Hey. I am 17 years old and I am a JS developer. Perhaps this is a sentence, and maybe this is a classic greeting in the "Club of anonymous
JS- programmers" - I do not know. Now, in many ways, my job is to work with data, its processing, filters, sorting, and so on. Naturally, I use non-native JS in projects. Today we will do filters on pure js-e. See how cool and fast this is. We recognize the possibilities of es6 and refactor the code. Interested please under the cat.
Isn't pure js cool?
Now, and in principle for a long time already, very few people write on pure js. Everyone wants to write on modern frameworks. I am no exception and at work I mainly write on VueJS (2.0+) and old AngularJS. And this choice is fully justified. I will not go into details, but in most cases, if you build an application properly on a framework, you will win in speed, maintainability and readability.
So why this article?
I want to tell that you should not forget about pure js-e. After all, we all started with a simple:
console.log('Hello world');
You will not write on the framework is? Although, perhaps, I am very bad with you. In this article I want to write filters on pure js. The task, in principle, is trivial and does not require any advances of genius. I will try to write as much as possible the compressed code. I do not guarantee that the reader who is not familiar with the features of js will be understandable. However, I will try to describe the whole process in as much detail as possible.
')
Let's start
For a start, roughly outline what we need and how it will work.
We need data. Let there be a static array. Go.
let list = ['JavaScript','Kotlin','Rust','PHP','Ruby','Java','MarkDown','Python','C++','Fortran','Assembler']
(I apologize in advance if your favorite language is not specified)
Great, we have the data. In fact, this data should be obtained through requests to the server, but we, like the LLC, have a simplified model.
For output, I made an input and list. It looks like this:
<input type="text" id="search"> <ul id="results"> </ul>
In input, the user enters a request, and the list will be populated depending on the request. And we also define that if input is empty, then we will output the entire list.
We have the data and the user interface (you can laugh). Now we need a function that will render us a list.
function renderList(_list=[],el=document.body){ _list.forEach(i=>{ let new_el = document.createElement('li') new_el.innerHTML=i el.appendChild(new_el) }) }
It turned out like this. The function takes two arguments — an array with elements and a DOM tree element to which we want to draw a list. If the first argument is not specified, then we tell the function that this is an empty array. Similarly with the tree element.
Next, we loop through all the elements of the array in a loop and for each element we create a DOM tree node, add the element itself inside this node and add it to the end of our parent element. In principle, nothing complicated.
At this stage, the code looks like this:
let list = ['JavaScript','Kotlin','Rust','PHP','Ruby','Java','MarkDown','Python','C++','Fortran','Assembler'] const result = document.getElementById('results') renderList(list,result) function renderList(_list=[],el=document.body){ _list.forEach(i=>{ let new_el = document.createElement('li') new_el.innerHTML=i el.appendChild(new_el) }) }
And the application itself is like this:
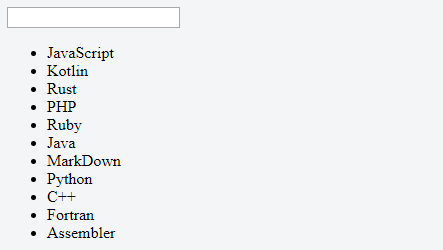
It's time to do the most interesting. We start to filter. We need a function that takes a substring (user request) and an array of all elements as input, and outputs an array of filtered elements as output. Let's get started The most obvious option is this:
function filter(val,list){ let result=[]; list.forEach(i=>{ if(i.indexOf(val)!=-1) result.push(i) }) return result; }
We have a draft. Now connect all together, add a handler and go. The code is now:
let list = ['JavaScript','Kotlin','Rust','PHP','Ruby','Java','MarkDown','Python','C++','Fortran','Assembler'] const result = document.getElementById('results') renderList(list,result) function filter(val,list){ let result=[]; list.forEach(i=>{ if(i.indexOf(val)!=-1) result.push(i) }) return result; } function renderList(_list=[],el=document.body){ _list.forEach(i=>{ let new_el = document.createElement('li') new_el.innerHTML=i el.appendChild(new_el) }) } document.getElementById('search').addEventListener('input',e=>{ let new_arr = filter(e.target.value,list) renderList(new_arr,result) })
And the browser shows us this:

Oops. Forgotten to clear the parent element before rendering. Add this to the render function:
el.innerHTML='';
Now everything works for us. Approximately also filters on your favorite framework work. But we are good programmers and we want to make everything more beautiful, and maybe more beautiful. Fortunately, I am not a philologist. Let's return to our filter function and try to rewrite it.
First, let's replace if with a ternary operator:
(i.indexOf(val)!=-1)?result.push(i):'';
Having rummaged in documentation, I found that it is possible to use bitwise negation. It works according to the formula - (N + 1). That is, with our value of -1 in indexOf, we get the formula - (- 1 + 1) = 0. That is, it is already finished false for our condition. Rewrite:
(~i.indexOf(val))?result.push(i):'';
And now dessert. forEach is no longer fashionable. There is ES6. There is a method to iterate through the array - filter. It returns a value if it matches the condition. Apply it:
function filter(val,list){ console.time('test') return list.filter(i=>(~i.indexOf(val))) };
Beautiful, isn't it?
Now we redo the handler. Let's write everything in one switch function. I did it like this:
document.getElementById('search').addEventListener('input',e=>renderList(filter(e.target.value,list),result))
I hope everything is clear. Take the filtered array and throw it as an argument for the render.
I wanted to measure the processing time. Used fashionable features.
I stopped at this. The complete and working code is as follows:
let list = ['JavaScript','Kotlin','Rust','PHP','Ruby','Java','MarkDown','Python','C++','Fortran','Assembler'] const result = document.getElementById('results') renderList(list,result) function filter(val,list){ console.time('test') return list.filter(i=>(~i.indexOf(val))) }; function renderList(_list=[],el=document.body){ el.innerHTML=''; _list.forEach(i=>{ let new_el = document.createElement('li') new_el.innerHTML=i el.appendChild(new_el) }) console.timeEnd('test') } document.getElementById('search').addEventListener('input',e=>renderList(filter(e.target.value,list),result))

Such an application was very quick and easy to understand. The average time for such data is 0.3 milliseconds. And the size after compression through babel gives only 782 bytes.

A working example can be found at the
link.With this article I just wanted to push people to not forget about pure js. Not so bad there. Use native functions and use es6.
Thanks
Houston Now the example looks like
this and is not case sensitive.
Thanks for attention.