TypeScript is based on the same syntax and semantics that are familiar to millions of JavaScript developers. TypeScript gives you the opportunity to work with the latest and most emerging JS features, including those that are in ECMAScript 2015, and those that still exist only in the form of sentences. Among such possibilities, for example, asynchronous functions and decorators. All this is aimed at helping the developer to create reliable and modern applications.
A typeScript program is compiled into regular JavaScript code that can be executed in any browser or in Node.js environment. This code will be clear to any JS engine that supports ECMAScript 3 standard or newer.
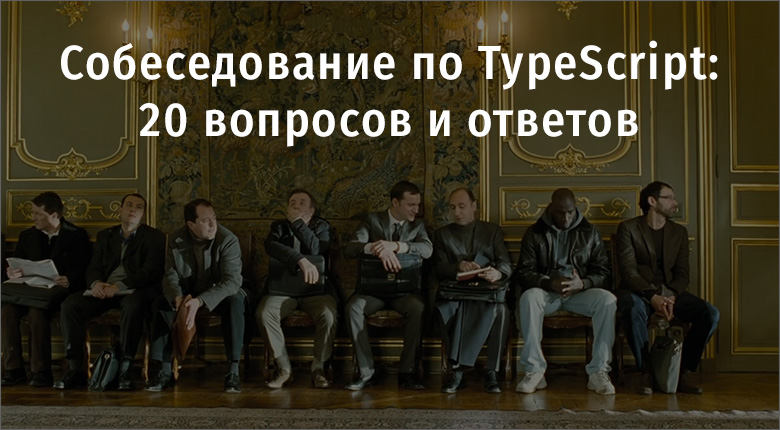
')
The material, which we are publishing today, contains an analysis of twenty questions that may well be asked to those who are going to have an interview, claiming the position of a TypeScript programmer.
Question number 1 (1). What is TypeScript and why use it instead of javascript?
In brackets, after the number of the question, its complexity is indicated, measured on a five-point scale.
TypeScript (TS) is a superset of JavaScript (JS), the main features of which include the possibility of explicit static type assignment, support for classes and interfaces. One of the major advantages of TS to JS is the ability to create, in various IDEs, such a development environment, which allows, in the process of entering the code, to detect common errors. The use of TypeScript in large projects can lead to an increase in the reliability of programs that, in doing so, can be deployed in the same environments where regular JS applications are running.
Here are some details about TypeScript:
- TypeScript supports modern editions of ECMAScript standards, code written with the use of which is compiled taking into account the possibility of its implementation on platforms that support older versions of standards. This means that a TS programmer can use the capabilities of ES2015 and newer standards, like modules, switch functions, classes, the spread operator, destructuring, and perform what he does in existing environments that do not yet support these standards.
- TypeScript is an add-on for JavaScript. Code written in pure JavaScript is a valid typeScript code.
- TypeScript extends JavaScript with the ability to statically assign types. The TS type system is quite extensive. Namely, it includes interfaces, enumerations, hybrid types, generic types (generics), union types and intersection types, access modifiers and much more. The use of TypeScript, in addition, slightly simplifies the work by using the type inference.
- Using TypeScript, in comparison with JavaScript, greatly improves the development process. The fact is that the IDE, in real time, gets information about types from the TS compiler.
- When using strict
null
checking mode (the compiler flag --strictNullChecks
is used for this), the TypeScript compiler does not allow assignment of null
and undefined
variables of types in which, in this mode, these values ββare not allowed. - To use TypeScript, you need to organize the project build process, which includes the step of compiling TS code in JavaScript. The compiler can embed a source map in the JS files generated by it, or create separate .map files. This allows you to set breakpoints and examine the values ββof variables at run time, working directly with the TypeScript code.
- TypeScript is an open source Microsoft project released under the Apache 2 license. The TypeScript development is initiated by Anders Hejlsberg. He is involved in creating Turbo Pascal, Delphi, and C #.
β
SourceQuestion number 2 (1). Tell us about generic types in TypeScript.
