
Among the variety of tools announced on
Android Dev Summit , I would like to pay special attention to the update mechanism of the In-App Updates application (IAUs), which helps developers to speed up the addition of new features, bug fixes and performance improvements. Since this functionality was
published after Google I / O 2019, in this article I will talk in detail about IAUs, describe the recommended implementation schemes, and give some code examples. I will also talk about our experience in integrating IAUs into
Pandao , an application for ordering goods from China.
The new API allows developers to initiate an application update to the latest version available on Google Play. Thus, IAUs complements the already existing Google Play automatic update mechanism. IAUs contains several implementation schemes that are fundamentally different in terms of user interaction.
- Flexible Flow offers users to download the update in the background and install it at a user-friendly time. It is intended for cases where users can still use the old version, but a new one is already available.
')
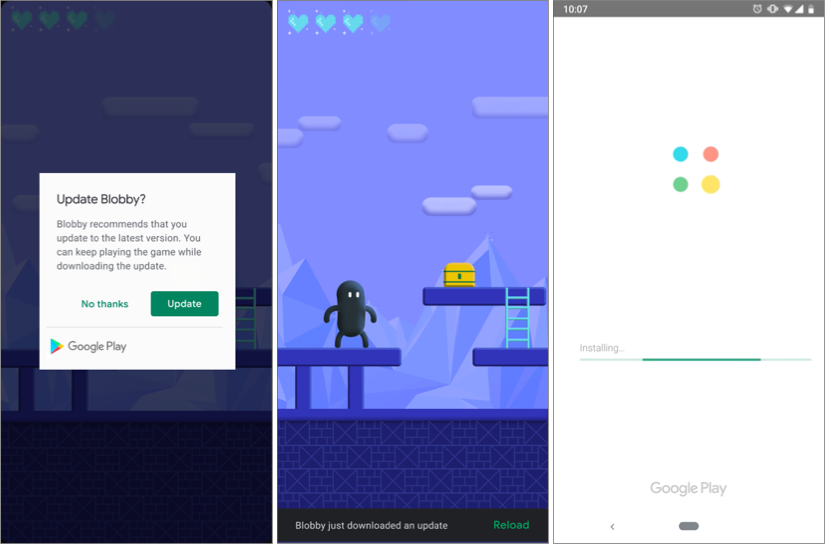
- Immediate Flow requires users to download and install the update before continuing to use the application. It is intended for cases where it is critical for developers to update the application.

Since the second option is not so important and less suitable for the Pandao application, we will examine in more detail the Flexible Flow scenario.
IAUs Flexible Flow Integration
Use cases
The process of updating using IAUs consists of several steps.
- An application using the Play Core library, which checks on Google Play if there are any updates available.
- If they are, then the application asks Google Play to show the IAUs dialog. Google Play shows the user a dialog asking for an update.
- If the user agrees, Google Play in the background downloads the update, showing the progress of the download to the user in the status bar.
- If the download is complete when the app is running in the background, Google Play automatically completes the installation. If the application is active at this time, then for such cases you need to define your own logic for the completion of the installation. Consider the following scenarios.
- The application starts the installation process by showing the user a Google Play dialog with a progress indicator. After the installation is completed, an updated version of the application is launched. In this case, it is recommended to display an additional dialog that will allow the user to confirm that he is ready to restart the application now. This is the recommended implementation .
- The application waits until it is in the background, and then completes the update. On the one hand, this is less intrusive behavior from the point of view of UX, since the user’s interaction with the application is not interrupted. But on the other hand, it requires the developer to implement logic to determine if the application is in the background.
If the installation of the downloaded update was not completed, then Google Play may complete the installation in the background. It is better not to use this option explicitly, because it does not guarantee the installation of the update.
Basic Testing Requirements
To manually perform the entire update process on a test device, you need to have at least two versions of the application with different build numbers:
source and
target .
- The original version with a higher number should be published on Google Play, it will be identified by Google Play as an available update. The target version with a lower build number and integrated IAUs must be installed on the device, we will update it. The bottom line is that when an application asks Google Play to check for an update, it will compare the build numbers of the installed and available version. So IAUs will only be launched if the build number on Google Play is higher than the current version on the device.
