The concept of time in the context of RTOS was introduced in one of the
previous articles , along with the idea of the time-related functions available in the RTOS.
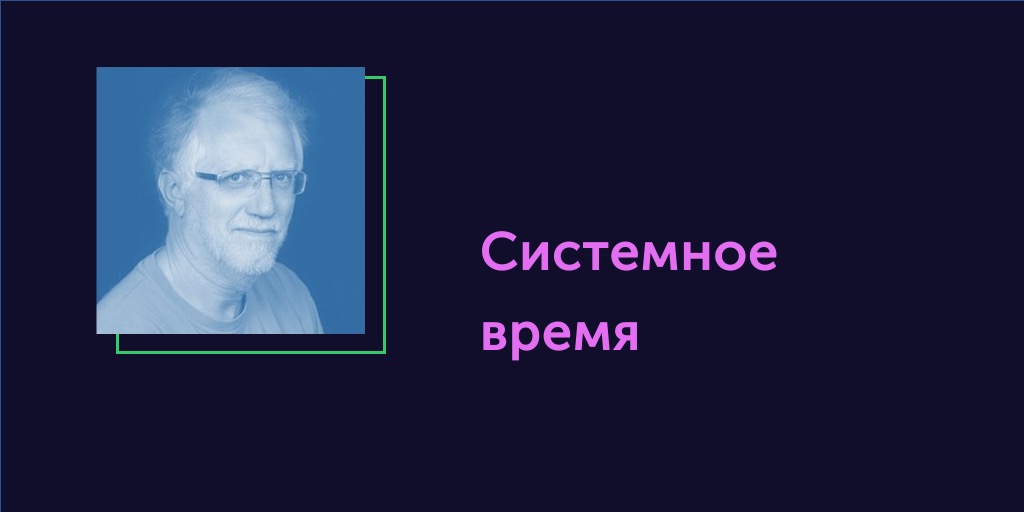
Previous articles in the series: Timer Tick
All functions related to time are controlled by the hardware clock. This is a simple oscillator that generates interrupt requests at regular intervals. For clock numbers to make sense for application programs, the generator frequency must be known.
Timer Interrupt Handling
Interrupts generated by the hardware timer must be serviced in a certain way in the interrupt handler (English Interrupt Service Routine, ISR), which implements all the RTOS functions related to time. The details of the timer interrupt handler in Nucleus SE will be covered in one of the following articles.
')
Time related functions
Nucleus RTOS and Nucleus SE contain several time-related mechanisms:
- System Tick Clock (Tick clock) : a simple counter that is incremented with a timer interrupt handler. Both in the Nucleus RTOS and in the Nucleus SE, the bit width is 32 bits, and tasks have mechanisms for reading and writing its value. In the Nucleus SE, the clock timer is optional.
- Software timers (Application timers) : Both Nucleus RTOS and Nucleus SE support timer objects. Their use and implementation in the Nucleus SE will be discussed in more detail in the next article.
- Planning time slicing (Time slice scheduling) : in the Nucleus RTOS, tasks with the same priority are served using the Round-robin algorithm, but other than that, you can use time slicing. In Nucleus SE, the time slicing scheduler is optional; this has been discussed in detail in previous articles ( general idea of the TS (Time slice) scheduler and TS in the Nucleus SE ).
- Pause task (Task sleep) : A task can pause itself (“fall asleep”) for a fixed period of time. This mechanism has already been described in detail earlier .
- API call timeouts (timeout) : in both Nucleus RTOS and Nucleus SE, some API calls allow you to pause a task while the resource is available. The suspension may be undefined, or, in the case of a Nucleus RTOS, an optional timeout period may be specified (waiting period). In Nucleus SE, API call timeouts are not supported.
Accuracy
And now it is worth briefly talking about the accuracy of the system timer.
The accuracy of the time functions is directly dependent on the frequency of the clock generator. For example, if the pulses arrive every 10 milliseconds, and the application task needs a delay of 100 milliseconds, it obviously needs 10 pulses. However, it is not known when the previous impulse was received: it could have just happened, or almost 10 milliseconds ago. Therefore, a 100 millisecond delay can take up to 110 milliseconds.
An obvious way to solve this problem is to increase the frequency of the generator. If pulses follow at intervals of 1 millisecond, a 100-ms delay will never take more than one-hundred-millisecond. The disadvantage of this solution is that the timer interrupt handler will take 10 times more CPU time, which will be excessive. The system developer must find a balance between the required accuracy of the timer and the available processor power.