Generic types (generics) allow you to create components or functions that can work with different types, and not with any one. Consider an example:
class Queue<t> { private data = []; push = (item: T) => this.data.push(item); pop = (): T => this.data.shift(); } const queue = new Queue<number>(); queue.push(0); queue.push("1");
β
SourceQuestion number 3 (2). Does TypeScript support all the principles of object-oriented programming?
Yes, it does. There are four basic principles of object-oriented programming:
- Encapsulation
- Inheritance
- Abstraction
- Polymorphism
Using simple and clear TypeScript tools, you can implement all these principles.
β
SourceQuestion number 4 (2). How in TypeScript to check values ββon equality of null and undefined?
To perform such checks, it is sufficient to use the following construction:
if (value) { }
The expression in brackets will be returned to
true
if it is not something from the following list:
null
undefined
NaN
- Empty line
- 0
false
TypeScript supports the same type conversion rules as JavaScript.
β
SourceQuestion number 5 (2). How to implement TypeScript properties that are constants?
In TypeScript, when declaring class properties, you cannot use the
const
keyword. When you try to use this keyword, the following error message is displayed:
A class member cannot have the 'const' keyword
. In TypeScript 2.0, there is a
readonly
modifier that allows you to create read-only class properties:
class MyClass { readonly myReadonlyProperty = 1; myMethod() { console.log(this.myReadonlyProperty); } } new MyClass().myReadonlyProperty = 5;
β
SourceQuestion number 6 (2). What are .map files in TypeScript?
Files with the .map extension store source maps, which contain information about the correspondence of code written in TypeScript to the JavaScript code created on its basis. Many debuggers can work with these files (for example, Visual Studio and Chrome developer tools). This allows, during debugging, to work with the source code of TypeScript programs, and not with their JS equivalents.
β
SourceQuestion number 7 (2). What are getters and setters in TypeScript?
TypeScript supports getters and setters that allow you to control access to members of objects. They give the developer a means to control reading and writing the properties of objects.
class foo { private _bar:boolean = false; get bar():boolean { return this._bar; } set bar(theBar:boolean) { this._bar = theBar; } } var myBar = myFoo.bar;
β
SourceQuestion number 8 (2). Can I use TypeScript in server development, and if so, how?
Programs written in TypeScript are suitable not only for front-end development, but also for creating server applications. For example, on TS you can write programs for the Node.js platform. This gives the programmer additional tools for type control and allows you to use other language features. To create server applications on TS, all that is needed is to establish the correct code processing process, to the input of which TypeScript files arrive, and the output will be JavaScript files suitable for execution in Node.js. In order to organize such an environment, you must first install a TypeScript compiler:
npm i -g typescript
Compiler parameters are set using the
tsconfig.json
file, which defines, among other things, the purpose of the compilation and the place in which the finished JS files need to fit. In general, this file is very similar to the babel or webpack configuration files:
{ "compilerOptions": { "target": "es5", "module": "commonjs", "declaration": true, "outDir": "build" } }
Now, provided that the compiler has something to handle, you need to run it:
tsc
And, finally, given that JS files suitable for execution in the Node.js environment are located in the
build
folder, you need to run this command from the root directory of the project:
node build/index.js
β Source
Question number 9 (3). Tell us about the main components of TypeScript.
TypeScript includes three main components:
- Tongue. This, from the developers' point of view, is the most important part of TypeScript. βLanguageβ is syntax, keywords, everything that allows you to write programs in TypeScript.
- Compiler. TypeScript has an open source compiler, is cross-platform, open specification, and is written in TypeScript. The compiler converts TypeScript code to JavaScript code. In addition, if something is wrong with the program, it gives error messages. It allows you to combine several TypeScript files into one output JS file and can create code maps.
- Auxiliary tools. TypeScript helper tools are designed to facilitate the development process using it in various IDEs. Among them - Visual Studio, VS Code , Sublime, various tools for quick launch of TS-code, and others.
