Last time we discussed the authorization mechanism for working with the Office 365 API (in particular with the Microsoft Graph API):
- each time you call an API, you must pass token. Token has a limited lifespan
- token issues a Microsoft service, the so-called “Azure AD Authorization Endpoint”
- You can get token without a server using only JavaScript in the browser. For this, Microsoft made the ADAL JS JavaScript library, which simplifies communication with the “Azure AD Authorization Endpoint” to get token.
Then we made a simple static HTML page, on which our vanilla JavaScript made a request to the Microsoft Graph API and displayed a list of letters from Office 365. In this note we will develop an example and do the same on Angular5.

')
We will also talk about the differences between work (school or school account) and personal (personal account) accounts when using the Office 365 API.
An example on
github .
We assume that you are familiar with the
previous article , understand the process of obtaining and using token, have already registered the application in Azure, received the Application ID.
ADAL JS and MSAL JS
Microsoft has two types of accounts:
- Work or school Account
- Personal Account
The work or school account is created (and deleted) by the administrator of the company that purchased the Office 365 subscription. Employees use it, for example, to connect to corporate email, SharePoint, OneDrive.
Personal account you create yourself, is used to connect to personal services, such as personal OneDrive.
In more detail about Work and Personal accounts you can read here “ Understanding Microsoft Work And Personal Accounts ”.
Office 365 API (for example, Microsoft Graph API) has different support for personal and work accounts. For example, both the Work account and Personal account can work with mail, but there is no personal SharePoint (and hence the SharePoint API for the Personal account).
We already know that the token for API calls is issued by “Azure AD Authorization Endpoint”. Authorization points exist 2:
- Authorization endpoint
Issues tokens only for Work Account.
Upon login, the user is sent to
https: //login.microsoftonline.com/common/oauth2/authorize?client_id = ...
- Authorization Endpoint v2.0
Issues tokens for both Work and Personal Account.
Upon login, the user is sent to
https: //login.microsoftonline.com/common/oauth2/v2.0/authorize?
client_id = ...
Why then do I need an “Authorization Endpoint” at all? The peculiarity is that “Authorization Endpoint v2.0” will issue a token only for those API features that exist for both Work and Personal accounts. Those. using Endpoint v2.0 and Work account you will be able to access mail, but there will be no access to the SharePoint API (for details on which APIs support Endpoint v2.0, see the links at the end).
Currently, v2.0 endpoint does not work with applications that are registered in the corporate Azure portal (such registration we did in the last note), you need to use a separate
Microsoft Application Registration Portal .
Microsoft promises to expand the capabilities of v2.0 endpoint in the future, and provide support for applications registered in the corporate Azure portal - v2.0 endpoint will be able to issue tokens for them. In the meantime, for corporate applications, Microsoft recommends using “Authorization Endpoint”:
If you use the v2.0 endpoint
ADAL JS works with “Authorization Endpoint”, MSAL JS with v2.0 endpoint. In this article we will use Authorization Endpoint of the first version and accordingly ADAL JS.
Angular 5 and ADAL JS
We will need the installed Node.js, npm, and
Angular CLI - a utility that helps create and build Angular projects. The whole process is well described in
Angular QuickStart .
Let's make a new project:
ng new angular5-office365-adal-example
There are no official (from Microsoft) TypeScript d.ts descriptions for Adal.js. Several options for using Adal.js in Angular 5:
- Do not use d.ts descriptions, declare the AuthenticationContext object (from Adal.js) as a global any and write untyped code as in regular JavaScript
- Most do d.ts description
- Take advantage of the adal-angular5 project , which is a tracing copy of the original adal-angular.js for AngularJS from Microsoft ( Adal js )
- There are projects in which Adal js is completely rewritten to TypeScript ( adal-ts , adal-typescript )
- Use ready-made d.ts description.
5 option looks the most simple and convenient: using the original Adal.js from Microsoft and using d.ts descriptions add intellisense and checks during assembly.
