At some point in my life, I realized that in order to be happy, I need to create 10 pages of copies of myself on Vkontakte and be simultaneously added as friends to people from the freelist of my main page. For details of the implementation of the idea - I ask under the cat.

Project Goals
- Make 10 pages in Vkontakte
- Copy avatar from main page
- Copy all posts from the main page
- Copy all videos from main page
- Add to all groups of the main page
- Make a full graph of friends among bots (like every entry and add each bot to friends)
- Add to each of 80 people from a pre-compiled list of friends
check in
To create pages, I used the sms-reg.com service, more information about SMS reception services is well written
here .
We register all 10 pages and write their logins / passwords into the accounts.json file (where id is the id of the registered page and zero_id is the id of our main page):
{ "zero_id": "21102****", "accounts": [ { "phone": "7985002****", "password": "tTod********", "id": 53980**** }, { "phone": "7985317****", "password": "tglh********", "id": 53980**** }, ... { "phone": "7916033****", "password": "ZsRq********", "id": 54038**** } ] }
python + vk_api
I will work with the library vk_api.
')
pip install vk_api --user
Let's create the bots.py file, it will be useful to us for other scripts:
import json import vk import vk_api from captcha import captcha_handler with open('accounts.json', 'r') as file: data = json.loads(file.read()) accounts = data['accounts'] zero_id = int(data['zero_id']) vk_apis = [] def init_apis(): current_id = 0 for account in accounts: vk_session = vk_api.VkApi(account['phone'], account['password'], captcha_handler=captcha_handler) vk_session.auth() vk_apis.append(vk_session.get_api()) print('account', current_id, 'successfully inited') current_id += 1 init_apis()
It reads accounts.json and initializes api for each of the accounts.
Copy avatar
def change_photo(vk): upload_url = vk.photos.getOwnerPhotoUploadServer()['upload_url'] answer = json.loads(requests.post(upload_url, files={'photo': open('avatar.jpg', 'rb')}).text) vk.photos.saveOwnerPhoto(photo=answer['photo'], server=answer['server'], hash=answer['hash']) counter = 0 for vk in vk_apis: change_photo(vk) print(counter, 'done') counter += 1
Video copying
videos = default_vk.video.get(owner_id=zero_id, count=100) counter = 0 for vk in vk_apis: for video in videos['items']: vk.video.add(video_id=video['id'], owner_id=video['owner_id'])
Add to groups
from time import sleep from bots import vk_apis, zero_id, accounts, default_vk groups = default_vk.groups.get(user_id=zero_id, count=100) counter = 0 for vk in vk_apis: for group in groups['items']: print('add to', group) vk.groups.join(group_id=group) print(counter) counter += 1
Creating a complete friend graph

counter = 0 for vk, account in zip(vk_apis, accounts): for friend in accounts: if friend['id'] == account['id']: continue vk.friends.add(user_id=friend['id'], captcha_handler=captcha_handler) print(counter, friend['id'], account['id']) counter += 1 sleep(1)
Creating a full graph of likes
counter = 0 for vk, account in zip(vk_apis, accounts): for friend in accounts: if friend['id'] != account['id']: continue print(counter, friend['id'], account['id']) for post in vk.wall.get(owner_id=friend['id'])['items']: flag = True while flag: try:
Add friend
create a friends file in which we write in every line
id_ #_ ( )
Vkontakte allows you to add about 80 people per day.
friends_str = '' with open('friends', 'r') as file: friends_str = file.read() friends = [{'id': x.split(' #')[0], 'name': x.split(' #')[1]} for x in friends_str.split('\n')] ids_ = ([x['id'] for x in (default_vk.users.get(user_ids=[x['id'] for x in friends]))]) for friend, id_ in zip(friends, ids_): print(friend['name']) print(friend) for vk in vk_apis: flag = True while flag: try: vk.friends.add(user_id=int(id_)) flag = False except: print('error') sleep(10)
Bypass captcha
The attentive reader has already seen the line
from captcha import captcha_handler
Since Vkontakte does not always like such a number of requests, it is necessary to process captcha. There are a huge number of services that provide such an opportunity. You just need to send a post request with captcha to base64 and wait.
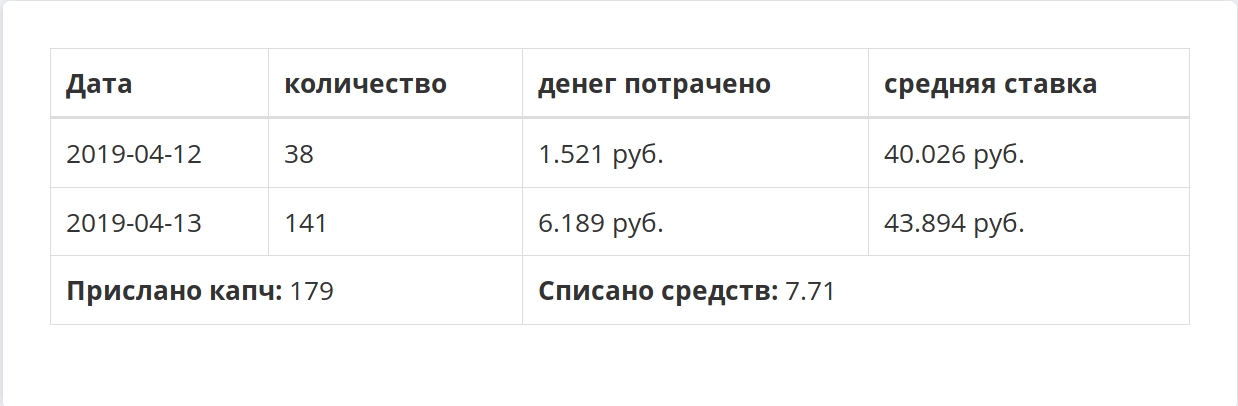
def uncapcha(url): imager = requests.get(url) r = requests.post('http://rucaptcha.com/in.php', data = {'method': 'base64', 'key': RUCAPTCHA_KEY, 'body': base64.b64encode(imager.content)}) if (r.text[:3] != 'OK|'): print('captcha failed') return -1 capid = r.text[3:] sleep(5) capanswer = requests.post('http://rucaptcha.com/res.php', data = {'key': RUCAPTCHA_KEY, 'id':capid, 'action':'get'}).text if (capanswer[:3] != 'OK|'): print('captcha failed') return -1 return capanswer[3:]
results
Here are some screenshots of what people sent me.
githubThe main question remains open - why did I do it? I have no idea.