I heard such an expression that in order to become a programmer, you need to be lazy. But sometimes laziness in programming leads to terrible technical debt. In my note on SRP, I mentioned that a violation of this principle can increase the complexity or even multiply it. One of my colleagues produced an interesting example, and I decided to demonstrate how it looks with his help.
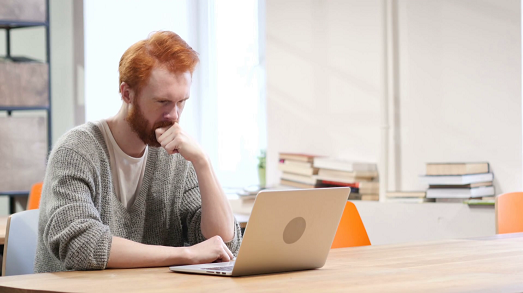
Let's define what this excess complexity is. But first, let's talk about its opposite, about the complexity of the requirements. For example, there are requirements to calculate the employee’s salary from the hourly rate and the hours worked. And, if the employee has been working in the company for more than five years, accrue a bonus. This “if” comes from the requirements and cannot be avoided. In one form or another, it will become an element of complexity in the application code, most likely in the form of a conditional “if” operator. But sometimes the complexity does not come from the requirements, but arises from the developer’s approach to solving the problem.
The “if” operator, patterns such as “strategy”, polymorphic methods are not a complete list of programming techniques capable of containing this excessive complexity. Personally, by the way, I am always against the application of patterns by developers simply because they can, and not to solve a specific problem.
')
Here is a simple example. It may seem fictional, but it is not. It is not even simplified, it was in this form that I met it during code review a couple of years ago. In two places in the code there were calls of the same function but with a different boolean parameter:
Such designs always look suspicious and this function did not disappoint me. This parameter was transmitted with the sole purpose to be checked inside this function:
doSomething(flag: boolean): void { if(flag) {
This test can be described as “if I was called from place A, we do one thing, otherwise I was called from place B, we do another”. This flag, this “if” is what the whole article is about. Difficulty not arising from business requirements. Naturally, I recommended changing the code as follows:
Everything, excess complexity is no more. It is here that the developer should not be too lazy and write another function signature.
Here you can exclaim: "But this is just one 'if'", or: "This violation is obvious, who in general writes this code?". And here comes the second example. It shows that it can be noticeably more difficult to see the violation, and that the cost of this violation can be more than just one “if”. As in the first example, the function is used in two places:
The method, as its name implies, checks the validity of the object. However, it was not obvious that he could also check the validity of an array of objects. I corrected the variable names to focus on this violation. The method looks like this:
checkValidity(parm: MyType | MyType[]): void { if(Array.isArray(parm)) { parm.forEach(p => checkValidity(p)); } else {
Here it is. One “if” becomes a set of “if” s. If the array contains 100 objects, then this “if” will be executed 101 times. And on real data we could have 30 thousand objects there, and this is already an impressive performance loss.
Obviously, following the principle of sole responsibility, this method needs to be refactored so that it turns out 2 methods:
checkItemsValidity(parms: MyType[]): void { parms.forEach(p => checkItemValidity(p)); } checkItemValidity(parm: MyType): void {
Accordingly, it is also necessary to correct the points of challenge.
Interestingly, the examples that I cited in the note on SRP led to an increase in the SLOC, these same examples, on the contrary, lead to a slight decrease, along with the expected improvement in the quality of the code.
That's all. Just a couple of simple examples to demonstrate the most important of the principles of good code.