Install verified d.ts descriptions for
Adal.js. Being in the project folder:
npm install
We have a test case, so let's make it as simple as possible: we will connect to the Microsoft Graph API directly in the AppComponent.
In the app.component.ts file, import HttpClient and adal
import { HttpClient, HttpHeaders } from '@angular/common/http'; import { } from 'adal';
In app.module.ts we will connect HttpClientModule
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { HttpClientModule } from '@angular/common/http'; //<- ADD import { AppComponent } from './app.component'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, HttpClientModule //<- ADD ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
Let's connect js-library to the page
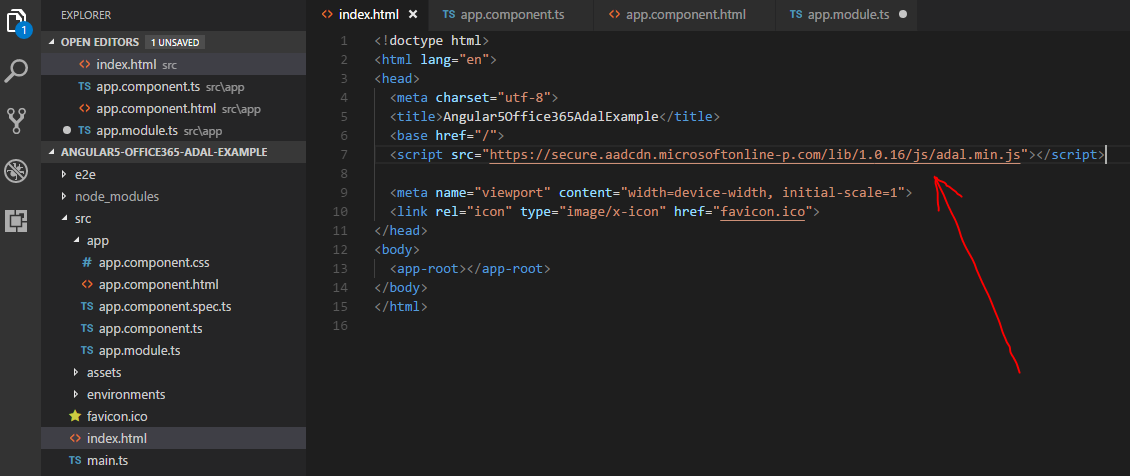
Next will be exactly the same code as in the previous example in JS.
In ngOnInit we will check whether the user is authorized or not, if not authorized or token is rotten, we will send the user to the registration page.
let config: adal.Config = { tenant: 'igtit.onmicrosoft.com', clientId: '21XXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX', postLogoutRedirectUri: window.location.origin, endpoints: { graphApiUri: "https://graph.microsoft.com", }, cacheLocation: "localStorage", redirectUri: '' }; let authContext = new AuthenticationContext(config); let isCallback = authContext.isCallback(window.location.hash); authContext.handleWindowCallback(); if (isCallback && !authContext.getLoginError()) { window.location.href = (<any>authContext)._getItem((<any>authContext).CONSTANTS.STORAGE.LOGIN_REQUEST); } // check if user need to login let user = authContext.getCachedUser(); let token = authContext.getCachedToken(config.clientId); if (!user || !token) { authContext.login(); }
Now you can use token to call Microsoft Graph API
authContext.acquireToken(config.endpoints.graphApiUri, (error, token) => { // call Microsoft Graph API with token let url = config.endpoints.graphApiUri + "/v1.0/me/messages"; this.http.get(url, { headers: { "Authorization": "Bearer " + token } }).subscribe(mgs => this.messages = (<any>mgs).value); });
Full
GitHub example.
Links
Active Directory Authentication Library (ADAL) for JavaScriptMicrosoft Authentication Library Preview for JavaScript (MSAL.js)Should I use the v2.0 endpoint?What's different about the v2.0 endpoint?Understanding Microsoft Work And Personal Accounts