The material about environment variables in Node.js, the translation of which we are publishing today, was written by
Berk Holland , a web developer whose interests include JavaScript, Node.js, and VS Code. In addition, it is worth noting that he had a difficult relationship with Java. Here is his story.
Environment variables are one of the fundamental constructs of the Node.js environment, but for some reason I never tried to learn how to use them correctly. Perhaps it happened because of their name - "Environment Variables". This title gave me something of a post-traumatic syndrome, unpleasant memories of how I tried to add the path to the Java home directory in Windows. Then I really could not understand whether it is necessary to add this path to the PATH variable, to the JAVA_HOME variable, or both there and there. It was also unclear whether it was necessary to have a semicolon at the end of this path. As a matter of fact, then I had a question about why I use Java. Whatever it was, I finally found the strength in myself and began to get acquainted with the environment variables Node.
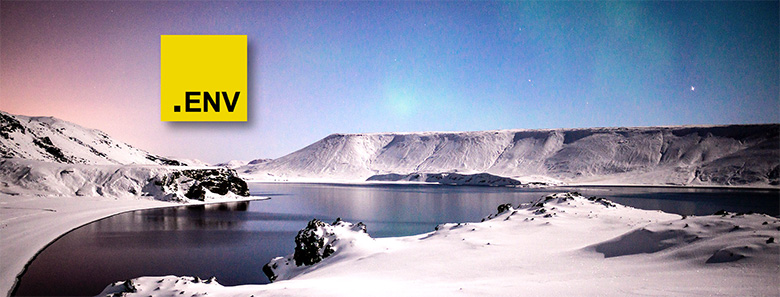
')
If you are writing for the Node.js platform, and, just like me, it does not matter - for what reasons, you are still not particularly familiar with environment variables - I suggest fixing this.
Environment Variables in Node.js
In Node, environment variables can be global (as in Windows), but they are often used to apply to a particular process in which the developer is interested. For example, if you have a web application, this means that the following environment variables can be used in it:
- The HTTP port that this application will listen on.
- The string to connect to the database.
- The path to JAVA_HOME, oh ​​wait, this is past. Please note that recovery takes time.
In this context, environment variables are in fact more like “Configuration Settings” - for me, it sounds much better than the “Environment Variables”.
If you previously programmed for .NET, you may be familiar with something like a
web.config
. The Node environment variables play almost the same role as the settings from the
web.config
- they are a mechanism for passing information to the application that the developer does not want to set hard in the code.
By the way, on the topic “hard code” - tasks in the code of certain values ​​instead of receiving them from external sources, I want to share my own tweet.
I quote myself at the height of insanityHow to use environment variables in Node.js applications? I had to work pretty hard to find good material on environment variables in Node, with the indispensable condition that there is enough jokes about Java in this material. I did not find such material, so I decided to write it myself.
Here are some ways to use environment variables in Node.js applications.
Specifying environment variables in the terminal
You can specify environment variables in the terminal where you plan to launch Node. For example, if you have an application that uses Express, and you want to send port information to it, you can do it like this:
PORT=65534 node bin/www
By the way, an interesting thing. It turns out that the largest number that a port number can take is 65535. How did I find out? Of course, I found it on
StackOverflow . How does anyone know anything? But in Node, the largest port number is 65534. Why? I have no idea. I can't know absolutely everything.
So, to use the environment variable in the code, you need to use the
process.env
object. It looks like this:
var port = process.env.PORT;
However, the use of the approach described here can end badly. If you have, for example, a database connection string, you probably will not be particularly pleased with the prospects for its entry into the terminal. In fact, entering long values ​​in the terminal looks like a painful dependency, which is completely useless to us. See for yourself:
PORT=65534 DB_CONN="mongodb://react-cosmos-db:swQOhAsVjfHx3Q9VXh29T9U8xQNVGQ78lEQaL6yMNq3rOSA1WhUXHTOcmDf38Q8rg14NHtQLcUuMA==@react-cosmos-db.documents.azure.com:19373/?ssl=true&replicaSet=globaldb" SECRET_KEY=b6264fca-8adf-457f-a94f-5a4b0d1ca2b9 node bin/www
This approach does not scale, but everyone wants to scale. According to each architect, next to whom I used to sit at different events, “scaling” is even more important than the fact that the application is working.
Therefore, we consider another approach, which is to use
.env
files.
