The EcmaScript 2015 (ES6) standard has been around for several years. He brought with him many new features, different ways of using which are far from always obvious. Here is an overview of some of these features with examples and comments.
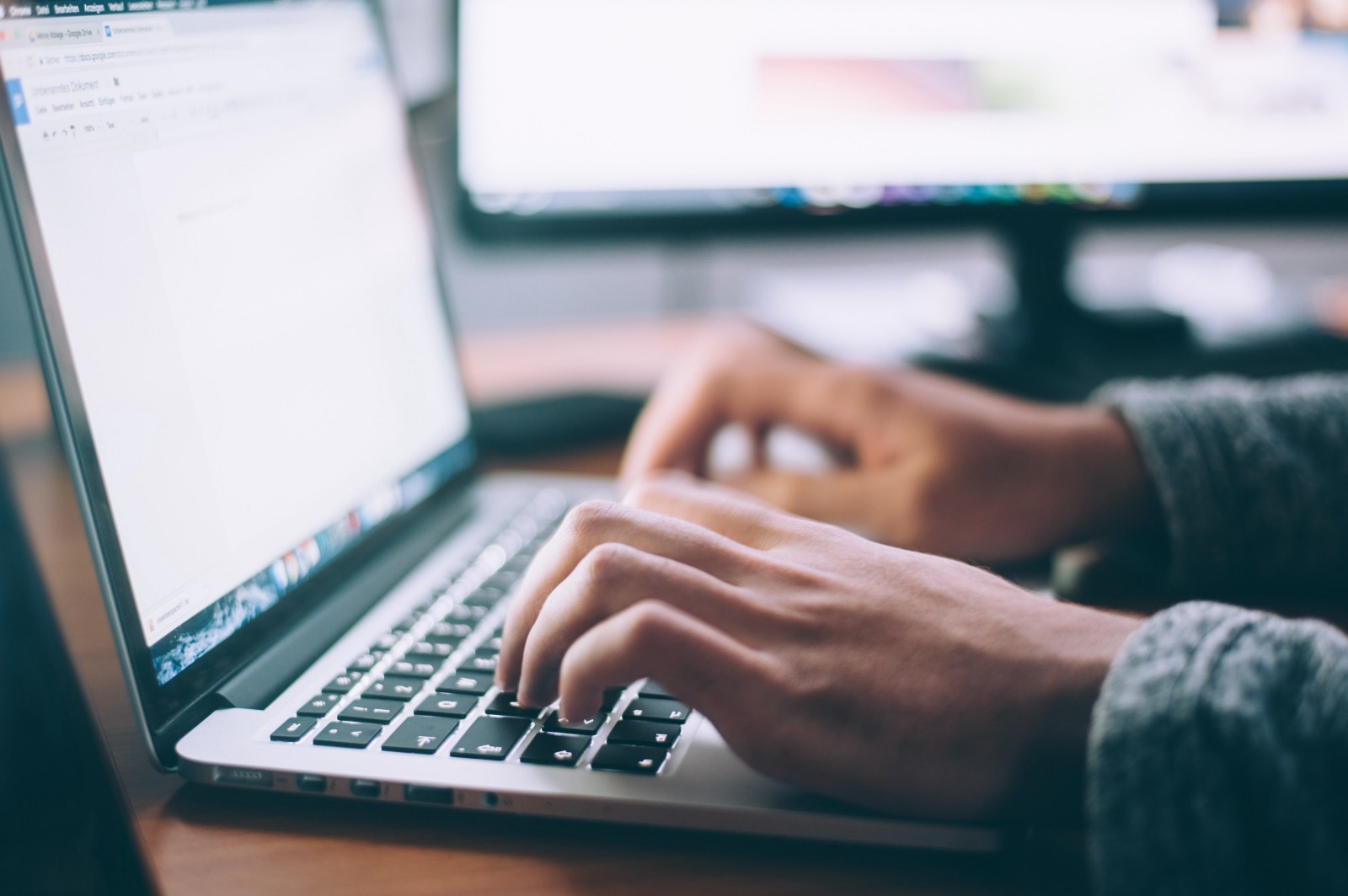
1. Required function parameters
ES6 allows you to
set the values of the formal parameters by default, which allows, when calling a function without specifying the values of these parameters, to substitute their standard values. This allows you to set parameters, without the transfer of which the function will not work.
In the following example, we set the
required()
function as the default value for parameters
a
and
b
. This means that if
a
or
b
not passed to the function when called, the
required()
function will be called and we will get an error message.
')
const required = () => {throw new Error('Missing parameter')}; // , "a" "b" const add = (a = required(), b = required()) => a + b; add(1, 2) //3 add(1) // Error: Missing parameter.
2. The secrets of the reduce method
The
reduce method of the
Array
object is extremely versatile. It is usually used to convert an array of certain elements to a single value. However, with it you can still make a lot of things useful.
Notice that in the following examples we rely on the fact that the initial value of a variable is either an array or an object, and not something like a string or a number.
▍2.1. Use reduce to simultaneously perform mapping and array filtering.
Imagine that we have the next task. There is a list of elements, each of which needs to be modified (which boils down to using the
map method), and then select several elements from it (this can be done using the
filter method). This problem is completely solved by the successive use of the
map
and
filter
methods, but you will have to go through the list of elements twice. And it does not suit us.
