Hello! You are reading a translation of the article Donavon West
“Conditional Rendering in React using Ternaries and Logical AND”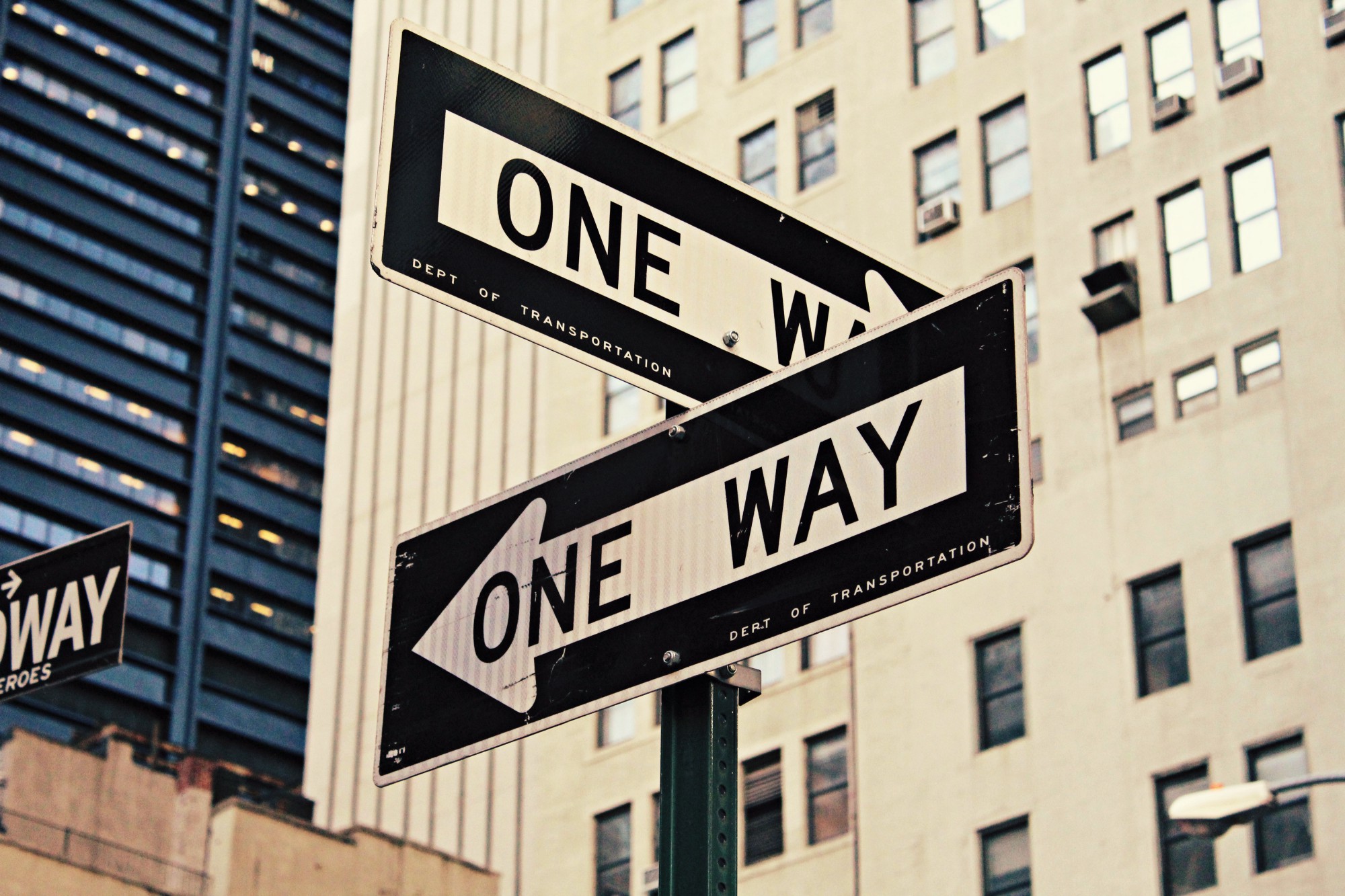
There are several ways to make React render what you need. You can use the traditional
if or
switch statement . In this article we will consider an alternative to these methods. Be careful: it carries some surprises that you should be more careful with.
