The programmer Travis Fisher, whose translation of the article we are publishing today, decided to tell about the most useful modules from his point of view and auxiliary tools for JS development. He considers the technologies he uses constantly and useful in the value of which he was convinced by his own experience as useful. In particular, we will focus on libraries and utilities designed for server and client development in JavaScript. Travis says that he did not strive to include something highly specialized in his material, or to do something like another
awesome list , which themselves are very useful, but usually turn out to be somewhat overloaded. Here we will talk only about the best that he can recommend with complete confidence to others.
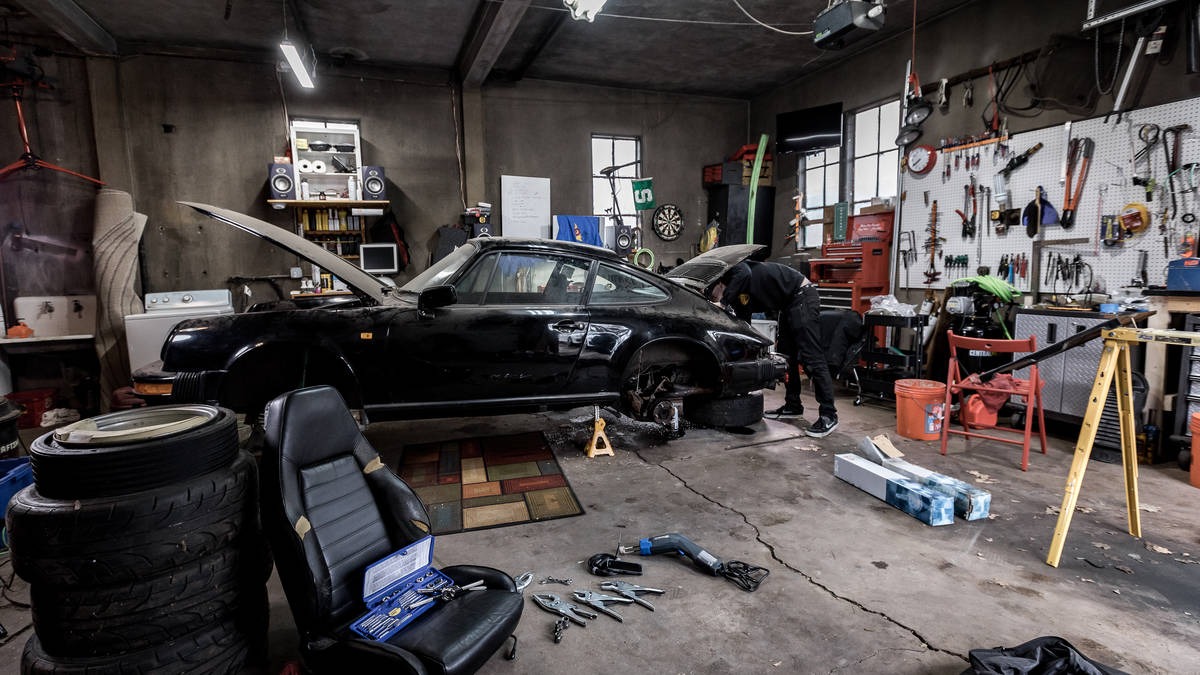
Command line tools
Let's start with some extremely useful command line tools.
â–ŤNp
The
np command is a very good alternative to
npm publish
.
')
If you publish your development in npm, I highly recommend looking at
np , as this command line tool greatly simplifies the versioning process, helps you work with git-release tags and publish packages in npm, especially if it happens that you support more than pairs of modules. Also, if you are interested in the
np
utility, take a look at the
release tool created by
Zeit . It is designed to automate the publication of releases of GitHub projects.
Publish package using np (image source - Sindre Sorhus )â–ŤYarn
Yarn is a package manager that is compatible with npm and features advanced features.
Although npm
v5 is much faster than its previous versions, I still, for local development, prefer yarn for its speed and stability. In any case, using yarn, you work with the same databases of modules as when using npm, while it is impossible to say with certainty - which is better - yarn or npm. Choose the manager that best meets the needs of your project. I suppose, the one who is engaged in JS-development should learn npm and yarn.
â–ŤPrettier
Prettier is a tool for formatting code.
Prettier allows you to maintain a uniform style of code, reducing the text of programs to the form given by its rules, which, in particular, take into account the maximum permissible length of the line, transferring the code to a new line where it is needed.
I like
eslint , and I, in particular, have long used the JavaScript
Standard Style , but the ideas on which the automatic tools for formatting the code, like
prettier and
gofmt , are
built , seem to me very attractive.
Developers spend too much energy thinking about the presentation and styling of the code, while the
prettier eliminates the need for such reflections. Automatic code formatting allows programmers to focus on what they write and not think about how it should look.