In the following example, you need to double the value of each element in the array, and then select only those that are more than 50. Pay attention to how we can use the powerful
reduce
method both for doubling and filtering elements. It is very effective.
const numbers = [10, 20, 30, 40]; const doubledOver50 = numbers.reduce((finalList, num) => { num = num * 2;
▍2.2. Use reduce instead of map or filter
If you analyzed the above example, then you will be completely clear the possibility of using the method of
reduce
instead of
map
or
filter
.
▍2.3. Use reduce to analyze parentheses
Here is another example of the useful features of the
reduce
method.
Suppose that we have a string with parentheses, and we need to find out if they are balanced, that is, first, whether the number of opening brackets is equal to the closing brackets, and, second, that the corresponding opening brackets are before the closing brackets.
This task can be solved using the
reduce
method as shown below. Here we use the
counter
variable with an initial value of 0. We increase its value by one if we find the “(”) symbol and decrease it if we find the “)” symbol. If the brackets in the string are balanced, the output should be 0.
// 0 . const isParensBalanced = (str) => { return str.split('').reduce((counter, char) => { if(counter < 0) { //matched ")" before "(" return counter; } else if(char === '(') { return ++counter; } else if(char === ')') { return --counter; } else { // - return counter; } }, 0); //<-- } isParensBalanced('(())') // 0 <-- isParensBalanced('(asdfds)') //0 <-- isParensBalanced('(()') // 1 <-- isParensBalanced(')(') // -1 <--
▍2.4. Counting the number of matching array values (converting an array into an object)
Sometimes you need to count the same elements of an array or convert an array into an object. To solve these problems, you can also use
reduce
.
In the following example, we want to count the number of cars of each type and put the results of these calculations in the object.
var cars = ['BMW','Benz', 'Benz', 'Tesla', 'BMW', 'Toyota']; var carsObj = cars.reduce(function (obj, name) { obj[name] = obj[name] ? ++obj[name] : 1; return obj; }, {}); carsObj;
In fact, the possibilities of
reduce
are not limited to this. If you are interested in details about this useful method - study the examples from
this page.
3. Object restructuring
▍3.1. Remove unwanted properties
It so happens that the object is required to remove unnecessary properties. Perhaps these are properties that contain sensitive information, perhaps - they are too great. Instead of iterating over the entire object and deleting similar properties, you can extract these properties into variables and leave the necessary properties in the rest parameter.
In the following example, we need to get rid of the properties of
_internal
and
tooBig
. They can be assigned to variables with the same names and save the remaining properties in the rest parameter
cleanObject
, which can be used later.
let {_internal, tooBig, ...cleanObject} = {el1: '1', _internal:"secret", tooBig:{}, el2: '2', el3: '3'}; console.log(cleanObject); // {el1: '1', el2: '2', el3: '3'}
▍3.2. Destructure nested objects in function parameters
In the following example, the
engine
property is an embedded object of the
car
object. If we need to know, say, the
vin
property of an
engine
object, we can easily destruct it.
var car = { model: 'bmw 2018', engine: { v6: true, turbo: true, vin: 12345 } } const modelAndVIN = ({model, engine: {vin}}) => { console.log(`model: ${model} vin: ${vin}`); } modelAndVIN(car);
▍3.3. Merge objects
ES6 supports the spread operator, which looks like three points. It is usually used to work with arrays, but it can also be used with ordinary objects.
In the following example, we use the extension operator to form a new object from two existing ones. The property keys of object number 2 will override the property keys of object number 1. In particular, the properties
b
and
c
from
object2
override the same properties of
object1
.
let object1 = { a:1, b:2,c:3 } let object2 = { b:30, c:40, d:50} let merged = {…object1, …object2}
4. Collections
▍4.1. Remove duplicate values from arrays
In ES6, you can get rid of duplicate values using collections (
Set ). Collections can contain only unique values.
let arr = [1, 1, 2, 2, 3, 3]; let deduped = [...new Set(arr)]
▍4.2. Using array methods with collections
Converting collections to arrays is no more difficult than using the above extension operator. All array methods can be used with collections.
Suppose we have a collection that needs to be filtered so that it contains only elements that are greater than 3. Here’s how to do it.
let mySet = new Set([1,2, 3, 4, 5]); var filtered = [...mySet].filter((x) => x > 3)
5. Destructuring arrays
It often happens that the function returns a set of values as an array. You can extract these values from an array using the destructuring technique.
▍5.1. Swapping variable values
Here is an example of how new array tools allow you to organize the exchange of the values of two variables.
let param1 = 1; let param2 = 2;
▍5.2. Receiving and processing multiple values returned by a function
In the following example, we load a certain article located at
/post
, and related comments from the address
/comments
. Here the
async/await
construction is used, the function returns the result as an array.
Restructuring simplifies the assignment of the results of the function to the corresponding variables.
async function getFullPost(){ return await Promise.all([ fetch('/post'), fetch('/comments') ]); } const [post, comments] = getFullPost();
Results
In this article, we looked at a few simple but not obvious ways to use the new features of ES6. We hope they will be useful to you.
Dear readers! If you know any interesting tricks for using ES6 features, please share them.