Setting the system time
As with most Nucleus SE objects, the system time setting is mostly controlled by the
#define directives in the
nuse_config.h file. The main parameter is
NUSE_SYSTEM_TIME_SUPPORT , which activates the system time support mechanism. The number of objects does not need to be specified: the system time is either activated or not.
The choice of a non-zero value is the main activator of the system time. This parameter is used when defining data structures, which will be discussed in detail later in this article. In addition, a non-zero value activates the API settings.
API activation
Each API function (service call) in Nucleus SE has an activating #define directive in the nuse_config.h file. For system time, these characters are:
NUSE_CLOCK_SETNUSE_CLOCK_RETRIEVEBy default, they are assigned the value
FALSE , thus all service calls are disabled, blocking the inclusion of the code implementing them. To set the system time in the application, select the necessary API service calls and set them to
TRUE .
Below is the default code snippet from the nuse_config.h file.
#define NUSE_SYSTEM_TIME_SUPPORT FALSE /* */ #define NUSE_CLOCK_SET FALSE /* */ #define NUSE_CLOCK_RETRIEVE FALSE /* */
If you try to use the system time API service call when the system time activator is off, a compilation error will occur. If your code uses an API call that has not been activated, a build error will occur because the implementation code was not included in the application.
System Time Utility Calls
Nucleus RTOS supports two service calls that relate to system time and provide the following functionality:
- Set the system time value. The Nucleus SE is implemented as a NUSE_Clock_Set () function.
- Getting the system time value. In Nucleus SE, it is implemented in the NUSE_Clock_Retrieve () function.
Consider the implementation of each of these calls in more detail.
Service calls to set and get system time
With system time, you can perform only the installation operation at the specified value and get the current value. Nucleus RTOS and Nucleus SE each provide two basic API calls to implement these operations.
Interpretation of the system time value depends on the application, since it is in its essence a counter for the number of clock ticks that have occurred since the last reset of the counter. To use this information, the oscillator frequency must be known.
Time setting
Any task can set the system time by calling this API function.
Call to set the system time in Nucleus RTOSService Call Prototype:
VOID NU_Set_Clock (UNSIGNED new_value);Options:
new_value - the value that will be assigned to the system time
Return value: none.
Call to set the system time in Nucleus SEThis API call supports the core functionality of the Nucleus RTOS API.
Service Call Prototype:
void NUSE_Clock_Set (U32 new_value);Options:
new_value - the value that will be assigned to the system time
Return Value: None
Implementing a time setting in the Nucleus SEThe code is very simple. The provided value is written to
NUSE_Tick_Clock inside the critical section.
Getting system time
A task can get the system time value using this API function.
Call for getting system time in Nucleus RTOSService Call Prototype:
UNSIGNED NU_Retrieve_Clock (VOID);Parameters: None
Return Value: current system time
Call to get system time in Nucleus SEService Call Prototype:
U32 NUSE_Clock_Retrieve (void);Parameters: None
Return Value: current system time
Implement getting time in Nucleus SEThe code is very simple. The function returns the
NUSE_Tick_Clock value obtained in the critical section.
Data structures
System time uses a single data structure (located in RAM), which is a 32-bit word.
I strongly recommend that the application code does not use direct access to this data structure, but access it through the provided API functions. This will avoid incompatibility with future versions of the Nucleus SE and unwanted side effects, as well as simplify porting applications to the Nucleus RTOS. Detailed information about the data structures is provided below to simplify the understanding of how the service call code works and for debugging.
RAM data
Data structure:
NUSE_Tick_Clock is a
U32 variable in which the system clock clock counter is stored.
This data structure is initialized to zero by the
NUSE_Init_Task () function when the Nucleus SE is started. One of the following articles will contain a full description of how Nucleus SE runs.
ROM data
There are no system time data structures in ROM.
Memory for system time
As with all other Nucleus SE objects, the amount of memory required for system time is predictable.
The amount of memory in the ROM is 0.
The amount of memory in RAM (in bytes) is always 4.
Unrealized API calls
All Nucleus RTOS API service calls related to system time have an equivalent in Nucleus SE.
Nucleus PLUS Compatibility
As with all other Nucleus SE objects, my goal was to ensure the highest possible compatibility of application code with Nucleus RTOS. System time is no exception and, from the user's point of view, it is implemented in much the same way as in the Nucleus RTOS. Nucleus RTOS API calls can be directly transferred to the Nucleus SE.
In the next article we will look at software timers.