From translator
Greetings We continue to translate
documentation for the cocos2d-x engine. This article contains two chapters at once, small in size. As you have already guessed, in the first we will analyze the ways of working with scenes, and at the end we will consider several more types of nodes.
I hope you find a lot of useful things for yourself!
What is a scene?
The
Scene object is a container containing sprites, labels, nodes, and other objects needed by your game.
Scene is responsible for running the game logic and rendering the content in each frame. You will need at least one scene to start the game. Think of your game as a movie. When
Scene is running, users see what is happening in real time. There can be any number of scenes in your game and you can easily switch between them. Cocos2d-x is capable of transitions between scenes and you can even do it with cool effects.
Scene creation
It is very simple to create a scene:
')
auto myScene = Scene::create();
Remember the scene graph?
In the
first part of the tutorial, we learned about
Scene Graph and how it affects the design of our game. It is important to remember that it determines the order in which the
GUI (graphical user interface) elements are drawn. Also remember about the z-order!
Simple scene
Let's build a simple scene. Remember that Cocos2d-x uses the
right Cartesian coordinate system . This means that the coordinate
0,0 is in the lower left corner of the screen / display. To correctly position the elements of your game, you must calculate everything in advance. Let's create a simple scene and add a few elements to it:
auto dirs = Director::getInstance(); Size visibleSize = dirs->getVisibleSize(); auto myScene = Scene::create(); auto label1 = Label::createWithTTF("My Game", "Marker Felt.ttf", 36); label1->setPosition(Vec2(visibleSize.width / 2, visibleSize.height / 2)); myScene->addChild(label1); auto sprite1 = Sprite::create("mysprite.png"); sprite1->setPosition(Vec2(100, 100)); myScene->addChild(sprite1);
If we run this code, we should see a simple scene containing a
label and a
sprite . This is a bit, but only for now.
Transition between scenes
You may need the ability to move between scenes in your game. Probably at the beginning of a new game, changing the level or at its completion. Cocos2d-x provides a number of ways to transition between scenes.
Ways to replace the scene
There are many ways to change your scenes. Each has a certain functionality. Let's go over them.
Given: auto myScene = Scene::create();
runWithScene () - used only for the first scene. With this method we set the scene for the start of the game.
Director::getInstance()->runWithScene(myScene);
replaceScene () is an immediate scene replacement.
Director::getInstance()->replaceScene(myScene);
pushScene () - suspends the execution of the current scene, adding it to the stack of suspended scenes. Call only when there is an active scene.
Director::getInstance()->pushScene(myScene);
popScene () - this scene will be replaced by the active one. The current scene will be deleted. Call only when there is an active scene.
Director::getInstance()->popScene(myScene);
Scene change with effects
You can add visual effects to change your scenes.
auto myScene = Scene::create();
Other types of nodes
You are already using
Sprite ,
Label and
Action - objects in your game and this is progress. In addition to the basic node types described in previous chapters, Cocos2d-x provides more advanced node types with special functionality. Maybe you want to make a game
based on tiles ? Or maybe a
2D platform game ? Or maybe you want to add
particle effects to your game? Cocos2d-x provides Node objects to help you with this!
Tilemap
TileMaps are maps consisting of tiles (tiles). Each tile can have independent behavior.
TileMaps are stored in an
XML -based map format called
TMX .
TMX was originally designed for tile maps, but it is also suitable for creating common game levels thanks to the support of various types of objects. TMX objects are easy to create:
Tile cards can have several layers defined by a z-order. You can access a specific layer by its name:
Each tile has a unique position and ID. This makes it very easy to choose the desired tile. You can get any by its id (id):
Example of layers in the tile map:
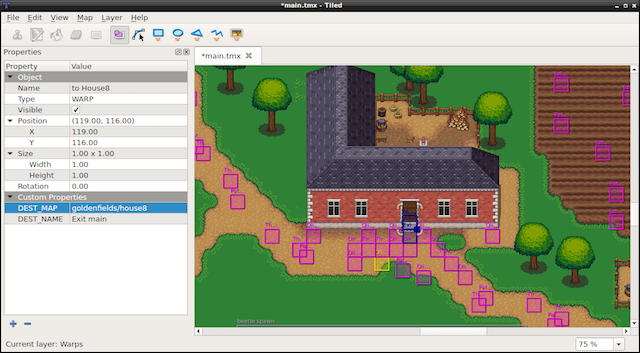

