Introduction
As soon as the ban on anonymity in messengers came into effect on the territory of the Russian Federation, I got around to write a post about a
telegram bot . In the course of creating the bot, I ran into a large number of problems that had to be solved individually, and literally wiped out grains of information from all over the Internet. And after several months of suffering and torment (coding is not my main occupation), I finally finished with the bot, figured out all the problems and am ready to tell my story to you.

The first steps
First you need to install a
telegram on your PC and register with the messenger. Find in search @BotFather is the father of all bots in the telegram, it is he who creates them. We write him / newbot and answer two simple questions: the name of the bot and its username. After that, @BotFather congratulates us on the successful creation of the bot and sends it token to us - 523870826: AAF0O8T-e7riRi8m6qlRz4pBKKdh0OfHKj8.

')
Attention: token is the only identification key to the bot. Do not post it anywhere, otherwise other people will be able to control your bot. The bot with this token at the time of posting the article was deleted.What programming language to choose to write a bot?
Then I did not bother for a long time and stopped at Python, because I know it well enough, and the convenient library is also present. I decided to use the
PyTelagramBotAPI (at the time of this writing, the latest available version is 3.5.1).
Let's go to the first code.
Import the PyTelegramBotAPI library.
Create a bot.
bot = telebot.TeleBot("523870826:AAF0O8T-e7riRi8m6qlRz4pBKKdh0OfHKj8")
Let's write a simple message handling using the
bot .message_handler decorator.
@bot.message_handler(content_types=["text"]) def handle_text(message): if message.text == "Hi": bot.send_message(message.from_user.id, "Hello! I am HabrahabrExampleBot. How can i help you?") elif message.text == "How are you?" or message.text == "How are u?": bot.send_message(message.from_user.id, "I'm fine, thanks. And you?") else: bot.send_message(message.from_user.id, "Sorry, i dont understand you.")
Put the bot in the mode of continuous processing of information coming from the telegram servers.
bot.polling(none_stop=True, interval=0)
In the variable message telegram passes the dictionary (map) of the form:
{'content_type': 'text', 'message_id': 1, 'from_user': { 'id': None, 'is_bot': False, 'first_name': None, 'username': None, 'last_name': None, 'language_code': None}, 'date': None, 'chat': { 'type': 'private', 'last_name': None, 'first_name': None, 'username': None, 'id': None, 'title': None, 'all_members_are_administrators': None, 'photo': None, 'description': None, 'invite_link': None, 'pinned_message': None, 'sticker_set_name': None, 'can_set_sticker_set': None}, 'forward_from_chat': None, 'forward_from': None, 'forward_date': None, 'reply_to_message': None, 'edit_date': None, 'author_signature': None, 'text': '/start', 'entities': '[<telebot.types.MessageEntity object at 0x037CB6B0>]', 'caption_entities': None, 'audio': None, 'document': None, 'photo': None, 'sticker': None, 'video': None, 'video_note': None, 'voice': None, 'caption': None, 'contact': None, 'location': None, 'venue': None, 'new_chat_member': None, 'new_chat_members': None, 'left_chat_member': None, 'new_chat_title': None, 'new_chat_photo': None, 'delete_chat_photo': None, 'group_chat_created': None, 'supergroup_chat_created': None, 'channel_chat_created': None, 'migrate_to_chat_id': None, 'migrate_from_chat_id': None, 'pinned_message': None, 'invoice': None, 'successful_payment': None}
There are also other decorators who can receive audio files, videos, pictures, documents, geolocation, etc.
