efficient use of processor capabilities for proactive execution of instructions.
- If you have the opportunity to use bitwise operators to perform certain actions, do this. Performing such calculations takes less processor resources.
12. Use application performance research tools
To explore various aspects of web projects, the Lighthouse tool can be recommended. He rates the application based on the following indicators: Performance, Progressive Web App, Accessibility, Best Practices, SEO. Lighthouse not only gives marks, but also gives recommendations for improving the project. Another productivity analysis tool,
Google PageSpeed , was created to help developers explore their sites and see how they can improve.
Both Lighthouse and PageSpeed are not perfect tools, but their use helps to see problems that, at first glance, may not be noticeable.
In the Chrome menu, you can find the command that opens the task manager. It displays information about system resources used by open browser tabs. You can obtain more detailed information about what is happening on the page by opening the Performance tab of the Chrome Developer Tools (other browsers also have similar tools). This tab allows you to analyze many indicators related to site performance.
Performance tab in Chrome Developer ToolsWhen you collect page performance information using Chrome, you can configure processor and network resources available to pages, which helps you identify and fix problems.
Chrome page performance analysisIn order to analyze the website in more depth, you can use the Navigation Timing API. It allows you to measure various indicators directly in the application code.
If you are developing server-side projects using JavaScript using Node.js, then you can use the NodeSource platform for an in-depth analysis of your applications. Measurements made using this platform have little impact on the project. In the Node.js environment, as in the browser, many problems can arise - like the same memory leaks. Analysis of projects based on Node.js helps to identify and fix problems with their performance.
Summary
It is important to maintain a balance between code optimization and readability. The code is interpreted by a computer, but people have to support it. Therefore, the code should be understandable not only to the computer, but also to the person.
In addition, it is useful to remember that performance should always be taken into account, but it should not be more important than ensuring error-free operation of the code and implementing the capabilities of applications that users need.
Dear readers! How do you optimize your JS projects?
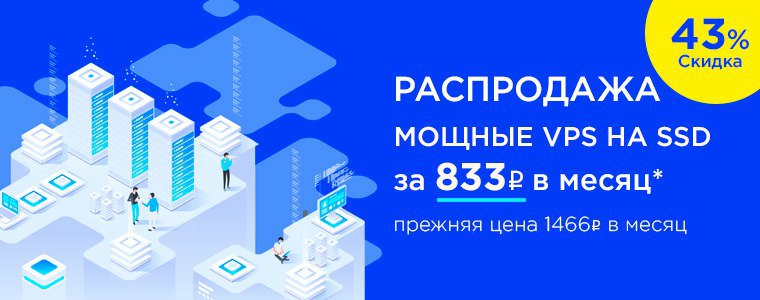