When developing plugins for Jira, you occasionally have to use libraries that are not in Jira, so they need to be installed along with the plugin being developed. In Jira, third-party libraries can be packaged into a plugin in two ways:
Specify the compilation scope of the third-party library, and then the classes from this library will be packed into the jar-file of the plugin being developed. To do this, in the file pom.xml write:
<dependency> <groupId>com.google.code.gson</groupId> <artifactId>gson</artifactId> <version>2.2.2-atlassian-1</version> <scope>compile</scope> </dependency>
Assemble the plugin so that the jar file of the third-party library is located in the dependencies folder of the obr file of the plugin being developed.
We will step by step analyze the packaging of the dependent library in the dependencies folder of the obr-file.
The theory of how to put additional dependencies in obr can be
read here .
')
Consider an example. We have a main library (jira-library) containing the LibraryService service with a single function getLibraryMessage. From another plugin (jira-obr) we will call the getLibraryMessage function. The resulting value will be issued in the jira-obr plugin servlet.
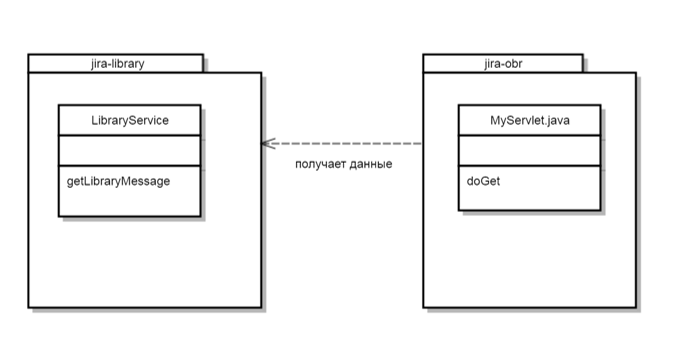
Putting jira-obr in such a way that jira-library.jar is located in the dependencies folder of jira-obr.obr. Installing jira-obr.obr will also install jira-library.jar.
The source code for the
jira-library and
jira-obr plugins can be downloaded here.
Create jira-library plugin
Open the terminal and execute the following command:
atlas-create-jira-plugin-module
As the plugin is created, the terminal will ask questions. Below are the questions and answers to them:
Define value for groupId: : ru.matveev.alexey.tutorial.library Define value for artifactId: : jira-library Define value for version: 1.0.0-SNAPSHOT: : Define value for package: ru.matveev.alexey.tutorial.library: : Y: : Y
After creating the plugin, you need to add the LibraryService service. To do this, we first create an interface (LibraryService.java) in the ru.matveev.alexey.tutorial.library.api package
package ru.matveev.alexey.tutorial.library.api; public interface LibraryService { String getLibraryMessage(); }
Then we create the implementation of the LibraryService interface (LibraryServiceImpl.java) in the ru.matveev.alexey.tutorial.library.impl package:
package ru.matveev.alexey.tutorial.library.impl; import com.atlassian.plugin.spring.scanner.annotation.export.ExportAsService; import ru.matveev.alexey.tutorial.library.api.LibraryService; import javax.inject.Named; @ExportAsService({LibraryService.class}) @Named public class LibraryServiceImpl implements LibraryService { public String getLibraryMessage() { return "jira-library test message"; } }
The @ExportAsService annotation is needed to make the service available for other plug-ins, which will allow the jira-obr plugin to access it.
We assemble the plugin:
altas-mvn package
After the build is completed, you can see the assembled jar file in the jira-library / target folder.
Create jira-obr plugin
We execute the following command in the terminal:
atlas-create-jira-plugin
Answer the questions as follows:
Define value for groupId: : ru.matveev.alexey.tutorial.obr Define value for artifactId: : jira-obr Define value for version: 1.0.0-SNAPSHOT: : Define value for package: ru.matveev.alexey.tutorial.obr: : Y: : Y
Then go to the jira-obr folder and create the servlet with this command:
atlas-create-jira-plugin-module
We answer questions like this:
Choose a number (1/2/3/4/5/6/7/8/9/10/11/12/13/14/15/16/17/18/19/20/21/22/23/24/25/26/27/28/29/30/31/32/33/34): 21 Enter New Classname MyServlet: : Enter Package Name ru.matveev.alexey.tutorial.obr.servlet: : Show Advanced Setup? (Y/y/N/n) N: : N Add Another Plugin Module? (Y/y/N/n) N: : N
Then add the dependency for the jira-library plugin to the pom.xml file:
<dependency> <groupId>ru.matveev.alexey.tutorial.library</groupId> <artifactId>jira-library</artifactId> <version>1.0.0-SNAPSHOT</version> <scope>system</scope> <systemPath>/home/alexm/atlasplugin/obr/jira-library/target/jira-library-1.0.0-SNAPSHOT.jar</systemPath> </dependency>
You must change /home/alexm/atlasplugin/obr/jira-library/target/jira-library-1.0.0-SNAPSHOT.jar to the path that jira-library-1.0.0-SNAPSHOT.jar is located.