- The source and target versions must have the same package name and must be signed with the same release certificate .
- Android 5.0 (API level 21) or higher.
- Library Play Core 1.5.0 or higher.
Code example
Here we look at sample code for using IAUs Flexible Flow, which can also be found in the
official documentation . First you need to add the Play Core library to the build.gradle file at the module level.
dependencies { ... implementation "com.google.android.play:core:1.5.0" }
Then create an instance of
AppUpdateManager
and add a callback function to
AppUpdateInfo
, which will return information about the availability of the update, the object to start the update (if available) and the current download progress, if it has already begun.
To show a dialog for requesting an update from Google Play, you must pass the resulting
AppUpdateInfo
object to the
AppUpdateInfo
method.
appUpdateManager.startUpdateFlowForResult(
To track the status of an update, you can add an
InstallStateUpdatedListener
event listener to the IAUs manager.
As soon as the update is downloaded (
DOWNLOADED
status), you need to restart the application to complete the update. Restarting can be initiated by calling
appUpdateManager.completeUpdate()
, but before that it is recommended to show the dialog box so that the user explicitly confirms that he is ready to restart the application.
Snackbar.make( rootView, "An update has just been downloaded from Google Play", Snackbar.LENGTH_INDEFINITE ).apply { setAction("RELOAD") { appUpdateManager.completeUpdate() } show() }
Error "Update is Not Available"
First, recheck the requirements listed in the “Basic Implementation Requirements” section. If you have done everything, but the update according to the
onSuccess
call is still not available, then the problem may be caching. It is likely that the Google Play application does not know about the available update due to the internal caching mechanism. To avoid this with manual testing, you can forcibly reset your cache by going to the “My Apps and Games” page on Google Play. Or you can simply clear the cache in the settings of the Google Play application. Please note that this problem occurs only during testing, it should not affect the end users, since their cache is still updated daily.
IAUs Flexible Flow in the Pandao app
We participated in the early access program and integrated IAUs Flexible Flow (recommended implementation) into the Pandao app, a platform on which manufacturers and vendors can trade Chinese goods. The IAUs dialog was displayed on the main screen, so that the maximum number of users could interact with it. Initially, we wanted to show the dialogue no more than once a day, so as not to distract people from interacting with the application.
Since A / B testing plays a key role in the life cycle of any new feature, we decided to evaluate the effect of IAUs in our application. We randomly divided users into two non-overlapping groups. The first was a control one, without using IAUs, and the second group was a test one; we showed the IAUs dialogue to these users.
IAUs Flexible Flow A / B test in the Pandao app.Over the past several releases, we have measured the proportion of active users of each version of the application. It turned out that among the active users with the latest version available at that time, the main part consisted of participants from group B, that is, with the IAU function. The purple line on the graph shows that in the first days after the publication of version 1.29.1, the number of active users with IAUs exceeded the number of users without this function. Therefore, it can be argued that users with IAUs update the application faster.
Dialogue IAUs Flexible Flow in the Pandao application.According to our data (see chart above), users click the confirmation button most of all in the IAUs dialog in the first days after the release, and then the conversion is constantly decreasing until the next version of the application is published. The same is observed with the install button in the dialog box that initiates the installation of the downloaded update. Therefore, it can be said that the average conversion in both cases is directly proportional to the frequency of releases. In Pandao, the average conversion within one month reaches 35% for a click on the confirm button and 7% for a click on the install button.
We assume that the decrease in the share of confirmations over time is only a problem of user experience, because people who are interested in the new version will be updated fairly quickly, and those who are not interested in updating will not be interested. Based on this assumption, we decided not to disturb those who are not interested in updating, and not to ask them every day. A good practice would be to use a different query logic, which is based on “obsolescence”, that is, in order not to disturb users, we estimate how much older versions they have and how often we have already offered them to upgrade.
In general, IAUs performed well during A / B testing, so we rolled out IAUs for all users.
Acknowledgments
Thanks for the help in writing the article to Marina Pleshkova
Maryna_Pliashkova , Alexander Cherny
alexchernyy , Ilya Nazarov
RolaRko , Gleb Bodyachevsky, Daniil Polozov
jokerdab , Anastasia Kulik, Vladislav Breus and Vladislav Goldin
Vladiskus .