This is a translation of an article by Chidume Nnamdi, published on blog.bitsrc.io. The translation is published with the permission of the author.

The advent of the RxJS library has opened up a host of new opportunities in the JS world. The goal of RxJS is to achieve a lot with a small amount of code. After reading this article, you will learn how to exchange data between application components on React, using the features of RxJS.
')
Tip : Use
Bit to organize and share React components. This will allow your team to develop their applications faster. Just try it.

React Components Collection
Redux
Data exchange between unrelated React components is what state management libraries were created for. There are many templates for managing states, but the most famous are two: Flux and Redux.
Redux is popular for its simplicity and the use of pure functions. After all, thanks to them, there is no doubt that the use of reduser will not lead to any side effects.
Working with Redux, first of all we create a centralized data repository:

Next, we associate the components with this repository and, if desired, update or delete the state. Any changes made to the repository will be reflected in the components associated with it. Thus, the data flow extends to all components, regardless of their nesting degree. A component located at the nth level of the hierarchical structure is capable of transmitting data to a component of the highest level. The latter, in turn, can transfer data to a component of level 21.
Rxjs
With the advent of RxJS, using state management libraries has become much easier. Many liked the Observer pattern provided by RxJS.
We simply create the
Observable
stream and enable all components to listen on it. If a component is added to a stream, the listening (or “signed”) components respond to a DOM update.
Installation
Create an application on React using
create-react-app
. If you do not have
create-react-app
, then first install it globally:
npm i create-react-app -g
Next, generate the project in React:
create-react-app react-prj
Go to the directory:
cd react-prj
Install the rxjs library:
npm i rxjs
We should have a file that creates a new
BehaviourSubject
instance.
Why do we use BehaviorSubject?
BehaviorSubject
is one of the Subjects in the RxJS library. As a child component of the Subject, the
BehaviorSubject
allows multiple observers to listen to the stream, and also does a mass mailing of events to these observers.
BehaviorSubject
saves the last value and sends it to all new subscribed components.
Thus,
BehaviorSubject
:
- Allows bulk mailing.
- Stores the latest values ​​published by subscribers, and does a mass mailing of these values.

The src folder contains the
messageService.js
file, which exports a
BehaviorSubject
instance and a
messageService
object to the
messageService
. A subscriber object is created at the beginning of the file - so it is available for any importing component. The
messageService
object has a send function that takes an
msg
parameter: it contains the data that is needed to pass to all listening components. In the body of the function, we call the
emit
method. It bulk sends data to subscribed components in a subscriber object.
Suppose we have the following components:
- ConsumerA;
- ConsumerB;
- ProducerA;
- ProducerB.
In a hierarchical structure, they look like this:

The application component transmits the message ProducerA and ConsumerB. ProducerA sends the data to ConsumerA, and the message from ConsumerB goes to ProducerB.

The components ConsumerA and ConsumerB have an individual status counter. In their
componentDidMount
method, they are subscribed to the same
subscriber
stream. As soon as an event is published, the counter is updated for both components.
ProducerA and ProducerB have the
Increment Counter
and
Decrement Counter
, which, when pressed, give out
1
or
-1
. The signed ConsumerA and ConsumerB components pick up the event and start their callback functions by updating the value of the state counter and the DOM.
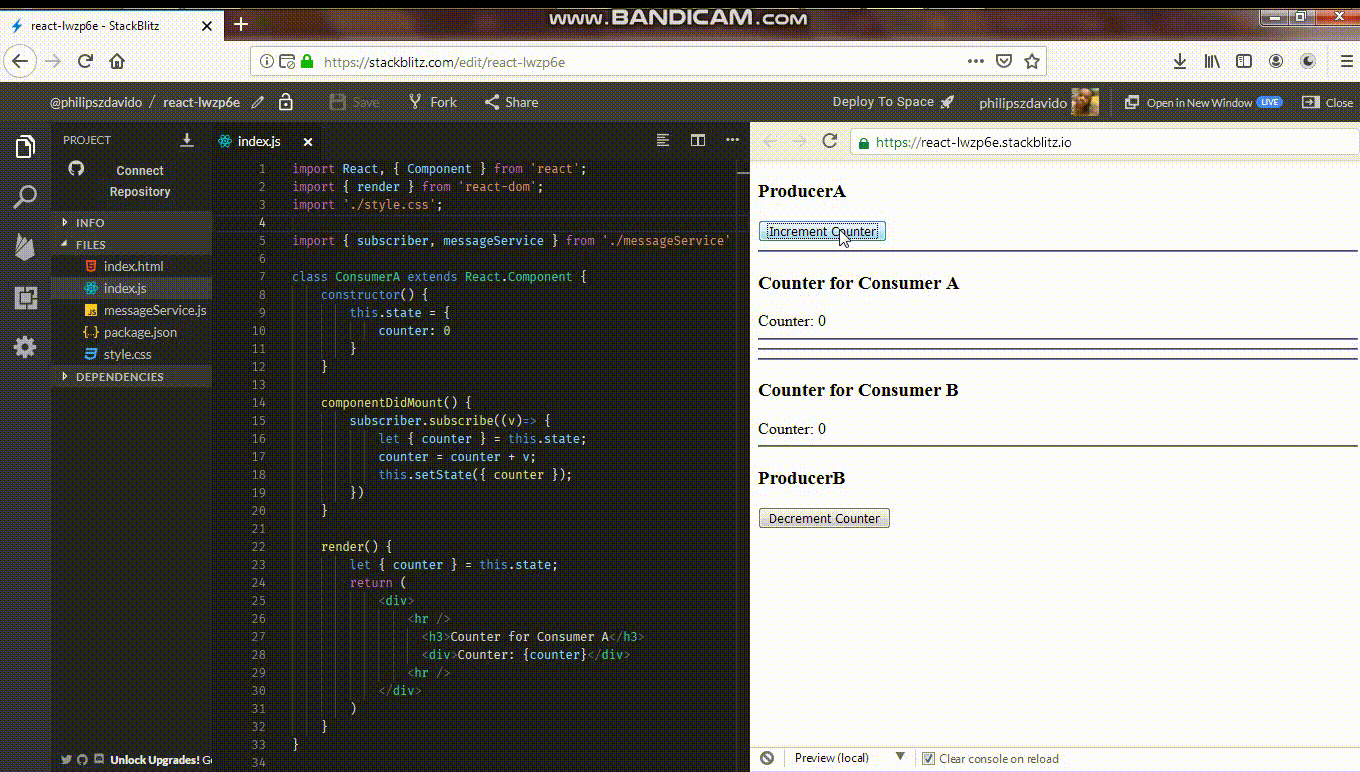
Let's look at the hierarchical structure again:

ProducerB transmits ConsumerA data, although they are completely unrelated. ProducerA transfers data to ConsumerB, not being its parent component. That's the whole point of RxJS: we just created the central node of the event flow and let the components listen on it. When a component generates events, the listening components immediately pick them up.
Play with the application on stackblitz:
https://react-lwzp6e.stackblitz.ioConclusion
So, we saw how data can be exchanged between React components using RxJS. We used the
BehaviourSubject
to create a centralized data stream, and then allowed the rest of the components to subscribe to this stream. Now, when one of the components generates data, the other components also receive it. The level of components in the hierarchical structure is unimportant.
If you have questions about this topic or you want me to add, correct or delete something,
write about it in the comments, in an email or in a personal message.
Thanks for attention!