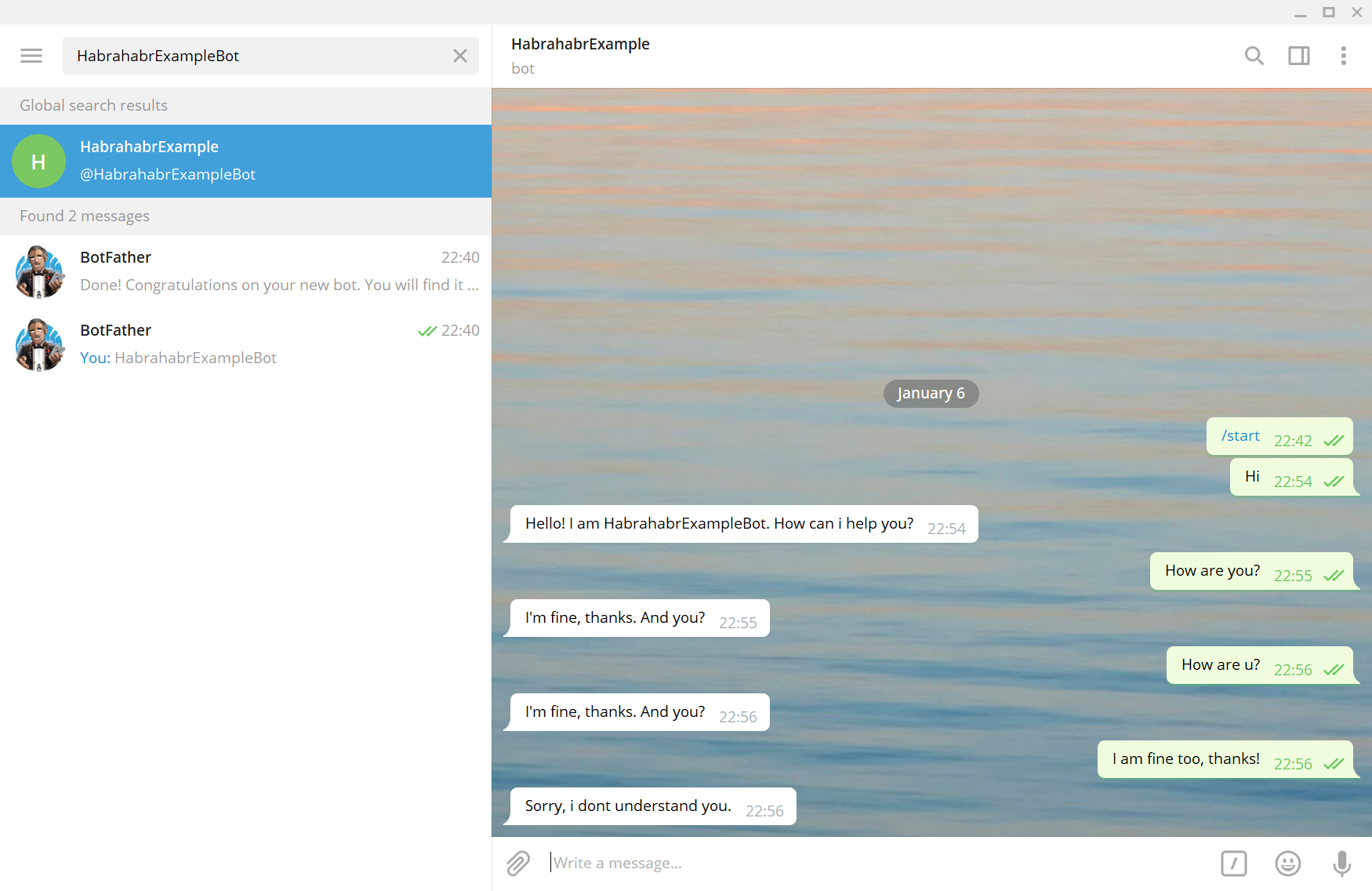
In general, the telegram allows bots to perform a lot of cool operations from creating custom keyboards to making payments.
