ReactJS library is designed to create UI. As a rule, the user interface is not only graphics, but also sound. New message in the messenger, warning of an error, pressing buttons, etc. - in full-fledged applications such events are marked by some kind of audio effect.
For sound effects in ReactJS, you can use the
midi-sounds-react component . An example for assessing the functional component can be viewed (listened) on the
test page .
Consider the connection and use of the component in the project.
Setting up the environment
Install
Node.js and
ReactJS , then
create a new project for tests.
')
Add a link to midi-sounds-react to your package.json. It should look like this:
{ "name": "my-test", "version": "0.1.0", "private": true, "homepage": "https://myserver", "dependencies": { "react": "^16.2.0", "react-dom": "^16.2.0", "react-scripts": "1.1.0", "midi-sounds-react": "^1.2.45" }, "scripts": { "start": "react-scripts start", "build": "react-scripts build", "test": "react-scripts test --env=jsdom", "eject": "react-scripts eject" } }
Add import
import MIDISounds from 'midi-sounds-react';
and place the component anywhere on the page
<MIDISounds ref={(ref) => (this.midiSounds = ref)} appElementName="root" instruments={[]} drums={[]} />
specifying the value of attributes:
- this.midiSounds - variable for accessing the component
- appElementName - id of the main diva of the application
- instruments - an array with the numbers of the instruments that will be loaded when the page is displayed
- drums - array with drum numbers that will be loaded when the page is displayed
Sample code App.js
import React, { Component } from 'react'; import logo from './logo.svg'; import './App.css'; import MIDISounds from 'midi-sounds-react'; class App extends Component { playTestInstrument() { this.midiSounds.playChordNow(3, [60], 2.5); } render() { return ( <div className="App"> <header className="App-header"> <img src={logo} className="App-logo" alt="logo" /> <h1 className="App-title">Welcome to midi-sounds-react example 1</h1> </header> <p className="App-intro">Press Play to play instrument sound.</p> <p><button onClick={this.playTestInstrument.bind(this)}>Play</button></p> <MIDISounds ref={(ref) => (this.midiSounds = ref)} appElementName="root" instruments={[3]} /> </div> ); } } export default App;
It will look like this page with one button and the component logo:

the button will sound one note of the piano; by clicking on the logo, the settings dialog will open:
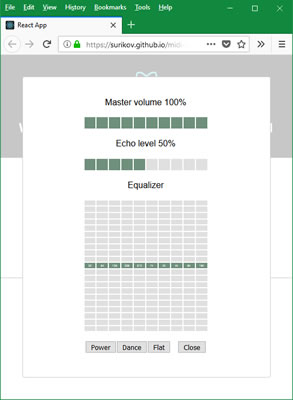
Open the
example in the browser.
Other examples
In addition to sound effects, the midi-sounds-react component can be used to play
musical fragments created on the fly, as well as the development of
musical editors :

or
virtual instruments :

The code for all the examples
is available on GitHub .
Restrictions
For trouble-free operation of the component, it is desirable to use the Chrome browser or Safari.
For mobile browsers, echo (reverb) is too heavy a function and it is advisable to turn it off in mobile versions.
Source Code and Documentation
Available on
page .