
Modern developers are engaged in building cross-platform applications, their maintenance and deployment. To facilitate their hard work, a new Microsoft framework, ASP.NET Core, was created. Now you have a wide variety of open source libraries at your disposal; moreover, the framework itself is an open source product.
How to master all the new features provided by ASP.NET Core? The authors explain the solution of specific problems on the example of the fictional company Alpine Ski House. Each chapter is preceded by a short story about the problem faced by the development team, and how they overcome this problem. You have to get acquainted with the architecture of applications, tools for deploying and designing applications for working in the cloud and many others.
This book covers the first application development sprints at the fictional company Alpine Ski House. Each chapter is preceded by a short story about the problem faced by the development team, and how they are going to overcome it. Despite the presence of an element of fiction, the book covers not only the ASP.NET Core MVC functionality, but also the tools used by developers to build, maintain, and deploy applications.
In addition to the stories and technical information about ASP.NET Core MVC, the book covers the new version of the Entity Framework, package management systems and peripheral technologies used by modern web developers. In addition to the theoretical educational material, the accompanying project is also attached to the book - the same one that is developed by programmers from Alpine Ski House.
')
Who is this book written for?
The book will guide the programmer through all stages of creating a new application based on ASP.NET Core and providing access to it via the Internet. Many programmers are still far from the world of web development or have only slightly come into contact with it through WebForms, despite the wide range of software tools currently available. The book will help the reader to master the skills necessary for the design of modern applications on the rapidly developing framework. You will learn about application architecture, deployment tools, and cloud application design.
This book will not suit you if ...
This book may not be suitable for you if you are an experienced ASP.NET MVC developer who closely followed or directly participated in the development of ASP.NET Core MVC.
Book structure
The book uses an unusual approach: it takes developers to individual sprints during the development of the application. It considers not only technology, but also the process of error recovery and the reaction of developers to feedback from users. We will start with a blank sheet and finish with a full-fledged workable product.
The book is divided into four parts:
• Part I “Sprint One: The Alpine Ski House app” introduces the reader to the core of the situation: it describes the app used in the book and the fictional characters in the story.
• Part II “Sprint Two: Journey 1000 steps in length” is devoted to the main functional aspects of the application and the configuration of the pipeline through which the deployment will occur on the fly, and the process will be understandable for the entire development team.
• Part III, Sprint Three: In the Mouth of the Beast, focuses on the basic functionality necessary to start using the test application in real business processes. In particular, it deals with working with data through the Entity Framework Core, creating representations based on Razor, configuring and logging, security and rights management, and finally, dependency injection.
• Part IV “Sprint Four: The Finish Line” describes JavaScript and dependency management, and also develops some previous themes.
At the end of the book, such important topics as testing, refactoring, and adding extensions are covered.
Excerpt Refactoring and improving the quality of the code
Few developers are satisfied with the code they wrote a year ago. An ordinary joke in many programming workshops: the programmer exclaims "Who wrote this garbage?", Looks into the story and finds out that it was him. This is actually a great opportunity. When you start writing code, often you just try to understand the subject area, the programming problem and the structure of the application that you have to write. The amount of information that the mind can learn at one time is limited, and a large number of "moving parts" often leads to errors in the structure or functionality of the code. Returning and fixing the old code a few months later, when your understanding of the application has improved, is the greatest luxury.
This chapter discusses the idea of ​​refactoring and the possibility of improving the functionality of an application without affecting its performance.
What is refactoring?
The term “refactoring” was proposed in the early 1990s by William Opdyke and Ralph Johnson. Yes, there is a book written by the "Gang of Four" 1. Refactoring is understood as a change in the internal structure of a block of code, as a result of which the code becomes clearer, easier to test, better adapts to changes, and its external functionality does not change. If we imagine a function in the form of a “black box” that receives input data and provides output data, changing the internal structure of the “black box” does not play a special role if the input and output remain unchanged (Fig. 23.1).
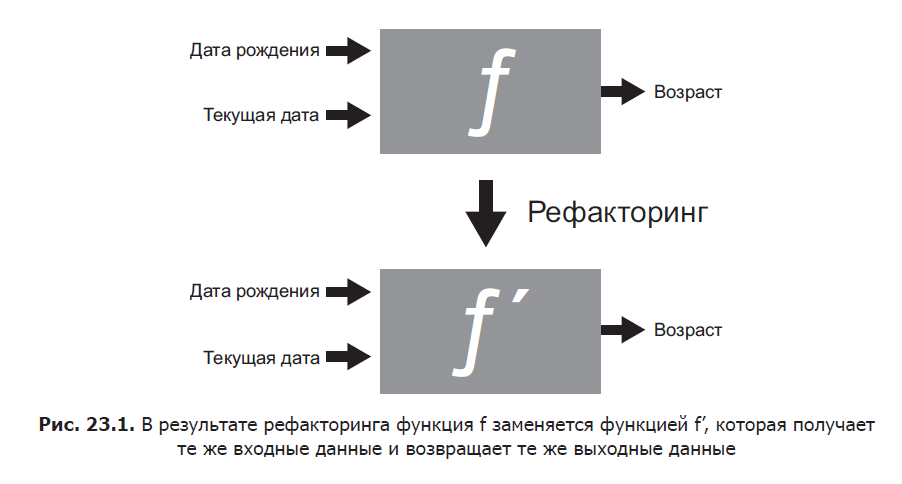
This observation allows you to replace the code in the application while preserving the functionality and improving the overall quality of the application. And although we use the term “function” as the designation of a block of code, its scope is not limited to the internal structure of the function. The whole essence of object-oriented programming is the widespread introduction of abstractions to protect against the disclosure of implementation details. There are many constructs that can be considered as “black box” for refactoring purposes. The first candidate are classes. A class implementation is irrelevant if the input and output data remain unchanged, so we can change its internal mechanisms as we see fit. Namespaces do not provide the same level of protection as classes, but can also be considered candidates for substitution. Finally, in the world of microservices, the entire application can be replaced by another application, and such a replacement will also become part of refactoring.
