Today, in the final part of the translation of the course on React, we will talk about the modern possibilities of React and discuss the ideas of React-applications, creating which you can repeat what you have learned, passing this course, and learn many new things.
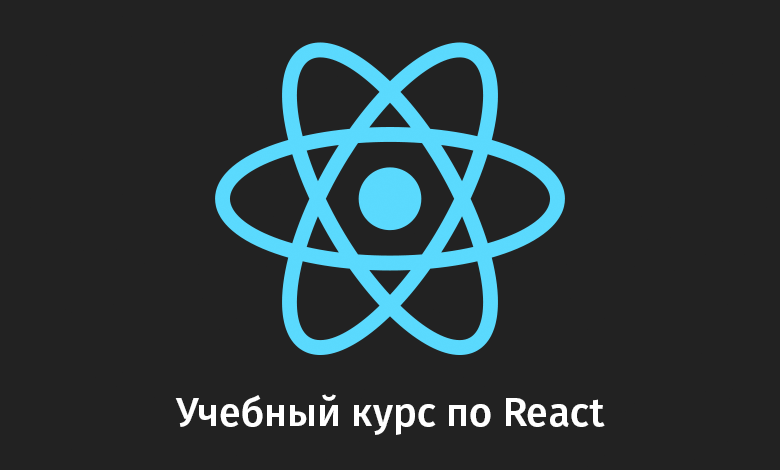
→
Part 1: Course Overview, React, ReactDOM, and JSX Reasons→
Part 2: Functional Components→
Part 3: Component Files, Project Structure→
Part 4: Parent and Child Components→
Part 5: Getting Started on a TODO Application, Basics of Styling→
Part 6: Some of the features of the course, JSX and JavaScript→
Part 7: Inline Styles→
Part 8: continued work on the TODO application, familiarity with the properties of the components→
Part 9: Component Properties→
Part 10: Workshop on working with the properties of components and styling→
Part 11: dynamic markup generation and the map array method→
Part 12: workshop, the third stage of work on the TODO application→
Part 13: Class Based Components→
Part 14: Workshop on Class Based Components, Component State→
Part 15: workshops on working with the state of components→
Part 16: the fourth stage of work on a TODO application, event handling→
Part 17: the fifth stage of working on a TODO application, modifying the state of components→
Part 18: Sixth Stage of Work on a TODO Application→
Part 19: Component Life Cycle Techniques→
Part 20: The first lesson in conditional rendering.→
Part 21: the second lesson and workshop on conditional rendering→
Part 22: the seventh stage of work on a TODO application, loading data from external sources→
Part 23: First Form Lesson→
Part 24: Second Form Lesson→
Part 25: Workshop on working with forms→
Part 26: Application Architecture, Container / Component Pattern→
Part 27: course project→
Part 28: Modern React Features, Project Ideas, Conclusion→
The end!Lesson 46. Developing Modern React Applications
→
Original')
A lot of Facebook programmers are working on the React library; members of the large community around React also contribute to the project. All this leads to the fact that React is developing very quickly. For example, if you, while studying React in early 2019, looked at the materials on this library, say, two years ago, you could not help noticing the changes that occurred in React since the release of those materials. For example, in React 16.3 some new methods for the life cycle of components have appeared, and some methods have been recognized as obsolete. And, say, in React 16.6, there are even more new features. A huge number of innovations are expected in React 17.0 and in the next versions of this library.
Now we will talk about some of the modern features of React.
Many of these features depend on which version of the ECMAScript specification is supported by the tools used when developing a React project. Say, if you use the Babel transpiler, this means that you have the latest JavaScript features available. At the same time, it should be noted that when using in projects certain features of JavaScript that are not yet included in the standard, you may encounter the fact that they, if included in the standard, may change.
One of the modern features of JavaScript, which can be used in the development of React-applications, is the ability to declare class methods using the syntax of arrow functions.
Here is the
App
component code that displays a text field:
import React, {Component} from "react" class App extends Component {
Here is the page of this application in the browser.
Application in browserLet's rewrite the
handleChange()
method as an arrow function, bringing the component code to the following form:
import React, {Component} from "react" class App extends Component {
In the course of this conversion, small changes have been made to the code, but these changes have a major impact on how the method will work. The keyword
this
in the arrow functions indicates the lexical scope in which they are located. These functions do not support
this
binding. This feature of the switch functions leads to the fact that the methods declared with their use do not need to be tied to
this
in the class constructor.
Another possibility that we will consider here is to use the properties of classes. Now we, when initializing the state in the constructor, use the
this.state
instruction. So we create a class instance property. Now properties can be created outside of the constructor. As a result, you can convert the code as follows:
import React, {Component} from "react" class App extends Component {
Here we got rid of the constructor, initializing the state when declaring the corresponding property.
All indications are that this JS feature will be included in the standard in the foreseeable future.
Here is a list of materials on modern features of React.
In general, it can be noted that since React is developing very quickly, everyone who is engaged in React-development is recommended to constantly monitor the innovations of this
library .
Lesson 47. Ideas React-projects
→
OriginalDuring the development of React, we have created a couple of projects - a Todo application and a meme generator. It is possible that you already know what you want to create using React. Maybe you are already developing your own application. If you have not yet made your choice, and considering that practice is the best way to master computer technologies -
here ,
here and
here - materials in which you will find a whole bunch of web application ideas that you can create with React.
Lesson 48. Conclusion
→
OriginalCongratulations! You have just completed a course study on the React library. You are familiar with the basic building blocks of React-applications, which you can already use to create your own projects. True, if you want to create something using React, be prepared for what you have to learn a lot more new things.
Let's walk on the basic concepts that you have learned in the course of this course.
- Jsx JSX allows you to describe user interfaces using syntax that is very similar to regular HTML code.
- Two approaches to the development of components. Class-based and functional components.
- Different ways of styling React-applications.
- Passing properties from parent components to child components.
- Using state components to store data and to work with them.
- Conditional rendering.
- Work with forms in React.
We thank you for your attention!
Dear readers! Please share your impressions of this course and tell us what you want to develop (or are already developing) using React.