Blazor 0.7.0 is now available! This update focuses on ADRs (ancestor-descendent relationships). In addition, we have added some improvements to the debugging process. More under the cut!
A little bit about Blazor: a browser application framework written in .NET and launched using WebAssembly. It gives you all the advantages of modern single-page applications (SPA), while allowing you to use .NET from beginning to end, up to the common code on the server and client. [
1 ]
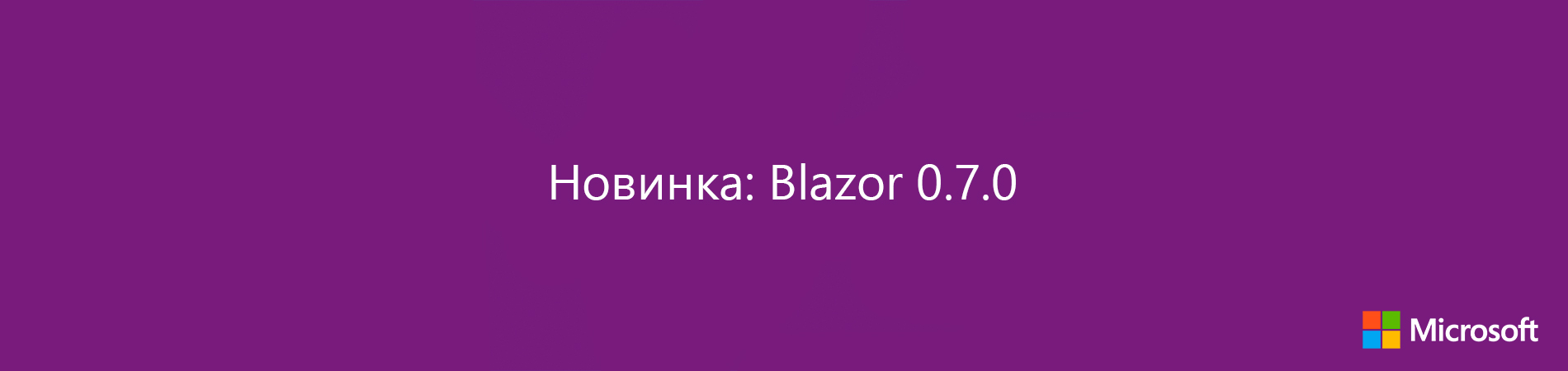
')
A small introduction or "What is Blazor?" .
Here's what's new in the Blazor 0.7.0 version:
- Cascading Values and Parameters
- Debug enhancements
A full list of changes in this version can be found in the
Blazor 0.7.0 release notes .
Get Blazor 0.7.0
Install the following:
- .NET Core 2.1 SDK (2.1.500 or later).
- Visual Studio 2017 (15.9 or later) with ASP.NET.
- The latest Blazor Language Services extension from the Visual Studio Marketplace.
- Command line blazor templates:
dotnet new -i Microsoft.AspNetCore.Blazor.Templates
You can find instructions, documents and manuals for Blazor on
blazor.net .
Upgrade an existing project to Blazor 0.7.0
To upgrade your Blazor 0.6.0 project to 0.7.0:
- Set the prerequisites listed above.
- Upgrade Blazor packages and .NET CLI tool links to 0.7.0. The updated Blazor project file should look like this:
<Project Sdk="Microsoft.NET.Sdk.Web"> <PropertyGroup> <TargetFramework>netstandard2.0</TargetFramework> <RunCommand>dotnet</RunCommand> <RunArguments>blazor serve</RunArguments> <LangVersion>7.3</LangVersion> </PropertyGroup> <ItemGroup> <PackageReference Include="Microsoft.AspNetCore.Blazor.Browser" Version="0.7.0" /> <PackageReference Include="Microsoft.AspNetCore.Blazor.Build" Version="0.7.0" /> <DotNetCliToolReference Include="Microsoft.AspNetCore.Blazor.Cli" Version="0.7.0" /> </ItemGroup> </Project>
This is it! Now you can appreciate the latest Blazor features.
Cascading Values and Parameters
Blazor components can take parameters that can be used to transfer data to a component and affect the rendering of the component. Parameter values are provided from the parent component to the child component. Sometimes, however, it is inconvenient to transfer data from the ancestor component to the descendant component, especially when there are many layers between them. Cascading values and parameters solve this problem by providing a convenient way for the parent component to provide a value that is then available to all descendant components. They also provide a great way to coordinate components.
For example, if you want to provide some information about a topic for a specific part of your application, you can pass the appropriate styles and classes from component to component, but that would be tedious and time consuming. Instead, the common parent component can provide information about the topic as a cascading value, which the children can take as a cascading parameter, and then use it as necessary.
Suppose that the
ThemeInfo
class passes all the information about a topic that you want to pass through the component hierarchy so that all the buttons in this part of your application have the same appearance:
public class ThemeInfo { public string ButtonClass { get; set; } }
The parent component can provide a cascading value using the
CascadingValue
component. The
CascadingValue
component wraps the subtree of the component hierarchy and sets a single value that will be available to all components inside this subtree. For example, we could specify theme information as a cascading parameter for all components that make up the body of the layout:
@inherits BlazorLayoutComponent <div class="sidebar"> <NavMenu /> </div> <div class="main"> <div class="top-row px-4"> <a href="http://blazor.net" target="_blank" class="ml-md-auto">About</a> </div> <CascadingValue Value="@theme"> <div class="content px-4"> @Body </div> </CascadingValue> </div> @functions { ThemeInfo theme = new ThemeInfo { ButtonClass = "btn-success" }; }
To use cascading values, components can declare cascading parameters using the [CascadingParameter] attribute. Cascading values are tied to cascading parameters by type. In the following example, the Counter component is modified to have a cascading parameter that binds to the cascade value of ThemeInfo, which is then used to set the class for the button.
