Hello again. The translation of the following material has been prepared specifically for students of the
“Developer C ++” course, which classes will start on June 27th.
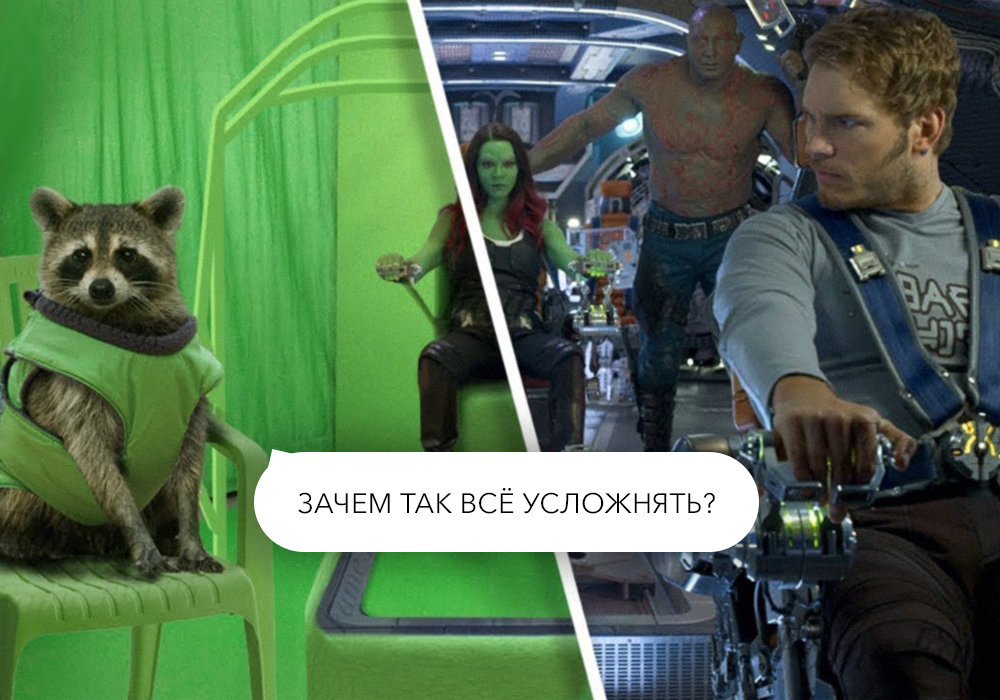
The Ranges library was adopted in C ++ 20 at the Standard Committee meeting in San Diego last November. The library provides components for processing ranges of values aimed at simplifying our code. Unfortunately, the Ranges library is not well documented, which makes it harder to understand for those who would like to master it. This post is intended to familiarize you with code examples written with and without Ranges.
An implementation of the Eric Niebler's Ranges library is available
here . It works with Clang 3.6.2 or newer, gcc 5.2 or newer, and VC ++ 15.9 or newer. The code examples below were written and tested with the latest compilers. It is worth noting that these examples are typical implementations and are not necessarily the only solutions that can be devised.
')
Although the standard namespace for the Ranges library is
std::ranges
, in this current implementation of the library it
ranges::v3
.
The following namespace aliases are used in the examples below:
namespace rs = ranges::v3; namespace rv = ranges::v3::view; namespace ra = ranges::v3::action;
Also, for simplicity, we will refer to the following objects, functions, and lambdas:
std::string to_roman(int value) { std::vector<std::pair<int, char const*>> roman { { 1000, "M" },{ 900, "CM" }, { 500, "D" },{ 400, "CD" }, { 100, "C" },{ 90, "XC" }, { 50, "L" },{ 40, "XL" }, { 10, "X" },{ 9, "IX" }, { 5, "V" },{ 4, "IV" }, { 1, "I" } }; std::string result; for (auto const & [d, r]: roman) { while (value >= d) { result += r; value -= d; } } return result; } std::vector<int> v{1,1,2,3,5,8,13,21,34}; auto print_elem = [](auto const e) {std::cout << e << '\n'; }; auto is_even = [](auto const i) {return i % 2 == 0; };
APDATE : I would like to thank Eric Niebler and all the others who commented below with suggestions for these code examples. I updated a few based on their feedback.Print all elements of the range:
Output all elements of the range in the reverse order:
Print only even elements of the range, but in reverse order:
Skip the first two elements of the range and output only even of the following three:
Print the numbers from 101 to 200:
Output all Roman numerals from 101 to 200. To convert a number to the corresponding Roman number, use the function
to_roman()
shown above.
Print the Roman numerals of the last three numbers, divisible by 7 in the [101, 200] range, in reverse order.
Create a string range containing the roman numerals of the last three numbers that are a multiple of 7 in the [101, 200] range, in the reverse order.
Modify the unsorted range so that it retains only unique values, but in reverse order.
Remove the two smallest and two largest range values and leave the rest, ordered in the second range.
Combine all rows in this range into one value.
Count the number of words (separated by a space) in the text.
Was the article helpful to you? Write in the comments.