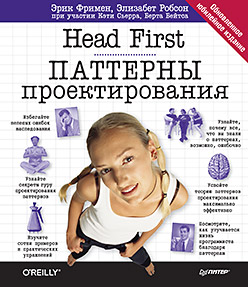
In the world, someone constantly encounters the same programming problems that you have. Many developers solve completely identical tasks and find similar solutions. If you do not want to reinvent the wheel, use ready-made design patterns (patterns) that this book is dedicated to.
Patterns appeared because many developers were looking for ways to increase the flexibility and reuse of their programs. The solutions found are embodied in a concise and easily applicable form in practice.
The peculiarity of this publication is a unique way of presenting the material, highlighting the “Head First” series of O'Reilly publishing house in a series of many boring books on programming. Dalle presents the passage “Simplifying the RemoteControl code with lambda expressions”
As you have seen, the command pattern is fairly straightforward. However, the Java language provides a handy tool that allows you to further simplify the code - namely, lambda expressions. A lambda expression is an abbreviated form of a method record (as a sequence of calculations) at the exact point where it is used. Instead of creating a separate class with a method, creating an instance of this class, and then calling the method, you simply specify: “This is the method you want to call” with the help of a lambda expression. In our case, the execute () method should be called.
')
To understand how this is done, replace the LightOnCommand and LightOffCommand objects.
lambda expressions. Perform the following steps so that the light on / off commands are implemented by lambda expressions instead of command objects:
Step 1. Create a receiverStep 2. Implementing remote control commands using lambda expressionsThis is where all the fun happens. Now, instead of creating LightOnCommand and LightOffCommand objects for passing to remoteControl.setCommand (), we simply pass instead of each object a lambda expression with code from the corresponding execute () method:
Step 3. Activating the buttonsThis step also has not changed - except for the fact that when the onButtonWasPushed (0) method was called, the command in slot 0 is represented by a function object (created using lambda expressions). When we call execute () for a command, this method is mapped to a method defined by a lambda expression, which is then executed.
We use lambda expressions to replace Command objects, and the Command interface contains just one method: execute (). The lambda expression we use must have a compatible signature — it is: the execute () method takes no arguments (like our lambda expression) and does not return values (like our lambda expression). The compiler is happy with everything. The lambda expression is passed in the Command parameter of the setCommand () method:
Remember: if the parameter interface in which the lambda expression is passed contains one (and only one!) Method, and the signature of this method is compatible with the signature of the lambda expression, everything will work as it should.
References to methods
To further simplify the code, you can use the links to the methods. If the passed lambda expression calls just one method, instead of the lambda expression, you can
pass method reference. This is done like this:
Instead of passing a lambda expression that calls the object's on () method
livingRoomLight, we pass the link to the method itself.
But what if a lambda expression has to perform several operations at once?Sometimes lambda expressions used to replace Command objects are not limited to a single operation. Let's see how to replace stereoOnWithCDCommand objects and
stereoOffCommand lambda expressions. Next will be the complete RemoteLoader code, so that you understand how all these ideas fit together.
The stereoOffCommand object executes just one simple command:
stereo.off();
For this command, instead of a lambda expression, you can use the link to the stereo :: off method.
But stereoOnWithCDCommand performs not one, but three operations:
stereo.on(); stereo.setCD(); stereo.setVolume(11);
In this case, the use of the method reference will fail. Instead, you will have to either write the lambda expression in the built-in view, or create it separately, assign a name, and then transfer it to the setCommand () method of the remoteControl object by name. Creation option
A separate lambda expression and assigning it a name looks like this:
Command stereoOnWithCD = () -> { stereo.on(); stereo.setCD(); stereo.setVolume(11); }; remoteControl.setCommand(3, stereoOnWithCD, stereo::off);
Note: Command is used as a lambda expression type. Lambda expression
is mapped to the execute () method of the Command interface and with the Command parameter in which
It is passed to the setCommand () method.
Test drive commands using lambda expressions
To use lambda expressions to simplify source code implementation
RemoteControl (without cancel), we will change the RemoteLoader code and replace specific objects
Command lambda expressions. You also need to change the RemoteControl constructor.
to use lambda expressions instead of a NoCommand object. After that you can
but remove all specific Command classes (LightOnCommand, LightOffCommand,
HottubOnCommand, HottubOffCommand, etc.). That's all! Everything else remains the same
by him. Do not accidentally delete the Command interface; it is necessary for type matching
function objects created by lambda expressions that are stored in the object
remote and transmitted to various methods.