@page "/counter" <h1>Counter</h1> <p>Current count: @currentCount</p> <button class="btn @ThemeInfo.ButtonClass" onclick="@IncrementCount">Click me</button> @functions { int currentCount = 0; [CascadingParameter] protected ThemeInfo ThemeInfo { get; set; } void IncrementCount() { currentCount++; } }
When we launch the application, we see that the new style is applied:
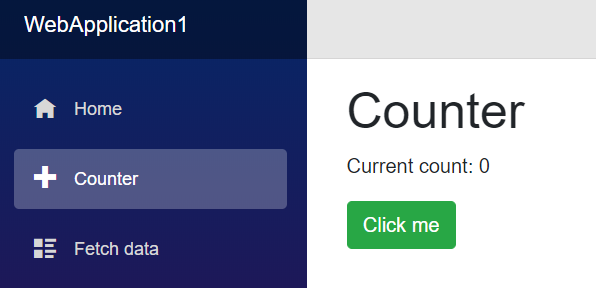
Cascading parameters also allow components to interact across a component hierarchy. For example, suppose you have a TabSet component that contains several Tab components:
<TabSet> <Tab Title="First tab"> <h4>First tab</h4> This is the first tab. </Tab> @if (showSecondTab) { <Tab Title="Second"> <h4>Second tab</h4> You can toggle me. </Tab> } <Tab Title="Third"> <h4>Third tab</h4> <label> <input type="checkbox" bind=@showSecondTab /> Toggle second tab </label> </Tab> </TabSet>
In this example, the child components Tab are not explicitly passed as parameters to the TabSet. Instead, they are simply part of the child contents of the TabSet. But TabSet still needs to know about each tab so that it can display headers and active tabs. To enable this coordination, without requiring any specific communication with the user, the TabSet component can present itself as a cascading value, which can then be picked up by the components of the Tab component:
In TabSet.cshtml:
<!-- Display the tab headers --> <CascadingValue Value=this> <ul class="nav nav-tabs"> @ChildContent </ul> </CascadingValue>
This allows the parent Tab components to capture the containing TabSet as a cascading parameter, so they can add themselves to the TabSet and coordinate on which Tab is active:
In Tab.cshtml:
[CascadingParameter] TabSet ContainerTabSet { get; set; }
See the complete TabSet sample
here .
Debug enhancements
In Blazor 0.5.0, we added support for
debugging Blazor client applications in the browser . Although this experiment demonstrated that debugging .NET applications in a browser is possible, it was a rather dangerous experience. Now you can more reliably install and remove breakpoints, and the reliability of step-by-step debugging has been improved.
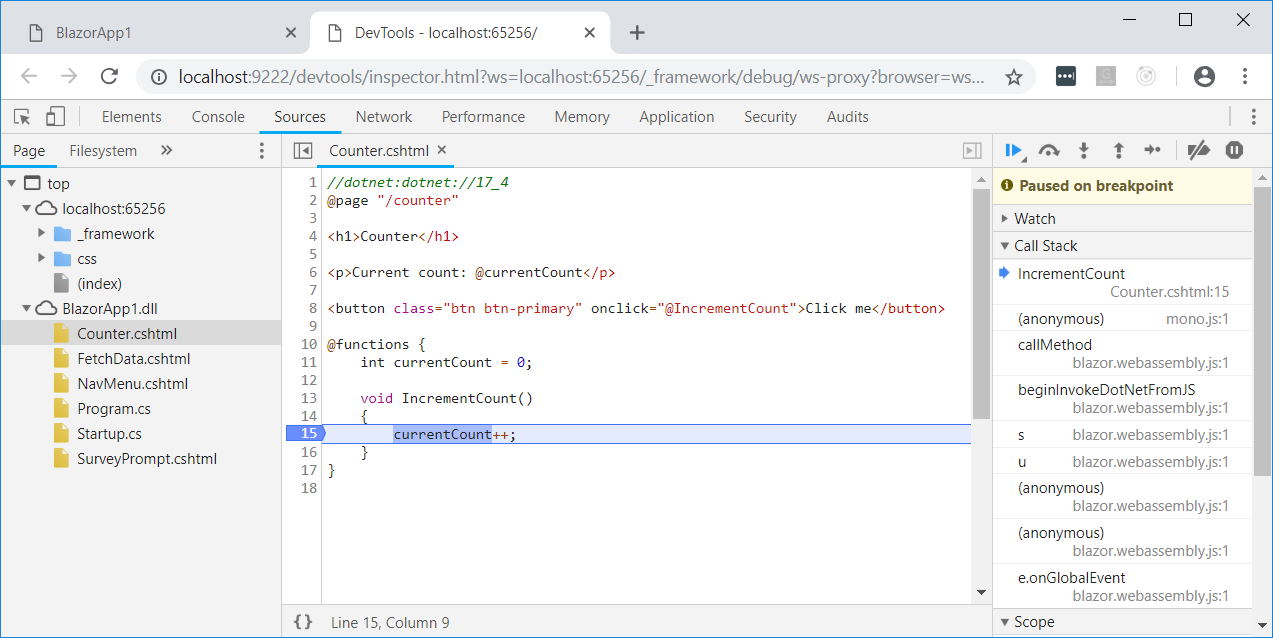