Now, including on our website and in the ivi applications, there is a clear tendency for the audience to grow on mobile devices. The share of purchases made on the Internet from mobile devices is also growing. Unlike the desktop, in addition to traditional payment options, mobile payment systems are available, such as
Apple Pay ,
Android Pay ,
Samsung Pay . These systems can be used not only for payment in terminals that accept bank cards, but also for payment in applications and on websites.
We started the introduction of mobile payments from the web version of ivi and chose a mobile website and Apple Pay.
Apple Pay is
Apple's mobile payment system that allows mobile devices to make payments in stores and on the Internet. The user binds the card to the phone, and then when you pay, you only need to confirm payment with a fingerprint or a digital code.
In this article I will talk about using the Apple Pay JS library.
')
The library is designed to use Apple Pay on sites. Apple Pay JS API is supported on:
- iOS from version 10 on Safari and iPhone models of contactless payments (SE and older 6);
- macOS from version 10.12 in Safari browser on computers with Touch ID or when iPhone or Apple Watch is connected to confirm payments.
In order to accept payments through Apple Pay on the site, you will need:
- Developer account Apple;
- HTTPS on a page using Apple Pay;
- Choose a payment gateway with which you will work. We use Payture services.
Next, you need to register a Merchant ID, create a Merchant Identity Certificate and Payment Processing Certificate and verify the domains on which Apple Pay will be used. The process description is in the
instruction from Payture.
- Merchant ID - the merchant ID that represents it on Apple Pay;
- Payment Processing Certificate - a certificate used to transfer payment data on the payment gateway side. Apple Pay servers use the public key of this certificate to encrypt billing information. The private key is used to decrypt data when making a payment;
- Merchant Identity Certificate - TLS certificate used to verify merchant data and authorize payment sessions through an Apple server. The billing session is created when the payment process is initiated. The certificate is used only on the site side.
It is worth paying attention to the fact that during verification you need to specify the full domain name, i.e. domain mask can not be specified.
After all the preparatory work, you can begin to integrate Apple Pay on the site. The integration process consists of 3 main parts:
- Creating a payment session, showing the payment dialog and processing the events of the payment session. Apple Pay JS API is used here;
- Verification of the payment session. Required for Apple Pay to make sure the request comes from a registered merchant. It is implemented on the backend;
- Payment through the payment gateway and the end of the payment session.
Creating a billing session
Before showing the button to pay through Apple Pay, you need to check if Apple Pay is available on the device. It is implemented like this:
if (window.ApplePaySession) {
Next, using Apple Pay JS API, you can check whether the user has active cards associated with Apple Pay. 2 methods
canMakePayments
and
canMakePaymentsWithActiveCard
. The first one checks only the fact of support for Apple Pay, the second one in addition allows you to find out if there is at least 1 card tied to Apple Pay.
We have, for example, these checks are used to decide to show the Apple Pay button over or under other payment methods. In case there are no added cards, the Set Up Apple Pay button is displayed, the settings of the phone with the Wallet section open at the click. As a result, the user leaves the site, and the payment process should not be interrupted, so the button is located under the main types of payment and is not even visible without the screen scrolling.