How to make a map of tiles? There are many tools that do this.
Tiled is a popular utility. He is actively developing and has a great community. Screenshots from above - real projects in
Tiled .
Particle effects
Maybe your game needs effects like fire, spells or explosions? How do you implement such complex effects? Is it possible? Of course! Use
particle systems .
Particle system is a computer graphics technology that uses a large number of very small sprites or other graphic elements to simulate fuzzy objects that are otherwise very difficult to reproduce using conventional rendering methods. Some real examples may include very chaotic systems: natural phenomena or processes caused by chemical reactions. Here are a couple of examples
of particle effects :


Particle Effecting Tools
Although you can create effects manually, specifying each property to your taste, for this there are several third-party utilities. Some of the tools are:
- Particle Designer : a very powerful effect editor for Mac.
- V-play particle editor : Cross-platform particle system editor for Cocos2d-x.
- Particle2dx : online particle effects designer.
These output tools provide a
.plist file that you can read using Cocos2d-x to use your creation inside your game. As with all the other classes we worked with, we use the
create () method:
Inline particle effects
Are you ready to add effects to your game? Is it difficult for you to create your own effects? For simplicity, there are a number of built-in particle effects from which you can choose. Take a look at this list:
- ParticleFire: point system of particles. Uses gravity mode.
- ParticleFireworks: point system of particles. Uses gravity mode.
- ParticleSun: point particle system: Uses gravity mode.
- ParticleGalaxy: point system of particles. Uses gravity mode
- ParticleFlower: point particle system. Uses gravity mode
- ParticleMeteor: point system of particles. Uses gravity mode
- ParticleSpiral: point system of particles. Uses gravity mode
- ParticleExplosion: point system of particles. Uses gravity mode
- ParticleSmoke: point system of particles. Uses gravity mode
- ParticleShow: point system of particles. Uses gravity mode
- ParticleRain: a point particle system. Uses gravity mode
Using, for example,
ParticleFireworks , you can easily create inline effects:
auto emitter = ParticleFireworks::create(); addChild(emitter, 10);
The result is an effect that looks something like this:

But what if your
particle effect is not exactly what you would like it to be? Yes, you can manually change it! Take the same firework and change its properties:
auto emitter = ParticleFireworks::create();
Parallax
Parallax - is a unique type of
nodes that simulates
parallax scrolling .
Couple .. what? Yes, parallax. Simply put, you can view a
parallax node as a
special effect that creates a different appearance of the position and direction of an object, depending on the point of view. We meet with parallax all the time when we watch TV or shoot something on camera. You can recall many games that work this way. Super Mario Bros is a classic example.
Parallax nodes can be moved by performing a
sequence , as well as manually using the mouse, touching, or using the keyboard.
Parallax nodes are a bit more complicated than regular nodes. Why? Because they require several nodes for their work.
Parallax nodes cannot work by themselves. You need at least 2
nodes to work. As usual, in Cocos2d-x,
parallax nodes are easy to create:
We need several
Node objects, they are also easily added:
Okay, looks familiar, right? Pay attention to a few things! Each
node added is assigned a unique z-order so that they overlap each other. Also note the additional two parameters of type
Vec2 in the
addChild () call. This is the
ratio and
offset . These parameters can be considered as the ratio of the speed of internal nodes to the speed of the parent node.
It is difficult to show the
parallax node in the text, but you can try to write this code yourself, or run a test project -
Programmer Guide Sample to see it in action!
Next article:
Cocos2d-x - Event Manager