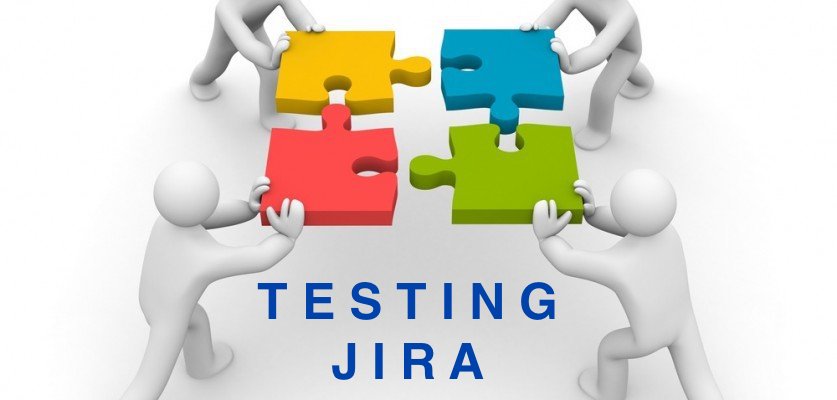
Let's talk about how to do integration tests for Atlassian Jira Server. For these purposes, we will use the libraries that Atlassian provides: jira-func-test-plugin and jira-testkit-plugin.
Integration tests allow you to test the operation of Jira plugins through the services exported by the plugin and the Jira user interface. Of course, you can simply take Selenium WebDriver and write code to test the Jira user interface. However, in this case it is necessary to prescribe the entire logic. For example, logging in a user, entering a JQL request, creating a project, checking elements in the form of creating a task, managing project permissions and so on. Atlassian libraries provide methods that are already ready for this. Moreover, your code does not need to be changed when upgrading to a new version of Jira, just take the Atlassian libraries that are suitable for this version.
There are three ways to write integration tests for Atlassian Jira, which Atlassian provides:
')
- Wired tests allow you to test services that your plugin exports to the outside. An example of such a test is here .
- Integration tests require the jira-func-test-plugin and jira-testkit-plugin plugins to run. These tests verify the correctness of the Jira user interface using HTTP requests and responses. An example of such a test is here .
- Page objects-tests also check the Jira user interface, but they work through Selenium WebDriver.
Atlassian writes that testing the user interface through Page objects tests is preferable to testing through the jira-func-test-plugin. But in this article we will learn how to write integration tests using this plugin.
The source code of the plug-in developed in the article with integration tests can be found
here .
What are integration tests
The main difference between integration tests and modular tests is that modular tests do not require a working Jira instance, unlike integration tests. Therefore, it is necessary that during the integration test a Jira instance is raised. Testing can take place both on a local instance (Jira, lifted from our plugin with integration tests), and on a remote one.
Let's first write tests for a local instance, and then I will tell you how to run these tests on a remote instance.
Create a Jira plugin
A plugin is created using the
atlas-create-jira-plugin command .
Add dependencies to our plugin
The following dependencies must be added to our plugin's pom.xml file:
<dependency> <groupId>com.atlassian.jira.tests</groupId> <artifactId>jira-testkit-client</artifactId> <version>${jira.version}</version> <scope>test</scope> </dependency> <dependency> <groupId>com.atlassian.jira</groupId> <artifactId>jira-func-tests</artifactId> <version>${jira.version}</version> <scope>test</scope> </dependency>
These dependencies will allow us to develop integration tests.
Install the necessary plugins to the local Jira instance
For the integration tests to work, we need to make sure that when we start our local Jira instance, the jira-func-test-plugin and jira-testkit-plugin plug-ins are installed on it.
To do this, add the following lines to the maven-jira-plugin plugin configuration in pom.xml:
<pluginArtifacts> <pluginArtifact> <groupId>com.atlassian.jira</groupId> <artifactId>jira-func-test-plugin</artifactId> <version>${jira.version}</version> </pluginArtifact> <pluginArtifact> <groupId>com.atlassian.jira.tests</groupId> <artifactId>jira-testkit-plugin</artifactId> <version>7.12.3</version> </pluginArtifact> </pluginArtifacts>
We write a test
Create the file src / test / java / it / en / matveev / alexey / jira / integrationtests / selenium / ExampleTest.java:
package it.ru.matveev.alexey.jira.integrationtests.selenium; import com.atlassian.jira.functest.framework.BaseJiraFuncTest; import org.junit.After; import org.junit.Before; import org.junit.Test; public class ExampleTest extends BaseJiraFuncTest { @Before public void setup() { backdoor.project().addProject("testproject", "TP", "admin"); backdoor.issues().createIssue("TP", "test issue"); } @Test public void goToIssueNavigator() { navigation.login("admin"); navigation.issueNavigator().createSearch("project = TP"); tester.assertTextPresent("TP-1"); } @After public void cleanup() { backdoor.project().deleteProject("TP"); }
By default, all tests must have a
Test
suffix for the integration test files to run. This can be overridden using the
functionalTestPattern
parameter in the maven-jira-plugin plugin configuration.
In the above test, we test the job search by request in the Issue Navigator. In the
setup
method, we create a project with a task, then we write a request that receives this task, and check that we could actually select it. Then in the
cleanup
method we delete the created project.
Run the test
The test is started using the
atlas-integration-test
command. At this command, the local Jira is raised and integration tests are performed on it.
Test structure
There are several classes from which you can inherit when writing integration tests:
BaseJiraEmailTest
,
BaseJiraFuncTest
,
BaseJiraRestTest
.
The most used class is
BaseJiraFuncTest
. It allows you to test all the necessary functionality.
In this class there are such class variables:
backdoor
: allows you to perform administrative functions, such as managing projects, indexes, project authorization schemes, users, and so on.navigation
: allows you to navigate in the user interface. Also allows you to log in and out of Jira as desired.tester
: allows you to work with pages: set field values, click on buttons, check if a field exists in a form, and so on.
The integration tests are addressed to Jira as the
admin
user with the password
admin
, so for the integration tests to work in Jira there must be this user with such a password.
When using the
navigation.login("username")
command, the user's password under which you log in to Jira must match the username.
Running integration tests on a remote instance
In order for integration tests to run on a remote instance, the plug-ins jira-func-test-plugin and jira-testkit-plugin must be installed on it.
In addition, in the integration test plugin, you need to make changes to the src / test / resources / localtest.properties file:
jira.protocol = http jira.host = remote-instance jira.port = 2991 jira.context = /jira jira.edition = all jira.xml.data.location = src/test/xml
Now, after running the
atlas-integration-test
command, the tests will be run on the remote instance.
Test examples
The good news is that the developers of the jira-func-tests package have included a large number of examples with tests for all occasions in this package. They are in the webtests package.
Good luck with your Jira testing!