I want to share an interesting find -
Bridge.net . In a nutshell, this is a framework that allows you to translate C # code in JavaScript. The idea that drives the development team is very simple and straightforward - translate the logic as identically as possible. What is good if you remember all the differences and the specifics of these languages.

Perhaps everyone can find their personal use, but I would like to mention those moments that attracted me personally:
- The possibility of application for mobile development. JavaScript works on all platforms
- Applicability for the Web. Especially if you need to rewrite an outdated project, for example, in the form of a SPA application
- The ability to reuse C # code at the server and client side simultaneously
- Translation run during project build
- The project is under active development, there is adequate support from the developers
- Bridge.net is an OpenSource project with a license for commercial use ( Apache 2.0 license )
As a demonstration of using Bridge, I want to share a solution to the task of validating fields in a Web application. I hope the example will be clear, because such a task can occur quite often. For simplicity I will use ASP.NET Web Forms.
')
So, we have a field in which the user must enter the registration number of the car and click on the button. In our case, the button will check for correct input (in real life, we could return, for example, registered fines).
Server input validation logicprotected void submitButton_OnClick(object sender, EventArgs e) { var isCorrect = IsCorrectPlateNumber(plateTextBox.Text); plateTextBox.BackColor = isCorrect ? Color.LightGreen : Color.Coral; } private static bool IsCorrectPlateNumber(string plateNumber) { var success = false; if (!string.IsNullOrWhiteSpace(plateNumber)) { plateNumber = plateNumber.Trim(); if (plateNumber.Length >= 8 || plateNumber.Length <= 9) { var rgx = new Regex(@"^[]\d{3}[]{2}\d{2,3}$"); success = rgx.IsMatch(plateNumber); } } return success; }
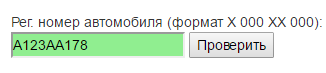
Well, now we want to reduce the server load and validate the field on the client side. There are several options here:
1) Re-write client validation , which will check the most simple scenarios, so to speak, "protection against a fool." Basic checks will still be performed on the server side.
Simple client validation . ( X 000 XX 000):<br/> <asp:TextBox ID="plateTextBox" runat="server"/> <asp:Button ID="submitButton" runat="server" Text="" OnClientClick="return validatePlate();" OnClick="submitButton_OnClick"/>
function validatePlate() { var value = document.getElementById('<%=plateTextBox.ClientID%>').value; if (value && value.length) { return true; } alert(" . !"); return false; }
2) Port server validation , as far as possible, thereby repeating the code. The advantages are obvious - the minimum discrepancy between the validation results on the server and on the client. There are also disadvantages - repeating the server logic, I would also like to keep it up to date, which can add headaches. I will not manually rewrite the code, it is quite understandable. Let's better see how Bridge would help us. To do this, use the
online editor . The translated code will look like this:
Full client validation (function (globals) { "use strict"; Bridge.define('Demo.ServerLogic', { statics: { isCorrectPlateNumber: function (plateNumber) { var success = false; if (!Bridge.String.isNullOrWhiteSpace(plateNumber)) { plateNumber = plateNumber.trim(); if (plateNumber.length >= 8 || plateNumber.length <= 9) { var rgx = new Bridge.Text.RegularExpressions.Regex("constructor", "^[]\\d{3}[]{2}\\d{2,3}$"); success = rgx.isMatch(plateNumber); } } return success; } } }); Bridge.init(); })(this);
It remains for us to
connect the Bridge.js file (for simplicity, I took the script file from the online editor), and also
slightly modify our initial validation function:
function validatePlate() { var value = document.getElementById('<%=plateTextBox.ClientID%>').value; if (Demo.ServerLogic.isCorrectPlateNumber(value)) { return true; } alert(" . !"); return false; }
3) Reuse logic. If we want to keep it up to date, then we can configure the translation of validation from C # to JavaScript to be performed with each build of the project. Thus, the logic really becomes reusable, programmers do not waste their time, the application becomes more stable, and users are even happier! To do this, you need to perform several steps:
Embedding Bridge in the project1. Create a
WebApplication1.Bridge project in the existing solution
.2. Install the
Nuget package into it. Install this package in a separate project, because installation removes conflicting References.

3. In the
WebApplication1 project, we
move the logic to a separate file / class. Accordingly, now this class will be used in the event handler for pressing the button (the changes are clear, I don’t cite the code).
ServerLogic.cs using System.Text.RegularExpressions; namespace WebApplication1 { public class ServerLogic { public static bool IsCorrectPlateNumber(string plateNumber) { var success = false; if (!string.IsNullOrWhiteSpace(plateNumber)) { plateNumber = plateNumber.Trim(); if (plateNumber.Length >= 8 || plateNumber.Length <= 9) { var rgx = new Regex(@"^[]\d{3}[]{2}\d{2,3}$"); success = rgx.IsMatch(plateNumber); } } return success; } } }
4. Add the created file to the
WebApplication1.Bridge project
as a link5. Change the output directory for the
WebApplication1.Bridge project. To do this, configure the file
bridge.json :
"output": "../WebApplication1/Scripts/Bridge"
6. After building in Solution Explorer, click Show All Files and we will be able to see the generated
webApplication1.js script
7. Add the necessary links and update the scripts on the
Demo.aspx page:
Demo.aspx scripts <script src="Scripts/Bridge/bridge.js" type="text/javascript"></script> <script src="Scripts/Bridge/webApplication1.js" type="text/javascript"></script> <script type="text/javascript"> function validatePlate() { var value = document.getElementById('<%=plateTextBox.ClientID%>').value; if (WebApplication1.ServerLogic.isCorrectPlateNumber(value)) { return true; } alert(" . !"); return false; } </script>
8. Everything. You can build a project and run it. Now, any changes to the validation logic will automatically work for both server and client checks.
So, on a simple example, we saw how you can easily reuse C # code, even when it needs to be done using JavaScript. And that may be of greater value - how this approach is embedded in the process of developing and building a Web application. However, this is not the only advantage of Bridge.net. I see him as an indisputable assistant in cross-platform mobile development, especially for those who can't imagine their life without .NET, but more on that next time!