Link to the official Telegram documentation.To save data about users decided to use the
sqlite3 database.
import sqlite3 connection = sqlite3.connect("database", check_same_thread = True) cursor = connection.cursor() cursor.execute("CREATE TABLE IF NOT EXISTS Inventory_on (ID INT, 'Primary weapon' TEXT, 'Secondary weapon' TEXT)") cursor.execute("CREATE TABLE IF NOT EXISTS Clans (Name TEXT, Points INT)") cursor.execute("CREATE TABLE IF NOT EXISTS WorkStatus (ID INT, Status INT)") connection.commit() connection.close()
I started parallel processes using the threading library. For example: the function of calculating the battles.
import threading threading.Thread(target=name_of_your_function).start()
Then everything depends on your imagination.
Where to run your bot?
I don’t want to leave my own PC on 24/7, and it’s not practical. Therefore, I decided to use the free heroku service, but I suffered a failure due to the database I selected. It turned out that every time the bot is restarted, heroku deletes all sqlite3 commits for the last session without exceptions. After that, I decided to buy
VDS (Virtual Dedicated Server, Virtual Dedicated Server) - a remote PC on which a certain amount of power and memory is allocated for you, and to the command line of which you are given access. Most often, the operating system of such a machine is linux. The fee is small - 400 rubles per month, so without much moral suffering I paid for VDS based on
Debian GNU / Linux and began to figure out how to enable the bot on a remote server.
How to connect to VDS?
There are different methods, I decided on an SSH connection via Putty. Download Putty through the
official website and open it. Enter the IP address of the VDS and press open.

This should open a window where you need to enter the username and password from the server.
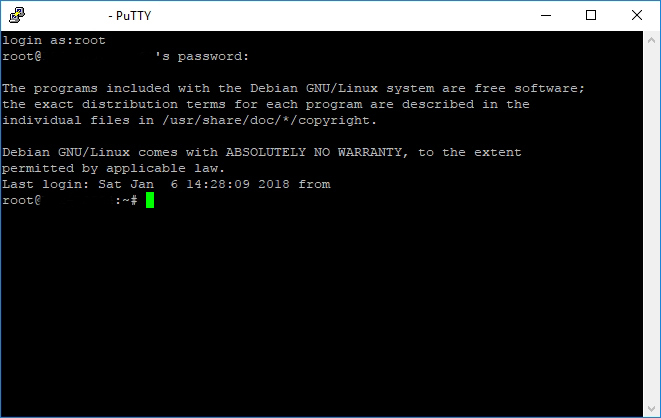
All of the above data will give the company from which you purchase VDS. Next VDS - server.
How to install all the programming languages ​​and libraries you need on the server?
It's simple. By entering these 5 commands into the server console in this sequence, you install python3, setuptools, pip3, and the pyTelegramBotAPI library on the server.
apt-get update apt-get install python3 apt-get install python3-setuptools apt-get install python3-pip pip3 install pyTelegramBotAPI
All additional libraries that are not included in the main python3 package should also be installed according to the principle.
pip3 install 'name_of_site_package'
How to download files from my PC to the server?
To begin, create a folder in which we will upload all the necessary files. On the server, go to the / usr / local / bin directory and create a bot folder.
cd /usr/local/bin mkdir bot
I have windows installed on my PC, respectively, and the commands will be for the
windows command line . First you need to go to the directory where putty.exe is located.
cd /program files/putty
Next, we load bot.py, which is located in the C: \ Users \ Ilya \ PycharmProjects \ Bot directory (you need to substitute your directory) into the directory on the / usr / local / bin / bot server.
pscp.exe "C:\Users\Ilya\PycharmProjects\Bot\bot.py" root@123.123.12.12:/usr/local/bin/bot
Line root@123.123.12.12 should be replaced with a line of the form login @ IP_address, respectively, with your login and IP address (mentioned above in the section “How to connect to VDS?”). Replacing bot.py with the names of other files, download all the necessary ones.
How to download files from server to PC?
Just as when uploading files to the server on the command line to the directory where putty.exe is located. And enter this command to download the database file to the desktop of your PC.
pscp.exe root@123.123.12.12:/usr/local/bin/bot/database "C:\Users\Ilya\Desktop
How to run the bot?
The first and easiest option is to go into the directory with executable files and set up python3 bot.py, but then when you close putty, the bot will turn off.