The new code for the RemoteLoader class looks like this:
public class RemoteLoader { public static void main(String[] args) { RemoteControl remoteControl = new RemoteControl(); Light livingRoomLight = new Light("Living Room"); Light kitchenLight = new Light("Kitchen"); CeilingFan ceilingFan = new CeilingFan("Living Room"); GarageDoor garageDoor = new GarageDoor("Main house"); Stereo stereo = new Stereo("Living Room"); Command ( ). ( 22 9). remoteControl.setCommand(0, livingRoomLight::on, livingRoomLight::off); remoteControl.setCommand(1, kitchenLight::on, kitchenLight::off); remoteControl.setCommand(2, ceilingFan::high, ceilingFan::off); Command stereoOnWithCD = () -> { stereo.on(); stereo.setCD(); stereo.setVolume(11); }; remoteControl.setCommand(3, stereoOnWithCD, stereo::off); remoteControl.setCommand(4, garageDoor::up, garageDoor::down); , , - , .( -. ; — -, .) System.out.println(remoteControl); remoteControl.onButtonWasPushed(0); remoteControl.offButtonWasPushed(0); remoteControl.onButtonWasPushed(1); remoteControl.offButtonWasPushed(1); remoteControl.onButtonWasPushed(2); remoteControl.offButtonWasPushed(2); remoteControl.onButtonWasPushed(3); remoteControl.offButtonWasPushed(3);
And do not forget: we must change the RemoteControl constructor, remove the NoCommand object construction code from it, and replace it with lambda expressions.
public class RemoteControl { Command[] onCommands; Command[] offCommands; public RemoteControl() { onCommands = new Command[7]; offCommands = new Command[7]; for (int i = 0; i < 7; i++) { onCommands[i] = () -> { }; offCommands[i] = () -> { }; } }
And now let's check the results of all these commands with lambda expressions ...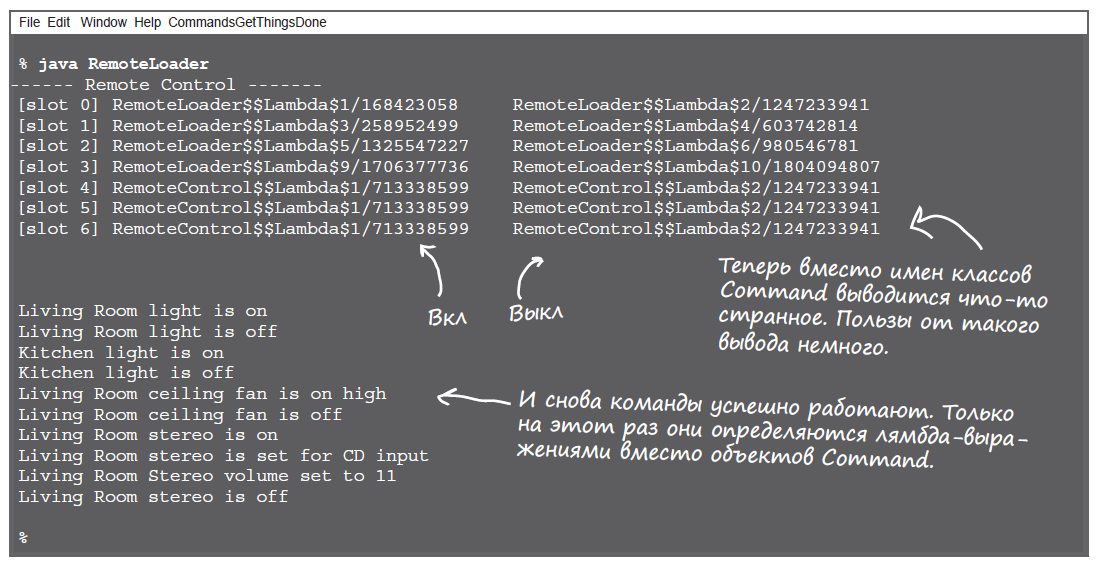
»More information about the book can be found on
the publisher's website.»
Table of Contents»
ExcerptFor Habrozhiteley 20% coupon discount -
Head First