I have been working with React for almost 3 years, I used both Redux and MobX and I have a question by now. Why do the absolute majority of front-end developers continue to firmly believe that Redux + Redux Saga + Reselect + 100,500 other libraries that “make life easier” is the best solution for today? I will give 4 arguments in favor of the fact that in the next project you use MobX instead of Redux.
MobX allows you to write cleaner and more understandable code.
Let's get 2 snippets of code that do the same thing. Here is what the reducer looks like in Redux:

To change the state, you need to call a function called action in Redox terminology:
')

And in most cases (not always, but these are the “best practices” that are used on many projects), you will need to write this boilerplate:

Then it will be necessary to initialize the store (this will have to be done once, but still):

And pass our initialized store further into the application through the provider (also a one-time operation):

Now you can perform some operations with the data in your components:

It turns out that in the case of Redux, in order to change the data in your storage, you have to call some functions that will create a new state object ... Personally for me, this sounds like complete nonsense. Let's take a look at the same functional MobX. Our site:

And then you can use in the component:

Yes, that's right, instead of some functions that modify objects, you can use the classic OOP approach, with classes, their properties and methods. Do not be afraid of decorators (@) inside, they simply add the functionality necessary to track data changes. By the way, a similar approach with classes for data storage is used in Angularjs (the screen is taken from here
angular.io/start/data ):

MobX allows you to write less code
To see this, just look at the examples above. And now, instead of writing an infinite boilerplate, you can finally focus on writing the business logic of the application, which is good news.
Third - performance optimization
If you look at the examples above, you can see that in the case of MobX I did not use the pure component and this is not an error. You simply do not need to use any optimization in this case, because your component will be re-rendered only when the data that you use in it changes. And yes, you can forget about Pure Components, shouldComponentUpdate and what else you use there in these cases. Ideally, each of your components that are not HOC and use some kind of data from the storage should be observable and then you will forget about problems with optimization forever.
Fourth - less dependencies
Anyone who uses Redux should not know by hearsay that there are a lot of “wonderful” bundles bundled with it. And well, if this is just thunk, and maybe so, the developers will follow the path of the
light of darkness and want to use Redux Saga, Reslect and a bunch of incomprehensible libraries that make your code not only slower, but also more difficult to understand. And to finish some insignificant functionality or to find a bug in this work will be incredibly difficult and long. MobX is the ultimate solution == does not require additional libraries, will deprive you of all these charms, so with it the business logic of your application will be as pure as a baby’s tear.
UPD. Thank you
mazaaaThe fifth reason - the ability to opt out of setState
setState has a number of shortcomings (a short translation of an article
that can be read here in the original ):
1. It is asynchronous.
This can lead to unexpected behavior:

On the screenshot above, the alert should have been 2, but since setState is asynchronous, it comes later.
2. setState leads to unnecessary re-renders of the component:
but. It is re-rendered, even if the new value == the old value
b. There are situations when a state change will not lead to any change, for example, when we have conditions for display in which there is a state. On the screenshot below, a pererender occurred during the click, although the data should not be rendered due to the fasle condition:

at. Sometimes the data that setState updates does not play a role in DOM rendering (for example, timers). And still the component rerender occurs.
3. setState is not suitable for all cases.
There are components that use the hooks / methods of the component's life cycles and in this case there will not only be an extra rerender, but these events (hooks) will be triggered each time, which can lead to strange behavior.
Using MobX will protect you from these shortcomings, because you can completely abandon setState:
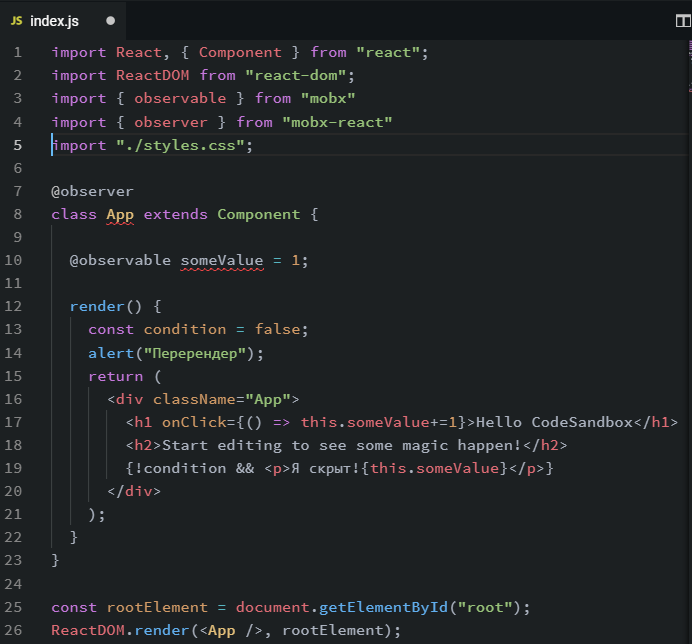
If you disagree with something or I don’t understand something, provide counter arguments in the comments. Link to the sandbox from which MobX screenshots are taken:
codesandbox.io/s/mobxreact-s7db5 , with Redux:
codesandbox.io/s/oj7px08qy9