In a real environment, the jira-library-1.0.0-SNAPSHOT.jar file should be in an Artifactory, Nexus Repository Manager, or other similar program. In this case, the file is in the file system to simplify the example.
Next, in the pom.xml for the maven-jira-plugin, add the following lines to the configuration tag:
<pluginDependencies> <pluginDependency> <groupId>ru.matveev.alexey.tutorial.library</groupId> <artifactId>jira-library</artifactId> </pluginDependency> </pluginDependencies>
and add to the Import-Package tag:
ru.matveev.alexey.tutorial.library.api,
The settings are needed for the automatic packaging of the jira-library-1.0.0-SNAPSHOT.jar in the dependencies folder of the jira-obr.obr file, as well as the formation of the jira-obr plug-in dependency on the jira-library plugin.
Next, change the contents of MyServlet.java:
package ru.matveev.alexey.tutorial.obr.servlet; import com.atlassian.plugin.spring.scanner.annotation.imports.ComponentImport; import org.slf4j.Logger; import org.slf4j.LoggerFactory; import ru.matveev.alexey.tutorial.library.api.LibraryService; import javax.inject.Inject; import javax.inject.Named; import javax.servlet.*; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.IOException; @Named public class MyServlet extends HttpServlet{ private static final Logger log = LoggerFactory.getLogger(MyServlet.class); private final LibraryService libraryService; @Inject public MyServlet(@ComponentImport LibraryService libraryService) { this.libraryService = libraryService; } @Override protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { resp.setContentType("text/html"); resp.getWriter().write("<html><body>" + libraryService.getLibraryMessage() + "</body></html>"); } }
Everything. Now we can run Jira with this command:
atlas-run
Jira will be running on localhost: 2990 / jira. You need to log in to Jira as the admin: admin account, go to Add-ons -> Manage add-ons and install the jira-obr plugin by selecting the jira-obr.obr file. After this, you will see jira-library and jira-obr among the installed plugins. It will look something like this:
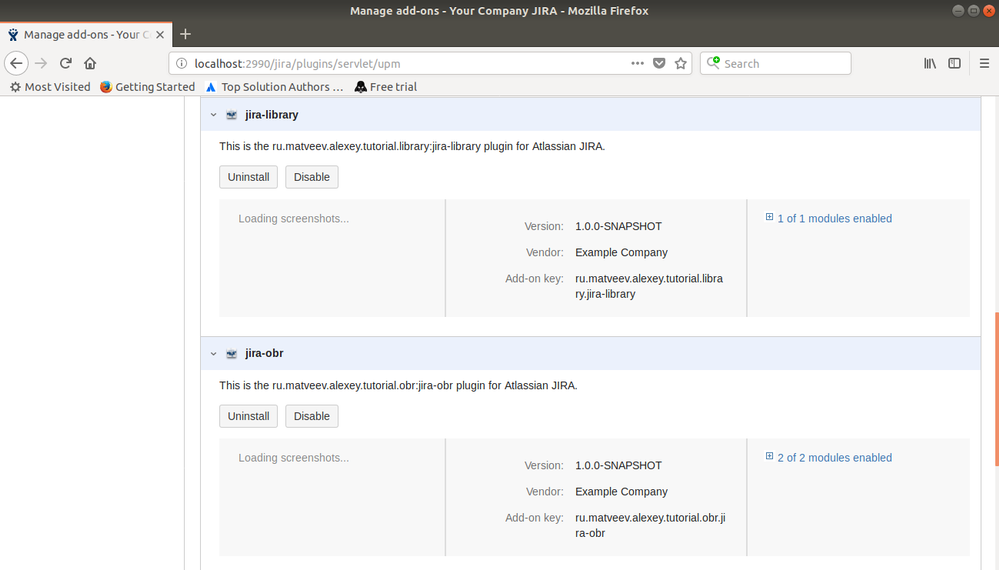
Next, you need to start the servlet at the address
localhost : 2990 / jira / plugins / servlet / myservlet
A message from the jira-library plugin will appear in the browser:

Done: we packed the jira-library.jar file into jira-obr.obr and were able to successfully call the getLibraryMessage function.