β
SourceQuestion number 10 (3). Does the TypeScript code provided to you have any errors? Explain your answer.
Here is the code snippet:
class Point { x: number; y: number; } interface Point3d extends Point { z: number; } let point3d: Point3d = {x: 1, y: 2, z: 3};
There are no errors in this code. A class declaration creates two entities: this is the data type used to create instances of the class, and a constructor function. Since classes create data types, you can use them in the same place where you can use interfaces.
β
Source
Just love this movie very much)Question number 11 (3). Tell about the use of property decorators in TypeScript.
Decorators can be used to change the behavior of classes, with even more benefit from them can be obtained when using them with any framework. For example, if your framework has methods that are restricted to access (say, they are intended only for the administrator), it will be easy to write the
@admin
method
@admin
, which will deny access to the corresponding methods for non-administrator users. You can create an
@owner
decorator that allows you to modify an object only to its owner. Here is what decorator use might look like:
class CRUD { get() { } post() { } @admin delete() { } @owner put() { } }
β
SourceQuestion number 12 (3). Is it possible to use strongly typed functions as parameters in TypeScript?
Consider the following example:
class Foo { save(callback: Function) : void { // var result : number = 42; // // - , number? callback(result); } } var foo = new Foo(); var callback = (result: string) : void => { alert(result); } foo.save(callback);
Is it possible in the
save
method to organize work with a typed callback? Rewrite the code to demonstrate this.
In TypeScript, you can declare a callback type, and then rewrite the code:
type NumberCallback = (n: number) => any; class Foo { // save(callback: NumberCallback): void { console.log(1) callback(42); } } var numCallback: NumberCallback = (result: number) : void => { console.log("numCallback: ", result.toString()); } var foo = new Foo(); foo.save(numCallback)
β
SourceQuestion number 13 (3). How to make classes declared in a module be available outside this module?
Classes declared in a module are available within this module. Outside it, access to them is impossible.
module Vehicle { class Car { constructor ( public make: string, public model: string) { } } var audiCar = new Car("Audi", "Q7"); }
In the code above, an error will occur when attempting to initialize the
fordCar
variable. To make a class declared in a module available outside of this module, you need to use the
export
keyword:
module Vehicle { export class Car { constructor ( public make: string, public model: string) { } } var audiCar = new Car("Audi", "Q7"); }
β
SourceQuestion number 14 (3). Does TypeScript support function overloading?
TypeScript supports function overloading, but the implementation of this mechanism is different from that seen in other object-oriented languages. Namely, in TS they create only one function and a certain number of declarations. When such code is compiled into JavaScript, only one specific function is visible. This mechanism works because JS functions can be called by passing them a different number of parameters.
class Foo { myMethod(a: string); myMethod(a: number); myMethod(a: number, b: string); myMethod(a: any, b?: string) { alert(a.toString()); } }
β
SourceQuestion number 15 (4). What is wrong with the code provided to you?
Here is the code in question:
interface Fetcher { getObject(done: (data: any, elapsedTime?: number) => void): void; }
It is recommended to use optional parameters in callbacks only if you absolutely understand the consequences of such a step. This code has a very specific meaning: the
done
callback can be called with either 1 or 2 arguments. The author of the code probably intended to tell us that a callback might not pay attention to the
elapsedTime
parameter, but in order to achieve this, you can always create a callback that accepts fewer arguments.
β
SourceQuestion number 16 (4). How in TypeScript to overload the class constructor?