The second option is to launch the bot using
screen - a module that creates parallel desktops, but then the bot will not restart automatically in the event of a crash, and this happens often - several times a week due to the night restart of the telegram servers (at 3:00 AM MSC).
The third method is
systemd — the system manager, a daemon for initializing other daemons on Linux. Simply put, systemd will launch the bot and restart it in case of a crash.
Install systemd:
apt-get install systemd
Create a file on your PC with the name bot.service with the following content:
[Unit] Description=Telegram bot 'Town Wars' After=syslog.target After=network.target [Service] Type=simple User=root WorkingDirectory=/usr/local/bin/bot ExecStart=/usr/bin/python3 /usr/local/bin/bot/bot.py RestartSec=10 Restart=always [Install] WantedBy=multi-user.target
And load it into the desired directory:
pscp.exe "C:\Users\Ilya\PycharmProjects\Bot\bot.service" root@123.123.12.12:/etc/systemd/system
Next, you need to register 4 commands in the server console:
systemctl daemon-reload systemctl enable bot systemctl start bot systemctl status bot
In my case, due to certain implementation errors, and specifically multithreading, I had to transfer the function for calculating the battles (battle_counter.py) into a separate daemon.
[Unit] Description=Battle counter for telegram bot 'Town Wars' After=syslog.target After=network.target [Service] Type=simple User=root WorkingDirectory=/usr/local/bin/bot ExecStart=/usr/bin/python3 /usr/local/bin/bot/battle_counter.py RestartSec=10 Restart=always [Install] WantedBy=multi-user.target
After that, a message should appear about this content:
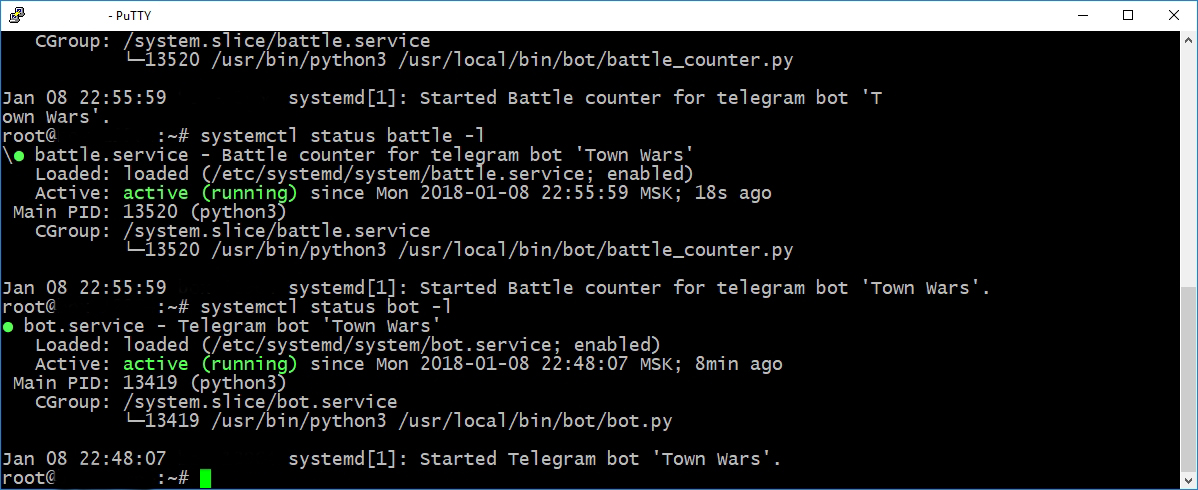
Your bot is up and ready to go!
ACKNOWLEDGMENTS
This was my first relatively large project and I ran into a huge number of new problems for me. I want to express my gratitude to
Yurii Drake , who helped me deal with them!
LINKS
TelegramTelegram botOfficial telegram documentationInformal documentationPyTelagramBotAPIVdsDebian GNU / LinuxPuttyWindows Command PromptMac Command PromptScreenSystemd