
Localization of products is very important for international companies developing new countries and regions for themselves. Similarly, localization is needed and mobile applications. If a developer starts an international expansion, it is important to allow users from another country to work with the interface in their native language. In this article we will create a React Native application using the
react-native-localize package.
Skillbox recommends: Online Profession Java Developer Educational Course.
We remind: for all readers of "Habr" - a discount of 10,000 rubles when writing to any Skillbox course on the promotional code "Habr".
Tools and skills
To understand this article, you need basic skills for working with React Native. To get acquainted with the settings of the working machine, you can
use the official instructions .
We need these versions of software tools:
- Node v10.15.0
- npm 6.4.1
- yarn 1.16.0
- react-native 0.59.9
- react-native-localize 1.1.3
- i18n-js 3.3.0
Getting started
We will create an application that will support English, French and Arabic. First, create a new project using react-native-cli. To do this, in the terminal you need to type this:
')
$ react-native init multiLanguage
$ cd multiLanguageAdd the necessary librariesFirst you need to install react-native-localize by typing the following:
$ yarn add react-native-localize
If the installation process has problems, you
should study the installation manual .
The react-native-localize library gives the developer access to multilingual functions. But she needs another library - i18n.
This article describes the use of
I18n.js in order to provide a translation in JavaScript.
$ yarn add i18n-jsWell, since i18n-js does not provide caching or memoization, I suggest using lodash.memoize for this:
$ yarn add lodash.memoizeWork with translationsIn order for the application to work skillfully with other languages, you first need to create a directory of translations within src, then three JSON files, for each of the languages.
1. en.json for English;
2. fr.json for french;
3. ar.json for Arabic.
These files contain JSON objects with keys and values. The key will be the same for each language. It is used by the application to display textual information.
The value is the text to be shown to the user.
English:
{"Hello": "Hello World!"}French
{"Hello": "Salut le Monde!"}Arab
{"Hello": "أهلاً بالعالم"}Other languages can be added in the same way.
Main code
At this stage, you need to open the App.js file and add import to it:
import React from "react"; import * as RNLocalize from "react-native-localize"; import i18n from "i18n-js"; import memoize from "lodash.memoize";
After that, auxiliary functions and constants are added, which will be useful later.
const translationGetters = {
And now let's create the App class component:
export default class App extends React.Component { constructor(props) { super(props); setI18nConfig();
The first element, setI18nConfig (), sets the initial configuration.
Then in the componentDidMount () you need to add listening to events, this element will track the updates and call handleLocalizationChange () when they occur.
The handleLocalizationChange () method activates setI18nConfig () and forceUpdate (). This is necessary for Android devices, since the component must be rendered so that the changes become noticeable.
Then you need to remove the audition from the componentWillUnmount () method.
Finally, hello is returned in render () by using translate () and adding the key parameter to it. After these actions, the application will be able to "understand" what language is needed, and show messages on it.
Application launch
Now it's time to check how the translation works.
First, run the application in a simulator or emulator, typing
$ react-native run-ios
$ react-native run-android
It will look something like this:

Now you can try to change the language to French by running the application then.

With the Arabic language doing the same thing, there is no difference.
So far, so good.
But what happens if you choose a random language that is not translated in the application?
It turns out that the task of findBestLanguage is to provide the optimal translation from all available. As a result, the default language will be displayed.
It's about phone settings. For example, in the iOS emulator, you can see the order of languages.
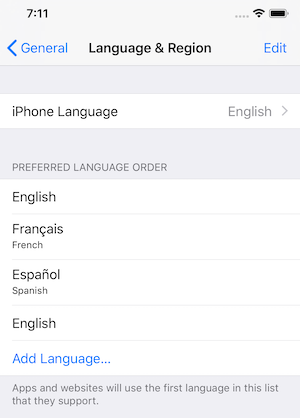
If the selected language is not preferred, findBestAvailableLanguage returns undefined, so the default language is displayed.
Bonus
React-native-localize has an API that provides access to a large number of language elements. Before you get started, you
should read the documentation .
findings
The application can be made multilanguage without any problems. React-native-localize is a great option that allows you to expand the circle of users of the application.
The source code of the project
is here .
Skillbox recommends: