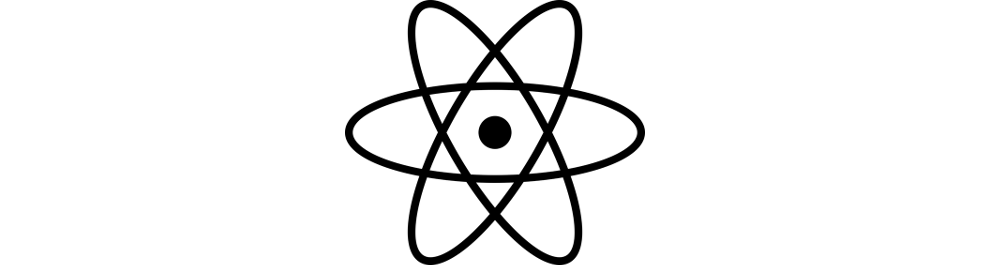
From the first lines of code, each person begins to understand the importance of properly organizing it and optimizing the workspace as a whole.
It does not matter which industry to talk specifically, but it’s important to understand that wherever there is code, there must be rules for its creation and storage.
In the first couple, of course, it may seem that sticking to certain rules and orders only takes time, which in practice looks quite different. The quintessence of any code-writing principles is that we do not write it once and for all - we constantly return to it for the purpose of editing and modifying.
')
This process can turn into a real problem if chaos and unpredictability prevails there. This problem can be aggravated if this chaos was created not by you, but by a person with whom it is not possible to establish contact. It is to eliminate such situations and there are methodologies.
If we talk about css, then with confidence we can say - each of us uses a certain methodology, consciously or not. Even if a person does not use certain rules and standards, he still has his habits and constantly repeated techniques.
Ways of writing code do not have to be generally accepted and standardized, there must be something else that is obligatory - they must be predictable.
The list of methodologies that are worth paying attention to is not so great:
-BEM,
-Smacss,
-OOCSS,
-MCSS,
-Atomic CSS
Atomic CSS is perhaps the most unusual and even to some extent frightening methodology, which fortunately does not prevent it from being very clear and predictable.
To justify my choice, I must roll back a little.
Almost Atomic CSS
There were times when the root directory of the overwhelming number of projects at the stage of creating the interface looked like three files and two folders:
>fonts >img -index.html -style.css -script.js
But this simplicity may be deceptively convenient, in fact, as in at least two of these three files, something terrible was happening.
I was always annoyed by two things - confusion and lack of style. In Css, a lot of different nuances fall under the classification of disorder, but most of all I was not satisfied with the constant repetition of the same rules and properties for different selectors.
The first solution to this problem was the following:
- creating a file called “re-use.css”,
- creating instructions in this file that, in theory, may be needed by more than one selector,
- adding different selectors to the same instruction.
It looked like this:
... .class { display: inline-block;} .class { text-transform: uppercase;} ...
and subsequently took the following:
... .menu-link, .primary-button, .form-button, .footer-link, .social-link { display: inline-block;} ...
Of course, this solution was not very convenient and fortunately temporary. Without long suffering with endless switching between files, and searching for the right place in them, I came to the conclusion that it would be much more convenient to create separate classes that are responsible for one instruction each:
...
.inline-block { display: inline-block;} .block {display: block;} .uppercase {text-transform: uppercase;}
In html files, tags with a large number of classes looked odd, but this solution seemed to me quite acceptable:
<aside class=”sidebar fixed left top w-30 h-100 main-fill”></aside>
At one glance, it is enough to understand that this is a side column, with fixed positioning, on the left side of the screen, occupying 30% of its width and 100% of its height, filled with the main color.
Everything related to numerical values, mostly external and internal indents, I wrote in a standard format. To do this, I added a separate class going first to each tag, or group of tags; in the example above, this is the “slider” class.
It was almost an atomic and almost convenient approach. Several disadvantages prevented him from becoming comfortable, the most important of which were the following situations:
- when creating a large number of similar tags, for example, list items, I had to copy a lot of classes and it looked cumbersome,
- when making any changes, it was necessary to delete and append classes for each element separately,
- there were a lot of unused classes that were difficult to catch.
And then two things came to the rescue that helped solve all the problems - this is a preprocessor and a template engine.
With their help, I modified my methodology and made the layout pleasant and convenient.
Almost perfect Css
I'll start with the preprocessor. His choice is not fundamental, I initially used Sass, then SCSS and eventually switched to Stylus, because I respect minimalism, and Stylus was as minimal as possible (in the examples below, scss will be used because of its popularity).
So, the first thing I did was write additional classes that looked like real atoms with the @extend directive:
.flex { display: flex; flex-wrap: no-wrap; } .flex-jc-center { @extend .flex; justify-content: center; } .flex-ai-center { @extend .flex; align-items: center; }
I liked the idea, and the @extend directive caused a similarity with the atomic nucleus, next to which there were additional instructions.
I decided that the idea should be developed and created a separate file for organisms. Organisms I called classes that include several @extend directives:
.header { @extend .fixed; @extend .w-100; @extend .main-fill; @extend .left; @extend .top; @extend .flex-ai-center; @extend .z-top; }
Having created a small “zoo” from various organisms, I decided to do something with classes that require numerical values ​​for indents and sizes.
The preprocessor capabilities helped me cope with this again, with the help of which I wrote some functions and added variables.
First, I studied in detail the graphic projects that I get from designers and revealed a number of regularities:
- number of colors for each project,
- number of fonts
- number of different sizes for text and headings,
- repeated indents (for sections, buttons, etc.)
The first step was to write functions and mixins to create the necessary classes:
// px em @function em($pixels, $context: $browser-context) { @return #{$pixels/$context}em };
// $text-size: ( l: 18, m: 16, s: 14, xs: 12 );
@mixin emFonts($list, $n) { // $list – , n – . @each $status, $size in $list { // - &-#{$status} { font-size: em($size - $n); // } } }
Now we can call this combination of mixin and function in any place convenient for us:
.txt { @include emFonts($text-size, 0) }
And at the output we get 4 classes for text of different sizes:
.txt-m { font-size: 1.125em; } .txt-s { font-size: 1em; }
Similarly, functions are created and called for the sizes of headings, text colors and fills, fonts, and so on.
That is, work on each project begins with filling in the values ​​for variables, while classes and functions themselves migrate from one project to another.
Template engine.
I think many of you use template engines, and most likely this is a Pug (which used to be called Jade).
For atomic layout it is needed thanks to 3 things:
Pug completely relieves us of cumbersome HTML code from microclasses, since we can turn the following code:
… <ul class=”menu__list flex-ai-center w-100 relative “> <li class=”menu__item m-color m-font txt-s inline-block bold-border”>first</li> <li class=”menu__item m-color m-font txt-s inline-block bold-border”>second</li> <li class=”menu__item m-color m-font txt-s inline-block bold-border”>third</li> </ul>
Easy to edit:
-let menuItemClasses = 'menu__item m-color m-font txt-s inline-block bold-border' // ul li(class=`${menuItemCLasses}`) frst li(class=`${menuItemCLasses}`) second li(class=`${menuItemCLasses}`) third ... </ul>
or alternatively, using a loop:
let menuItems = ['first', 'second', 'third'] ul -for(let item of menuItems) { li(class=”menu__item m-color m-font txt-s inline-block bold-border”) -}
This is no less convenient, because the line with the necessary classes is not repeated more than once.
The last weak point of this methodology was that a large number of unused classes remained in the code. But this seemingly serious problem is easily resolved by adding plugins to the project builder that remove unnecessary classes.
Conclusion
In conclusion, I would like to say the following:
Before starting to use “almost atomic methodology”, I used smacss for a long time and then BEM. As a result, I left from bem only names for classes that require descriptions of indents and sizes, and the hierarchy of files and folders. A set of ready-made classes and functions, I connect to the project as a library.
An important point that I would like to note is the convenience of the layout of the villages and its individual sections entirely. Thanks to microclasses, it is fairly easy to create a “skeleton” of a page or section in a templating engine and only then proceed to writing styles for it.