Introduction
Recently, actively learning the programming language Python. I was especially interested in using Python in recognizing and classifying faces. In the article, I will try to apply face recognition for the Big Bang Theory series.

A little about the seriesThe series tells the story of two young talented physicists (Sheldon Cooper and Leonard Hofstedter), their attractive neighbor on the landing, a waitress and aspiring actress Penny, and their friends astrophysics Radzhesh Kutrappali and engineer Howard Volovice. The action of the series takes place in Pasadena, California. At the moment, shot 265 episodes (12 seasons).
IMDB rating - 8.2.
I am a non-professional programmer. Programming is my hobby. All code is terrible, but I'm learning and trying to make it better.As a tool for recognizing and classifying faces, I used the
Face Recognition Project on MXNet .
InsightfaceIn this repository, we provide training for deep face recognition. The training data includes the MS1M and VGG2 datasets, which were already packed in the MxNet binary format. The network backbones include ResNet, InceptionResNet_v2, DenseNet, DPN and MobileNet. The loss functions include Softmax, SphereFace, CosineFace, ArcFace and Triplet (Euclidean / Angular) Loss.
Also it needs pre-trained models of
Face Recognition models and
Gender-Age model (for determining gender and age).
')
The rest of the tools:
- laptop (Intel Core i5-7200, NVIDIA GeForce 940MX 2Gb, 8 Gb DDR4 RAM, operating system Manjaro KDE);
- Python 3;
- CUDA Toolkit 10.0;
- mxnet with CUDA support;
- tensorflow with CUDA support;
- opencv;
- pandas;
- downloaded the series “The Big Bang Theory” (265 episodes).
Most additional Python packages are installed with the command:
pip install _
Stage 1. Preparatory
For classification, it is necessary to create a selection of images of persons (different in perspective, season) for each character we are interested in. For this, I took literally the first video from Youtube that I got at the request “funny moments of the big bang theory”. With the help of opencv, I extracted the images from the video at 1 second intervals, manually selected them and renamed them using the script for the characters.
Further, with the help of a neural network and the use of a pre-trained model, I walked through all the selected images. From each detected face, a 512-dimensional vector representation is extracted that characterizes it. This view and character name is stored in pandas.dataframe.
Code for filename in glob.glob('knownimage/*.jpg'): img = cv2.imread(filename) ret = detector.detect_face(img, det_type=0) if ret is not None: bbbox, points = ret
For the 24 main and minor characters of the series, 382 files were selected.
Character list- Howard Joel Wolowitz ( govard , here and hereinafter in brackets indicates the name of the character in the face recognition system) - aerospace systems engineer, husband Bernadette Rostenkovski-Wolowitz, father Halley Wolowitz, best friend Rajesh Kutrappali. He graduated from the Massachusetts Institute of Technology.
- Dr. Rajesh Ramayan Kutrappali ( rajesh ) is an astrophysicist from India, the best friend of Howard Wolowitz.
- Priya Kutrappali ( priya ) is the younger sister of Raja, the daughter of a doctor and Mrs. Kutrappali.
- Penny Hofstedter ( penny ) - Leonard Hofstedter’s wife, a sales representative for a pharmaceutical company.
- Dr. Bernadette Maryann Rostenkovsky-Wolowitz ( bernaded ) is a microbiologist, Howard's wife Wolowitz, mother of Halley Wolowitz and Michael Wolowitz.
- Dr. Beverly Hofstedter ( mama_leonarda ) - Leonard Hofstedter's mother, psychiatrist and neurobiologist
- Leonard Leakey Hofstedter ( leonard ) - experimental physicist, employee of the California Institute of Technology, holder of a doctoral degree.
- Dr. Leslie Winkle ( lesly ) is a University of California employee who works with Leonard Hofstedter.
- Dr. Sheldon Lee Cooper ( sheldon ) is a theoretical physicist working at the California Institute of Technology. He has several degrees, including a doctoral degree.
- Melissa "Missy" Cooper ( sestra_sheldona ) is Sheldon Cooper's twin sister.
- Mary Cooper ( mama_sheldona ) is Sheldon Cooper's mother.
- Dr. Amy Farrah Fowler ( emmy ) is a neuroscientist, Sheldon Cooper's wife.
- Wil Wheaton ( will ) - Star Trek: New Generation actor, whom Sheldon considered his worst enemy.
- Stuart David Blum ( stuart ) - the owner of a comic book store, after a fire became unemployed and moved to live with Howard Volovitsa.
- Dr. V. Kutrappali ( papa_rajesh ) - the father of Rajesh Kutrappali.
- Barry Kripke ( barry ) - a colleague of the main characters in the university.
- Ramona Nowitzki ( navitsky ) - graduate student of the Department of Physics.
- Dr. Geiblhauser ( geibelhauser ) - the new head of the Faculty of Physics.
- Zack ( bivshiy_penny ) - Penny's former boyfriend.
- Mrs. Kutrappali ( mama_rajesh ) is the mother of Rajesh Kutrappali.
- Dr. Stephanie Barnett ( medik_leonarda ) is one of Leonard's girls.
Stage 2. Video processing
Now we begin to process the entire series. With the help of opencv, we extract 1 frame per second from each video and find faces on it. For these persons, we obtain a 512-dimensional vector representation, which characterizes it and compares it with the base that we obtained in the previous step. We also determine the estimated age and gender.
For each found and classified person in the csv file we save the name, gender, age, frame number, series, season. Detected but not classified persons are saved in a separate directory.
Processing of all series took ~ 30 hours, 263775 frames were extracted, 353031 characters were classified. Processing of one frame takes from 0.2 to 1 second depending on the number of faces. In the folder with unclassified persons - ~ 20,000 persons (ie, 6%). This is mostly extras, rare characters or bad angle of the main characters of the series.
Examples of unclassified face images Piece of code home_video = '/run/media/home/DATA/torrent/The.Big.Bang.Theory/' all_series = (len(glob.glob(home_video+'*.avi'))) now_series = 0 for filename1 in glob.glob(home_video+'*.avi'): now_series = now_series + 1 print(filename1) cam = cv2.VideoCapture(filename1) fps = cam.get(cv2.CAP_PROP_FPS)
Stage 3. A little analysis
In the beginning I wanted to use Pandas to analyze the data obtained, but, unfortunately, I did not master it to the necessary extent. On the Internet, the
Falcon SQL Client program was found, into which all the data was downloaded. Also note that the 12 season is not removed until the end.
Falcon SQL ClientFalcon is a free, open-source SQL editor with inline data visualization. It currently supports connecting to RedShift, MySQL, PostgreSQL, IBM DB2, Impala, MS SQL, Oracle, SQLite and more.