TypeScript allows you to declare many variants of methods, but there can be only one implementation, and this implementation must have a signature that is compatible with all variants of the overloaded methods. To overload the class constructor, you can use several approaches:
- You can use the optional parameter:
class Box { public x: number; public y: number; public height: number; public width: number; constructor(); constructor(obj: IBox); constructor(obj?: any) { this.x = obj && obj.x || 0 this.y = obj && obj.y || 0 this.height = obj && obj.height || 0 this.width = obj && obj.width || 0; } }
- You can use the default parameters:
class Box { public x: number; public y: number; public height: number; public width: number; constructor(obj : IBox = {x:0,y:0, height:0, width:0}) { this.x = obj.x; this.y = obj.y; this.height = obj.height; this.width = obj.width; } }
- You can use additional overloads in the form of static factory methods:
class Person { static fromData(data: PersonData) { let { first, last, birthday, gender = 'M' } = data return new this( `${last}, ${first}`, calculateAge(birthday), gender ) } constructor( public fullName: string, public age: number, public gender: 'M' | 'F' ) {} } interface PersonData { first: string last: string birthday: string gender?: 'M' | 'F' } let personA = new Person('Doe, John', 31, 'M') let personB = Person.fromData({ first: 'John', last: 'Doe', birthday: '10-09-1986' })
- You can use a union type:
class foo { private _name: any; constructor(name: string | number) { this._name = name; } } var f1 = new foo("bar"); var f2 = new foo(1);
β
SourceQuestion number 17 (4). What is the difference between the interface and type keywords in TypeScript?
Here are examples of using these keywords:
interface X { a: number b: string } type X = { a: number b: string };
Unlike an interface declaration, which always represents a named type of an object, using the
type
keyword allows you to specify an alias for any kind of type, including primitive types, union-types, and intersection types.
When using the
type
keyword instead of the
interface
keyword, the following features are lost:
- The interface can be used in an
extends
or implements
expression, and an alias for an object type literal is not. - An interface can have multiple merged ads, and when using the
type
keyword, this feature is not available.
β
SourceQuestion number 18 (5). Tell us about when the declare keyword is used in TypeScript.
The
declare
keyword is used in TypeScript to declare variables that originate from a file that is not a TypeScript file.
For example, imagine that we have a library called
myLibrary
. It does not have a TypeScript type file, it has only the
myLibrary
namespace in the global namespace. If you want to use this library in your TS code, you can use the following construction:
declare var myLibrary;
TypeScript assigns the type
any
to
myLibrary
. The problem here is that you will not have, during development, intelligent tips on this library, although you can use it in your code. In this situation, you can use a different approach, leading to the same result. We are talking about the use of a variable of type
any
:
var myLibrary: any;
In either case, when compiling a TS code in JavaScript, you get the same thing, but the option using the
declare
keyword has better readability. The use of this keyword leads to the creation of a so-called external variable declaration (ambient declaration).
Question number 19 (5). What are external variable declarations in TypeScript and when should they be used?
An external variable declaration (ambient declaration) is a mechanism that lets you tell the TypeScript compiler that some source code exists somewhere outside the current file. External declarations help integrate third-party JavaScript libraries into TS programs.
These declarations make type declarations with the extension .d.ts in the file. External variables or modules are declared as follows:
declare module Module_Name { }
The files in which the external code is located must be included in the TS file using them, like this:
β
SourceQuestion number 20 (5). Can I automatically generate TypeScript ad files from JS libraries?
JavaScript does not always contain enough information that allows TypeScript to automatically infer types. Therefore, it is almost impossible to automatically create JavaScript type-based type declarations. However, you can try to do this using the following tools:
- Microsoft / dts-gen is the official tool used by Microsoft as a starting point when creating type declarations.
- dtsmake is a promising tool for automatic creation of type declarations based on JS files, which is in the process of development. It depends on the Tern code analysis system, which some editors use to implement a completion mechanism when entering JS code.
β
SourceResults
We hope that the analysis of the questions in this material will help you to better know TypeScript, perhaps, to pay attention to what you didnβt pay attention to before, and if you are preparing for an interview, it will increase your chances for its successful completion.
Dear readers! What questions would you ask the interviewer for a position requiring TypeScript knowledge?
- And what a discount on this promo code?
- It seems, 7%. Check if you want ...
- Yeah, you check. In my opinion, the discount is a bit overpriced!
...
- Sorry, I was a little mistaken about the discount. 10% on all virtual servers.