In mid-January of this year, Amazon announced Go
support in its lambdas.
Great news, but now I am writing these lines, without having any experience writing code on Golang, so that, passing through the hemp and potholes in parallel with writing the article, come to my first working lambda on Go.
Setting Go and setting up the working space
Start, in fact, have to install
Go . My machine is Windows 10 on an Intel processor. Nothing complicated here: download, launch. Check that Go has set the path to <installation path> \ bin to the PATH environment variable or start go through cli (cmd, for example) to check.
Create a project folder:
C:\Users\a_gol\go>cd C:\Go C:\Go>mkdir workspace C:\Go>set GOPATH=C:\Go\workspace C:\Go>cd %GOPATH% C:\Go\workspace>
To edit the code, I use
Sublime Text 3 , so I need to adapt it to Go. To do this, you need to install the
package control and with its help (
Preferences -> Package Control -> Install Package -> GoSublime ) download the
GoSublime plugin.
Restart Sublime Text.
')
More information on setting up Sublime Text can be found
here .
Now let's try Go with the first
Hello, world app
!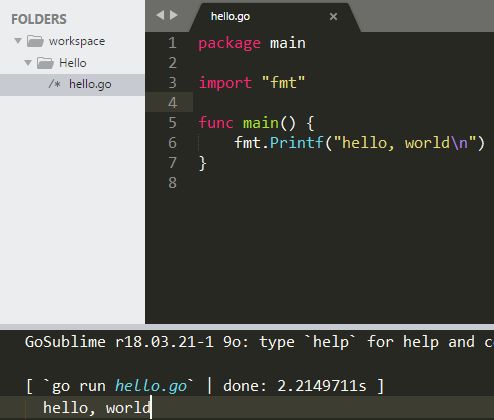
Lambda on go
Let's try to write and test a lambda that accepts an object from two fields in json format and gives a response in json format with a successful completion identifier.
Request:
{ "id": 12345, "value": "some-value" }
Answer:
{ "message": "processed request ID 12345", "ok": true }
This example is described in the
article .
package main import ( "fmt" "github.com/aws/aws-lambda-go/lambda" ) type Request struct { ID float64 `json:"id"` Value string `json:"value"` } type Response struct { Message string `json:"message"` Ok bool `json:"ok"` } func Handler(request Request) (Response, error) { return Response{ Message: fmt.Sprintf("Processed request ID %f", request.ID), Ok: true, }, nil } func main() { lambda.Start(Handler) }
To build an archive for lambda, you need to load the
aws-lambda-go library. There you can see what steps to build the archive you need to perform on Linux, macOS and Windows.
Let's do it all in steps:
- Download the package:
go get -u github.com/aws/aws-lambda-go/cmd/build-lambda-zip
- Set the environment variables in cmd:
set GOOS=linux set GOARCH=amd64
- In the Go console, you can run the following commands to build the archive, which we will deploy to the lambda (these commands are for Windows, for other axes here ).
go build -o main main.go %USERPROFILE%\Go\bin\build-lambda-zip.exe -o main.zip main
After these steps, you should have an archive main.zip, which you need to load into the lambda.