Prettier (image source - Prettier )â–ŤNow
Now is an extremely simple code deployment system created by Zeit.
Now is the best of the existing systems for deploying projects, considering factors such as simplicity, reliability and functionality. It is great for different situations and scales well with the growth of project needs. And, by the way, it is free as long as you do not need its ability to scale.
Now is suitable for working with web applications written in JS, using Node.js as the server side. In addition, I recommend looking at other
Zeit designs. This company produces decent products thanks to a strong team of JS-developers.
Zeit (image source - Zeit )â–ŤAsciinema
Asciinema is a free tool for high-quality recording of what is happening in the terminal.
Here is my material on this topic, devoted to the analysis of the features of using
asciinema to create high-quality, professional-looking code demonstrations and screencasts.
Promises
This section deserves a separate article, especially now that the
async / await mechanism has begun to become the de facto standard in the field of parallel programming in JavaScript. Having noted this, I highly recommend a look at the excellent collection of tools for working with promises presented by the
promise-fun module from
Sindre Sorhus .
The only thing I would like to warn you about is that these tools probably won't work without additional settings with most frontend tools like
create-react-app or
rollup .
Here are some useful tools for working with promises and asynchronous code in the Node.js environment.
â–ŤPify
The
pify package is a means for converting callback functions to promises.
There are many ways to convert callback functions to promises, but I found out that
pify is the best thing that exists in this area. This is a small module that has some nice little things like built-in automatic binding methods, which is missing in the standard
util.promisify .
â–ŤP-map
The
p-map module is designed to organize parallel work with promises.
Parallel code execution is a very useful technique, but in practical use it is necessary to set limits, because otherwise, for example, too many parallel network requests can overload the network or require too much system resources. It is in such situations that the
p-map shows itself in all its glory. I use it, almost always, as a replacement for the
promise.all(…)
construct, which does not support the assignment of constraints.
Before I learned about
p-map
, I created
something similar on my own, but I recommend
p-map
to everyone, since this tool is definitely better than my design.
â–ŤP-retry
P-retry allows
you to re-execute asynchronous functions or functions that return promises.
Usually, I wrap any HTTP requests and external service calls in
p-retry in order to equip the code with basic fault tolerance mechanisms. In combination with this
p-map tool, you can handle large sets of requests to external resources, controlling the parallel execution of such requests and not particularly worrying about intermittent errors during transmission of data, such as those caused by socket problems or server timeouts.
â–ŤP-timeout
P-timeout completes a failed
timeout promise.
The
p-timeout module, along with
p-retry , are simply the necessary tools for organizing reliable work with third-party APIs and services. In addition,
p-timeout
allows you to assign functions that are performed in case of unsuccessful promise. Usually returning something meaningful, performed within a reasonable time, is better than endless fruitless waiting or getting results for an indefinite time.
PP-cache and p-memoize modules
The
p-cache and
p-memoize modules allow you to
memoize the results of asynchronous functions using the
LRU cache .
The purpose of many of the above promis utilities reminds me a lot of design of fault-tolerant
microservices , where each external dependency can be handled using a common interface that supports query re-execution, timeouts, caching, request interruption, backup mechanisms, and so on.
A simplified version of a certain functionality is usually preferable to system overload or a complete lack of results, so if you are not familiar with microservices, take a look at them and try to understand whether approaches to their design can improve your ability to work with promises.
Web scraping
There are many excellent utilities for web scraping, some of which, like
cheerio , work at the level of the source HTML code, and some, such as
puppeteer , allow you to process pages with a full browser. What exactly to choose depends on the goals of a specific project, since working with HTML is much faster and requires less system resources, while automating a browser without a user interface is more complicated and requires more serious preparation.
â–ŤCheerio
Cheerio is a fast, flexible and miniature implementation of the basic jQuery features, created specifically for the server and designed to work with HTML.
Cheerio
is a great tool for quick web scraping, suitable for parsing HTML pages. This tool has a convenient syntax similar to jQuery and is designed to bypass HTML documents and perform manipulations with them.
Cheerio
fits well with the
request-promise-native library, designed for downloading remote HTML documents.
â–ŤPuppeteer
Puppeteer is a Node-API for managing Chrome browser without a user interface.
Unlike
cheerio
,
puppeteer
is a wrapper for Chrome automation without a user interface, which is very useful for working with modern web applications, including single-page, JavaScript-based. Since work is being done with the Chrome browser,
puppeteer
characterized by a high level of support for modern web standards. This affects the ability to properly form pages and run scripts. The Chrome browser without a user interface is a relatively new tool, but it is very likely to gradually replace older tools such as
PhantomJS .
As a result, it can be said that
puppeteer has no equal in the matter of advanced web scraping, programmatically creating screenshots of pages and automating various works with web projects. In addition, it is worth noting that over time
puppeteer
will become increasingly popular.