Ternary operators vs if / else
Let's imagine that our component gets the
name property. If the string is not empty, then we will display a greeting, otherwise we will prompt the user to register.
')
This can be done using the stateless function component (
Original SFC - Stateless Function Component ):
const MyComponent = ({ name }) => { if (name) { return ( <div className="hello"> , {name} </div> ); } return ( <div className="hello"> , </div> ); };
Everything is pretty clear here. But we can do better! Here is the same example written with ternary operators:
const MyComponent = ({ name }) => ( <div className="hello"> {name ? `, ${name}` : ', '} </div> );
Notice how our code shrank. Since we use the arrow function, we do not need
return , and the ternary operator allows us to get rid of the repetition of html-tags.
Ternary operators vs logical “AND”
As you can see, ternary operators are convenient in terms of
if / else . But what if we only have
if ?
Let's give another example. Now if
isPro is
true for us, we display the inscription (in the picture after it is the cup). We also derive the number of stars if there are more than 0. You can do this:
const MyComponent = ({ name, isPro, stars}) => ( <div className="hello"> <div> Hello {name} {isPro ? '' : null} </div> {stars ? ( <div> Stars:{'* '.repeat(stars)} </div> ) : null} </div> );
Notice that
else returns
null . Since the ternary operator expects
else , this cannot be avoided.
For
if alone, there is something more pleasant, a
logical “AND” :
const MyComponent = ({ name, isPro, stars}) => ( <div className="hello"> <div> Hello {name} {isPro && ''} </div> {stars && ( <div> Stars:{'* '.repeat(stars)} </div> )} </div> );
Not much different, but now we don’t have the annoying
: null (i.e. else condition). Everything, in theory, should be rendered as before.

What is it? What happened to John? Instead of emptiness, somewhere we took 0. This is the surprise that was mentioned earlier.
If we turn to MDN, then the logical “AND” (&&):
expr1 && expr2
Returns expr1 if it can be converted to false ; otherwise, it returns expr2 . Moreover, when used with logical values (boolean), && returns true if both values are true ; otherwise returns false .
Okay. Before you go ingest antidepressants, let me explain.
In our case,
expr1 is
stars whose value is
0 . Since zero is a lie, it returns the original state. See, not so difficult.
You can rewrite humanly:
If expr1 is false, then returns expr1 ; otherwise returns expr2 .
Therefore, when you use a logical “AND” with a non-zero value, you need to do something with a lie so that React does not render it.
Consider a few solutions:
{!!stars && ( <div> {'* '.repeat(stars)} </div> )}
Notice the sign
!! before the
stars - it means double negation. That is, if
stars - 0, then
!! stars are already
false . Namely, this is what we need.
If you don’t really like double denial, here’s another example:
{Boolean(stars) && (
The
Boolean () function
trivially converts a variable to
true or
false .
You can compare the value of a variable with 0 - the result is the same:
{stars > 0 && (
By the way, empty lines are waiting for the fate of numbers. But since the empty string is rendered as '', you will not notice it. If you are a perfectionist, you can treat the strings as numbers in the examples above.
Another solution
When you increase your web application, it will be convenient to split the variables and create one
shouldRenderStars condition. Then you will immediately work with logical values in a logical “AND”:
const shouldRenderStars = stars > 0; return ( <div> {shouldRenderStars && ( <div> {'* '.repeat(stars)} </div> )} </div> );
In the future, when a business requires you to check, say, for authorization, the presence of a dog, preferences in beer, you just need to slightly change
shouldRenderStars , and what is returned will not change:
const shouldRenderStars = stars > 0 && loggedIn && pet === 'dog' && beerPref === 'light`; return ( <div> {shouldRenderStars && ( <div> {'* '.repeat(stars)} </div> )} </div> );
Conclusion
I am one of those who believe that you need to use all the functions of your programming language. For JavaScript, this means the intended use of ternary operators, if / else conditions, and logical “AND” for a simple if replacement.
I hope you found this article helpful. And be sure to write: how do you use the above? Thanks for attention.