Using .env files
The
.env
files are for storing environment variables. To use this technology, it is enough to create a file in the project with the name
.env
and enter into it the environment variables, starting each with a new line:
PORT=65534 DB_CONN="mongodb://react-cosmos-db:swQOhAsVjfHx3Q9VXh29T9U8xQNVGQ78lEQaL6yMNq3rOSA1WhUXHTOcmDf38Q8rg14NHtQLcUuMA==@react-cosmos-db.documents.azure.com:10255/?ssl=true&replicaSet=globaldb" SECRET_KEY="b6264fca-8adf-457f-a94f-5a4b0d1ca2b9"
You can read these values ​​in different ways. Perhaps the easiest way is to use the
npm
dotenv
npm
:
npm install dotenv
After installing the package, you need to connect it to the project, and then you can use it to work with environment variables. This package will find the
.env
file and load the variables described in it into the Node. Here's what it looks like:
// dotenv .env Node require('dotenv').config(); var MongoClient = require('mongodb').MongoClient; // .env, process.env MongoClient.connect(process.env.DB_CONN, function(err, db) { if(!err) { console.log("We are connected"); } });
By the way, I want to share important advice. Do not upload
.env
files to GitHub. It contains information that is not intended for prying eyes. Do not repeat my mistakes.
So, everything is fine so far, but what we have said here is not very convenient. You
dotenv
to connect
dotenv
in every file where you need to use environment variables, and
dotenv
will
dotenv
to be used in production when, in essence, you don’t need it. I do not belong to fans of deploying useless code in production, although, it seems to me, so far I have been doing just that.
What to do? Fortunately, you are using
VS Code (I am absolutely sure of this), which means that you have a few more options.
Working with .env files in VS Code
For starters, you can install the VS Code
DotENV extension, which will give a nice syntax highlighting for
.env
files.
This is what an .env file looks like without syntax highlighting and with highlighting.In addition, the VS Code debugger, if you use it, offers some more convenient options for loading values ​​from
.env
files.
VS Code Startup Configuration
The Node.js debugger for VS Code (it is installed by default) supports loading of
.env
files through launch configurations. Details on launch configurations can be found
here .
Creating a basic launch configuration for NodeAfter you create the basic startup configuration for Node (click the gear icon and select Node), you can do one of the following, or do both.
The first option is to include variables in the configuration file.
Variables in the configuration fileThis is an acceptable option, but I am a little worried that each value should be a string. Still, some values ​​are numbers, not strings, and in JavaScript there are only, let's say, three main data types, and I would not want to lose one of them.
Passing environment variables to VS Code is possible in a simpler way. We have already found out that the
.env
files are our friends, so instead of
.env
values ​​of variables in the configuration file, we simply specify the path to the
.env
file
.env
.
Path to the .env file in the configuration fileAs long as we start the Node.js process from VS Code, the file with environment variables will be transferred to this process. At the same time, we will not have to squeeze numbers into quotes, making them strings, and we will not have to deploy unnecessary code in production. Well, at least you won't have to do this.
Running node scripts via NPM
Perhaps you have reached this place and thought that you never start Node projects with commands like
node …
, always using npm scripts like
npm start
. VS Code launch configurations can also be used in this case. However, instead of using the standard launch Node, you can configure the Launch Via NPM task.
Task Launch Via NPMAfter that, you can customize the value of the
envFile
parameter by specifying the path to the
.env
file, and the
runtimeArgs
parameter to run the desired script. Usually, the script is
start
or
debug
.
Setting up a project run using npmNote that in
package.json
you need to add the
--inspect
flag to the npm script so that VS Code can connect a debugger to the process. Otherwise, although the task will start, the debugger cannot do anything useful.
--Inspect flag in package.jsonProduction variables
So, we figured out how to use environment variables during development. You, most likely, will not use the
.env
files in production, and the VS Code launch configuration on the server will not bring any special benefit.
In production, the definition of environment variables will depend on the particular platform used. For example, in the case of Azure, there are three ways to declare and manage such variables.
The first way is to use the
Azure CLI .
az webapp config appsettings set -g MyResourceGroup -n MyApp
It works, but it doesn't look very good. Another way is to use the Azure web portal. I don’t often use the web portal, but when it happens, I refer to it to set environment variables.
Here, what we call “environment variables” is called “Application Settings”.
Setting Environment Variables in AzureAnother option, given that you are using VS Code, is to install the App Service extension and configure the above-described Application Settings directly from the editor.
Setting Environment Variables from VS CodeI like to do absolutely everything in VS Code, and if I could write e-mails there, I would do that.
By the way , it seems my dream has come true.
Results
Now you know the same thing that I know (not so much, I will allow to notice), and I feel that I have fulfilled my goal on jokes on the subject of Java. If suddenly they are not enough here - here is another one whose author is unknown: "Java is a very powerful tool for turning XML into stack-traces."
Hopefully, this material made it possible for those who had not used them to fill in the gaps in knowledge about environment variables in Node, and for those who already knew about them, they allowed to learn something new about .env files, about using environment variables on combat servers , and how to make your life easier by developing Node-projects in VS Code. If the topic of environment variables Node is interesting to you -
here is one of our previous publications on this topic, dedicated to the features of working with process.env.
Dear readers! How do you solve the problems of using environment variables in the development and production of Node?