Creating Lambda in Amazon
I will create a lambda through the AWS console.
For this you need:
- Select Lambda Service in the required region
- Click Create function
- Fill in the fields
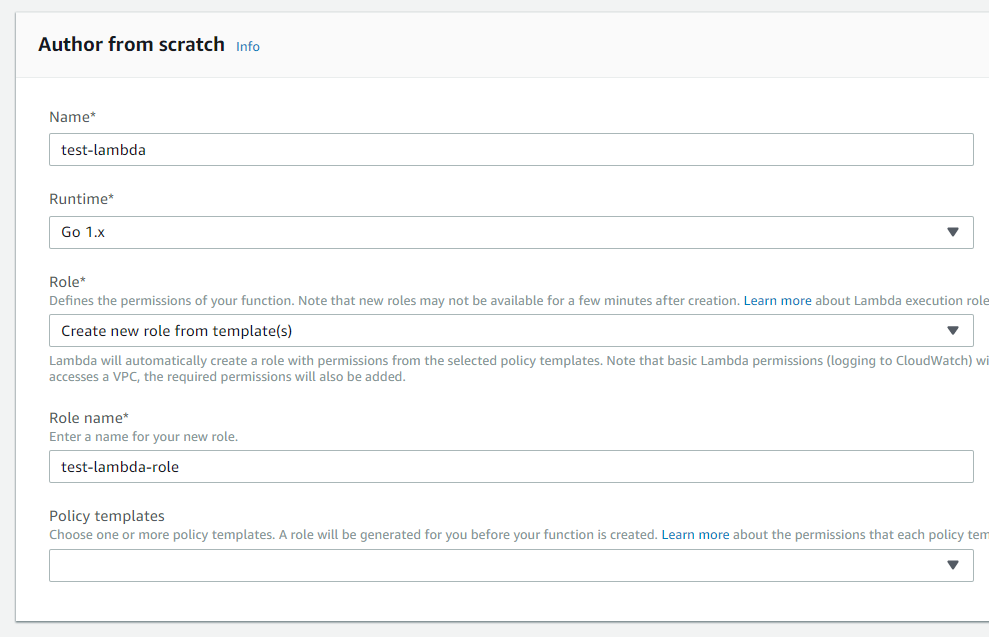
- After creation, the lambda console will open, where in the Function code block you need to click on the Upload button and load the main.zip archive. Do not forget to specify the value of main in the Handler field.
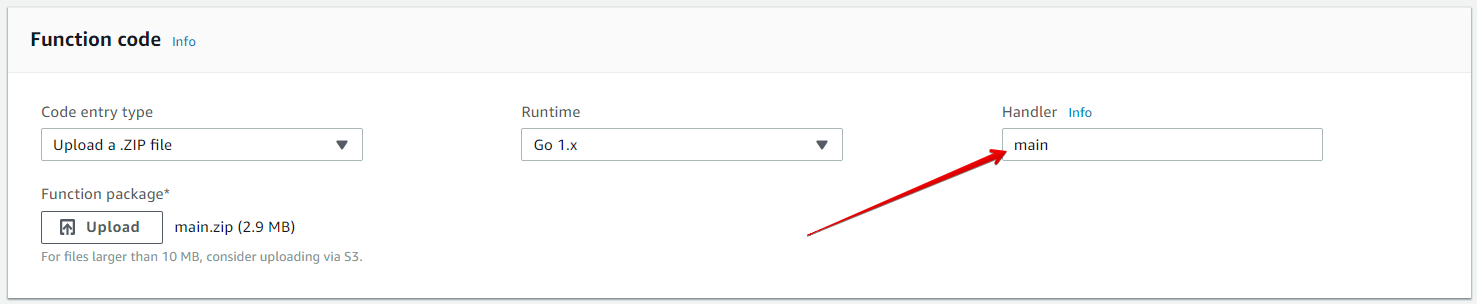
- At the top of the console, click Save.
Let's test the work of the lambda. To do this, create a test by clicking the Test button at the top of the console. Add test input and save.
{ "id": 12345, "value": "some-value" }

Now we can call this test script at the top of the console by clicking the Test button.

Bangles
Environment Variables Not Established
{ "errorMessage": "fork/exec /var/task/main: exec format error", "errorType": "PathError" }
set GOOS=linux set GOARCH=amd64
Check them in cmd:
echo %GOOS% echo %GOARCH%
Possible bumps
1. Main is not spelled out in the Handler field of the Function code block, hello is the default.
{ "errorMessage": "fork/exec /var/task/hello: no such file or directory", "errorType": "PathError" }
2. Invalid test json is set.
It should look like this:
{ "id": 12345, "value": "some-value" }