Android version 26 of the SDK brought new features to our Android apps, including fonts as resources, downloadable fonts, support for emoji, automatic TextView sizes, physics-driven animation through Spring and Fling, backward compatibility for vector animations, and reducing the library by increasing the minimum sdk version up to 14.
If you are not familiar with the Android Support Library, then know that you need to compile the application at the same level of the Android API as the latest version of Android Support Library. In other words, make sure the TargetFramework tag is set to 8.0 (API 26). In this case, the application will be compiled using the latest version of the Android Support Library (V26).
XML font support
Now you can place fonts in the new font font resource folder. Use Resources.GetFont or ResourcesCompat.GetFont to download font resources into your application.
')
Example: defining a font in XML in the Resourcesfont folder:
<?xml version="1.0" encoding="utf-8"?> <font-family xmlns:android="http://schemas.android.com/apk/res/android"> <font android:fontStyle="normal" android:fontWeight="400" android:font="@font/lobster_regular" /> <font android:fontStyle="italic" android:fontWeight="400" android:font="@font/lobster_italic" /> </font-family>
Use font resource in View:
<TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:fontFamily="@font/lobster"/>
Downloadable fonts
There is a new FontsContractCompat class that will allow you to request fonts from the font provider instead of packaging them inside your application. You can use the font provider from Google Fonts (800+ fonts).
To use it, first create a FontRequest:
FontRequest request = new FontRequest( "com.google.android.gms.fonts", "com.google.android.gms", query, Resource.Array.com_google_android_gms_fonts_certs);
Secondly, you need to register FontRequestCallback, which implements OnTypefaceRetrieved (Android.Graphics.Typeface typeface) and OnTypefaceRequestFailed (int reason). We created one that you can use, in this example:
FontsContractCompat.FontRequestCallback callback = new FontRequestCallbackImpl { mActivity = this, mDownloadableFontTextView = DownloadableFontTextView, mRequestDownloadButton = RequestDownloadButton, mProgressBar = progressBar };
Finally, you need to request a font:
FontsContractCompat.RequestFont (this, request, callback, GetHandlerThreadHandler ());
You can also request the font directly in XML:
<font-family xmlns:android="http://schemas.android.com/apk/res/android" android:fontProviderAuthority="com.google.android.gms.fonts" android:fontProviderPackage="com.google.android.gms" android:fontProviderQuery="Lobster Two" android:fontProviderCerts="@array/com_google_android_gms_fonts_certs" />
Emoji Compatibility
The EmojiCompat support library will allow your devices to use the latest emojis without requiring an Android OS update. This helps to avoid the image of annoying empty squares (â–ˇ)!
EmojiCompat has two main libraries: downloadable or batch.
Downloadable
As noted above in the “Downloadable Fonts” section of this post, you first need to create a FontRequest to create the FontRequestEmojiCompatConfig.
EmojiCompat.Config config; var fontRequest = new FontRequest( "com.google.android.gms.fonts", "com.google.android.gms", "Noto Color Emoji Compat", Resource.Array.com_google_android_gms_fonts_certs); config = new FontRequestEmojiCompatConfig(this, fontRequest) .SetReplaceAll(true) .RegisterInitCallback(new InitCallbackImpl());
Batch
A batch library makes life a little easier in about 7 Mbps of batch font. All you need to do is create a BundledEmojiCompatConfig:
EmojiCompat.Config config; config = new BundledEmojiCompatConfig(this);
Emoji widgets
The EmojiCompat library provides us with three main elements for displaying emoji:
EmojiTextView, EmojiEditTExt, and EmojiButton
Automatic textview size selection
Your TextView now automatically increases the size of the text when the container is enlarged. There are three ways you can automatically select a TextView size, and they are explained below.
Start by describing android: autoSizeTextType as uniform.
<TextView android:layout_width="match_parent" android:layout_height="200dp" app:autoSizeTextType="uniform" />
Degree of breakdown
You can also define a range between the minimum and maximum text size for your TextView. It may also increase incrementally according to the degree of breakdown you specify.
<TextView android:layout_width="match_parent" android:layout_height="200dp" app:autoSizeTextType="uniform" app:autoSizeMinTextSize="12sp" app:autoSizeMaxTextSize="100sp" app:autoSizeStepGranularity="2sp" />
Dimensioning
Finally, you can specify all the values ​​that TextView can use when automatically selecting the size. You can specify an array resource of previously specified sizes:
<resources> <array name="autosize_text_sizes"> <item>10sp</item> <item>12sp</item> <item>20sp</item> <item>40sp</item> <item>100sp</item> </array> </resources>
Now you only need to specify the value of android: autoSizePresetSizes for the array that we have already created:
<TextView android:layout_width="match_parent" android:layout_height="200dp" app:autoSizeTextType="uniform" app:autoSizePresetSizes="@array/autosize_text_sizes" />
Dynamic animation
Now you can use speed based animation instead of duration based animation. Such animation looks more natural, with movements that mimic a sudden movement or spring.
To create our first dynamic animation, create a new SpringAnimation object using View, ViewProperty and finalPosition.
SpringAnimation animX = new SpringAnimation (box, DynamicAnimation.TranslationX, 0);
There are two basic concepts you can ask for a spring: Stiffness and DampingRatio.
Stiffness determines how quickly the spring returns to its original state, and DampingRatio determines how elastic the spring is.
animX.Spring.SetStiffness(Stiffness); animX.Spring.SetDampingRatio(Damping);
Then you can set your StartVelocity speed and start the animation!
animX.SetStartVelocity(velocityTracker.XVelocity); animX.Start();
AnimatedVectorDrawableCompat (bonus)
If you don’t know about AnimatedVectorDrawableCompat, then this is a beautiful stylish library for navigating between paths and interpolating along the path to create stunning animations, transform logos and more. All of them are now tied to API 14, which allows these beautiful animation vectors to work with older devices.
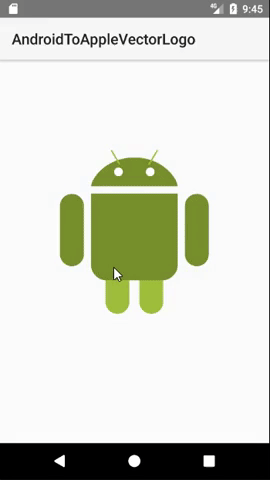
You can create your own beautiful animations in vector graphics using XML, creating an animated-vector element and attaching a pathInterpolators to a specific objectAnimator. If you are not the best animator in the world, you can start here with the Alex Lockwood tool:
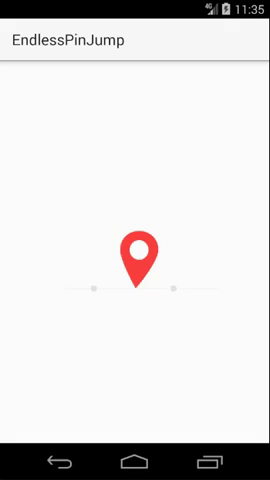
findings
There are many good features that Android provides within its supporting libraries that you can use when developing Xamarin applications. These characteristics are usually compatible with previous versions of minSdkVersion, which are defined by the helper library. Now you have a chance to explore what auxiliary libraries can give your applications!
Source:
John Douglas's Android Support Library v26 Overview article dated October 2, 2017.