Consider a service that is responsible for sending email on the mailing list. Input can be a list of recipients and the message to be sent. The output as such is taken here by the result: the receipt of the message by all the recipients from the list of recipients (Fig. 23.2).
It doesn't matter how microservice sends messages: using Sendgrid or from its own server. In any case, the result remains the same. In this case, the replacement of microservice can be considered as refactoring.
Programmers usually call refactoring anything that improves code. Eliminate the extra parameter of the method? Added logging in the insidious method to provide improved support for DevOps? Although the quality of the code or the quality of the application in both cases improves, none of them can formally be considered as refactoring, because they change the functionality of the application. Full adherence to the definition of refactoring requires that both input (including the composition of parameters) and output (including log messages) remain unchanged.
Code quality assessment
If the developer is engaged in refactoring to improve the quality of the code, of course, there must be some criterion for evaluating the quality of the code. It would be great to have a tool for assessing the quality of a code — a perfect metric that can represent all aspects of code quality in one number. Unfortunately, this metric does not exist. Over the years, countless quality metrics have been offered, from centrifugal and centripetal adhesions to estimating the number of errors in a line of code and the degree of test coverage of a code. Choosing a set of code quality metrics is a topic that in itself would deserve a whole book.
However, it will be useful to highlight a few simple metrics to assess the quality of the code:
• Does the code do what it is supposed to do? At first glance, it is obvious, but this metric is often forgotten. It can be estimated by the number of reported errors per thousand lines of code or by the number of errors per class. Some developers use this metric globally, comparing the relative quality of two projects by the number of defects found. However, this approach is unproductive, because two projects never solve one problem. Is it possible to expect that the system for creating and delivering electronic greeting cards will have the same degree of complexity or number of errors as the insulin dispenser control system? Of course not. This metric, like most others, simply helps to know if a group and product is improving over time.
• Does the code satisfy its non-functional requirements? These are requirements that are not related to the input or output of a function (such as, for example, the performance of a code fragment or its security).
• How clear is the code? The ideal code does not exist at all, and sooner or later someone will need to update it due to a detected error or changed requirements. This "someone" can even be the author of the code, that is, you! Take a look at the following snippet:
for (var i = 1; i < parts.length; i++) { root[parts[i]] = root[parts[i]] || (root[parts[i]] = {}); root = root[parts[i]]; }
Perhaps this is an efficient and smart code, but it is almost impossible to understand it. Finding an objective metric of intelligibility or readability is quite difficult. This metric is not so much a number as the embodiment of the consensus opinion of the developers regarding the ease of maintenance. In groups of normal people who are not burdened with ambitions, serious differences rarely arise about how well the code is read. Code review is a great tool for detecting obscure parts of code.
Another simple but practical metric, cyclomatic complexity, estimates the complexity of functions by the number of code branches in the method. The method with one if command contains two possible branches of the code, which means that its cyclomatic complexity is 2 (Fig. 23.3).
• Is the code able to respond easily to changes in requirements? Again, this metric cannot be expressed with a prime number. Rather, this feeling arises in a group of experienced developers. According to the sour expression on the face of the developer, when major changes are assumed in his code, it is possible to determine with confidence whether this goal has been achieved.
These four simple metrics will be a good starting point for deciding which parts of the application may need refactoring. You will probably be able to discover and add new metrics during the growth and development of the application.
When you have several metrics at your disposal, it is important to make sure that the application is not optimized by the error metric. People tend to deceive the system, consciously or not. If you select a performance metric that provides the fastest return from a function, but does not add a metric that establishes a reasonable limit on memory costs, you are tempted to optimize the function so that it consumes memory in exchange for computing resources. Think about whether this is really what you need, or in fact the ultimate goal is balance. Analyze the metrics and make sure that the optimization is not going in the wrong direction, and if it does, update the set of metrics.
About the authors
James Chambers, a five-time Microsoft MVP in technology development, is currently developing using ASP.NET Core and MVC Framework on Azure and AWS. He is an independent consultant, teacher and active blogger, as well as a member of several open source projects.
David Paquette, a four-time Microsoft MVP status holder, is a software developer and independent consultant. He has extensive experience using .NET for building Windows and web applications, deep technical knowledge and a passion for quality user interfaces.
Simon Timms, Microsoft MVP status holder for many years, is a community organizer, blogger, developer, and independent consultant. His technological interests are very diverse, he is interested in everything - from distributed systems to modern JavaScript frameworks. Possessing good preparation in the field of development and in the organization of current work, he often drives his groups mad, participating in all stages, from building and developing to server maintenance.
»More information about the book can be found on
the publisher's website.»
Table of Contents»
ExcerptFor Habrozhiteley a 20% discount on the coupon -
ASP.NET Core