The figure below shows a graph of the number of frames with the main and secondary characters in the seasons. Here it is clearly seen that the share of Sheldon and Leonard is decreasing, but the role of Bernadette and Amy is growing.

Here, the main characters of the series are taken out separately.

The main characters of the series as a percentage. The clear leader is Sheldon Cooper.

I suggested that the appearance of a “pair” in the series should correlate with the joint appearance in the frame, i.e. the closer the relationship, the more often they appear together in the same frame. A schedule was built for the “couples”: Leonard - Penny, Leonard - Sheldon, Sheldon - Amy, Howard - Rajesh, Howard - Bernadette.

The graph clearly shows the dependencies. Let's consider separately the "couples" Leonard - Penny and Leonard - Sheldon.

We see that as Leonard and Penny evolve, Sheldon more and more remains in the “span”. It is also known that Penny and Leonard begin to meet in season 3, which can also be seen on the chart.
Consider separately Sheldon-Amy and Sheldon-Leonard.

The peak of the development of Sheldon-Amy relations falls on season 10, which we see on the graph.
The following pair of "pairs", which we consider separately - Howard-Bernadette and Howard-Rajesh.
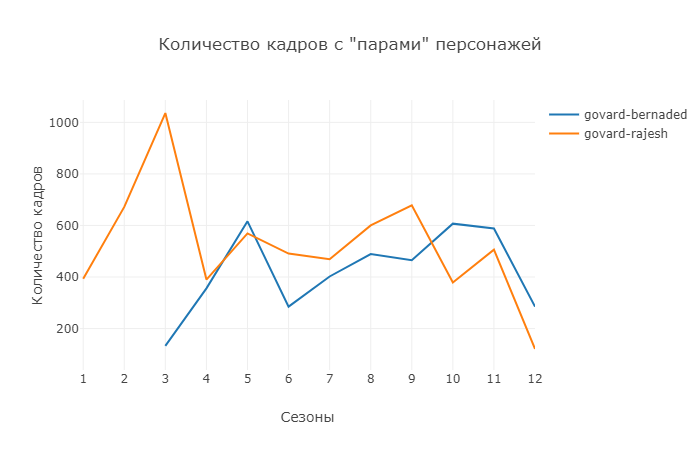
Judging by this schedule, “strong male friendship” no one will destroy and Rajesh continues his communication with Howard. We also note that the peak of the Howard-Bernadette relationship falls on season 5 (this season they have preparation and the wedding itself).
Now let's check how the pre-trained model for determining age and sex works. Let's start with the age: I took for each main character the average age in the episode and put it in a separate table (the actual age of the hero at the time of the shooting of the season is shown in brackets).

It can be seen that the make-up, the right light and the post-processing does its job and the neural network does not do well with the determination of age. However, she discovers an increase in age with each subsequent season.
Let's look at the definition of gender for the main characters (closer to 1 - male, to 0 - female).
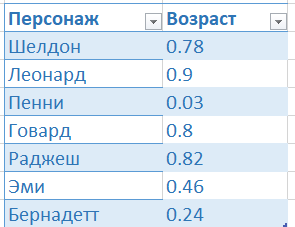
Here the neural network does much better and shows results close to real ones. Only with Amy, the result is a bit suspicious.
It seemed to me interesting to use a neural network to detect and classify individuals. It can be seen that it does not provide 100% accuracy, but this should not be. In the future, I think to simplify the stage of creating the initial sample, using the search by image on the Internet and using this project in video surveillance.
Thanks for attention.
List of materials:
- MTCNN face detection implementation for TensorFlow, as a PIP package.
- Face Recognition Project on MXNet
- The big bang theory
- List of characters of the series
- Falcon SQL Client
- Summary CSV file