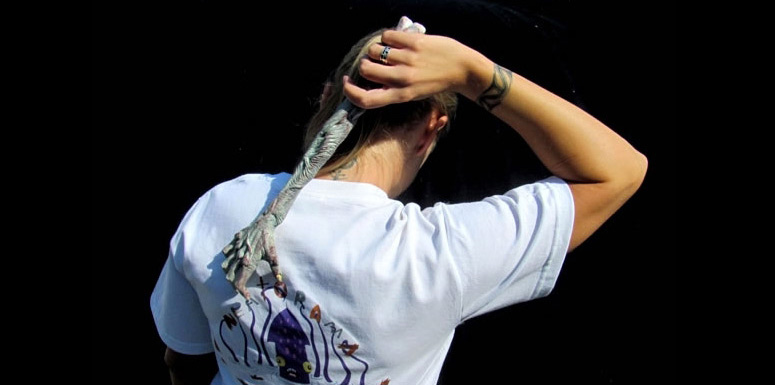
Gone are the days when, during the development process, I had to spend time switching between terminal, browser and editor. Now everything is done differently - faster and more convenient. Today I will talk about optimizing the daily affairs of a React-developer. Namely, it will be discussed how to connect Visual Studio Code and Google Chrome. This will make it possible to debug the browser code in the editor.
Debugging Tools VS Code and jest from FacebookTest project setup
Before we begin to learn how to debug React in VS Code, we will create an educational application with which we will experiment. I often use the
create-react-app , because I really don't like to manually create empty projects. Therefore, I propose to use it in this guide. Alternatively, if you already have an application, you can use it.
')
So, let's create a test project. To do this, do the following:
- Install the
create-react-app
globally by running the npm i -g create-react-app
.
- After installation, create a project with the
create-react-app vscode-tutorial
.
As a result, you should have a new folder with a React-application.
Configure VS Code
Now we’ll install the VS Code extension, which will allow the editor to interact with Chrome. VS Code connects to Chome using
remote debugging protocol . This is the same protocol that Chrome’s developer tools use. But thanks to this approach, instead of working with standard Chrome tools, you can use VS Code to debug browser code.
Installing the Debugger for Chrome extension
So, in order to establish interaction between VS Code and Chrome, you need to install an extension called
Debugger for Chrome
. To install it, go to the extensions panel and search by extension name. The result should be something like this:
Finding the Debugger for Chrome extensionConnect VS Code to Chrome
Next, you need to configure VS Code to connect to Chrome. This is done like this:
- Click the debug icon.
- Open the drop-down menu (near the
Play
button) and select Add Configuration…
- In the
Select Environment
drop-down list, select Chrome
.
If you mess up, this is how it all looks.
Configuring VS Code and Chrome communicationA
.vscode
folder will be automatically added to the project root. It will contain the
launch.json
file, which is used to configure the VS Code debugger for the current project. Every time you create a new project, you need to perform the same sequence of actions to set up remote debugging (you can also just copy the
.vscode
folder from one project to another).
Modify the
url
property in this file so that it points to the server used during development. For
create-react-app
this is
http://localhost:3000
. As a result, your
launch.json
should look like this (thanks to
Kenneth Auchenberg from the VS Code team for the advice on working with this file):
{ "version": "0.2.0", "configurations": [ { "name": "Chrome", "type": "chrome", "request": "launch", "url": "http://localhost:3000", "webRoot": "${workspaceRoot}" } ] }
A complete list of configuration options can be found
here .
Use debugger
Now almost everything is ready for work. The next step is to use a test project in order to test the debugger.
Running debugger
You can start the debugger by doing one of the following:
- Press
F5
.
- Click on the green
Play
button on the debug panel.
- Select the menu command
Debug → Start Debugger
.
If the debugger is successfully launched, you will see a small toolbar appear at the top of the VS Code window:
The toolbar that appears when you turn on the debuggerSetting a breakpoint
Breakpoints are used to inform the debugger that it needs to suspend code execution in a specific place. This allows the programmer to explore the variables, the call stack and make changes to the code during the execution of the application.
Set a breakpoint in the test application. Open the
src/App.js
and click on the field to the left of line
11
. A red dot should appear, indicating that a breakpoint has been added. Here, to make it clear, an animated version of this instruction:
Setting a breakpointStarting the development server
Finally, in order to see how it all works, you need to start the development server by running the
npm start
command in the terminal. This command will launch a new server available at
http://localhost:3000/
.
Server startupGo to
http://localhost:3000/
and you will see the page freeze. This is due to the breakpoint being triggered. If you go to VS Code, you will notice that line
11
will be highlighted, and information about the call stack will appear in the debug panel.
Tripped breakpointIf everything worked for you - congratulations! You have just learned how to set up remote debugging in VS Code. If you want to know the details about the debugging process in VS Code - read
this .
Continuous testing with jest
It’s convenient for me to run unit tests in the process of working on the code. Namely, that they are called whenever I make changes to the program. Thanks to
create-react-app
everything you need to implement such a script is automatically configured. To run
jest
, just enter the
npm test
command in the terminal. Due to this, the system will monitor the files and automatically run tests when they are saved. It looks like this:
Continuous Testing in VS CodeResults
In this article, we talked about how to configure VS Code and Chrome interaction to organize remote debugging of React-applications. We hope this simple technique will help save some time, if earlier you had to constantly switch between the editor, browser and terminal.
Dear readers! Do you use any useful little things that increase productivity in the development of web applications?