Libraries for Node.js
OtenDotenv-safe
Dotenv-safe allows you to load environment variables from an
.env
file and ensures that all the necessary variables are present.
This module extends the highly popular
dotenv module by supporting an indication of the expected environment variables using
.env.example
. Like the base library,
dotenv-safe
provides fast, safe and reliable work with environment variables in the Node.js environment.
In addition, this module is well integrated with the deployment system
now.sh with the
"dotenv": true
option set
"dotenv": true
at
now.json .
â–ŤRequest and request-promise-native
The
request library and the
request-promise-native library based on it, which equip it with support for ES6 promises, are clients that simplify the execution of HTTP requests.
Execution of HTTP requests is an operation that is needed almost always and everywhere. I use the
request-promise-native
module to perform this operation. This happens in 95% of cases when it is required to organize waiting for the result of an HTTP request wrapped in a promise. In the remaining 5% of cases, when you need to work with the response flow directly, I use the
request
module, which does not support promises.
For reliability, I often wrap
request-promise-native
calls into some combination of
p-retry ,
p-timeout , and
p-cache .
In addition, as a new alternative to
request
, it is worth noting the
got library, which has built-in support for promises. I don’t use this library very often, but you might like it.
Here is an example of loading an HTML document using
request-promise-native
.
const request = require('request-promise-native') const html = await request('https://github.com') console.log(html)
â–ŤConsolidate
Consolidate is a library that provides consistent work with different template engines for Node.js.
Consolidate is great for working with any server templates. For example, these are templates for emails, tweets, HTML pages. I usually use
handlebar s as a template engine, but I work with it through
consolidate
. This gives me the uniformity of the template interface, which does not depend on the system used to work with templates. For example, I used
consolidate
in
create-react-library to output base patterns.
â–ŤExeca
Execa is a very good alternative for
child_process
.
The
execa
module
execa
very useful in cases where you need to create child processes, for example, to work with the command shell.
â–ŤFs-extra
Fs-extra is an improved version of
fs
that supports additional methods and promises.
I rarely use
fs
directly. Try
fs-extra
and you probably won't want to use
fs
again.
Calculations
D3 (Data Driven Documents) is a popular front-end library for data visualization and animation. In addition, it contains great math computational packages that can be used independently. I often find myself using these packages.
â–ŤD3-random
D3-random allows you to generate random numbers from various distributions.
When
Math.random
does not give the desired result - try the library
d3-random
. This library supports work with various distributions of random variables, including uniform, normal and exponential.
â–ŤD3-ease
D3-ease gives the developer various functions to create smooth animations.
D3-ease (image source - D3-ease )â–ŤD3-interpolate
D3-interpolate allows you to interpol numbers, colors, strings, arrays, objects, in general - anything.
This module provides a variety of interpolation methods to ensure a smooth transition between two arbitrary values. These values ​​can be numbers, colors, strings, arrays, or even objects that have other objects.
Testing
â–ŤAva
Ava is a great tool for running JS tests.
Given the quality of the tools created by Sindre Sorhus, it is not surprising that Ava, my favorite tool for running unit tests on Node.js platform, is their development. The Ava project borrowed a lot of good things from
mocha ,
tape ,
chai and other testing tools. All this is collected in a single package, the standard settings of which are suitable for real work.
It is worth noting that the Ava tests are run, by default, in parallel, although this can be changed at the level of individual files. This is necessary in cases where the order of running tests is important, for example, when testing systems that work with databases.
Ava (image source - ava )â–ŤNock
Nock is a library for simulating the execution of HTTP requests.
Nock
great for isolated testing of modules that perform HTTP requests. If your Node module performs HTTP requests and you want to prepare good tests for it - pay attention to
nock
.
â–ŤSinon
Sinon is a system that allows you to test JS projects using spies (spy), caps (stub) and imitations (mock).
The Sinon library contains very useful tools for writing isolated tests using the dependency injection mechanism. Perhaps she is one of the worthy candidates for a place in the toolkit of each developer.
Results
I hope you found in my review something useful, at least one quality tool that you didn’t know about before. I know many experienced developers who are prone to creating their own tools to solve common problems. This is not to say that it is bad. However, I believe that it is useful for anyone to know about what has already been done by others, about quality tools, the use of which will allow not to engage in the constant invention of bicycles.
The breadth of problems inherent in the NPM module library is incredible, and in my opinion, this is one of the great advantages of JavaScript in comparison with other languages. A programmer who masterfully uses the capabilities of the npm ecosystem can seriously improve the efficiency of his work. Perhaps it is this skill that is one of the distinguishing features of a developer who, according to
myths , is ten times more productive than the average programmer.
Dear readers! What tools and libraries that facilitate and accelerate the development of JS-projects, you usually use?