Payment pages for customized and unconfigured Apple Pay:

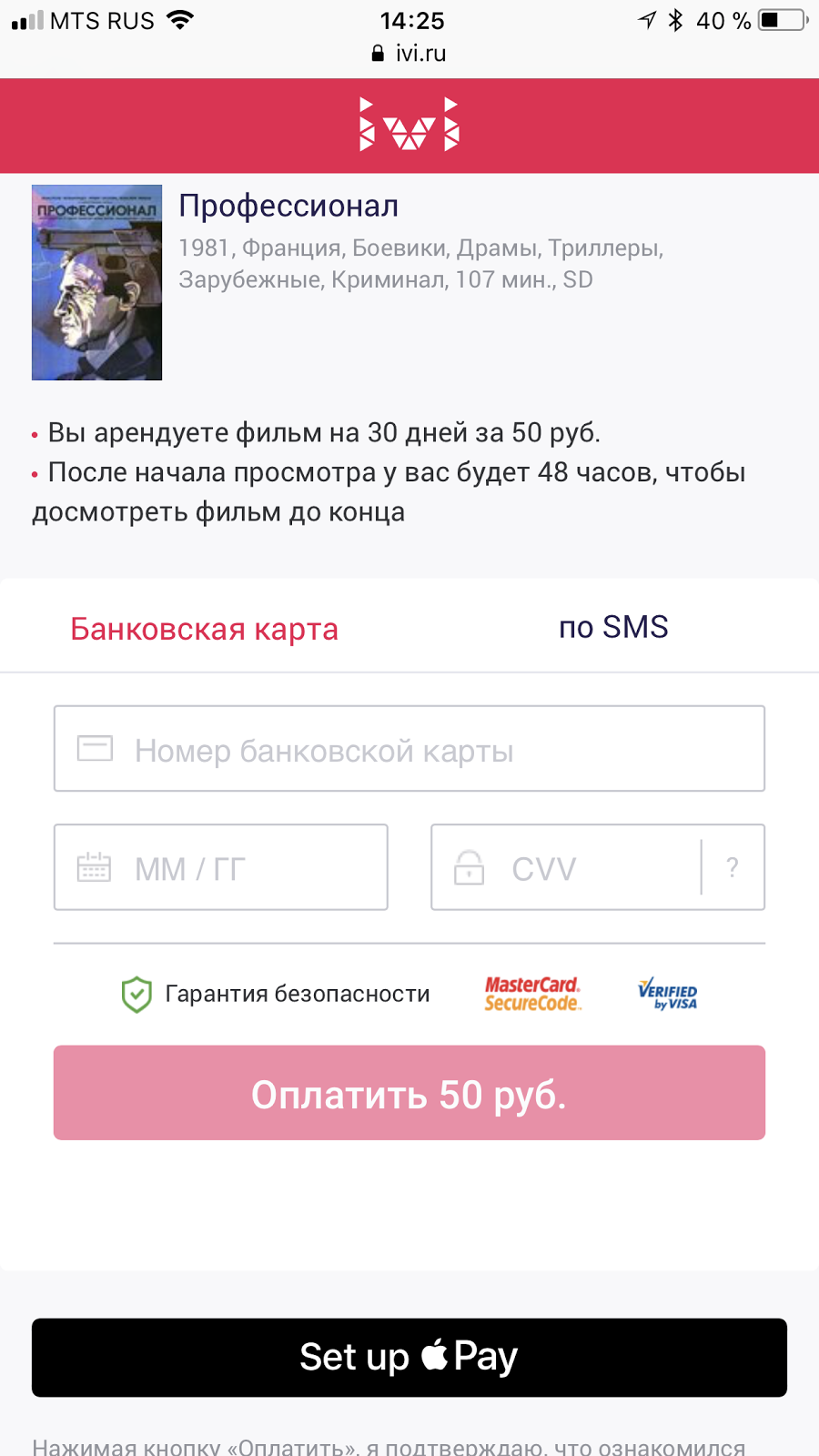
Once the button is displayed on the page, you can create a billing session to display the payment dialog. A session can only be created by an explicit user action. By clicking on the button you need to run the following code:
const paymentRequest = { total: { label: 'ivi.ru', amount: 50 }, countryCode: 'RU', currencyCode: 'RUB', merchantCapabilities: ['supports3DS'], supportedNetworks: ['masterCard', 'visa'] }; const applePaySession = new window.ApplePaySession(1, paymentRequest);
The example shows the minimum set of properties for an
ApplePayPaymentRequest object. To display more detailed information about the purchase you need to use other properties of this object.
To handle payment session events, you need to implement at least the following methods:
onvalidatemerchant
triggered when you open the Apple Pay payment form. In the event handler, you need to verify the billing session. The verification process is described below;
onpaymentauthorized
triggered when a user confirms a payment on an Apple Pay payment form using a Touch ID, Face ID, or code. Here a payment token is available that needs to be transferred to the payment gateway for making a payment
After creating the
ApplePaySession
object, call the begin method to display the payment dialog:
applePaySession.begin();
As a result, the user will see the following:
When the form is
onvalidatemerchant
event is
onvalidatemerchant
. In order to continue the payment we will implement the next stage.
Verification of the payment session
In the
onvalidatemerchant
event
onvalidatemerchant
, the
validationURL
field comes. To this address from the backend you need to send data signed with the Merchant Identity Certificate.
applePaySession.onvalidatemerchant = (event) => { // performValidation(event.validationURL) .done( (merchantSession) => { // this.applePaySession.completeMerchantValidation(merchantSession); } ).fail( () => { this.applePaySession.abort(); // .. } ); };
The
performValidation
method returns a promise from a validation request. The handler for this request is on our side, the implementation is as follows: a post request is sent to the URL from the onvalidatemerchant event parameter.
The request body contains json:
{ "merchantIdentifier": "MERCHANT_IDENTIFIER", "domainName": "HOST", "displayName": "DISPLAY_NAME" }
The MERCHANT_IDENTIFIER and DISPLAY_NAME parameters are taken from the development account (they were set up at the very beginning), and HOST is the domain from which the payment is made.
The request must be signed with a Merchant Identity Certificate. The answer will come json, and it must be returned. After receiving this response, call the
completeMerchantValidation
method from the payment session.
The session verification process has been completed, now the user is required to confirm the payment with a finger or code. After that, the
onpaymentauthorized
event is
onpaymentauthorized
. Go to the stage of the payment.
Making payment
The
onpaymentauthorized
event
onpaymentauthorized
contains an object with a payment token that needs to be
onpaymentauthorized
to the payment gateway. All information contained in the token is described in the
documentation .
As soon as the payment is made, we complete the payment on the client side.
applePaySession.completePayment(success);
in success you need to transfer one of 2 statuses
window.ApplePaySession.STATUS_SUCCESS
or
window.ApplePaySession.STATUS_FAILURE
.
This process of paying through Apple Pay ends. More details about Apple Pay JS can be found in the
official documentation .